Welcome to the Java programming language. The first task any beginner should take on is the “Hello, World!” script. In this task, you have to print a message to the console. This is another way of saying make a line of text appear on the screen.
This guide walks you through an example “Hello, World!” program and explain how it works. Along the way, you’ll learn the building blocks that make up a Java program.
Prerequisites
Before we begin, make sure you have installed Java on your computer. You should have a text editor, like Atom or Sublime Text, as well. Text editing tools are used to view and edit programs in a Java project.
Java Hello World
Let’s introduce your program to the world by writing a program that shows “Hello, World!” on the console. This is what the typical HelloWorld program looks like:
class HelloWorld { // Show a message to the screen public static void main(String[] args) { System.out.println("Hello, World!"); } }
Create a file called HelloWorld.java
on your computer. Then, copy the text that you see above in that file. In Java, the names of a class must match up to the name of a file. Your file must be called HelloWorld because our class is called HelloWorld.
This is a feature of Java that helps keep programs consistent. As you write more advanced code, having classes in files with the same name makes it easier to navigate through those classes and find the code you are looking for.
Our code prints “Hello, World!” to the command line console:
Hello, World!
Java Hello World: A Deep Dive
That’s all you need to show a message to the console. In total, our program is six lines of code. As a beginner, you’re probably wondering what they mean.
We’ll start with the first line. On the first line, we define a class:
class HelloWorld { }
For a program to work in Java, it must be enclosed within a class. We have called our class HelloWorld. All the code within the curly braces is part of the class. Whereas languages like Python use spaces to indicate what code is part of a class, Java uses curly braces.
On the next line, we write a comment:
// Show a message to the screen
Comments are text that are readable by a programmer who is viewing or editing a file. The Java compiler, which runs your code, will not execute any comments. The compiler knows that comments are for human use, and not instructions for a machine.
Next, we define a main method:
public static void main(String[] args) { }
This line of code is a bit more complicated than the others, but it’s actually quite simple.
Every class must have a main method. This is where Java starts running a program. If your program did not have a main method, Java would not know where to begin executing your code.
We’re going to skip over the “public static void” part for now. Those are more advanced topics you’ll learn later. For now, you should know that most main methods in beginner programs use these keywords.
The String[]
args method lets you pass arguments into a method. We’re going to skip over this concept because you do not need to know how it works when you first start.
Like our class, our main()
method ends in a set of curly braces. In those curly braces, we have a statement that prints a message to the console:
System.out.println(“Hello, World!”);
The System.out.println()
method displays a message to the console. We don’t have to do any work other than tell this method what should be displayed.
In our program, we ask the method to print the statement “Hello, World!” to the console. This statement appears in curly brackets so our program knows our message is part of the method.
Moving On From “Hello, World!”
You have successfully written your first program in Java. You should give yourself a pat on the back. It is a big deal that you have written anything in code.
The “Hello, World!” program may be simple but that’s not a problem. You do not need to expect too much from yourself when you first begin. There will always be opportunities to master hard topics further down the line. The basics come first.
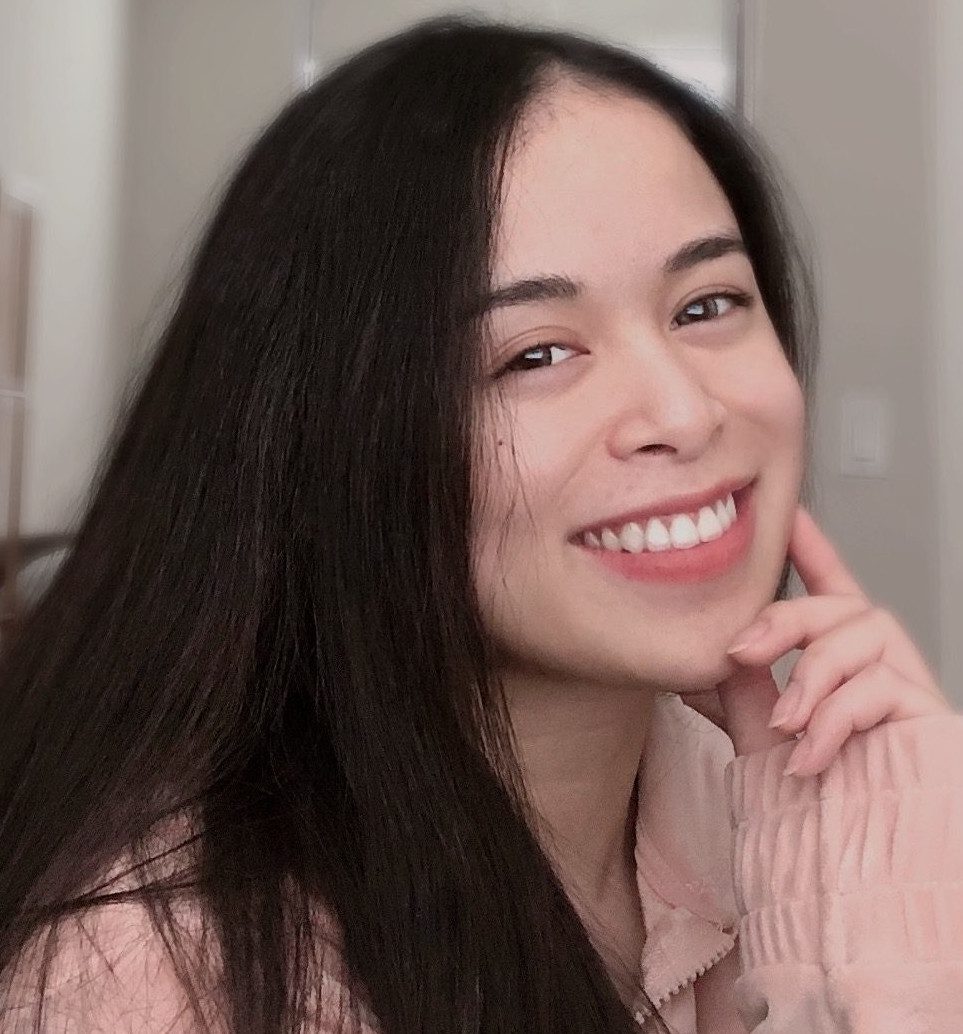
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
What we’ve learned is that every program must contain a class. This class should share the same name as the file in which it appears. Every program must contain a method called main(). This tells Java what code should run.
Files can contain comments. Comments are pieces of text that are readable by humans but are not executed by a computer.
Now you’re ready to move on to the next step of learning the Java language!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.