The Java Arrays.asList()
method and ArrayList
class are used to initialize arrays in Java. The normal List
interface cannot be used to create arrays, so the ArrayList
class is required to create an empty array. The Java Arrays.asList()
method allows us to easily initialize the resulting array.
Initializing an array list refers to the process of assigning a set of values to an array. In order to work with ArrayLists in Java, you need to know how to initialize an ArrayList.
That’s where Java’s Arrays.asList()
method comes in. The Arrays.asList()
method allows you to initialize an ArrayList in Java.
This tutorial will explore, with examples, how to initialize an ArrayList in Java using the asList()
method. After reading this tutorial, you’ll be an expert at initializing array lists in Java.
Java ArrayList
In Java, the List
interface provides a set of features that allows data to be stored sequentially. However, since List is a Java collections interface, we cannot directly create a list from the interface—Java interfaces cannot be used to create objects.
ArrayList is a Java class that implements the List interface and allows us to create resizable arrays.
In Java, arrays have fixed sizes. When declaring an array in Java, you have to specify the size of that array. It can be difficult to change the size of an array once you set it.
One way to get around the difficulty of resizing an array in Java is to use the ArrayList class. Arrays stored in the ArrayList class are easy to resize. Therefore, developers often use the ArrayList class when storing data in an array.
Before we can use ArrayLists in our code, we need to import the ArrayList class. Here’s the code we can use to import this class into a Java program:
import java.util.ArrayList;
Now that we’ve imported the ArrayList class, we can start creating ArrayLists in our code. Here’s the syntax for creating a Java ArrayList:
ArrayList<Type> arrayName = new ArrayList<>();
Here are the main components of our syntax:
- ArrayList tells our program to create an array list.
- Type is the type of data our array list will store.
- arrayName is the name of the array list we are creating.
- new ArrayList<>() tells our program to create an instance of ArrayList and assign it to the arrayName variable.
Once we’ve created an ArrayList, we can start to initialize it with values.
Initialize an ArrayList in Java
In Java, you can initialize arrays directly. This means that when you declare an array, you can assign it the values you want it to hold. However, this is not the case with ArrayLists.
When you’re working with ArrayLists, you’ll typically add values to them using: add()
. For instance, the following code shows a program that adds the value London
to an ArrayList of cities:
import java.util.ArrayList; class Main { public static void main(String[] args) { ArrayList<String> cities = new ArrayList<>(); cities.add("London"); System.out.println("Cities: " + cities); } }
Our code returns:
Cities: [London]
This method works fine if we want to add a few values to an ArrayList. But what if we wanted to add 20 values from an existing array to an ArrayList?
We can use the Arrays.asList()
method to work around the array initialization limitation in ArrayList and give our ArrayList a set of default values.
The syntax for the asList()
method is as follows:
Arrays.asList(item1, item2, item3);
The asList()
method accepts any number of parameters, depending on the number of values you want to add to the ArrayList with which you’re working.
Let’s walk through an example to demonstrate the asList()
method in action.
Suppose we are creating a program that stores a list of student names who won the most gold stars every week for the last eight weeks. Instead of using the add()
method to add eight students to our list, we can use asList()
to initialize a list with eight values.
Here’s the code we could use to initialize a list of students who won the most gold stars every week for the last eight weeks:
import java.util.ArrayList; import java.util.Arrays; class Main { public static void main(String[] args) { ArrayList<String> students = new ArrayList<>(Arrays.asList("Paul", "David", "Lisa", "Alyssa", "Alex", "Ronald", "Todd", "Hope")); System.out.println("Students: " + students); } }
Our code returns:
Students: [Paul, David, Lisa, Alyssa, Alex, Ronald, Todd, Hope]
Let’s break down our code. We first declare an ArrayList—called students—that can store string values. We use the following code to initialize this ArrayList with a set of default values:
new ArrayList<>(Arrays.asList("Paul", "David", "Lisa", "Alyssa", "Alex", "Ronald", "Todd", "Hope"));
In this code, we created an array of eight elements. Then, we used the asList()
method to convert that list of elements to an ArrayList.
This method of initializing an ArrayList is easier to read than using eight separate add()
statements to add values to our ArrayList.
Conclusion
Java developers use the Arrays.asList()
method to initialize an ArrayList. Using asList()
allows you to populate an array with a list of default values. This can be more efficient than using multiple add()
statements to add a set of default values to an ArrayList.
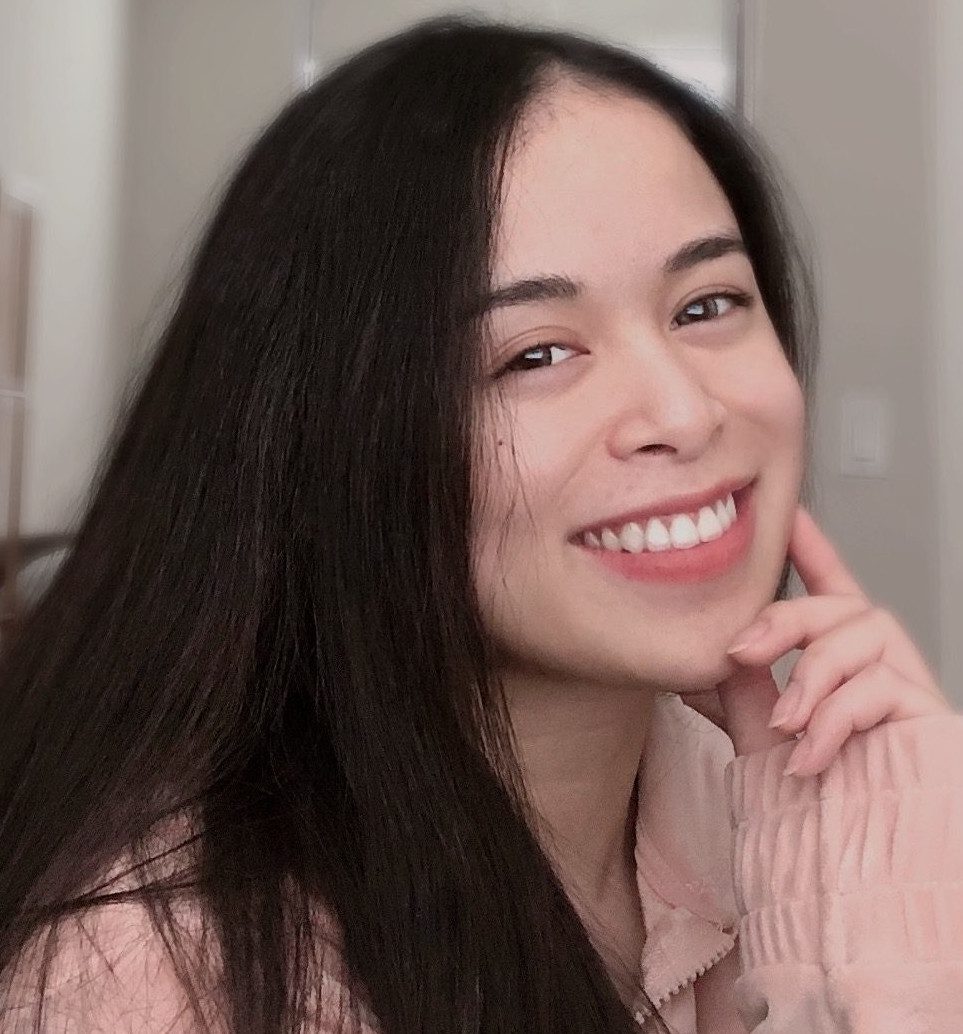
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
This tutorial discussed, using examples, how to initialize an ArrayList in Java. Now you’re equipped with the knowledge you need to initialize ArrayLists in Java like a professional!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.