Loops in programming are used to automate similar tasks that will be repeated multiple times. For instance, if you’re creating a program that merges together the price and name of all lunchtime menu items for a restaurant, you may want to use a loop to automate the task.
In Java, for
loops are used to repeat the execution of a block of code a certain number of times. Whereas while
loops run until a condition evaluates to false, for
loops run for a certain number of iterations.
This tutorial will discuss how to use for
and foreach
loops in Java, with reference to a few examples of for
and foreach
loops in Java programs.
For Loop Java
Suppose you want to print the contents of an array that contains 100 items to the console. Or suppose you want to raise the price of everything in your store by 5 cents. Instead of doing these tasks manually, you would want to use a loop.
In Java, for
loops are used to run a specific task multiple times. Here’s the syntax for a for
loop in Java:
for (initialization; expression; updateCounter) { // Execute code }
Our loop has three components:
- initialization is the statement used to initialize a variable that keeps track of how many times the loop has executed.
- expression is the condition the for loop will evaluate. This must be a boolean expression whose condition is true or false.
- updateCounter is executed after the code block and updates the initialization variable.
These components are all required.
Let’s walk through an example to illustrate how for
loops work in Java. Suppose we are creating a program that allows a third-grader to find out the 10 times table. This program is used to help them revise for their upcoming math test.
Instead of calculating 1 * 10, 2 * 10, etc. individually, we can instead use a “for” loop to calculate each value in the 10 times table. Here’s the code we would use to calculate all values from 1-10 in the 10 times table:
public class TimesTable { public static void main(String[] args) { for (int i = 1; i <= 10; ++i) { int answer = i * 10; System.out.println(i + " x 10 is " + answer); } } }
Our code returns:
1 x 10 is 10 2 x 10 is 20 3 x 10 is 30 4 x 10 is 40 5 x 10 is 50 6 x 10 is 60 7 x 10 is 70 8 x 10 is 80 9 x 10 is 90 10 x 10 is 100
As you can see, our program has calculated all values in the 10 times table up to 10 * 10. Let’s explore the code and discuss how it works step-by-step.
First, we declare a class called TimesTable, which stores our code for this program. Then we create a for
loop that executes the code within the loop 10 times over. Here are the three components of our for
loop:
int i = 1
tells our code to start counting from the value 1.i <= 10
tells our code to execute thefor
loop only if the value ini
is less than or equal to 10.++i
adds 1 to thei
variable after thefor
loop has executed. This is called an increment counter.
Then, we print out a statement with the math problem and its answer. This statement is formatted as [i counter number] x 10 is
, followed by the answer to the problem.
For Each Loop Java
When you’re working with an array and collection, there is another type of for
loop you can use to iterate through the contents of the array or collection with which you are working. This is called a for-each
loop, or an enhanced for loop.
The syntax for the for-each
loop is as follows:
for (dataType item : collection) { // Execute code }
There are three components in our for-each
loop:
- dataType is the type of data our item uses.
- item is a single item in the collection.
- collection is an array or collection variable through which the
for
loop will iterate.
The for-each
loop iterates through each item in the collection, stores each item in the item
variable, then executes the code stored within the loop body. Let’s walk through an example to discuss how this works.
Suppose you are operating a coffee shop, and you want to reduce the price of every coffee by 25 cents for a flash-sale. You have a list that contains the prices of each coffee, and you want to subtract 25 cents from each price.
You could do so using the following code:
public class ReducePrices { public static void main(String[] args) { double[] prices = {2.50, 2.75, 3.00, 2.75, 2.75, 2.30, 3.00, 2.60}; for (double i : prices) { double new_price = i - 0.25; System.out.println("The new price is " + new_price); } } }
Our code returns:
The new price is 2.25 The new price is 2.5 The new price is 2.75 The new price is 2.5 The new price is 2.5 The new price is 2.05 The new price is 2.75 The new price is 2.35
As you can see, our program has reduced the price of every coffee by 50 cents. Now we have the data we need to change our coffee prices for the flash sale.
Let’s walk through our code step-by-step. First, we declare a class called ReducePrices, which stores our code for this example. Then we declare an array called prices
which stores the prices for our coffees. This array is declared as an array of doubles, or decimal-based numbers.
Then we create a foreach
loop that runs through each item in the prices
array. For each item in the array, our program reduces the price of the coffee by 50 cents and assigns the reduced price to the variable new_price
. Then, our program prints out “The new price is “, followed by the new price of the coffee.
Conclusion
The for
loop is used in Java to execute a block of code a certain number of times. The for-each
loop is used to run a block of code for each item held within an array or collection.
In this tutorial, we explored how to use the for
loop and the for-each
loop in Java. We also referred to an example of each of these loops in action. We also discussed how each example worked step-by-step.
You’re now equipped with the knowledge you need to use Java for
and for-each
loops like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
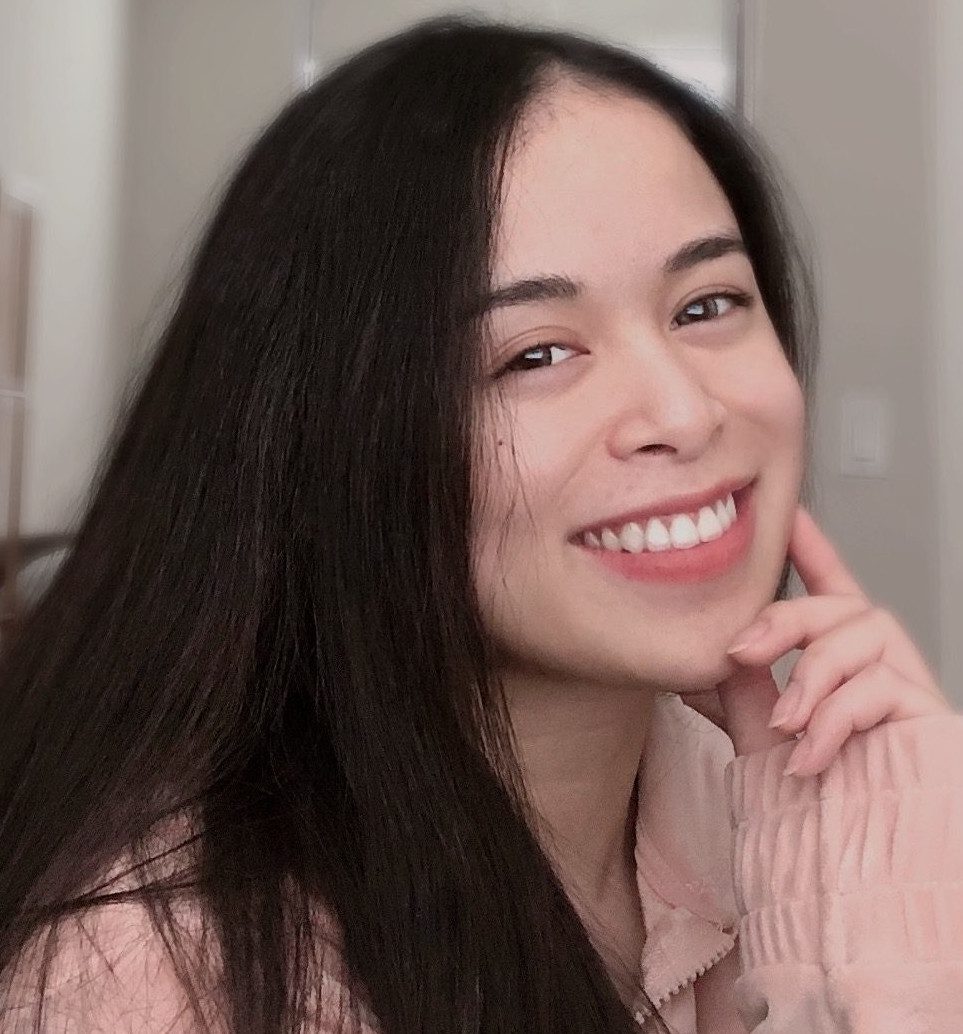
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot