In programming, data types are used to classify particular types of data. Each data type is stored in a different way, and the data type in which a value is stored will determine the operations which can be performed on the value.
When you’re working in Java, one class you may encounter is the Java HashMap class. This class is part of the collections framework and allows developers to store data using the Map data type.
This tutorial will discuss the basics of Java HashMaps, how to create a HashMap, and explore the main methods which can be used when working with the HashMap class. This article will refer to examples throughout so that we can explain the HashMap class in more depth.
Java Maps and HashMap
The Java Map interface is used to store Map values in a key/value pair. Keys are unique values that are associated with a specific value. In Java, a map cannot contain duplicate keys, and each key must be associated with a particular value.
The key/value structure offered by Map allows you to access values based on their keys. So, if you had a map with the key gbp
and the value United Kingdom
, when you reference the key gbp
the value “United Kingdom” will be returned.
The HashMap class is part of the collections framework and allows you to store data using the Map interface and hash tables. Hash tables are special collections used to store key/value items.
Before we can create a HashMap, we must first import the HashMap package. Here’s how we can do that in a Java program:
import java.util.hashmap;
Now that we have imported the HashMap package, we can start creating HashMaps in Java.
Create a HashMap
To create a HashMap in Java, we can use the following syntax:
HashMap<KeyType, ValueType> map_name = new HashMap<KeyType, ValueType>(capacity, loadFactor);
Let’s break this down into its basic components:
- HashMap is used to tell our code that we are declaring a hashmap.
- <KeyType, ValueType> stores the data types for the keys and values, respectively.
- map_name is the name of the hashmap we have declared.
- new HashMap<KeyType, ValueType> tells our code to initialize a HashMap with the data types we have specified.
- capacity tells our code how many entries it can store. By default, this is set to 16. (optional)
- loadFactor tells our code that when our hash table reaches a certain capacity, a new hash table of double the size of the original hash table should be created. By default, this is set to 0.75 (or 75% capacity). (optional)
Suppose we are creating a program for a local currency exchange business. They want to create a program that stores the names of the countries and the currency codes in which they offer exchange services. Using a HashMap is a good idea to store this data because we have two items that we want to store together: country name and currency code.
Here’s the code we would use to create a HashMap for this purpose:
import java.util.HashMap; HashMap<String, String> currencyCodes = new HashMap<String, String>();
In this example, we have declared a HashMap called currencyCodes
which stores two String values. Now that we have our HashMap, we can start adding items and manipulating its contents.
Add Items
The HashMap class offers a wide range of methods that can be used to store and manipulate data. The put() method is used to add values to a HashMap using the key/value structure.
Let’s go back to the currency exchange. Suppose we want to add the entry GBP
/United Kingdom
into our program, which will store the currency value for the UK. The GBP
key is mapped to the United Kingdom
value in this example. We could do so using this code:
import java.util.HashMap; class CurrencyExchange { public static void main(String[] args) { HashMap<String, String> currencyCodes = new HashMap<String, String>(); currencyCodes.put("GBP", "United Kingdom"); currencyCodes.put("USD", "United States"); System.out.println(currencyCodes); } }
In our code, we initialize a hash map called currencyCodes
, then we use the put() method to add an entry into the hash map. This entry has the key GBP
and the value United Kingdom
. Then we print out the value of the HashMap, which returns the following:
{GBP=United Kingdom,USD=United States}
As you can see, our HashMap now contains two values: GBP=United Kingdom and USD=United States.
Access an Item
To access an item in a HashMap, you can use the get() method. The get
method accepts one parameter: the key name for the value you want to retrieve.
Suppose we want to retrieve the country name associated with GBP. We could do so using this code:
… String gbp = currencyCodes.get("GBP"); System.out.println(currencyCodes); …
Our code returns: United Kingdom.
Remove an Item
The remove() method is used to remove an item from a HashMap. remove() accepts one parameter: the name of the key whose entry you want to remove.
Suppose we want to remove GBP
from our HashMap. We could do so using this code:
… currencyCodes.remove("GBP"); System.out.println(currencyCodes); …
When we run our code, GBP
is removed from our HashMap and the following response is returned: {USD=United States}
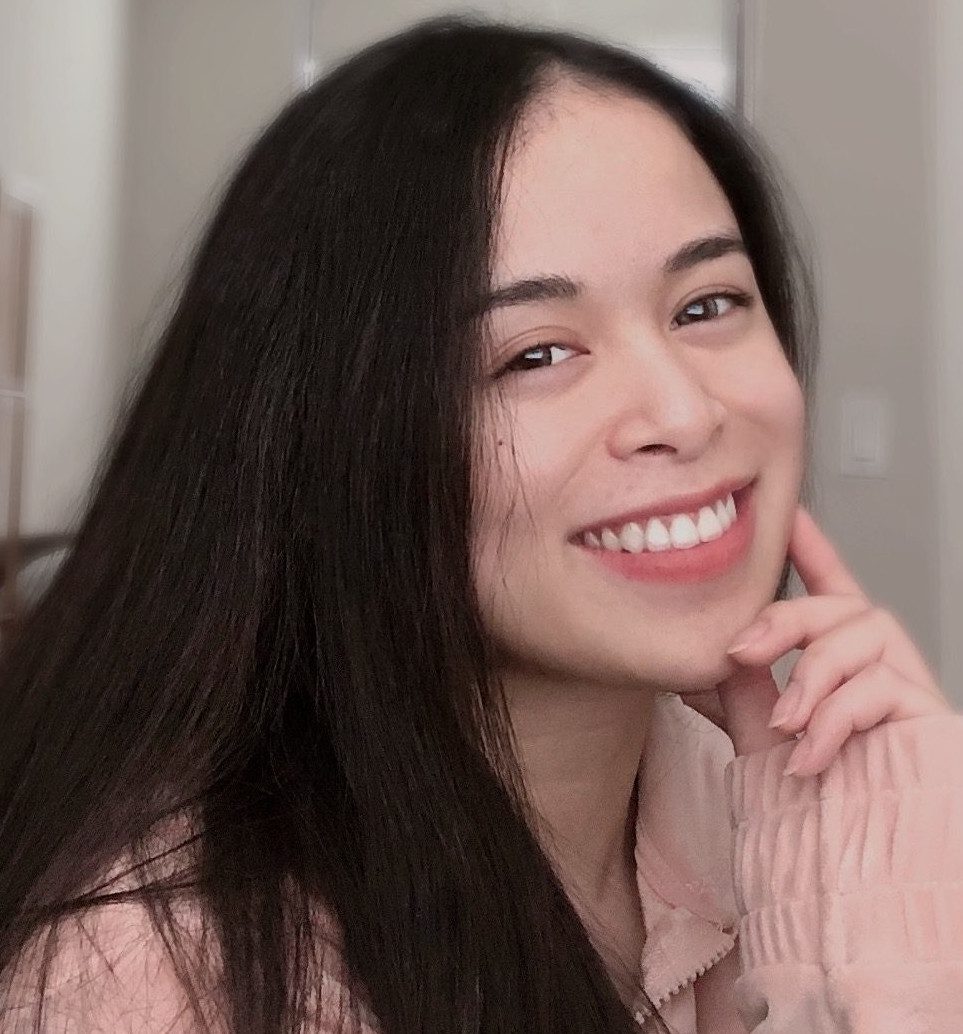
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
In addition, the clear() method is used to remove all items from a HashMap. clear() accepts no parameters. Here’s an example of the clear() method in action:
… currencyCodes.clear(); System.out.println(currencyCodes); …
Our code returns an empty HashMap: {}
.
Replace HashMap Elements
The replace() method is used to replace a value associated with a specific key with a new value. replace() accepts two parameters: the key of the value you want to replace, and the new value with which you want to replace the old value.
Suppose, for instance, that we wanted to replace the value United Kingdom
with Great Britain
in our HashMap. We could do so using this code:
… currencyCodes.replace("GBP", "Great Britain") System.out.print(currencyCodes); …
When we execute our code, the value of the key GBP
(which is United Kingdom
in this case) is replaced with Great Britain
and our program returns the following:
{GBP=Great Britain,USD=United States}
Iterate Through a HashMap
Additionally, you can iterate through a HashMap in Java. HashMap offers three methods which can be used to iterate through a HashMap:
- keySet() is used to iterate through the keys in a HashMap.
- values() is used to iterate through the values in a HashMap.
- entrySet() is used to iterate through the keys and values in a HashMap.
The easiest way we can iterate through a HashMap is to use a for-each
loop. If you’re interested in learning more about Java for-each loops, you can read our tutorial on the topic here.
Suppose we want to print out every value in our currencyCodes
”HashMap to the console so that we can show the currency conversion business a list of the currencies they offer that are stored in the HashMap. We could use the following code to do this:
import java.util.HashMap; class CurrencyExchange { public static void main(String[] args) { HashMap<String, String> currencyCodes = new HashMap<String, String>(); currencyCodes.put("GBP", "Great Britain"); currencyCodes.put("USD", "United States"); for(String value : currencyCodes.values()) { System.out.println(value); } } }
When we run our code, the following response is returned:
Great Britain
United States
In our code, we use a for-each
loop to iterate through every item in the list of currencyCodes.values()
. Then we print out each item on a new line.
If we wanted to iterate through every key and print out the name of each key in our HashMap, we could replace values()
with keySet()
in our code above. Here’s what our program would return:
GBP
USD
Conclusion
The Java HashMap class is used to store data using the key/value collection structure. This structure is useful if you want to store two values that should be associated with each other.
This tutorial covered the basics of HashMaps. We showed you how to create a HashMap, and explored a few examples of common HashMap methods in action. Now you’re equipped with the information you need to work with Java HashMaps like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.