In object-oriented programming, methods are blocks of code that perform a specific task. For instance, a method could check whether a customer has enough money in their bank account to make a purchase or sort the contents of a list of student names in alphabetical order.
This tutorial will discuss, with reference to examples, how to use methods in Java. We’ll explore how to create a method, the Java method syntax, and how to call a method. By the end of reading this guide, you’ll be an expert at using Java methods.
Java Method Introduction
Java methods are used to define blocks of code that perform a specific task. Methods are also known as functions in object-oriented programming.
There are two main reasons why developers use methods in their code. First, methods allow developers to reuse code. Once you have declared a method, you can reuse it multiple times in your code. So, if you need to execute the same task multiple times, you can call a method instead of retyping the code over again.
Methods also make your code easier to read because your code will be stored in a specific block with its own name, instead of in the main program that you are writing.
There are two types of methods in Java: standard library methods and user-defined methods.
Standard library methods are the methods that are built-into the Java programming language. For instance, the println()
method is part of the java.io.PrintStream
library.
Here’s an example of a program that uses the built-in println()
method:
class Main { public static void main(String[] args) { System.out.println("This is a print statement."); } }
Our code returns:
This is a print statement.
User-defined methods, on the other hand, are methods that are defined inside a Java class.
Java User-Defined Methods
In Java, user-defined methods are created by the user and are defined inside a class based on your needs. A user-defined method contains a block of code you have written that will be contained in a specific function in your code.
Before you start using a method, you need to define (or declare) one. Here’s the syntax for declaring a method in Java:
modifier static returnType methodName (arguments) { // Code goes here }
Let’s break down this syntax:
- modifier is the access type the function will use (public, private, etc.).
- static is an optional keyword that allows your method to be accessed without creating an object of the class.
- returnType is the type of data returned by the method (int, float, String, double, etc.).
- methodName is the name of the method you are declaring.
- arguments are the values passed into a method. This parameter list can include zero, one, or multiple values.
Let’s walk through an example of a Java program that uses a method to demonstrate how this works.
Suppose we are building an app for a local coffee shop that processes their orders. We want to create a program that prints out the message “The order is ready” at the end of the program. We could accomplish this task using the following code:
class Main { public static void orderReady() { System.out.println("The order is ready."); } }
In our code, we have declared a method called orderReady()
. On the first line, we declare a class called Main in which our main program is written.
Next, we have created a method called orderReady()
. orderReady()
does not accept any arguments and does not return any values.
When the orderReady()
method is called, the code within the method body will be executed. So, the message The order is ready.
will be printed to the console.
Right now, though, our code does nothing. That’s because we haven’t called our method. In order to run the code in our method, we need to call it. Here’s how we can call our method:
import java.util.Scanner; class Main { public static void orderReady() { System.out.println("The order is ready."); } public static void main(String[] args) { orderReady(); } }
When we run our code, the following response is returned:
The order is ready.
In our code above, we defined the main function, which includes the code for our main program. Then we called the orderReady
function using orderReady()
.
Java Method with Arguments
In addition, Java methods can accept arguments passed through the method, which allows you to pass data through to a method. Suppose we wanted our message to print out Order #[order number] is ready.
, which would make it clearer to the barista what order was ready. We could do so using this code:
class Main { public static void orderReady(int orderNumber) { System.out.println("Order #" + orderNumber + " is ready."); } public static void main(String[] args) { orderReady(12); } }
When we run our code, the following response is returned:
Order #12 is ready.
In this example, our code accepts a parameter called orderNumber. We pass the value 12 as the orderNumber parameter above, which is then read by the orderReady()
method. The orderReady()
method returns, Order #12 is ready.
in the above example, where 12 is the value we passed to the orderNumber
parameter.
Java Method with Return Value
Java methods can also return values to the main program. Suppose we are creating an app that multiplies two numbers together. We want to multiply these numbers in a function, then return the result to the main program.
We could do so using the following code:
class MultiplyNumbers { public static int multiplyNumbers(int numberOne, int numberTwo) { return numberOne * numberTwo; } public static void main(String[] args) { int numberOne = 7; int numberTwo = 9; int multiplied = multiplyNumbers(numberOne, numberTwo); System.out.println(numberOne + " x " + numberTwo + " is: " + multiplied); } }
Our code returns:
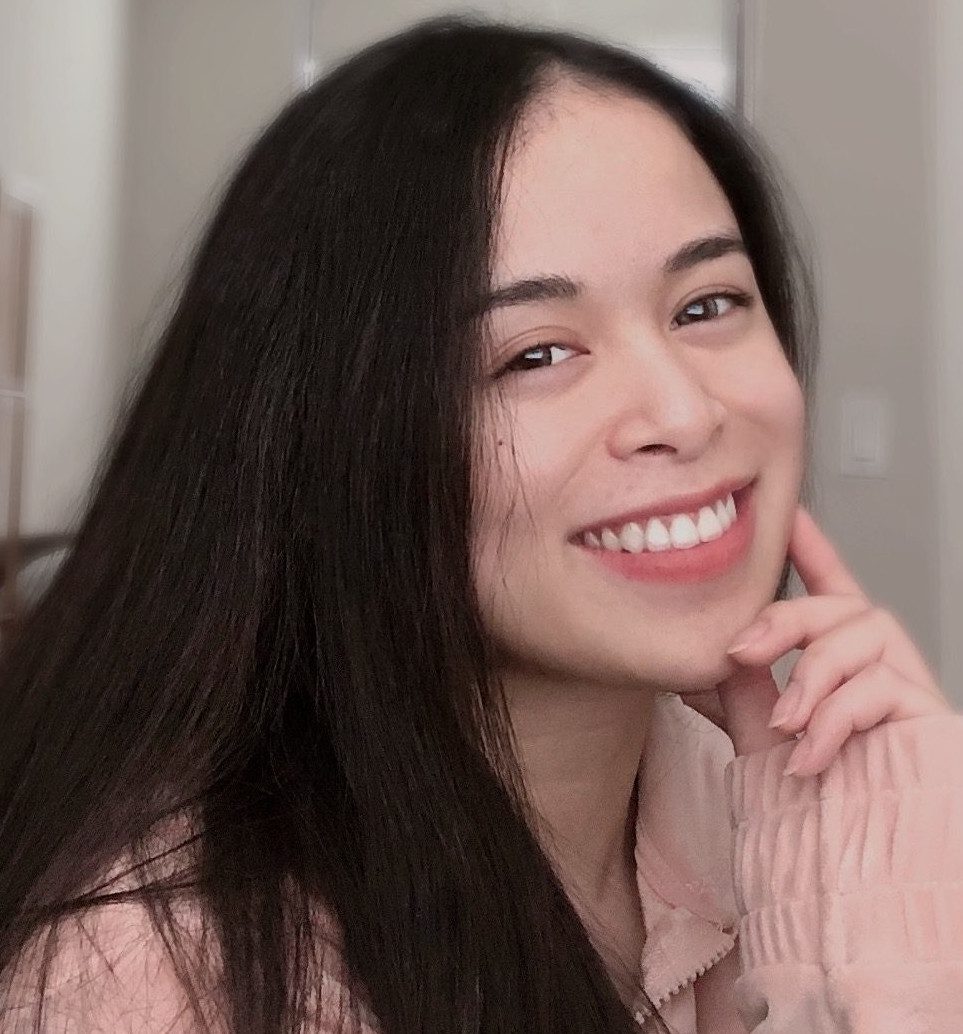
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
7 x 9 is: 63
In our code, the multiplyNumbers()
method is used to multiply two numbers together. When we call multiplyNumbers
, we need to specify two method parameters, which are the numbers we want to multiply together. In the above example, the numbers we multiply are 7 and 9.
Then, our multiplyNumbers()
method multiplies these two numbers together and returns the multiplied number. This number is then passed back to the main program.
Here’s what happens when we run our program, step-by-step:
- The variable
numberOne
is declared and assigned the value 7. - The variable
numberTwo
is declared and assigned the value 9. multiplyNumbers()
is called and the variablesnumberOne
andnumberTwo
are specified as parameters. The result of this method is assigned to the variable multiplied.- A message is printed to the console stating “[Number1] * [Number2] is: [Result]”, where “Number1” is the value of
numberOne
, “Number2” is the value ofnumberTwo
, and “Result” is the value ofmultiplied
.
Conclusion
Methods are a crucial part of object-oriented programming and allow you to define a block of code that performs a specific task and can be reused multiple times. Methods are used in Java to make code more readable and efficient.
This tutorial discussed how to use methods in Java, how to call methods in Java, and how to use parameters and return statements in Java methods. After reading this tutorial, you’ll be an expert at using methods in Java.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.