Java is one of the most popular programming languages and one of the best you can start with when entering the world of programming. It is the foundation for many network applications and software including mobile apps, games, and enterprise software. Our day-to-day interaction with computers and machines also involves relying on the Java programming language.
For a beginner in programming, Java exercises are a great tool with which you can learn and deepen your knowledge of coding. The various exercise examples and solutions shared below will help budding Java programmers improve their coding skills.
Java Exercises to Help You Learn Java
These Java exercises not only help you to code better but they also provide a great way to master basic programming constructs. Such constructs include break and continue with loop, recursion, Java source code, standard Java API, and Java operators like logical and arithmetic operators.
To build your data structure and algorithm skills, below are 10 beginner Java programming exercises and their sample solution. These exercises will help you hone your knowledge of Java arithmetic, command-line arguments, and the proper usage of an If-else statement. They may look simple but are actually tricky and will challenge you.
10 Java Exercises and Practice Problems (With Solutions)
1. Print an American Flag
In this exercise, you will write a Java program to input patterns of asterisks and equal signs and print out an American flag.
Solution: This exercise will require you to hard-code the asterisks and equal signs into the code and return each line of code.
2. Sum of Two Numbers
In this exercise, Java programmers must write a Java program to print the sum of two numbers. Though a basic exercise, learning how to add and compute numbers through code is an important part of coding in Java.
Solution: The solution to this exercise will return the added value of two variables.
3. Find the Maximum
Finding the maximum number in this exercise is done through the use of an If statement. If statements are vital to Java and many other programming languages.
Solution: Declare an If statement that compares the variable i to a list variable, where whichever is higher (greater than) will be returned.
4. Finding an Average
This exercise requires programmers to find the average number from a list of integers and display the result.
Solution: The code will request two numbers to be input, and then calculate and return the average of the input numbers.
5. Convert Celsius to Fahrenheit
In this exercise, programmers will code a formula to display temperatures in Celsius (C) and Fahrenheit (F) up to 100 degrees Fahrenheit. This program will require multiple command-line arguments.
Solution: This exercise will require the programmer to write code to convert C to F first, and then to loop through the conversion 100 times.
6. Even and Odd Counter
In this exercise, you must employ the use of booleans to determine how many even integers and how many odd integers are in a group of randomly generated numbers.
Solution: This exercise requires the use of a For loop containing an If-Else statement to determine whether the randomly generated number is even or odd. The program should return the number of even numbers and the number of odds numbers generated from the random list.
7. Area and Perimeter of Rectangle
Calculate the perimeter and area of a rectangle in this exercise. For this exercise, hard code two inputs, the height and width measurements of a rectangle, to return the expected result. This program will require multiple command-line arguments.
Solution: The solution should calculate both the perimeter and the area using sample hard-coded inputs. The sample output should return the formula used to find the area and perimeter.
8. Reverse a String
This exercise will require you to build a program that reverses a valid input string. For example, an input of “hello” would be returned as “olleh”.
Solution: The solution to this exercise will require the use of a string array to separate the characters and a For loop to return them in reverse order.
9. Character Counter
A Java program to calculate and display the number of times a single character appears in a valid string entered by the user. This exercise requires code for searching the string and code for identifying the string letter to search. This program will require multiple command-line arguments.
Solution: The code should request a string input from a user and then return the sample output along with the number of a single character.
10. Print Odd Numbers from 1 to 99
In this exercise, you must write code to print a numerical list of odd numbers between the positive integer 1 and 99.
Solution: This exercise requires a For loop to determine whether the numbers are divisible by 2. If they are not, then the code will determine it is an odd number and will print it before looping back to assess the next positive integer.
How to Get Help with Java
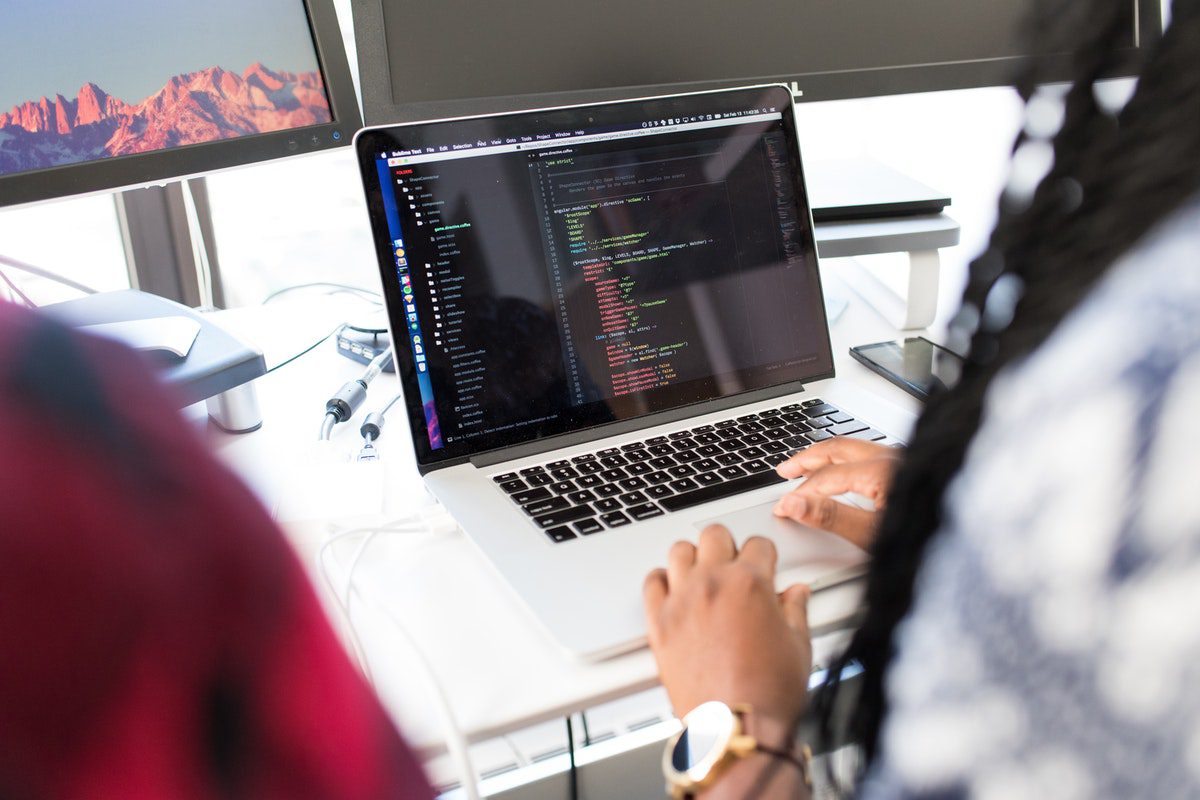
If you want to learn a coding language like Java, you will need to put in some time and effort. Programming contests, quizzes, exercises, and other forms of classes will improve your Java coding style.
Specifically, actually attempting programming exercises is one of the best ways to learn to code. However, you can even play coding games to learn Java. Discover other ways to develop your skills in Java below.
Java Exercises
Exercises in the Java programming language concepts allow you to practice both basic and more complex tasks. The tasks may involve writing a program showing a binary search tree, loop, array variable, or array of negative or positive integer values. It may also require you to compute and show an array element, prove a conditional statement, or swap array content.
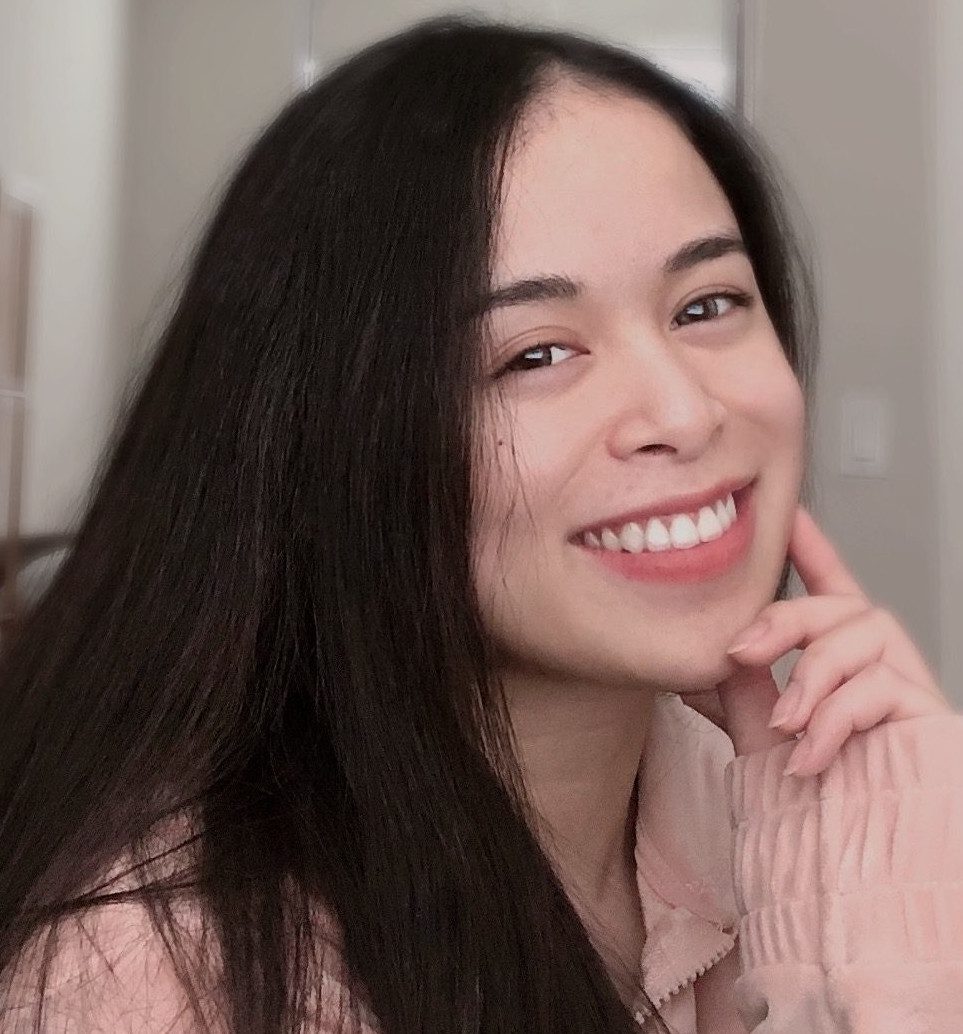
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Java Projects
You will be able to learn many concepts from project-based learning. Java projects refer to applying programming to solve a problem. Practicing with projects helps you understand the underlying theories of the language and apply them to problems in the real world. Some project ideas include creating a temperature converter or supermarket billing system where the tax-inclusive price is automatically calculated. You could also create a chat application.
Java Quizzes
Quizzes involve multiple-choice questions about Java. They help you cover many Java concepts and get acquainted with some basic Java terms such as object, loop, array, class, methods, series, arguments, entire array, class names, recursive functions, and others. You will be provided answers to the quizzes afterward so that you can check your answers and learn from your mistakes. There are many resources online that offer free Java quizzes.
Java Forums and Blogs
You can reply on forums and blogs when you run into problems with your Java programs. They usually consist of communities of Java programmers from different parts of the world with a wide range of skills. You can post content, expand your knowledge, engage with the Java community, and help others. Thankfully, there are many different forums and Java blogs that you can learn from.
Where Can I Practice Java?
As a beginner in Java, there are numerous online platforms where you practice and master Java. Some may be free, while others may require a registration fee. If you are looking to progress your Java skills, then you need to practice often. It can be difficult to choose the right resources, especially if you’re learning the language from scratch. However, to help you get started, here are the top five free websites to practice Java
Websites to Practice Java
- CodeAbbey. This is the popular go-to for beginners learning Java programming. You will find plenty of coding challenges that you can use to practice. The platform provides hundreds of practice problems, as well as user-ranked tasks and games, that users can sift through to hone their skills.
- Guru99. Guru99 is a very useful platform for beginner Java programmers. It contains code assignments, which you can practice after you have completed each tutorial. The website will help you learn both the basic and advanced concepts of Java.
- Code.org. Over 60 million users of different age groups learn Java at various difficulty levels on Code.org. The site also has online teachers always ready to assist you. Note that you are required to complete an introductory course before starting your learning journey.
- CodeWars. If you have little programming experience and are looking to hone your skills, then CodeWars is the place for you. It has small and straightforward problems that are suitable for beginners. It also offers users the opportunity to participate in coding challenges and partner with friends while doing so.
- Sololearn. A wide range of topics is covered in the Java course content on this website, including practice problems you can try. Regardless of your experience level, you can learn how to write real and functional code due to the wealth of free coding classes and interactive challenges. There is also a Sololearn app you can learn with.
What’s the Best Way to Learn Java?
Attending a Java bootcamp is the best way to learn Java. You will be trained in practical Java courses that include exercises and challenges to test your level of knowledge. This method helps you grow from solving basic problems to conducting complex exercises. You will engage in interactive classes and be able to track your growth with quizzes and projects.
Java Exercises FAQ
No, you cannot learn Java in one week. It requires gradual learning and frequent practicing. However, you can learn to code in Java quickly by joining an intensive coding bootcamp.
No, you can learn Java without a strong command of mathematics. However, understanding certain mathematical functions may help some Java programmers progress their skills more quickly. Math concepts such as standard deviation are helpful to understand.
You must know the basics of how to use a computer to learn Java. If you already have knowledge of the C++ programming language, Java will be relatively easy to pick up.
Java is relatively easy to learn when compared with other popular programming languages. However, it is still not easy for beginners, but you can learn it within a short time if you put in the effort.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.