Programmers use different data types to store different kinds of values. For instance, they use strings to store text-based data and integers to store whole numbers.
When you are writing code, you may decide that you want to convert a value’s data type to another data type. One of the most common type conversions performed in Java is converting a string to an integer. That’s where the parseInt() and valueOf() methods come in.
You can use the Java parseInt() and valueOf() methods to convert a string to an integer. In this tutorial, we will discuss—with examples—how to do so.
Java Data Types
Java, like any other programming language, uses different data types to classify the type of data a value holds. True/false values, for instance, are stored as booleans, and text is stored as a string.
This is an important feature in programming because the data type of a value will determine how that value can be manipulated. For instance, you can perform mathematical operations on integers and real numbers but not on strings.
When you’re programming, you may encounter a situation where you need to convert a string into an integer. For instance, if you want to perform a mathematical operation on a number that is stored as a string, you would need to convert the data type of the value.
Java String to Integer: Using parseInt()
You can use the parseInt() method to perform a string to integer conversion in Java. parseInt() accepts one parameter: the string value you want to convert into an integer.
The syntax for parseInt() is as follows:
Integer.parseInt(value);
Suppose we are writing a program for a theme park that checks the age of a customer on admission to certain attractions. The customer’s age is stored as a string, but we want to convert it to an integer so we can compare it to the appropriate age minimum. We could convert the customer’s age (15 in this example) to an integer using this code:
class ConvertAge { public static void main(String[] args) { String age = "15"; Integer integer_age = Integer.parseInt(age); System.out.println("This customer's age is: " + integer_age); } }
Our code returns:
This customer’s age is: 15
Let’s break down our code. On the first line in our ConvertAge
class, we declare a string—called age
—that stores the age of our customer.
Then we use the parseInt() method to parse the string value and convert it to an integer. We assign the new integer value to the variable integer_age
. Finally, we print out a message stating: “This customer’s age is: ”, followed by the age of the customer.
We can check the type of our data by using the instanceof
keyword. instanceof returns a boolean value (true
or false
) depending on whether the value we are checking holds a particular data type. The following code, when added to the end of our previous program, allows us to verify that integer_age
is an integer:
…
System.out.println(“Is age an integer? ” + age instanceof Integer);
…
Our code returns:
This customer’s age is: 15
Is age an integer? true
In our code, we use age instanceof Integer to check whether the age variable is an integer. age is an Integer, so the instanceof keyword returns: true.
Java String to Integer: Using valueOf()
You can also use the valueOf() method to convert a string to an integer in Java. valueOf() accepts one parameter: the value you want to convert to an integer.
Here’s the syntax for the valueOf() method:
Integer.valueOf(value);
Let’s walk through an example to demonstrate how the valueOf() method works. Suppose we are writing a program that stores the quantities of the Columbian and Italian roast coffee beans sold in a coffee shop. Our program currently stores these quantities as a string, but we want to convert them into integers. We could use the following code to accomplish this task:
class ConvertQuantities { public static void main(String[] args) { String columbian = "15"; String italian_roast = "19"; Integer columbian_integer = Integer.valueOf(columbian); Integer italian_roast_integer = Integer.valueOf(italian_roast); System.out.println("Quantity of Columbian coffee beans: " + columbian_integer); System.out.println("Quantity of Italian roast coffee beans: " + italian_roast_integer); } }
Our code returns:
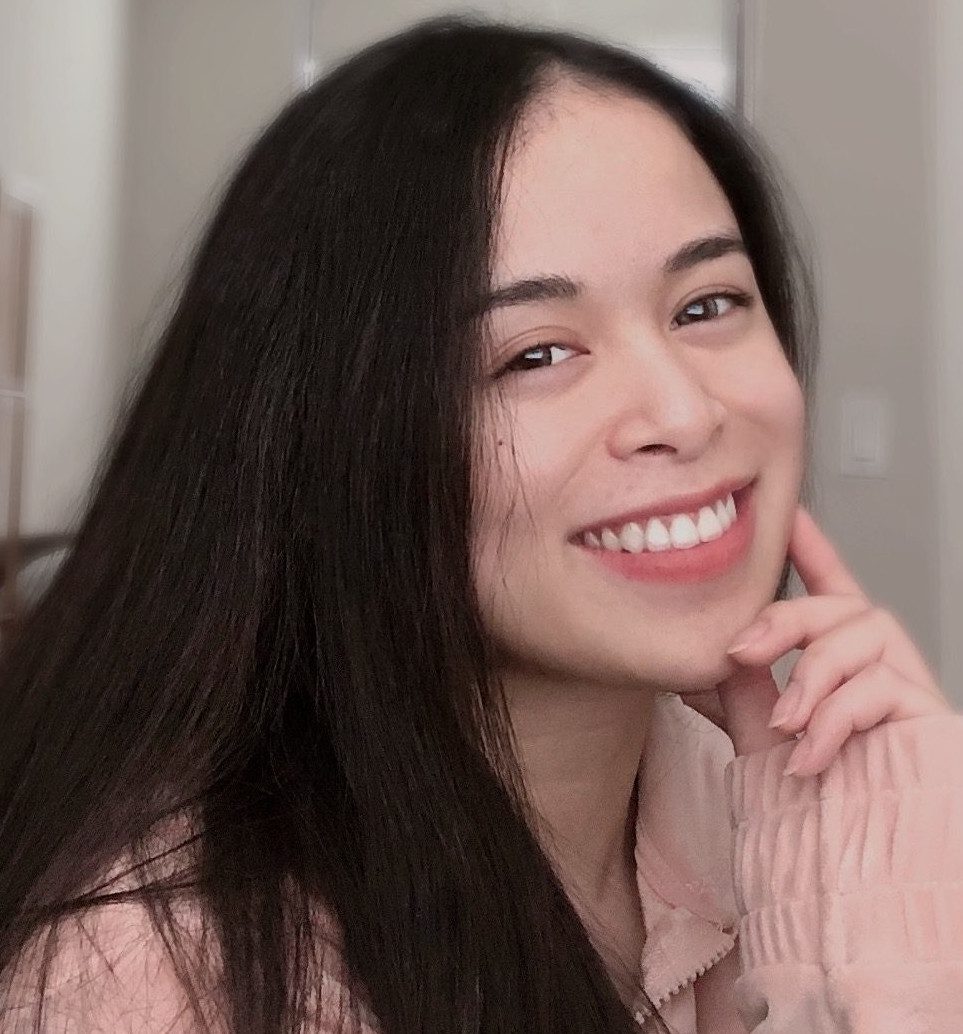
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Quantity of Columbian coffee beans: 15
Quantity of Italian roast coffee beans: 19
Let’s break down our code. On the first line in our ConvertQuantities class, we declare a variable called columbian and assign it the value 15. Then we declare a variable called italian_roast
and assign it the value 19.
We then use the valueOf() method to convert the value of columbian to an integer and assign that integer to the variable columbian_integer
.
We also use the valueOf() method to convert the value of italian_roast
to an integer and assign that integer value to the variable italian_roast_integer
.
Then, we print out the quantities of both beans, using the integer values of each bean.
Conclusion
There are two ways to convert a string to an integer in Java: the parseInt() method and the valueOf() method. This tutorial discussed the basics of data types in Java and how you can use both methods to convert a string to an integer. We also walked through an example of each method in action.
Now you’re equipped with the knowledge you need to convert strings to integers in Java like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.