The Java string replace()
method will replace a character or substring with another character or string. The syntax for the replace()
method is string_name.replace(old_string, new_string)
with old_string being the substring you’d like to replace and new_string being the substring that will take its place.
When you’re working with a string in Java, you may encounter a situation where you want to replace a specific character in that string with another character. For instance, if you are creating a username generation program, you may want to replace certain letters with other characters to give a user a more random username.
That’s where the String replace() method comes in. The Java replace() method is used to replace all occurrences of a particular character or substring in a string with another character or substring.
This tutorial will discuss how to use the String replace() method in Java and walk through an example of the method being used in a program.
Java Strings
Strings are used to store text-based data in programming. Strings can contain letters, numbers, symbols, and whitespaces, and can consist of zero or more characters.
In Java, strings are declared as a sequence of characters enclosed within double quotes. Here’s an example of a Java string:
String bread = “Seeded”;
In this example, we have assigned the string value Seeded
to a variable called bread
.
Strings are a useful data type because they allow developers to interact with text-based data that can be manipulated. Strings can be reversed, replaced, and otherwise changed using the string methods offered by Java.
When you’re working with a string, you may decide that you want to change a specific character in the string to another character. We can use the replace() method to perform such a change.
Java String replace
The Java string replace() method is used to replace all instances of a particular character or set of characters in a string with a replacement string. So, the method could be used to replace every s
with a d
in a string, or every “pop
” with “pup
”.
The syntax for the Java string replace() method is as follows:
string_name.replace(old_string, new_string);
The replace() method takes in two required parameters:
- old_char is the character or substring you want to replace with a new character or substring.
- new_char is the character or substring with which you want to replace all instances of
old_char
.
Now, replace() will replace every instance of a character or substring with a new character or substring and return a new string. So, if you only want to change one specific character in a string, the replace() method should not be used.
Additionally, replace() does not change the original string. Instead, the method returns a copy of the string with the replaced values. replace() is also case sensitive.
String replace Examples
Let’s walk through a few examples of the replace() method in action.
Replace Substring
Suppose we are building an app for a restaurant that stores the names and prices of various sandwiches on the menu. The chef has informed us that he wants to update the name of the Ham
sandwich to Ham Deluxe
to reflect the fact that new ingredients have been added to the sandwich. We could make this change using the following code:
Aclass ReplaceSandwiches { public static void main(String[] args) { String ham_entry = "Ham: $3.00."; String new_ham_entry = ham_entry.replace("Ham", "Ham Deluxe"); System.out.println(new_ham_entry); } }
Our code returns the following:
Ham Deluxe: $3.00.
Let’s break down our code. Firstly we define a class called ReplaceSandwiches, which stores the code for our program. Then we declare a variable called ham_entry
which stores the name of the ham sandwich and its price.
On the next line, we use the replace() method to replace the word Ham
with Ham Deluxe
in the ham_entry
string. The result of the replace() method is assigned to the variable new_ham_entry
. Finally, our program prints the contents of new_ham_entry
to the console.
Now we have the updated record for the ham sandwich.
Replace Character
Additionally, the replace() method can be used to replace an individual character with another character. For instance, suppose we misspelled the word Ham
in our string. Instead of appearing as Ham
, we typed in Hom
. We know there is only one o
in our string, and we want to replace it with an a
.
We could use the following code to correct our mistake:
class FixSandwich { public static void main(String[] args) { String ham_entry = "Hom Deluxe: $3.00."; String new_ham_entry = ham_entry.replace("o", "a"); System.out.println(new_ham_entry); } }
Our code returns:
Ham Deluxe: $3.00.
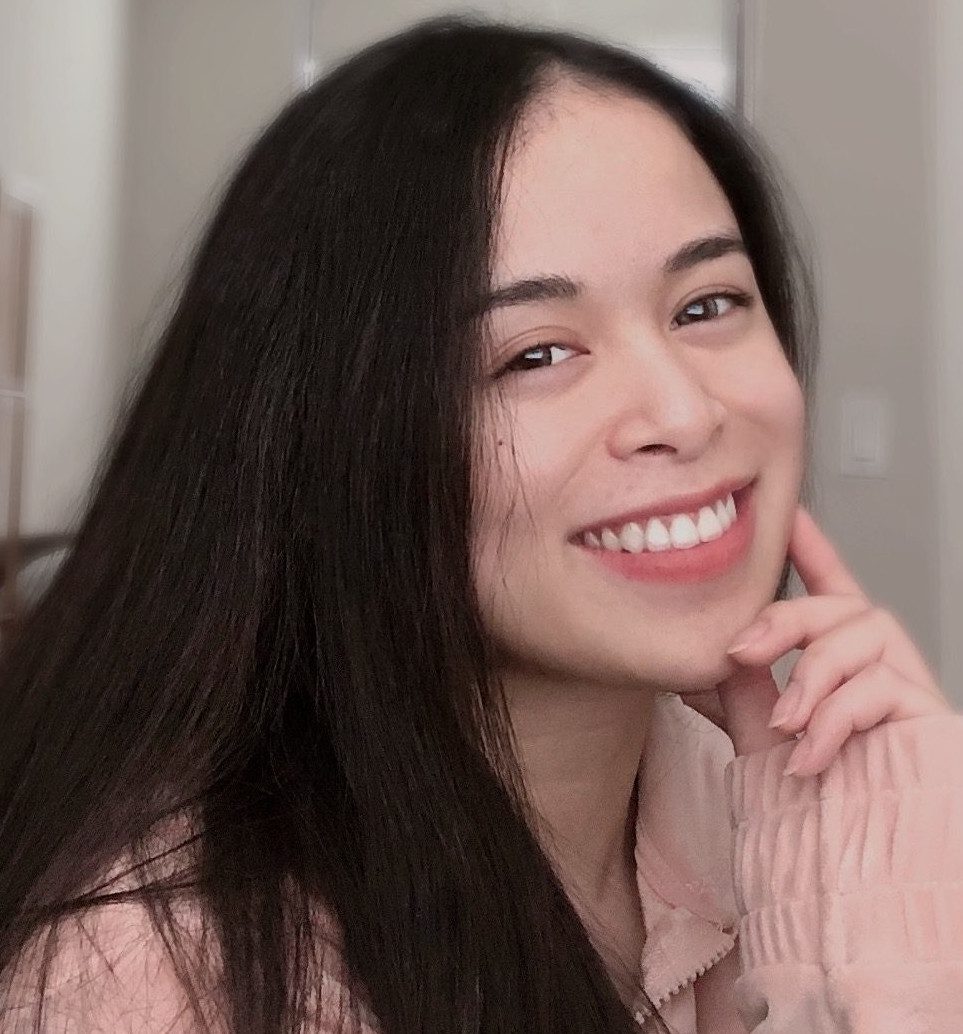
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Our code works in the same way as our first example, but instead of replacing a substring of characters, we instead replace an individual character. In this case, we replaced o
with a
.
Replace Multiple Instances
The replace() method will replace every instance of a character or substring with a new character or substring. In the above examples, we only replaced one instance of a substring and a character because there was only one instance in our string. But if multiple instances of a character or substring were present, they would all be replaced.
Suppose we have a string that stores the text that will appear on the banner, notifying customers that the restaurant with which we are working has a new menu. The banner appears as follows:
“MENU | Come see the new menu! | MENU”
The restaurant owner has decided he wants to replace the word “MENU” with his restaurant’s name, Sal’s Kitchen
. We could do this using the following code:
class ChangeBanner { public static void main(String[] args) { String old_banner = "MENU | Come see the new menu! | MENU"; String new_banner = ham_entry.replace("MENU", "Sal's Kitchen"); System.out.println(new_banner); } }
Our code returns:
Sal’s Kitchen | Come see the new menu! | Sal’s Kitchen
Our code works in the same way as our previous examples. However, because our initial string includes the word MENU
twice, the replace() method replaces both instances. It’s worth noting that the lowercase word menu
appears in our string too, but because replace() is case sensitive, it is not replaced.
Conclusion
The replace()
method is used in Java to replace every instance of a particular character or substring with another character or substring.
This tutorial discussed how to use replace()
to replace individual characters, substrings, and multiple instances of a character or a substring. We also explored a few step-by-step examples of the replace()
method in action.
You’re now equipped with the information you need to replace the contents of a string using replace()
in a Java program like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.