A Java enum is a data type that stores a list of constants. You can create an enum object using the enum keyword. Enum constants appear as a comma-separated list within a pair of curly brackets.
An enum, which is short for enumeration, is a data type that has a fixed set of possible values.
Enums are useful if you’re working with a value that should only hold a specific value that is contained within a list of values. For instance, an enum would be used if you wanted to store a list of coffee sizes sold at a store.
This tutorial will walk through the basics of enums in Java. We’ll refer to a few examples of an enum class in a Java program to help you get started.
Java Enum Syntax
A Java enum represents a list of constants. A variable assigned from an enum can only have a value that appears in the enum. Enums help developers store data that they know will not change.
Let’s say you decide that a Java variable that stores employee pay grades can only have one of five values. Or, you decide a variable that stores employee contracts can only store Part-time, Full-time, or Zero-hours. In these cases, you would want to use an enum to store data.
Enums are declared using the “enum” type. Here’s the syntax of the “enum” keyword:
enum name { VALUE1, VALUE2, VALUE3 }
Let’s break down this syntax:
- enum tells our program we want to declare an enumeration.
- name is the name of our enum.
- VALUE1, VALUE2, VALUE3 are the set of constant values our enum stores. These values are usually written in uppercase.
Why Use Enums in Java?
Using enums allows you to express an algorithm in a more readable way to both yourself and the computer.
Writing an enum tells the computer a variable can only have a specific number of values. It also tells you, the coder, that this is the case, which will make it easier to understand your code. If you see a variable that uses an enum, you know that the variable can only have one of a limited number of values.
Additionally, enums allow you to more effectively use constants. In fact, enum was introduced to replace int constants in Java, which spanned multiple lines and were difficult to read. Here’s an example of an old int constant in Java:
Our code takes up five lines to declare these constants. But by using an enum, we can reduce our code to three lines. In the example below, we declare a list of enum constants:
class IntConstant { public final static int ECONOMY = 1; public final static int BUSINESS = 2; public final static int FIRST_CLASS = 3; }
Our code takes up five lines to declare these constants. But by using an enum, we can reduce our code to three lines. In the example below, we declare a list of enum constants:
class AirplaneClasses { ECONOMY, BUSINESS, FIRST_CLASS }
This code is simpler and easier to read.
Declaring a Java Enum
We are building an app that wait staff can use to submit a coffee order to the baristas at a coffee shop.
When a barista inserts a value for the size of the drink, we only want there to be three possible options. These options are: SMALL, REGULAR, and LARGE. We could use an enum to limit the possible sizes of a drink to those options:
enum Sizes { SMALL, REGULAR, LARGE }
In this example, we have declared an enum called Sizes which has three possible values. Now that we have declared an enum, we can refer to its values in our code.
Java Enum Example
We are writing a program that prints out the size of coffee a customer has ordered to the console. This value will be read by the barista who prepares the customer’s drink.
We could use the following code to print out the size of the coffee a customer has ordered to the console:
enum Sizes { SMALL, REGULAR, LARGE } class PrintSize { Sizes coffeeSize; public PrintSize(Sizes coffeeSize) { this.coffeeSize = coffeeSize; } public void placeOrder() { switch(coffeeSize) { case SMALL: System.out.println("This coffee should be small."); break; case REGULAR: System.out.println("This coffee should be REGULAR."); break; case LARGE: System.out.println("This coffee should be large."); break; } } } class Main { public static void main(String[] args) { PrintSize order173 = new PrintSize(Sizes.SMALL); order173.placeOrder(); } }
Our code returns:
This coffee should be small.
First, we declare an enum called Sizes. This enum can have three values: SMALL, REGULAR, or LARGE. Next, we declared a class called PrintSize. This class accepts the size of the customer’s drink and prints out the drink size to the console.
In our main program, we declare an object called order173,” which uses the PrintSize class. We passed the Java parameter Sizes.SMALL through our code. This tells our program to assign the value SMALL to the variable coffeeSize in the PrintSize class.
Then we use order173.placeOrder() to execute the code within the switch case statement in the PrintSize class. This evaluates the value of the “coffeeSize” variable against three cases. A message is printed to the console depending on the size of the coffee a customer has ordered.
We specified that the customer ordered a small coffee. Our code prints out, “This coffee should be small.” to the console.
If you’re looking to learn more about Java switch case statements, you can read about them in our tutorial on the Java switch statement.
Java Enum Methods
The Java enum class includes a number of predefined methods that are used to retrieve and manipulate values that use the enum class. Below we have broken down five of the most commonly used enum methods.
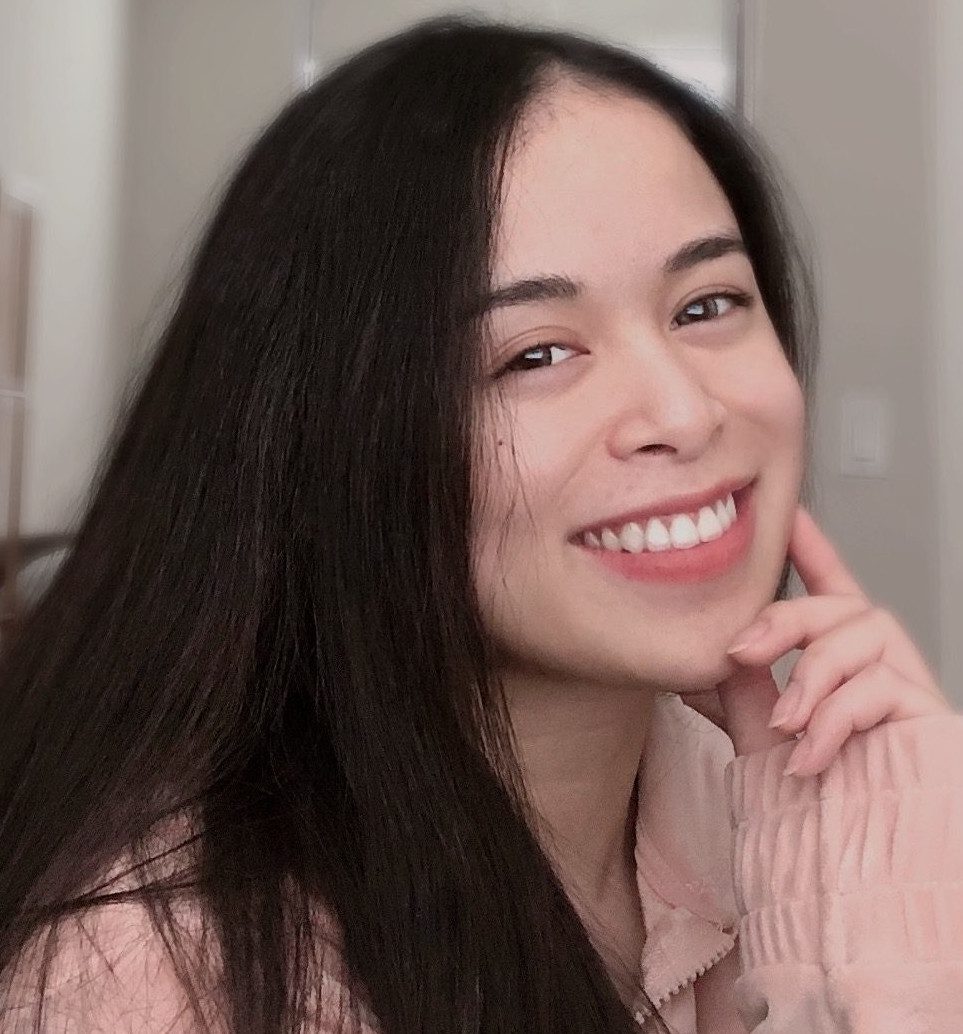
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
compareTo()
compareTo() compares the constants in an enum lexicographically and returns the difference between their ordinal values. Here’s an example of compareTo() being used with an enum value from our above example:
int difference = Sizes.SMALL.compareTo(Sizes.LARGE); System.out.println(difference);
Our code returns the difference between the SMALL
and LARGE
strings based on their ordinal value. In this case, our code returns:
-2
toString()
toString() converts the name of an enum to a string. Here’s an example of toString() being used to convert the LARGE
enum value to a string:
String large_string = LARGE.toString(); System.out.println(large_string);
Our code returns:
“LARGE”
name()
The name() method returns the name used to define a constant in an enum class. Here’s an example of the name() method being used to return the defined name of the REGULAR coffee size:
String regular_name = name(REGULAR); System.out.println(regular_name);
Our code returns:
“REGULAR”
values()
The values() method returns a Java array which stores all the constants in an enum. Here’s an example of the values() method in action:
Sizes[] sizeList = Sizes.values();
valueOf()
valueOf() accepts a string and returns the enum constant, which has the same name. So, if we wanted to retrieve the enum constant with the name REGULAR,
we could do so using this code:
String regular_constant = Sizes.valueOf("REGULAR"); System.out.println(regular_constant);
Our code returns:
REGULAR
Conclusion
An enum, short for an enumeration, is a Java data type that has a fixed set of values.
Enums are useful if you are working with variables that should only be capable of storing one out of a select range of values.
After practicing what you have read in this tutorial, you’ll be an expert at using enums in Java. To learn more about coding in Java, read our complete guide on How to Code in Java.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.