When you think of string, you may see a cat playing around with a ball of yarn. It’s true that a ball or yarn is string, but when programmers use the word string they mean something different.
In programming, a string is a sequence of one or more characters. Given how important text-based data is to our everyday interactions with computers, it will come at no surprise that the string is a crucial part of programming.
In this guide, we’re going to discuss how to create a string, how to concatenate a string, and how to store the value of a string in a variable. We’ll walk through a few examples to help guide you along the way.
Creating a Java String
A string is used to store text in Java. Whereas the char
data type stores individual characters, strings can hold as many characters as you want. Strings are sequences of bytes.
Strings are defined between double quotes. This is unlike languages like Python or JavaScript, which both accept both single and double quotes. Here’s an example of a string in Java:
“This is an example string.”
You can print out the contents of a string using the println()
function:
System.out.println("This is an example string.");
Our code returns: This is an example string
. We’ve constructed a new string. Now that you’re aware of how to declare a string, we can talk about how to work with them in your code.
String Concatenation
Concatenation, what a long word. It’s definition is quite simple: it means combining two strings to create a new string. You can use the +
operator (plus sign) to concatenate two strings.
Let’s say that we have two strings: William and Carter. The first string is a forename and the second string is a surname. We could combine them using the +
operator:
String fullname = "William" + "Carter"; System.out.println(fullname);
Our code outputs: WilliamCarter
. Wait, what happened? Why is there not a space between these two words?
By default, there is no whitespace added between concatenated strings. If we want a space to appear, we’ll need to add it after the name William
:
String fullname = "William " + "Carter"; System.out.println(fullname);
When we run our program, this is returned: William Carter
.
Calculating the Length of a String
How do you calculate the length of a string? What a good question. Luckily for us, we don’t need to write any complex functions to do it.
Java already has a built-in function that we can use to calculate the length of a string: length()
. It’s a convenient name for a method!
We’re going to create a program that calculates the length of the names of two people in a fourth grade class. Create a new Java file and paste the following code into your main class:
String name1 = "William Carter"; String name2 = "Helena Ohara"; int name1length = name1.length() - 1; int name2length = name2.length() - 1; System.out.println(name1 + "'s name is " + name1length + " letters long."); System.out.println(name2 + "'s name is " + name2length + " letters long.");
Let’s run our code:
William Carter's name is 13 letters long. Helena Ohara's name is 11 letters long.
We’ve defined two variables which store our student’s names: name1 and name2. We’ve then used the length()
method to calculate the length of each of these strings. We subtracted 1 from each of the names’ lengths to account for the space in the middle.
Then, we printed out a message informing us of the length of each name.
Find a String within a String
There may be times when you want to find a string within another string. That’s where the indexOf()
method comes in handy. This method returns the position of the first occurrence of a particular string of text within a string.
Before we discuss how it works, we’ll have to briefly talk about index
. In Java, strings are indexed using index numbers. This means that every character in a string is assigned its own number, which we can use to access each item individually.
Here’s a breakdown of the index within the string “William Carter”:
W | i | l | l | i | a | m | C | a | r | t | e | r | |
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 |
Notice that all characters in our string are assigned an index number, including whitespaces. In addition, notice how we start counting from 0. This is because strings are indexed starting at 0.
Let’s say we want to find out where the string Carter
appears in this string. We could do so using the indexOf()
method like this:
String name = "William Carter"; int position = name.indexOf("Carter"); System.out.println("Carter appears starting at index value " + position);
Our code returns: Carter appears starting at index value 8. indexOf()
returns the index position at which a character appears.
Check Whether Two Strings Are Equal
Comparing strings comes up all the time in programming. Think about when you enter a password into a login form. What does the application do? It compares the password you have entered with the one the application has stored in its database.
Let’s say that we want to write a program that checks whether a user’s password is correct. Open up a new Java file and paste in the following code into your main program:
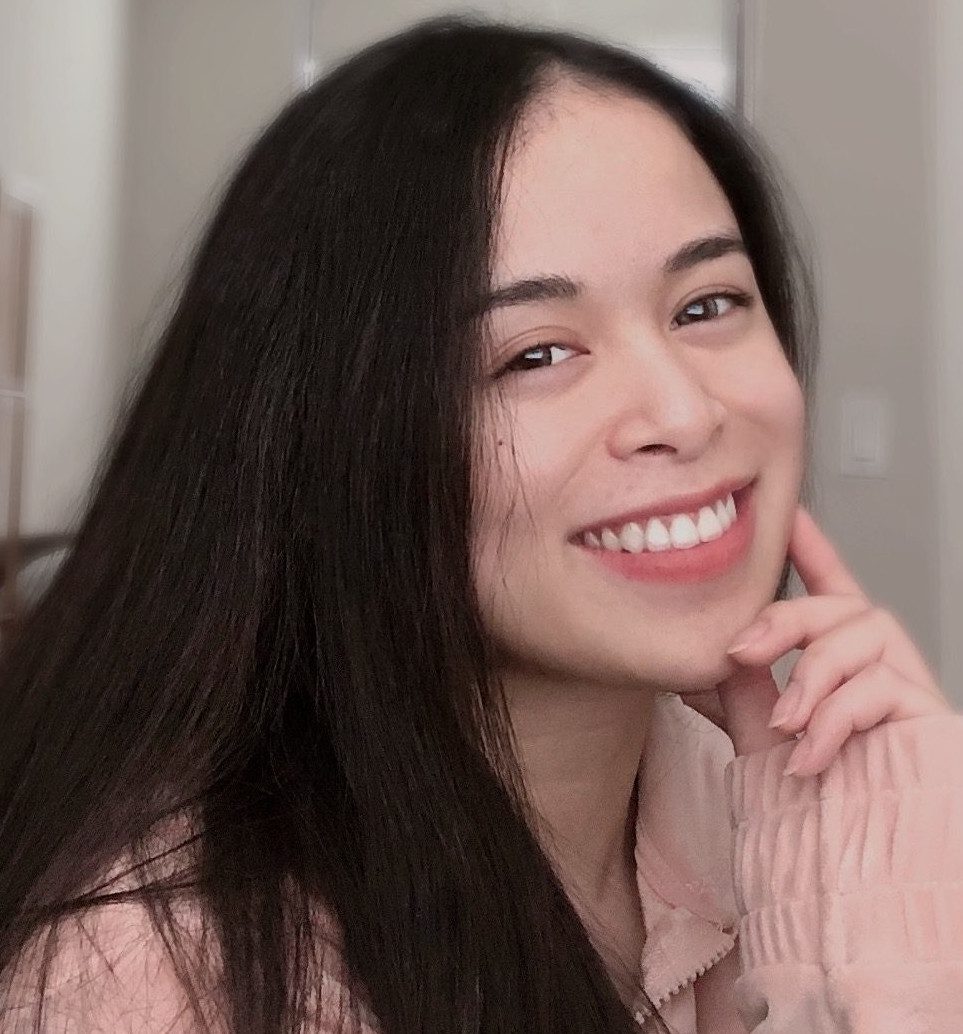
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
String entered_password = "Test123"; String saved_password = "Test12345"; boolean compare_passwords = entered_password.equals(saved_password); System.out.println("Does the entered password match the saved password? " + compare_passwords);
Our code returns:
Does the entered password match the saved password? false
Our program checks to see whether the two strings we have specified – entered_password
and saved_password
are equal. We use the equals()
method to perform this check. This method returns true if the strings are equal; otherwise, it returns false.
Let’s change the value of entered_password
to be equal to saved_password
:
String entered_password = "Test12345"; String saved_password = "Test12345"; ...
When we run this code, our program returns:
Does the entered password match the saved password? true
Both the strings we have specified match, which means that our equals()
method evaluates to true.
Special Characters
Strings must be written within double quotes. This sounds like no big deal until you want to include a double quote in your program. This will return an error.
The way to resolve this error is to use the backslash (\) character. This will turn any character that immediately follows the string into a special character. Consider this example:
String statement = "Your order for \"Chocolate Chip Cookie x2\" was processed.";
This string will work in our program because we’ve specified a backslash before each of the double quotes in our string. This string returns: Your order for Chocolate Chip Cookie x2 was processed.
Now we’ve got another problem: what if you want to use a backslash in your string? To do so, you can write two backslashes side-by-side:
String order_reference = "Your order reference is 202\\303."
This returns the string: Your order reference is 202\303."
There are a number of other special characters that you can use in Java. One of the most useful that you’ll encounter is one called \n, or new line. This character creates a new line in a string:
String order_reference = "Your order reference is: \n 202\\303."
Our string is equal to:
Your order reference is:
202\303
The string has been split up onto two lines because we specified a new line character after the is:
part of our string.
Conclusion
Strings allow you to work with text-based data in your programs. A string can hold any number of characters, including no characters at all.
Using string methods such as indexOf()
and equals()
, you can manipulate the contents of a string in Java. Now you’re ready to start working with strings in Java like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.