The Collections.sort() method sorts a Java ArrayList in ascending order. Collections.reverse() reverses the order of a Java ArrayList. You need to import the Java Collections package to use these methods.
How to Sort an ArrayList in Java
You may encounter a situation where you want to sort a Java ArrayList in ascending or descending order. For instance, you may have a list of student names that you want to sort in alphabetical order.
That’s where the Collections.sort() and Collections.reverse() methods come in.
The Collections.sort() method is used to sort the elements in an ArrayList in Java. Collections.reverse() is used to sort the elements in an ArrayList, but in reverse order.
Using examples, this article will discuss sorting an ArrayList in Java. We’ll explore the basics of ArrayLists and the syntax for both the sort() and reverse() methods.
Java ArrayList
The Java ArrayList class allows you to create resizable arrays in Java. ArrayList is an implementation of the List interface, which is used to create lists in Java. This means that the ArrayList class can access a number of List methods used to manipulate and retrieve its data.
Java Sort ArrayList: sort() Method
The Java Collections package includes a number of methods for manipulating the contents of collections. In Java, an ArrayList is a class that is also a type of collection, so we can use the Collections package with an ArrayList.
The Collections package includes a method called sort(). This method is used to sort a collection in ascending order.
Before we can use the sort() method, though, we need to import the Collections package. We can do so using this code:
import java.util.Collections;
Now that we’ve imported the Collections package, we can use sort(). Here is the syntax for the sort() method:
Collections.sort(listName);
sort() accepts one parameter: the name of the list you want to sort. This method automatically sorts lists in ascending order.
Java ArrayList Sort: sort() Method Example
Suppose we have a list of student names from an elementary school class, and we want to sort those names in alphabetical order. Here’s a Java program that will sort our unsorted list:
import java.util.ArrayList; import java.util.Collections; class Main { public static void main(String[] args) { ArrayList<String> students = new ArrayList<>(); students.add("Lindsay"); students.add("Peter"); students.add("Lucas"); students.add("Athena"); System.out.println("Student list: " + students); Collections.sort(students); System.out.println("Sorted student list: " + students); } }
Our code returns:
Student list: [Peter, Lindsay, Lucas, Athena] Sorted student list: [Athena, Lindsay, Lucas, Peter]
Let’s break down our code. First, we import the ArrayList and Collections packages that we use throughout our code. We then declare a class, called Main, that stores the code for our main program.
In our main program, we execute the following steps:
- We declare a Java variable called students.
- Next, we add the student names to the ArrayList.
- We print the unordered list of student names, preceded by the message Student list:, to the console.
- Collections.sort() is performs a natural ordering operation that sorts the list.
- We print the message Sorted student list: to the console, followed by the newly-sorted list of student names.
Collections.sort() changes the order of an existing list. In the above example, when we executed the Collections.sort() method, the method changed the order of our existing list of student names. It did not create a new, sorted list.
Sort ArrayList Java: reverse() Method
The Collections.reverse() method sorts an ArrayList in reverse order. reverse() accepts one parameter: the list whose order you want to reverse.
Here is the syntax for the reverse method:
Collections.reverse(list)
“list” refers to the ArrayList whose order you want to reverse.
Because the reverse() method reverses the order of items in an ArrayList, we first need to sort the list in ascending order. Then, we can use reverse() to reverse the order of its items. Using the Collections.sort() and reverse() methods together allows you to sort a list in descending order.
Sort ArrayList Java: reverse() Method Example
Suppose we wanted our list of student names from earlier to be sorted in descending order. We could accomplish this task using the following code:
import java.util.ArrayList; import java.util.Collections; class Main { public static void main(String[] args) { ArrayList<String> students = new ArrayList<>(); students.add("Lindsay"); students.add("Peter"); students.add("Lucas"); students.add("Athena"); System.out.println("Student list: " + students); Collections.sort(students); Collections.reverse(students); System.out.println("Sorted student list: " + students); } }
Our code returns:
Student list: [Lindsay, Peter, Lucas, Athena] Sorted student list: [Peter, Lucas, Lindsay, Athena]
First we sort our list in alphabetical order. We reverse the order of the items in the list to see them in reverse alphabetical order. After we sort the students list using Collections.sort(), we reverse the order of the items in the list using Collections.reverse().
Conclusion
The Java Collections.sort() method sort an ArrayList in ascending order. Collections.reverse() method reverses the order of items in an ArrayList. When used together, sort() and reverse() can sort the items in an ArrayList in descending order.
There’s still much more to learn about using ArrayLists in Java. To learn more, you may want to view the following tutorials:
To learn more about coding in Java, read our How to Learn Java guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
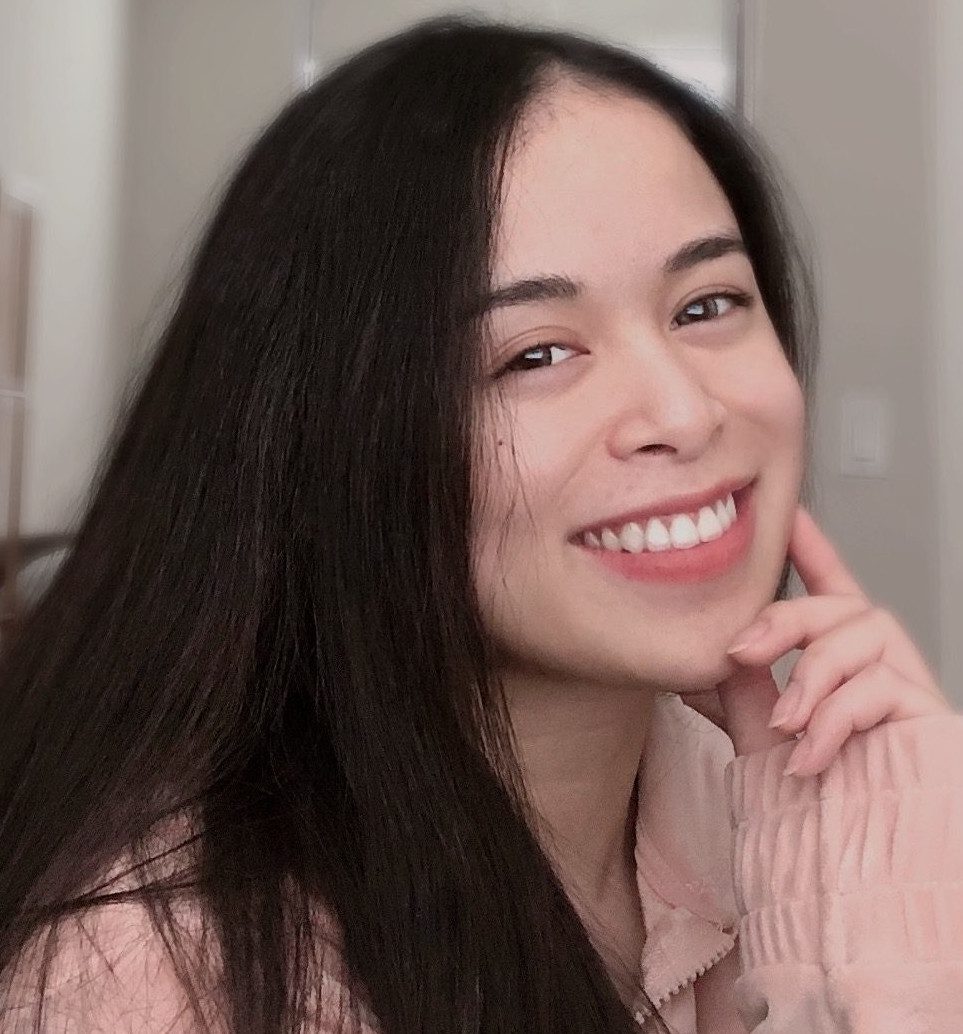
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot