How to Generate a Java Random Number
5. 7. 22. For us humans, it’s easy to generate a random number. All we have to do is ask ourselves for a number, and one pops into our mind.
If only it were so easy in programming, right? In Java, generating a random number is easy, if you know how.
In this guide, we’re going to discuss three approaches you can use to generate a random number. We’ll walk through examples of how each method works in the context of a number guessing game.
Generating a Random Number
We’ve all played one of those games that ask you to guess a number at some point. Some of them even award prizes if you can guess the right one. For this guide, we’re going to create a number guessing game in Java.
The first step is, of course, to generate a random number. Without a random number, our game would not be very fun. Let’s walk through each of the three methods we can use to do this.
Using the Random Class
Java has a handy class called “Random” which you can use to generate a random number. We’ll start our number guessing game by using the java.util.Random
class to generate a stream of random numbers:
import java.util.Random; class Main { public static void main(String args[]) { Random random_number_generator = new Random(); int random_number = random_number_generator.nextInt(25); System.out.println(random_number); } }
When we run this class three times, three random numbers are generated:
22 15 19
We’ve started by importing the class, java.util.Random
. Then, we have created an instance of this class called “random_number_generator”. Using this instance, we can generate random numbers.
The nextInt()
method allows us to generate a random number between the range of 0 and another specified number. Above, we specified the number 25. This means that all the numbers our generator will return will be between 0 and 25.
Using Math.random()
The Math.random() method takes a little bit more work to use, but it’s still a good way to generate a random number. Let’s create a random number generator sequence using Math.random()
:
class Main { public static void main(String args[]) { int small = 0; int large = 25; int random_number = (int)(Math.random() * (large - small + 1) + small); System.out.println(random_number); } }
Math.random()
comes built into Java. This means that we don’t have to import it in our code.
We’ve started by declaring two variables. “small” specifies the lower bound below which no number should be generated; “large” is the upper bound above which no number should be generated.
We’ve then used a formula to generate a random number. This is because Math.random()
in itself does not return a whole random number. The Math.random method returns a random number between 0.0 and 1.0. We’ve got to use the formula above to convert it to a whole number that’s within our range.
When we run our code three times, three random numbers are generated:
2 1 9
Using ThreadLocalRandom
ThreadLocalRandom is a class that you can use to generate random numbers.
This class is best to use when you want to generate multiple random numbers in parallel. This typically happens in multi-threading environments.
Let’s create a random number generator using this method:
import java.util.concurrent.ThreadLocalRandom; class Main { public static void main(String args[]) { int small = 0; int large = 25; ThreadLocalRandom random_number_generator = ThreadLocalRandom.current(); int random_number = random_number_generator.nextInt(small, large); System.out.println(random_number); } }
We’ve started by importing the ThreadLocalRandom library that contains the class we are using to generate a random number. Then we’ve specified two variables which set the lower and upper bounds for our random number generator.
We’ve declared a variable called random_number_generator which references the ThreadLocalRandom class. We’ve then used the nextInt()
method and specified our “small” and “large” variables as arguments in order to generate a random number.
Executing our program three times returns three random numbers:
4 23 15
Now we’re ready to start building the rest of our guessing game.
Creating the Guess Logic
We now know how to generate a random number. The next step in building our game is to create the logic that allows a user to guess a random number.
You can use any of the above examples with this code to make it work. The variable names in our code snippets have been set up so that they are compatible with the below example.
Let’s start by using the “Scanner” class to ask a user to guess a number:
import java.util.Scanner; class Main { public static void main(String[] args) { // Guessing code goes here Scanner guess = new Scanner(System.in); System.out.println("Guess a number between 1 and 25: "); int user_guess = guess.nextInt(); } }
This code asks our user to “Guess a number between 1 and 25: ”. The value that the user enters into the Java console will be stored as the variable “user_guess”.
We’re then going to write an if statement that checks if the number the user has guessed is equal to the one our program has generated:
... if (user_guess == random_number) { System.out.println("You have correctly guessed the number!"); } else { System.out.println("Your guess is incorrect!"); }
Let’s run our program and type in a number between 1 and 25:
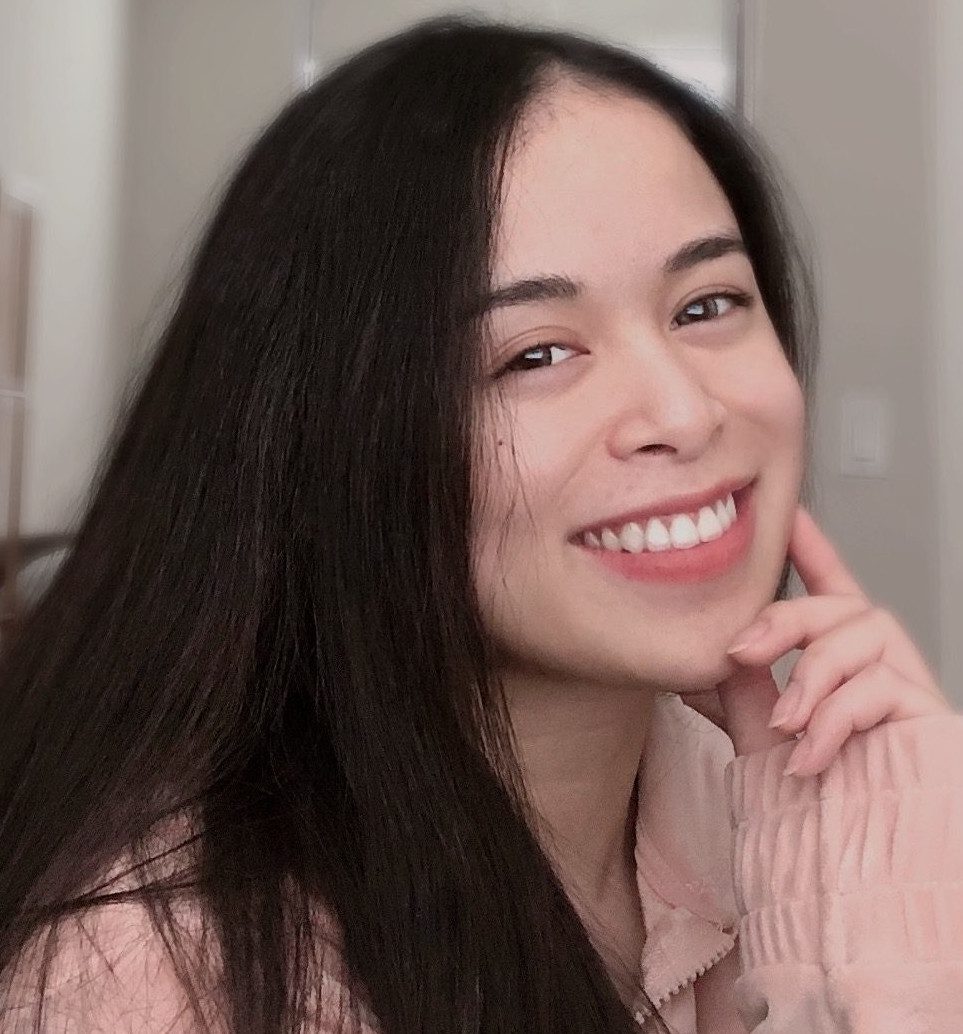
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Guess a number between 1 and 25: 7 Your guess is incorrect!
If we guess the number correctly, the following is returned by our program:
Guess a number between 1 and 25: 9 You have correctly guessed the number!
With only a few lines of code, we’ve been able to successfully create a game that generates a random number to guess.
Conclusion
Random numbers have a wide range of uses in programming. In this example, we’ve walked through how a random number could be used with a guessing game.
There are three methods you can use to generate random numbers:
- Using the Random class
- Using the built-in
Math.random()
method - Using the ThreadLocalRandom class
Are you looking for a challenge? Change our guessing game code so that you can make multiple guesses.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.