When you’re programming, it’s common to write code that should only be executed when a certain condition is met.
For instance, you may want an order to be placed on your e-commerce site only if a user has submitted their address. Or you may operate a coffee store and want to give a user a discount on their coffee if they have ordered more than five coffees in the last week.
In programming, we use conditional statements for this purpose. Conditional statements execute a specific block of code based on whether a condition evaluates to true or false. In Java, the if...else
statement is a control flow statement that allows you to execute a block of code only if a certain condition is met.
This tutorial will explore how to use the if...else
statement in Java, and explore a few examples of the if...else
statement being used in a Java program.
Java If Statement
The most basic conditional statement is the if
statement. if
statements evaluate whether a statement is equal to true or false, and will only execute if the statement is equal to true. If the statement evaluates to false, the program will skip over the if
statement and continue running the rest of the program.
In Java, if
statements are written like this:
if (condition_is_met) { // Execute code }
Let’s break it down. Our if
statement accepts a condition, which is the boolean expression that returns a true or false value. Then, the code that should be executed if the condition evaluates to true is enclosed within curly brackets ({}).
The code within an if
statement is indented. Additionally, the if
statement does not require a semicolon at the end, unlike other lines of code in Java.
Let’s walk through an example to illustrate how this works. Suppose we are running a coffee shop and we want to offer a 10% discount to any customer who has purchased more than five coffees in the last week. We could use the following code to check if a customer is eligible for the offer:
public class CheckDiscount { public static void main(String[] args) { int coffees_ordered_in_last_week = 4; int discount = 0; if (coffees_ordered_in_last_week > 5) { discount = 10; } System.out.println("This customer is eligible for a " + discount + "% discount."); } }
When we execute our code, the following response is returned:
This customer is eligible for a 0% discount.
Let’s break down our code. First, we declare a class called CheckDiscount in which our code for this program is enclosed.
Next, we declare a variable called coffees_ordered_in_last_week
that tracks how many coffees our customer has ordered in the last week. Then we initialize a variable called “discount” which keeps track of the discount for which our customer is eligible.
On the next line, we use an if
statement that checks whether coffees_ordered_in_last_week
is greater than 5. If it is, the customer will be eligible for a 10% discount; if it is not, the program skips over the code in theif
statement.
In this case, our customer has only ordered four coffees in the last week, and so our program does not run the discount = 10
line of code. At the end of our program, we print out a message to the console stating, This customer is eligible for a [X]% discount.
, where X is equal to the discount determined by the program.
If Else Java
When we use an if
statement, we only execute code when a condition is true. Often, however, we will want another block of code to run if the condition is false.
The else
statement is written after an if
statement and has no condition. The else
statement is optional and will execute only if the condition in the if
statement evaluates to false.
Here’s the syntax for a Java if...else
statement:
if (condition_is_met) { // Execute code } else { // Execute other code }
Let’s use our example from above to illustrate how this works. Suppose we are running a promotion where every customer is eligible for a 5% discount on Saturday. Today is Saturday, and so we want to give each customer a 5% discount. This discount is only available to people who have not ordered more than five coffees in the last week, as people who have ordered more than five coffees are eligible for a 10% discount.
We could use the following program to give each customer who has ordered more than five coffees in the last week a 10% discount, and give every other customer a 5% discount:
class CheckDiscount { public static void main(String[] args) { int coffees_ordered_in_last_week = 4; int discount = 0; if (coffees_ordered_in_last_week > 5) { discount = 10; } else { discount = 5; } System.out.println("This customer is eligible for a " + discount + "% discount."); } }
Our code returns:
This customer is eligible for a 5% discount.
Our code works in the same way as our first example. However, this time around, we have specified an else
statement in our code, which will run if the statement coffees_ordered_in_last_week > 5
evaluates to false. In this case, our customer has not ordered five coffees, so that statement evaluates to false.
As a result, our program executes the contents of the else
block, which sets the value of the discount
variable to five. So, when our program tells us the discount for which the customer is eligible, 5% is stated as the discount.
If Else If Java
You may be writing a program where you want to evaluate multiple statements and execute code depending on which statement (if any) evaluates to true. That’s where the if...else...if
statement comes in. The if...else...if
statement checks for one statement, then evaluates it for subsequent statements.
Here’s the syntax for a Java if...else...if
statement:
if (condition1_is_met) { // Run condition 1 code } else if (condition2_is_met) { // Run condition 2 code } else { // Run other code }
The if
statements are executed from the top downward. When an expression evaluates to true, the code within the corresponding block will execute. If no expression evaluates to true, the code within the else
statement will run.
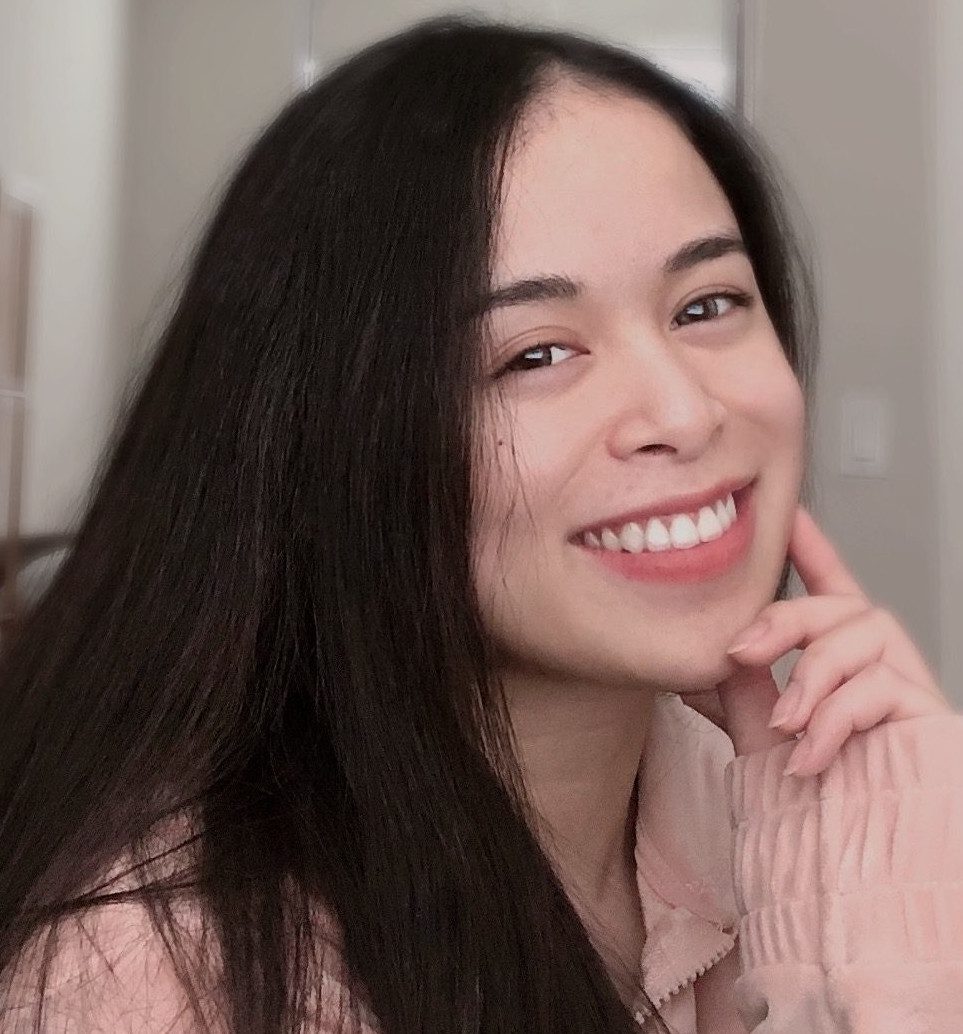
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Suppose we want to offer a 15% discount to everyone who has ordered more than ten coffees in the last week. These customers are often people who order for their friends or their workplaces, and so we want to do as much as possible to retain them.
We could use the following code to offer this discount, in addition to our 5% discount on Saturdays and our 10% discount to people who have ordered more than five coffees in the last week:
class CheckDiscount { public static void main(String[] args) { int coffees_ordered_in_last_week = 4; int discount = 0; if (coffees_ordered_in_last_week > 5) { discount = 10; } else if (coffees_ordered_in_last_weel > 10) { discount = 15; } else { discount = 5; } System.out.println("This customer is eligible for a " + discount + "% discount."); } }
When we run our code, the following is returned:
This customer is eligible for a 5% discount.
Our customer has only ordered four coffees in the last week, and so the contents of our else
statement are executed. But if our customer had ordered more than five coffees, the value of the discount
variable would be set to 10; if our customer had ordered more than ten coffees, the value of the discount
variable would be set to fifteen.
Conclusion
The if
statement is used in Java to run a block of code if a certain condition evaluates to true. The if...else
statement is used with an if
statement to run code if a condition evaluates to false. In addition, the if...else...if
statement is used to evaluate multiple conditions.
This tutorial discussed, with reference to examples, how to control the flow of your Java programs using if
, if...else
, and if...else...if
statements. You’re now ready to start using these Java conditional statements like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.