Object-oriented programming includes a number of features that help prevent repetitive code. One of these features is inheritance.
This tutorial will discuss, using examples, the basics of inheritance in Java. We will explore how inheritance works, why it is important, method overriding, and how to use the super
keyword.
Java Inheritance
Inheritance is one of the most important features of object-oriented programming. Class inheritance refers to a process whereby you can define a new class based on an existing class.
Through inheritance, subclasses
can inherit values from parent classes
, similar to the way children inherit physical traits from parents. We define these two types of classes and their alternative (and interchangeable) names in the table below.
Class Type | Alternative Name(s) | Description [or Definition] |
parent class | base class, superclass | A parent class is a class from which child classes can be derived. |
subclass | child class | A subclass is a class which inherits its values from a parent class. |
Inheritance is important because it allows you to reuse code. If you write code in a parent class, you usually don’t need to write it again in associated child classes; doing so would be inefficient and would make a program more difficult to read.
For example, suppose you are creating a program that stores bank account information for a bank. You may want to have one class that stores regular bank accounts and another class that stores bank accounts for customers who are under 18 years of age (minors
).
The bank accounts for minors will have many similarities to regular bank accounts—both types of accounts will store names and balances, for example. Therefore, you may decide to make accounts for minors a subclass of a parent bank account class to prevent you from having to repeat code.
In Java, programmers use the extends
keyword to inherit characteristics from a class. Here’s an example of the extends keyword in action:
class BankAccount { // Code here } class ChildAccount extends BankAccount { // Code here }
In this example, BankAccount is the parent class, and ChildAccount is the subclass. Subclasses inherit all the methods and fields of parent classes.
Java Inheritance Example
You should use inheritance in Java if there is an is-a
relationship between two classes. Consider the following two examples:
Example 1. In the above section, we talked about bank accounts for minors and regular bank accounts (the ones owned by people aged 18 or over, without restrictions). A bank account for a minor is a type of bank account, and so it can inherit values from the bank account class.
Example 2. Another example would be an iPhone and a phone. The parent class would be called phone
, and the child class would be called iPhone
. An iPhone is a phone, and so it could inherit characteristics from the phone
parent class.
Let’s walk through an example to demonstrate how inheritance works in Java. We’ll return to the bank account example from earlier.
Suppose we want to create a program that stores banking information for bank customers. The bank for which we are creating this program allows customers to have one of two account types: a regular account (for someone aged 18 or over, which has no restrictions) or an account for a minor. Regular accounts are for customers over the age of 18. Accounts for minors are for customers under the age of 18. Accounts for minors have a few restrictions.
Because an account for a minor has many similarities to a bank account—an account for a minor is a bank account—we can use inheritance. Here’s an example of inheritance in use in the program we just described:
class BankAccount { public void checkBalance() { System.out.println("Your balance is: $X"); } public void viewDetails() { System.out.println("Name: Helen Clarkson"); System.out.println("Address: 123 Main Street"); System.out.println("Overdraft Limit: $0"); } } class ChildAccount extends BankAccount { public void setSavingsAmount() { System.out.println("Savings amount set to $Y."); } } class Main { public static void main(String[] args) { ChildAccount helen = new ChildAccount(); helen.checkBalance(); helen.viewDetails(); helen.setSavingsAmount(); } }
Our code returns:
Your balance is: $X Name: Helen Clarkson Address: 123 Main Street Overdraft Limit: $0 Savings amount set to $Y.
In this example, we defined three classes. They are:
- BankAccount. BankAccount is a parent class. This class has two methods:
checkBalance()
andviewDetails()
. - ChildAccount. ChildAccount is a class of BankAccount for minors and inherits the methods
checkBalance()
andviewDetails()
from the BankAccount class. In addition, ChildAccount has its own method:setSavingsAmount()
. - Main. We also declare a class called Main in which the code for our main program is stored.
In the main program, we create an instance of the ChildAccount class and call it helen. Helen is the name of our customer. Then, we invoke each method available to the child class.
As you can see in the code above, the helen variable can access both checkBalance()
and viewDetails()
, which are part of the BankAccount parent class, as well as the setSavingsAmount()
method, which is part of the ChildAccount child class.
Java Method Overriding
In the above example, we talked about how a subclass inherits the values of its parent class. But what happens if the same method is defined in both a subclass and its parent class?
In this case, the subclass will override the method in the superclass. For instance, suppose we wanted the viewDetails()
method to be different for a ChildAccount. Regular BankAccounts can access overdrafts, and perhaps we don’t want any ChildAccount to be able to see an overdraft limit since they are not eligible for this service.
We could use the following code to override the viewDetails()
method in the child class:
class BankAccount { public void checkBalance() { System.out.println("Your balance is: $X"); } public void viewDetails() { System.out.println("Name: Helen Clarkson"); System.out.println("Address: 123 Main Street"); System.out.println("Overdraft Limit: $0"); } } class ChildAccount extends BankAccount { public void setSavingsAmount() { System.out.println("Savings amount set to $Y."); } @Override public void viewDetails() { System.out.println("Name: Helen Clarkson"); System.out.println("Address: 123 Main Street"); } } class Main { public static void main(String[] args) { ChildAccount helen = new ChildAccount(); helen.checkBalance(); helen.viewDetails(); helen.setSavingsAmount(); } }
When we run our code, our program returns the following response:
Your balance is: $X Name: Helen Clarkson Address: 123 Main Street Savings amount set to $Y.
The response is the same as the one in our first example except for one difference: the console does not print the customer’s overdraft limit.
In this example, viewDetails()
is declared in both the subclass ChildAccount and the superclass BankAccount.
When we declared viewDetails()
in the ChildAccount, we used the @Override statement, which tells the program to override the superclass’ method. So, this means that when we reference the viewDetails()
method for Helen’s account, the program references the viewDetails()
method in the ChildAccount class instead of the viewDetails()
method in the BankAccount class.
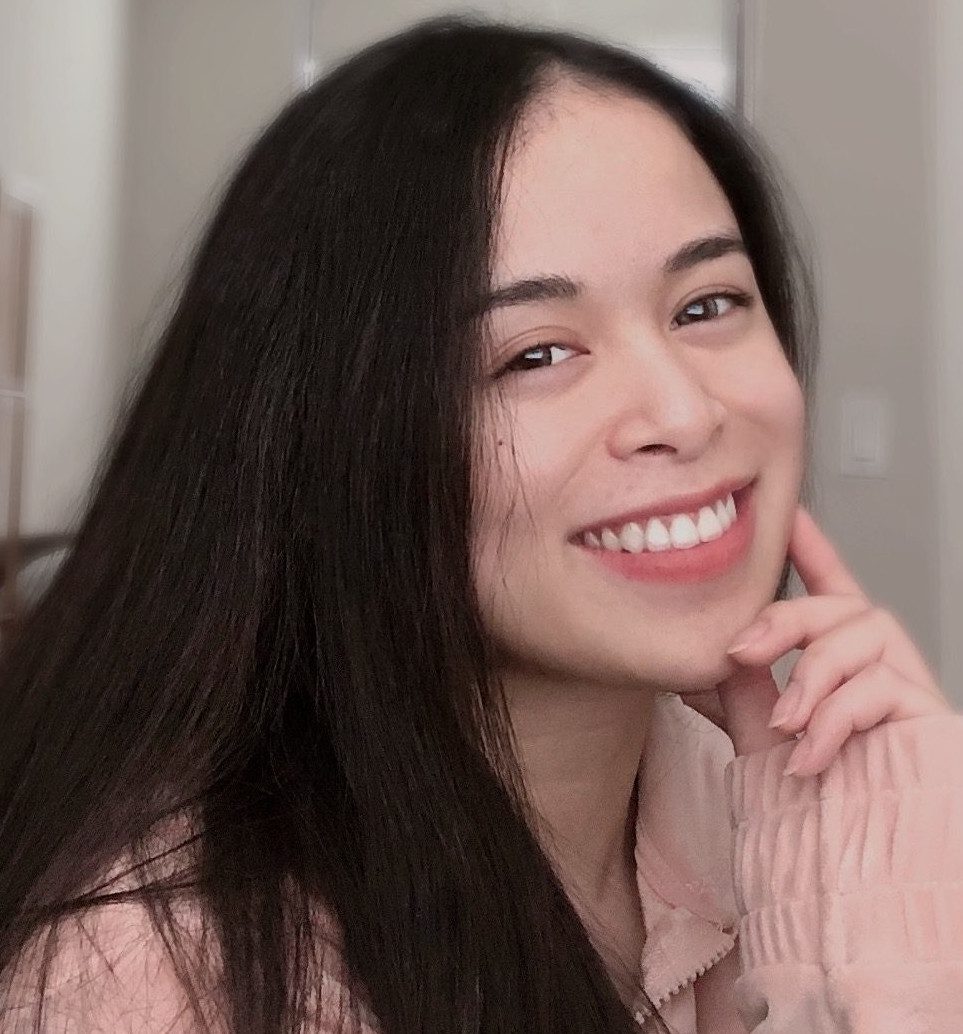
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Java Super Keyword
In Java, the super keyword is used to access an overwritten parent class method.
Let’s return to the bank account example. Suppose we decide that, instead of hiding the overdraft limit statement from customers who are minors, we want to show it and print a message to the user, telling them: You are not eligible for an overdraft until you reach the age of 18.
If this is the case, we will still want to access the code in the viewDetails()
method of the BankAccount class, but we want to add an extra message that only appears in instances of the ChildAccount class. We could do so using this code:
class BankAccount { // Code here } class ChildAccount extends BankAccount { // Code here @Override public void viewDetails() { super.viewDetails(); System.out.println("You are not eligible for an overdraft until you reach the age of 18."); } } class Main { public static void main(String[] args) { ChildAccount helen = new ChildAccount(); helen.checkBalance(); helen.viewDetails(); helen.setSavingsAmount(); } }
Our code returns:
Your balance is: $X Name: Helen Clarkson Address: 123 Main Street Overdraft Limit: $0 You are not eligible for an overdraft until you reach the age of 18. Savings amount set to $Y.
In this example, we used the super keyword to execute the viewDetails()
method from the BankAccount class, but from within the ChildAccount class.
So, when we execute helen.viewDetails()
to view Helen’s account details, the ChildAccount viewDetails()
method executes. In the ChildAccount viewDetails()
method, we use the super keyword, which causes the BankAccount viewDetails()
method to be run. This returns the following:
Name: Helen Clarkson Address: 123 Main Street Overdraft Limit: $0
Then, after the BankAccount viewDetails()
method is executed, the program continues to execute the ChildAccount viewDetails()
method. This results in the console printing the following message:
You are not eligible for an overdraft until you reach the age of 18.
After the viewDetails()
method in the ChildAccount class executes, our program continues to run.
Conclusion
Inheritance is used in object-oriented programming to define new classes from existing classes. This allows you to reuse code, thereby allowing you to write more efficient and readable code.
This tutorial discussed the basics of inheritance, how to use inheritance in Java, method overriding, and the super keyword. Now that you’ve read this tutorial, you are ready to start working with inheritance like a Java professional!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.