ArrayLists have been a trending topic in Java. They provide you with many more benefits than standard arrays. ArrayLists allow you to efficiently store objects in a row and clear them off when not needed. They also help you to perform standard operations on your lists like sorting and searching. If you were to use a standard array, you would have to write your own logic for these operations, which requires additional time and care.
Owing to the long list of benefits, ArrayLists have applications in a wide array of cases. However, a crucial operation that does not come in-built with ArrayLists is printing. ArrayLists can be composed of standard, primitive data types (like integers and strings), or of more advanced data types, like custom classes. In each of these cases, printing an ArrayList requires different steps.
In this article, we will take a look at the top three ways to print an ArrayList in Java, covering the two types of ArrayLists that we discussed above. Without further ado, let’s begin!
Print Java ArrayList: Three Ways
As mentioned above, there are two types of ArrayLists: one that contains objects having a primitive, simple data type (like integers and floats), and another that contains objects that are instantiated via custom classes. There are several ways to print these two types of ArrayLists.
These are the top three ways to print an ArrayList in Java:
- Using a for loop
- Using a println command
- Using the toString() implementation
Method 1: Using a for Loop
A for loop is one of the simplest ways of iterating across a list or array. This method works well with simple arrays and ArrayLists alike, as iterating through all items one by one allows you to handle each of them accordingly.
This method helps you define specific implementations for each element. Following is the syntax of a for loop:
for (int i=0; i<arr.size(); i++) { // Assuming arr is an ArrayList object int current = arr.get(i); // Do something with the current element here }
Now let’s take a look at some of the common ways in which a for loop can be used to traverse through an array of objects:
for Loop Examples
You can use the for loop to simply print all elements of ArrayList:
ArrayList<Integer> arr = new ArrayList<>(); arr.add(3); arr.add(4); arr.add(5); arr.add(6); for (int i=0; i<arr.size(); i++) { int curr = arr.get(i); System.out.println(curr); }
To take this up a level, you can define specific cases for each element based on any conditions:
ArrayList arr = new ArrayList<>(); arr.add(3); arr.add(5l); arr.add("Hello World!"); for (int i=0; i<arr.size(); i++) { if (arr.get(i) instanceof Integer) System.out.println(arr.get(i) + " is a number"); else if (arr.get(i) instanceof String) System.out.println(arr.get(i) + " is a string"); else if (arr.get(i) instanceof Long) System.out.println(arr.get(i) + " is a long"); }
When to Use This Method
The for loop is best suited for situations when you have a relatively shorter set of elements and their types do not vary much. If you have a list of 20 items that are either integers (int) or strings, for loop is the way to go. If you have a list of more than 100 items that can be string, int, boolean, or anything else, for loop will be too cumbersome to use.
Method 2: Using a println Command
The println
command can be used directly in the case of ArrayLists made using primitive data types. Primitive data types in Java include string, integer, float, long, double, and boolean. If a list is composed of data that does not match these types, it can not be printed using this method.
The syntax for this method is simple:
System.out.println(arr); // Assuming arr is an ArrayList object
The reason this method works is that all primitive data types can be printed directly to the standard output device. They do not have to be parsed into a printable format. Other data, such as that stored in an instance of a custom class, can not be parsed by the println command directly, so this method wouldn’t work in this case.
println Examples
If you are trying to print a simple integer ArrayList, here’s how you would do it:
ArrayList<Integer> arr = new ArrayList<>(); arr.add(5); arr.add(4); arr.add(6); System.out.println(arr); # => [5, 4, 6]
If you are trying to print a heterogeneous ArrayList, that would work too:
ArrayList<> arr = new ArrayList<>(); arr.add(7); arr.add("Foo"); arr.add(12l); System.out.println(arr); # => [7, Foo, 12]
When to Use This Method
This method is best suited for situations when you have a short, simple list of items and you are looking to print it out for debugging purposes. Note that this method is not as flexible as the previous one, and you can not format the content that gets printed to the output device.
In addition, if the list contains any non-primitive data, its value will not get printed to the device. Instead, a pointer to its location in the memory will be printed.
class Foo { int bar; public Foo(int bar){ this.bar = bar; }; } ArrayList<> arr = new ArrayList<>(); arr.add(4); arr.add(new Foo(40)); System.out.println(arr); # => [4, 34n2dsf@Foo];
Method 3: Implementing the toString() Method
This method doesn’t have the shortcomings of the previous method. The reason non-primitive objects can not be printed to the output directly is that the compiler does not know how to print them. The toString() method allows you to tell the compiler how to print the objects of a class to the command line.
Let’s take the class Foo from the last example:
class Foo { int bar; public Foo(int bar){ this.bar = bar; }; }
To make this print-friendly, you need to override and define the toString() method in the class definition:
class Foo { int bar; public Foo(int bar){ this.bar = bar; }; public String toString() { return "Bar: " + String.valueOf(bar); } }
Now if you try and create an instance of Foo:
Foo foo = new foo(20);
And print it:
System.out.println(foo);
You will get the output that you defined earlier:
// # => Bar: 20
This little fix can be coupled with the println method to print all kinds of ArrayLists easily and quickly.
toString() Examples
Let’s take the last example from method 2 and make it println-friendly:
class Foo { int bar; public Foo(int bar){ this.bar = bar; }; } ArrayList<> arr = new ArrayList<>(); arr.add(4); arr.add(new Foo(40)); System.out.println(arr); # => [4, Foo@2a139a55];
The only change that you need to do here is to add the toString() definition in the Foo class:
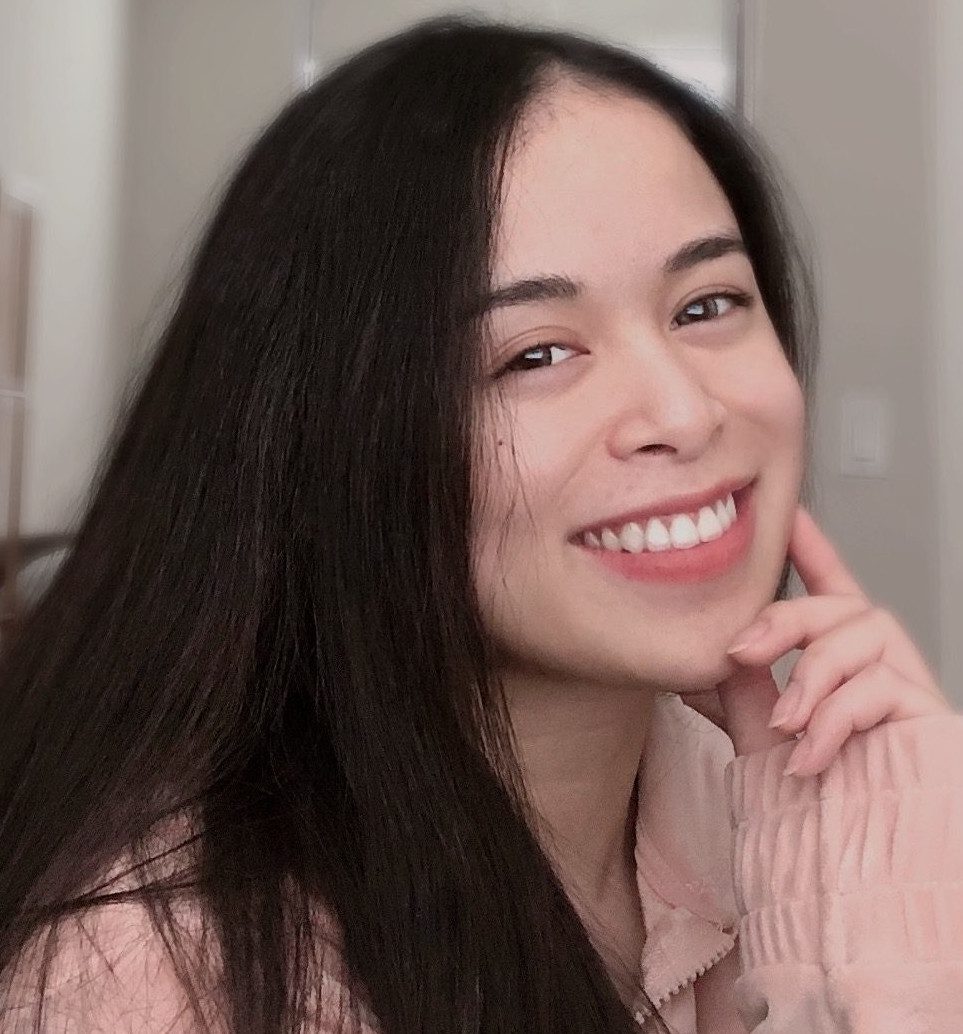
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
class Foo { int bar; public Foo(int bar){ this.bar = bar; }; public String toString(){ return "Value: " + String.valueOf(bar); } } Now, the println statement will give the desired output: // # => [4, Value: 40]
When to Use This Method
This method is appropriate for situations where you need to print heterogeneous ArrayLists, or a homogeneous ArrayList made up of a custom class. A single method definition in the class saves you the trouble you would encounter using a for loop.
However, this method can seem like overkill in simpler situations. If you have a small list of elements, you can consider going with the for loop method for ease and simplicity in logic.
Conclusion
Printing a Java ArrayList is not a straightforward task. The more dynamic an ArrayList’s contents are, the more difficult it is to print the list to the standard output. The println
command can cover most cases, but you might also need a toString()
override to get the job done.
In this article, we covered three ways of printing an ArrayList. We looked at their syntax, examples, and the situations that they are best suited for. For more advanced Java resources, explore our guide on how to learn Java.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
Hi
You missed printing out an element of an object in an ArrayList.
E.g. 10 var’s in each object, and 5 of those in the array for e/g.
So whats the best way to find variable y in each of those objects in that arrayList?