In Java, there are a number of data types that are used to store values. Each data type stores values in a different way, and offers a range of methods which can be used to manipulate the stored values. For instance, numbers can be manipulated using mathematical functions in Java.
HashSet is a data type in Java that is used to create a mathematical set. HashSet is part of the Java Collections framework and allows you to store data using the hash table data type.
This tutorial will discuss the basics of the Java HashSet class and how it can be used. We will also walk through the main methods offered by HashSet to retrieve and manipulate the data stored within a set.
Java Sets and HashSet
Sets are Java Collections that cannot contain duplicate elements. Whereas a list can contain the same value multiple times, sets can only contain a specific value once.
Sets can be useful in a wide range of situations. For instance, if you are creating a program for a local coffee shop that stores the phone numbers of their loyalty customers, you would only want the same phone number to appear once on the list.
In Java, the Set type is an interface, and so to utilize it, we have to use one of the classes associated with the data type. The HashSet class implements the Set data type and is used to create a set that uses the hash table data structure.
Before we can start working with HashSets, we have to import the HashSet package into our code. Here’s the code we can use to import HashSet into our code:
import java.util.HashSet;
Now we have imported HashSet, we can start working with the data type.
Java Create a HashSet
Here is the syntax you can use to create a Java HashSet:
HashSet<DataType> variable_name = new HashSet<>(capacity, loadFactor);
The main components of a HashSet are as follows:
- HashSet tells our program we want to declare a HashSet.
- DataType is the type of data that is held by the values stored in the hash set.
- variable_name is the name of our hash set.
- new HashSet<> initializes a HashSet and assigns it to
variable_name
. - capacity states how many values the hash set can store. By default, this is set to 8. (optional)
- loadFactor specifies that when a hash set is filled by a certain amount, the elements within the table are moved to a new table double the size of the original table. By default, this is set to 0.75 (or 75% capacity). (optional)
Suppose we want to create a HashSet that stores the list of fruits sold at our fruit stand. We could do so using the following code:
import java.util.HashSet;
HashSet<String> fruits = new HashSet<>();
In our code, we have created a HashSet called fruits
which will store string values. Now we have a HashSet ready, we can start working with it using the HashSet methods.
Add an Item
The add() method adds a specified element to a HashSet in Java. Suppose we wanted to add the values Pear
, Grapefruit
and Mango
to our list of fruits. We could do so using this code:
import java.util.HashSet;
class AddFruits {
public static void main(String[] args) {
HashSet<String> fruits = new HashSet<>();
fruits.add("Pear");
fruits.add("Grapefruit");
fruits.add("Mango");
System.out.println(fruits);
}
}
When we run our code, the following response is returned:
[“Pear”, “Grapefruit”, “Mango”]
Let’s break down our code. First, we import the HashSet class from java.util
, then we initialize a class called AddFruits, which stores the code for our program. On the next line, we declare a HashSet called fruits
which will store string values.
Then we use the add() method to add three values to our fruits
hash set: Pear, Grapefruit, and Mango. Finally, we print out the contents of the fruits
hash set to the console.
Remove an Item
The remove() method can be used to remove an item from a HashSet.
Suppose that our fruit stand has decided to stop selling mango due to a lack of demand. As a result, we want to remove the Mango
entry from the HashSet we created earlier. We could do so using this code:
…
fruits.remove("Mango");
System.out.println(fruits);
…
Our code returns:
[“Pear”, “Grapefruit”]
Our code has removed Mango
from our original HashSet and returns the revised HashSet with the two remaining values.
Additionally, the removeAll() method is used to remove all the elements from a set. So, if we decided that we wanted to start from scratch and build a new list of fruits, we could use removeAll() to delete all the items in our set. Here’s the code we could use to remove all the items in our “fruits” HashSet:
…
fruits.removeAll();
System.out.println(fruits);
…
Our code returns an empty hash set: []
.
Access Elements
Unlike Java arrays, Sets are not indexed. So, if we want to access the values in our set, we need to use the iterator() method and iterate through each value. iterator() is part of the “java.util.Iterator” package, so we’ll have to import the Iterator package before we can use the iterator() method.
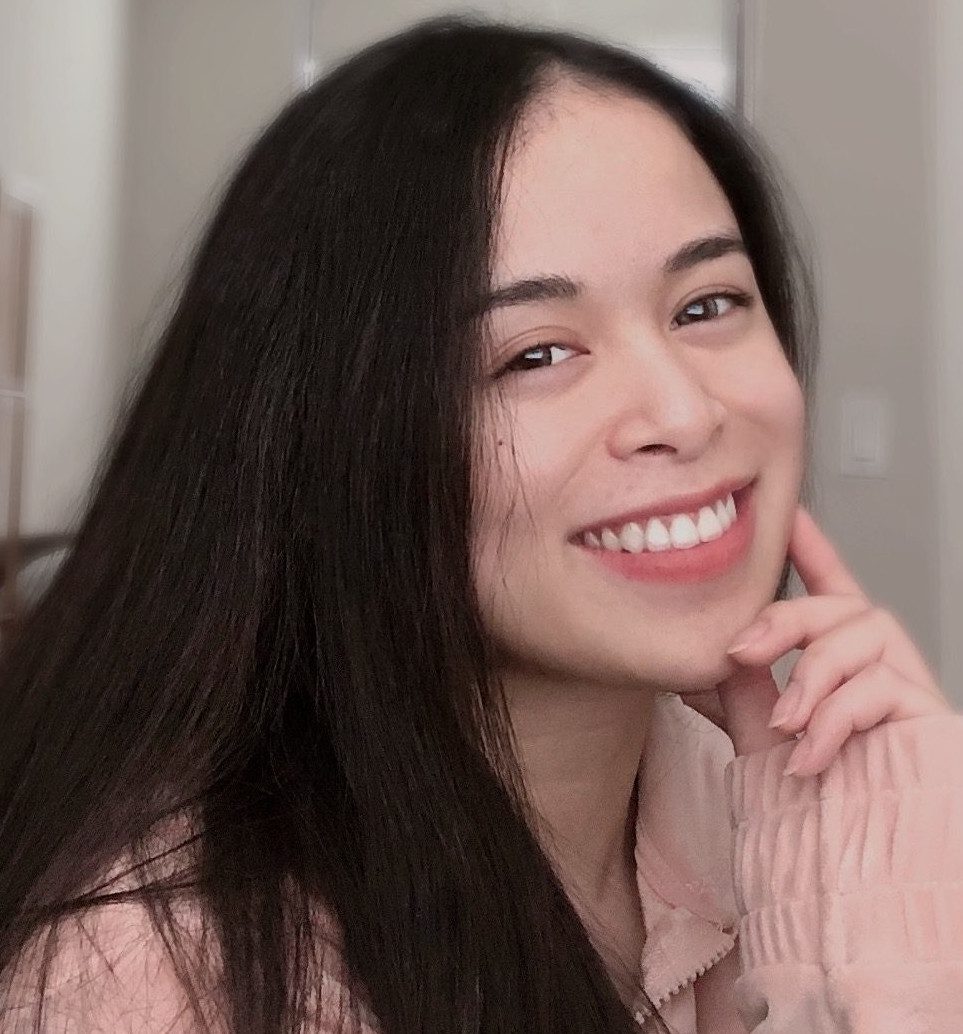
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Here’s a program we could use to iterate through every fruit in our fruits
array from earlier and print it out to the console:
import java.util.HashSet;
import java.util.Iterator;
class RetrieveFruits {
public static void main(String[] args) {
HashSet<String> fruits = new HashSet<>();
fruits.add("Pear");
fruits.add("Grapefruit");
fruits.add("Mango");
Iterator<String> iterate = fruits.iterator();
while(iterate.hasNext()) {
System.out.println(iterate.next());
}
}
}
Our code returns:
Pear
Grapefruit
Mango
In our code, we first import the HashSet and Iterator libraries from java.util
. Then we declare a class called RetrieveFruits in which the code for our program is located. Next, we initialize a HashSet called fruits
and assign it three values: Pear, Grapefruit, and Mango.
On the next line, we initialize an iterator, which allows us to iterate through every element in our HashSet. Then we create a while loop that goes through every item in the fruits
hashset and prints it out to the console.
Set Operations
The HashSet class is a Set, and so the class can access the various set operations offered by the Set data type. There are four main set operations that can be used with the HashSet class: union, intersection, subset, and difference. Let’s walk through how each of these methods works individually.
HashSet Union
The addAll() method can be used to perform a union between two sets. In other words, addAll() allows you to merge the contents of two sets together.
Suppose we have been storing our fruits in two hash sets. The first hash set, fruits
, stores a list of general fruits that we sell at our stand. The second hash set, berries
, stores the berries that we sell. We have decided that we want to merge these two sets together.
We can merge our two sets by using the following code:
import java.util.HashSet;
class UnionFruits {
public static void main(String[] args) {
HashSet<String> fruits = new HashSet<>();
fruits.add("Pear");
fruits.add("Grapefruit");
fruits.add("Mango");
HashSet<String> berries = new HashSet<>();
berries.add("Strawberry");
berries.add("Raspberry");
berries.add("Blueberry");
fruits.addAll(berries);
System.out.println(fruits);
}
}
The addAll() method returns:
[Pear, Grapefruit, Mango, Strawberry, Raspberry, Blueberry]
As you can see, our code has merged our fruits
and berries
hash sets into the fruits
hash set. Then, our code prints out the revised hash set to the console.
HashSet Intersection
The intersection is used to find common values in two data sets. We can use retainAll() to perform an intersection on two data sets.
Suppose we have our lists of berries
and fruits
and we want to make sure there are no common values. We could use the code from our Union
example with one change to accomplish this task:
…
fruits.retainAll(berries);
System.out.println(fruits);
…
Our code returns: [].
Instead of using addAll(), we use retainAll() to perform an intersection. As you can see, because there are no common values between our fruits
and berries
lists, an empty hash set is returned. This tells us that there are no duplicate values in our lists, which is exactly what we wanted.
HashSet Subset
The containsAll() method is used to check whether a set is a subset of another set. In other words, containsAll() checks whether a set contains only values from another set.
Suppose we wanted to check whether our berries
list was a subset of our fruits
list. We could do so using this code:
…
fruits.containsAll(berries);
System.out.println(fruits);
…
Our code returns: false. The values in berries
that we defined in our Union
example are not the same as those in fruits
. So, our code returns false.
HashSet Difference
The removeAll() method is used to calculate the difference between two sets. Suppose we have a list of fruits and a list of summer fruits and winter berries, and we want to know the difference between the two. We could use this code to calculate the difference:
class DifferenceFruits {
public static void main(String[] args) {
HashSet<String> summer_fruits = new HashSet<>();
summer_fruits.add("Pear");
summer_fruits.add("Grapefruit");
summer_fruits.add("Grape");
HashSet<String> winter_fruits = new HashSet<>();
winter_fruits.add("Grapefruit");
winter_fruits.add("Plum");
winter_fruits.add("Grape");
summer_fruits.removeAll(winter_fruits);
System.out.println(summer_fruits);
}
}
Our code returns:
[Pear]
We performed a difference search on the summer_fruits
list and checked which values existed in only the summer_fruits
list. In this case, Pear
is the only fruit that exists only in the summer_fruits
list, and so our list returned one value: Pear.
Conclusion
The HashSet class implements the Set interface with hash tables in Java. HashSet is commonly used if you want to access elements randomly or store a list of items which cannot contain duplicate values.
This tutorial discussed the basics of Java HashSets and, with reference to examples, explored how to declare and manipulate a Java HashSet. Now you’re ready to start using the Java HashSet class like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.