Strings are a data type used to store text-based data in Java. When you’re working with strings, you may encounter a scenario where you want to compare two strings with each other.
That’s where the Java string equals()
and equalsIgnoreCase()
methods come in. The equals()
method is used to check whether two strings are equal, and equalsIgnoreCase()
is used to compare whether two strings are equal while ignoring their cases.
This tutorial will walk through, with examples, how to use the string equals()
and equalsIgnoreCase()
methods in Java. By the end of reading this article, you’ll be an expert at comparing strings using these methods in Java.
Java Strings
Strings are used to store text and can include letters, numbers, symbols, and whitespaces. For instance, a string may include the name of a customer at a tailor’s store, or the address of a supplier for the tailor store.
In Java, strings are declared as a sequence of characters enclosed between quotation marks. Here’s an example of a Java string:
String favoriteCoffeeShop = "Central Perk";
In this example, we have declared a variable called favoriteCoffeeShop
and assigned it the value Central Perk
.
Java string objects are immutable, which means once a string is created, we need to use the equals()
method to compare it with another string. Now that we know the basics of strings, we can go on to discuss the string equals()
and equalsIgnoreCase()
methods.
Java String equals
The Java string equals()
method is used to compare objects and check whether the content of two strings are equal.
equals()
accepts one parameter: the string you want to compare to another string. Here’s the syntax for the string equals()
method:
stringName.equals(string2Name);
Let’s break this down:
- stringName is the name of the original string to which you want to compare string2Name
- equals() is the method used to compare stringName with string2Name.
- string2Name is the string which you want to compare to stringName.
The equals()
method returns a boolean value based on whether the strings are equal. So, if the two strings are equal, the value true is returned; otherwise, false is returned.
The string equals()
method is case sensitive. So, if two strings contain the same characters but use different cases, the equals()
method will return false.
Java String equals Example
Suppose we are operating a hotel and we are writing a program to streamline our check-in phase. When a customer checks in, they should give their name and booking reference. We will compare the booking reference the customer gives to the one stored with their name on file, to verify the identity of the customer.
We could use this code to compare the booking reference on file with the booking reference provided by the customer:
class Main { public static void main(String args[]) { String onFileBookingReference = "#ST2920198"; String customerGivenBookingReference = "#ST2920198"; boolean areEqual = onFileBookingReference.equals(customerGivenBookingReference); if (areEqual == true) { System.out.println("This booking is confirmed."); } else { System.out.println("The booking reference provided does not match the one on-file."); } } }
Our code returns:
This booking is confirmed.
Let’s break down our code:
- We declare a variable called
onFileBookingReference
which stores the booking reference associated with a customer’s name. - We declare a variable called
customerGivenBookingReference
which stores the booking reference given by the customer to the clerk at the check-in desk. - We use the
equals()
method to check whetheronFileBookingReference
is equal tocustomerGivenBookingReference
, and assign the result of the method to the areEqual variable. - An
if
statement is used to check whetherareEqual
is equal to true.- If areEqual is equal to true, the message
This booking is confirmed
. is printed to the console. - If areEqual is equal to false, the message
The booking reference provided does not match the one on-file
. is printed to the console.
- If areEqual is equal to true, the message
In this example, the customer has given the correct booking reference, so our program confirmed their booking.
Java String equalsIgnoreCase
The String equalsIgnoreCase()
method is used to compare two strings while ignoring their case. equalsIgnoreCase()
uses the same syntax as the equals()
method, which is as follows:
stringName.equalsIgnoreCase(string2Name);
Now, let’s walk through an example to illustrate this method in action.
Suppose we want to write a program that checks whether a customer’s name matches the one associated with the booking reference given. This check should be case insensitive to ensure that a capitalization error does not cause a booking to be lost by the check-in clerk.
We could use the following code to compare a customer’s name with the one linked to their booking reference:
class Main { public static void main(String args[]) { String onFileName = "Gregory Lamont"; String customerName = "gregory lamont"; boolean areEqual = onFileName.equalsIgnoreCase(customerName); if (areEqual == true) { System.out.println("This booking is confirmed."); } else { System.out.println("The customer name provided does not match the one on-file."); } } } When we run our code, the following response is returned: This booking is confirmed.
When we run our code, the following response is returned:
This booking is confirmed.
The customer name stored on-file is Gregory Lamont
, but the customer name entered by the clerk was gregory lamont
. If we used the equals()
method, these strings would not be considered the same because their cases are different.
However, in this example, we used the equalsIgnoreCase()
method, which ignores the cases in which the string’s characters are written.
Conclusion
The String equals()
method is used to check if two strings are exactly equal. The equalsIgnoreCase()
method is used to check if two strings are equal, irrespective of their cases.
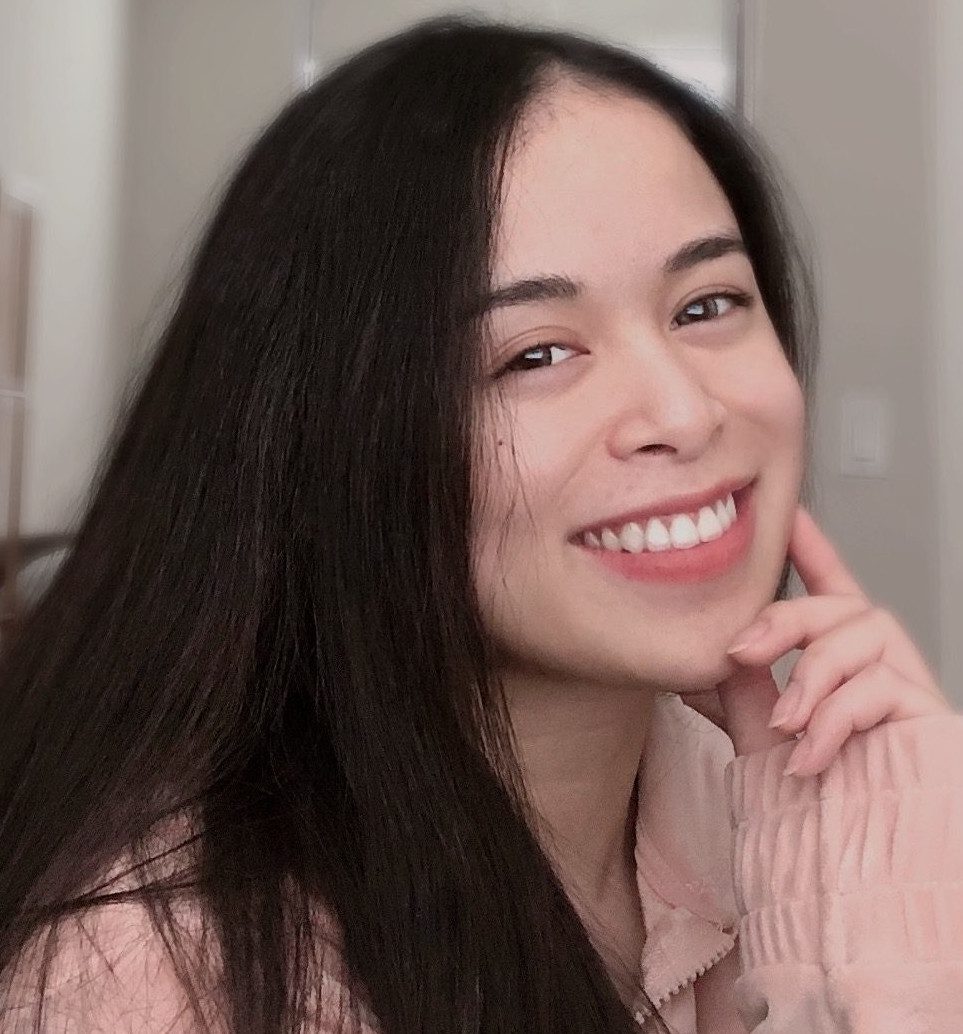
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
This tutorial walked through how to use the string equals()
and equalsIgnoreCase()
methods in Java, with reference to two examples. Now you’re ready to start comparing strings in Java using equals()
and equalsIgnoreCase()
like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.