There are a number of scenarios where you’ll want to work with files in Java. For instance, you may want to create a file to store the output of a program, or perhaps you decide that you want to read data from a file which is then processed by a program.
That’s where the java.io library comes in. The java.io library offers a number of methods that are used to work with files in Java.
This tutorial will discuss how to use the Java File, FileReader, and FileWriter classes and their core methods. This tutorial will also refer to an example of each of these methods in use, to demonstrate how you can use them in your code.
Java Files
Files are items in a computer that store a particular piece of information. For instance, a file could store a list of student names in a math class, or a list of ingredients used to bake a coffee cake. Directories, on the other hand, are folders that store collections of files and other directories.
The java.io library includes a number of packages that can be used to work with files and directories in Java. For this tutorial, we are going to focus on the Java File, FileReader, and FileWriter packages.
Because the methods offered by these packages are part of an external package, we first need to import these packages before we can use them in our code.
Here’s the code we can use to import the File, FileReader, and FileWriter classes into our program:
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
Now that we know how to import the Java file classes we will be working with in this tutorial, we’re ready to proceed.
Create a Java File
The Java File class is used to create an empty file in Java.
Before we can create a file, however, we need to create a File object. The file object is a representation of a specific file or folder in our code. That said, the file object does not create a file in itself. We first need to create a file object, then we can use it to create a file.
Here’s the syntax we can use to create a file object in Java:
File fileName = newFile(String filePath);
In this example, we have created a file system object called fileName
. This file object relates to the file or folder stored at the file path we specified in the filePath
variable.
To create a file in Java, we can use the createNewFile()
method. createNewFile()
creates a new file at the file path you specify. The method returns true if a new file is created and false if there is already a file in the location you have specified.
Suppose we are creating a data analysis program that analyzes historical stock performance for the S&P 500 in 2019. Before we analyze our data, we want to create a new file that will store the insights our program creates. We could use this code to create the file which will store the results of our analysis:
import java.io.File; class CreateFile { public static void main(String[] args) { File resultsFile = new File("/home/data_analysis/2019sandp500/result.txt"); boolean fileCreated = resultsFile.createNewFile(); if (fileCreated) { System.out.println("The results file has been created."); } else { System.out.println("The results file already exists."); } } }
When we run our code, a file at the path /home/data_analysis/2019sandp500/result.txt
is created. Then, the following response is returned to the console:
The results file has been created.
However, if the file we are trying to create already exists, this message would be returned to the console:
The results file already exists.
Let’s break down our code. First, we import the java.io.File
method which includes the file methods we will use in our code. Then we create a class called CreateFile
which stores the code for our program.
On the first line of our main program, we create a file object called resultsFile
, which represents the file at the file path /home/data_analysis/2019sandp500/result.txt
. Then we use the createNewFile()
method to create a new file at the file path we specified. The boolean result of the createNewFile()
method is stored in the variable fileCreated.
On the next line, we create an if
statement. If fileCreated is equal to true, the message The results file has been created
. will be printed to the console; otherwise, the message The results file already exists
. will be printed to the console. In this case, the results file does not exist, so our code created the new file and printed The results file has been created.
to the console.
Read a Java File
The read()
method in the Java FileReader class is used to read the contents of a Java file.
Suppose we have a file called /home/data_analysis/2019sandp500/raw_message.txt
that we want to access in our code. This file contains the following text:
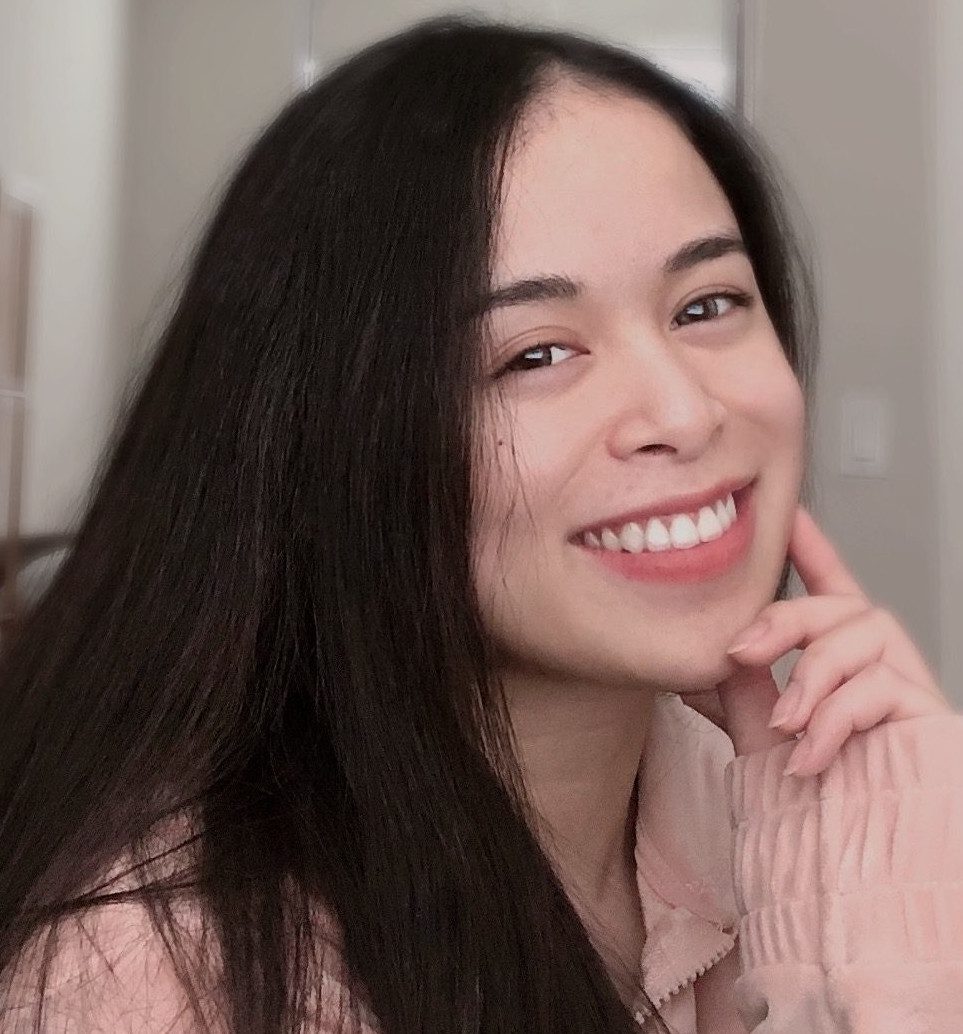
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
JAVA S&P 500 ANALYSIS PROGRAM
We could read this file using the following code:
import java.io.FileReader; class Main { public static void main(String[] args) { char[] array = new char[100]; try { FileReader fileContents = new FileReader("/home/data_analysis/2019sandp500/raw_message.txt"); fileContents.read(array); System.out.println(array); fileContents.close(); } catch(Exception error) { error.getStackTrace(); } } }
Our code returns:
JAVA S&P 500 ANALYSIS PROGRAM
In our program, we used the FileReader class to create a file object representing the contents of the file stored at /home/data_analysis/2019sandp500/raw_message.txt.
Then, we used the read()
method to read the contents of the file into an array. Finally, we printed out the contents of the array to the console and used close()
to close our file.
Write to a Java File
The write()
method from the FileWriter
package is used to write to a file in Java.
Suppose we want to write today’s date to the top of the results file that stores the results of our data analysis program. This file is stored at the file path /home/data_analysis/2019sandp500/results.txt
. We could do so using this code:
import java.io.FileWriter; class Main { public static void main(String args[]) { String date = "Thursday, March 12th"; try { FileWriter writeToFile = new FileWriter("/home/data_analysis/2019sandp500/results.txt"); writeToFile.write(date); writeToFile.close(); System.out.println("The date has been written to the file."); } catch (Exception error) { error.getStackTrace(); } }
Our code writes Thursday, March 12th
to the file /home/data_analysis/2019sandp500/results.txt
and prints the following to the console:
The date has been written to the file.
Here are the contents of the results.txt file which we wrote in our program:
Thursday, March 12th
In our above example, we used the FileWriter
method to write a sentence to a file in Java. First, we declared a variable called writeToFile()
which creates a representation of the file stored at the file path /home/data_analysis/2019sandp500/results.txt
. The write()
method is used to write a string to a file, then we use the close() method
to close the file.
Delete a File in Java
The Java File package offers a method that is used to delete a file or directory—delete().
delete()
returns true if the file specified is deleted, and false if the file does not exist. In addition, the delete()
method can only delete directories with no contents.
Suppose we want to delete the results.txt
file at the start of our program so that we can write new data to the file later in our program. We could do so using this code:
import java.io.File; class Main { public static void main(String[] args) { File results = newFile("/home/data_analysis/2019sandp500/results.txt"); boolean deleteFile = results.delete(); if (deleteFile) { System.out.println("results.txt has been deleted."); } else { System.out.println("results.txt has not been deleted."); } } }
Our code deletes the contents of the results.txt file and prints the following to the console:
results.txt has been deleted.
In this example, we created an object of File called results which represents the contents of the results.txt file. Then, we used the delete()
method to delete the contents of the file.
If the results.txt file was successfully deleted — like it was in the above example — the message results.txt has been deleted
. is printed to the console. Otherwise, results.txt has not been deleted
. is printed to the console.
Conclusion
The Java File package is used to create and delete files, the FileReader package is used to read the contents of a file, and the FileWriter package is used to write to a file.
This tutorial discussed, with reference to examples, how to use the File, FileReader, and FileWriter Java packages to work with files in your code. Now you’re ready to start working with files in Java like a professional programmer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.