How to Use the Java Static Keyword
When you’re working with classes in Java, you usually need to create an instance of the class before you can access its members. However, you may encounter a situation where you want to define a class member without creating an instance of a class.
That’s where the Java static keyword comes in. The Java static keyword is used to define class members that you can access without creating an instance of a class.
This tutorial will discuss, with examples, the basics of the Java static keyword and how to use it in your Java programs.
Java Classes
In Java, classes are blueprints for an object. For example, a class called OrderCoffee could include all the functions related to ordering a coffee, such as placing an order, changing an existing order, and adding new items to an order.
We can then create objects that are instances of that class. For example, an object called CoffeeOrder12 could use the blueprint from the CoffeeOrder class to store data for an individual coffee order. The CoffeeOrder12 object would have access to all the functions and methods declared within the CoffeeOrder class. See Image 1 for a visual representation of these ideas.
Now that we’ve discussed the basics of Java classes, we can move on to exploring the Java static keyword.
Java Static Methods
Static methods, also known as class methods, are methods that belong to a class and not its instances. Because static methods belong to a class, you can only invoke the method from the class itself, and not from an object of the class.
Here’s the syntax for invoking a static method:
Class.methodName();
Let’s look at an example to see how this works.
Suppose we are creating a program that manages the orders for a coffee store. We want to create a class called CoffeeOrder that stores all the functions for ordering a coffee.
We decide that we want to be able to print new order notifications to the barista’s console. We want this new order notification to be static because it is not dependent on any other code in the coffee order object. We can use the following code to accomplish this task:
class CoffeeOrder { int createOrder() { // Code here int orderNumber = 92; return orderNumber; } static void printOrder(int order) { System.out.println("Order #" + order + " order has been placed."); } } class Main { public static void main(String[] args) { CoffeeOrder order92 = new CoffeeOrder(); int number = order92.createOrder(); System.out.println("Order number: " + number); CoffeeOrder.printOrder(number); } }
Our code returns:
Order number: 92 Order #92 has been placed.
Let’s break down our code step by step. First we declare a class called CoffeeOrder. CoffeeOrder contains two methods: createOrder()
and printOrder()
.
createOrder()
is a regular method that creates an order and returns an order number. printOrder()
is a static method that prints a message to the console notifying the barista that a new order has been placed.
In our main program, we create an object of CoffeeOrder called order92. This object stores the data for order #92. Then we use the createOrder()
method to get an order number, and we print out that order number, preceded by Order number: , to the console.
When we invoke the createOrder()
method, we use order92.createOrder()
. This is because createOrder()
is not a static method, so we can access it when manipulating our object.
At the end of our main code, we use CoffeeOrder.printOrder()
to print a message stating Order #[order number] order has been placed. to the console. In this message, order number
is equal to the number associated with a specific order. Because printOrder()
is a static method, we have to use CoffeeOrder (our class) to access it.
If we tried to use order92.printOrder()
(which invokes an instance of our class), like we did when we used the createOrder()
method, our could would give us a warning.
Java Static Variables
The static keyword in Java can also be used to create static instance variables. Static variables belong to a class and are initialized only once, at the start of a program’s execution.
Static variables do not belong to an object. This means that in order to access them you need to reference the relevant class. Here’s the syntax to declare a static variable in Java:
static String COFFEE_NAME = "Espresso";
In this example, we declared a static variable called COFFEE_NAME, to which we assigned the string value Espresso.
Let’s walk through an example to showcase a Java static variable in use.
Suppose we are creating a program that processes orders at a coffee shop. This program should store the number of baristas on duty, and when an order is created, the program should associate that order with the name of the barista who will prepare that order.
We could use this code to create our coffee processing program:
class CoffeeOrder { int baristasOnDuty = 2; static String name = "Hannah Johnson"; public static void main(String[] args) { CoffeeOrder order111 = new CoffeeOrder(); System.out.println("Baristas on duty: " + baristasOnDuty); System.out.println("Assigned barista: " + order111.name); } }
Our code returns:
Baristas on duty: 2 Assigned barista: Hannah Johnson
In our code, we declare the following two variables:
- baristasOnDuty. This is a standard variable, which means that it can be accessed throughout our class.
- name. This is a static variable, which means we need to reference the object with which it is associated to retrieve its value.
In our main program, we create an instance of the CoffeeOrder class and call it order111. We then print out the message Baristas on duty: followed by the contents of the baristasOnDuty variable. Because this is a standard variable, we don’t need to reference its class name.
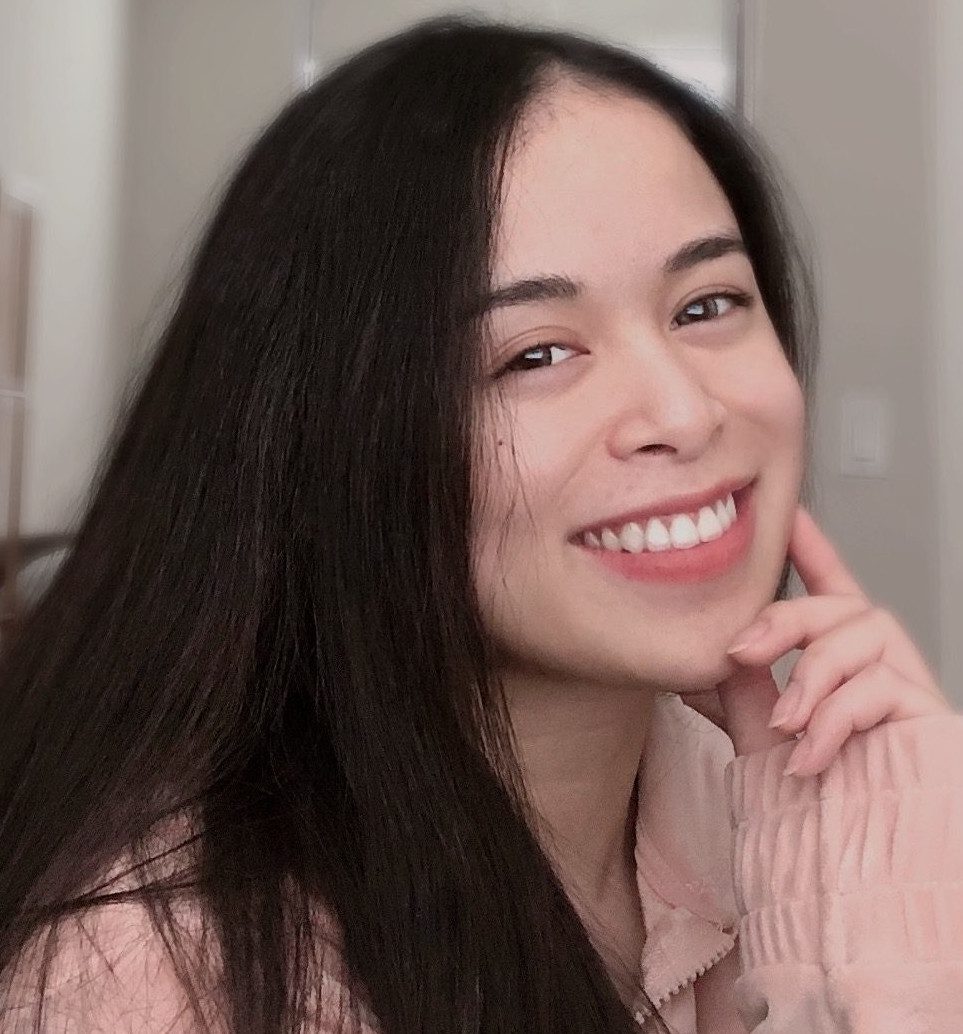
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
On the next line of our code, we print the message Assigned barista: to the console, followed by the name of the barista to whom the order is assigned. However, the barista’s name is stored as a static variable.
This means that, in order to retrieve the name of the barista, we need to reference the name of the object with which that variable is associated. In this case, we use order111.name to retrieve the barista’s name. This is because the variable name is static and therefore can only be accessed when referencing the order111 object.
Java Static Block
The Java static keyword can also be used to create a static block. If a class has different static variables that need to be initialized individually, then you should use a static block.
Static blocks are executed once—when the class is first loaded into the computer’s memory. Here’s the syntax for a static block of code in Java:
static { // Code here }
Let’s walk through an example to demonstrate how a static block works. Suppose we are asked to make the message A new order is incoming …
print to the console before the program assigns the order to a barista so the baristas know to prepare. We could do so by creating a static class. The static class will run the first time the program is executed.
Here’s an example of a static class that prints out the aforementioned message, then assigns an order to a barista:
class CoffeeOrder { int baristasOnDuty = 2; static String name = "Hannah Johnson"; static { System.out.println("A new order is incoming …"); } public static void main(String[] args) { CoffeeOrder order111 = new CoffeeOrder(); System.out.println("Baristas on duty: " + baristasOnDuty); System.out.println("Assigned barista: " + order111.name); } }
Our code returns:
A new order is incoming … Baristas on duty: 2 Assigned barista: Hannah Johnson
In our code, we first initialized baristasOnDuty and name variables. Then the program printed the message A new order is incoming … to the console. This message was stored within our static block of code. After the program executed the contents of the static block, it ran our main program, which returned the same output as the main program from our last example.
Conclusion
The Java static keyword has a number of uses. You can use the static keyword to create static methods, static variables, and static blocks of code.
This tutorial walked through, with examples, the basics of the static keyword and how you can use the static keyword with methods, variables, and code blocks. After reading this tutorial, you are ready to start using the Java static keyword like a pro.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.