When you’re working with arrays in Java, you may decide that you want to create a copy of an array. For instance, if you’re running a coffee shop and want to create a seasonal menu, you may want to create a copy of your original menu on which the new menu can be based.
In Java, there are a number of ways in which you can copy an array. This tutorial will explore four common methods to copy arrays and discuss how they work line-by-line. After reading this tutorial, you’ll be a master at copying arrays in Java.
Java Arrays
In Java, an array is a container that holds values that hold one single type. For example, an array could be used to store a list of books, or a list of scores players have earned in a game of darts.
Arrays are useful when you want to work with many similar values because you can store them all in one collection. This allows you to condense your code and also run the same methods on similar values at one time.
Suppose we want to create an array that stores the coffees sold at our coffeeshop. We could do so using this code:
String[] coffees = {“Espresso”, “Mocha”, “Latte”, “Cappuccino”, “Pour Over”, “Flat White”};
In this example, we declare an array called coffees
which stores String values. Our array contains six values.
Each item in an array is assigned an index number, starting from 0, which can be used to reference items individually in an array.
Now that we have explored the basics of Java arrays, we can discuss methods you can use to copy the contents of your array.
Copy Array Using Assignment Operator
One of the most common clone methods used to copy an array is to use the assignment operator.
The assignment operator is used to assign a value to an array. By using the assignment operator, we can assign the contents of an existing array to a new variable, which will create a copy of our existing array.
Let’s return to the coffeeshop. Suppose we want to create a copy of the coffees
array upon which we will base our summer coffee menu. We could use this code to create a copy of the array:
class CopyAssignment { public static void main(String[] args) { String[] coffees = {"Espresso", "Mocha", "Latte", "Cappuccino", "Pour Over", "Flat White"}; String[] summer_coffees = coffees; for (String c: summer_coffees) { System.out.print(c + ","); } } }
Our code returns:
Espresso, Mocha, Latte, Cappuccino, Pour Over, Flat White,
Let’s break down our code. On the first line of code inside our CopyAssignment class, we declare an array called coffees
which stores our standard coffee menu.
On the next line, we use the assignment operator to assign the value of coffees
to a new array called summer_coffees
. Then we create a “for-each” loop that iterates through every item in the summer_coffees
array and prints it out to the console.
There is one drawback to using this method: if you change elements of one array, the other array will also be changed. So if we changed the value of Latte
to Summer Latte
in our summer_coffees
list, our coffees
list would also be changed.
Loop to Copy Arrays
The first approach we discussed to copy an array — using the assignment operator — creates what is called a shallow copy.
This is the case because we have assigned an existing array object to a new one, which means that when we change any object, they will both be changed — the two objects are linked.
However, we often need to create a deep copy. Deep copies copy the values from an existing object and create a new array object. When you create a deep copy, you can change your new array without affecting the original one.
One approach that can be used to create a deep copy is to create a for
loop which iterates through the contents of an array and creates a new array.
Suppose we want to create a deep copy of our coffees
array called summer_coffees.
This needs to be a deep copy because we plan on changing the contents of the summer_coffees
array to reflect the new coffees we will offer in the summer months.
Here’s the code we would use to create a deep copy using a for
loop:
import java.util.Arrays; class LoopCopy { public static void main(String[] args) { String[] coffees = {"Espresso", "Mocha", "Latte", "Cappuccino", "Pour Over", "Flat White"}; String[] summer_coffees = new String[6]; for (int i = 0; i < coffees.length; ++i) { summer_coffees[i] = coffees[i]; } System.out.println(Arrays.toString(summer_coffees)); } }
When we run our code, the output is as follows:
[Espresso, Mocha, Latte, Cappuccino, Pour Over, Flat White]
As you can see, our code has created a copy of our original array. Let’s explain how it works step-by-step:
- We import
java.util.Arrays
which includes the toString() method, we’ll use to print our array to the console at the end of the example.
- We declare an array called
coffees
which stores the list of coffees on our standard menu. - We initialize an array called
summer_coffees
which will store six values. - We use a for loop to iterate through every item in the
coffees
list. - Each time the for loop runs, the item at index value
i
in summer_coffees will be assigned the item at the index valuei
in coffees. - We use Arrays.toString() to convert
summer_coffees
to a string, then print out the new array with our copied elements to the console.
Using copyOfRange() Java Methods
The Java copyOfRange() method is used to copy Arrays.copyOfRange() is part of the java.util.Arrays class. Here’s the syntax for the copyOfRange() method:
import java.util.arrays; DataType[] newArray = Arrays.copyOfRange(oldArray, indexPos, length);
Let’s break down the syntax for the copyOfRange() method:
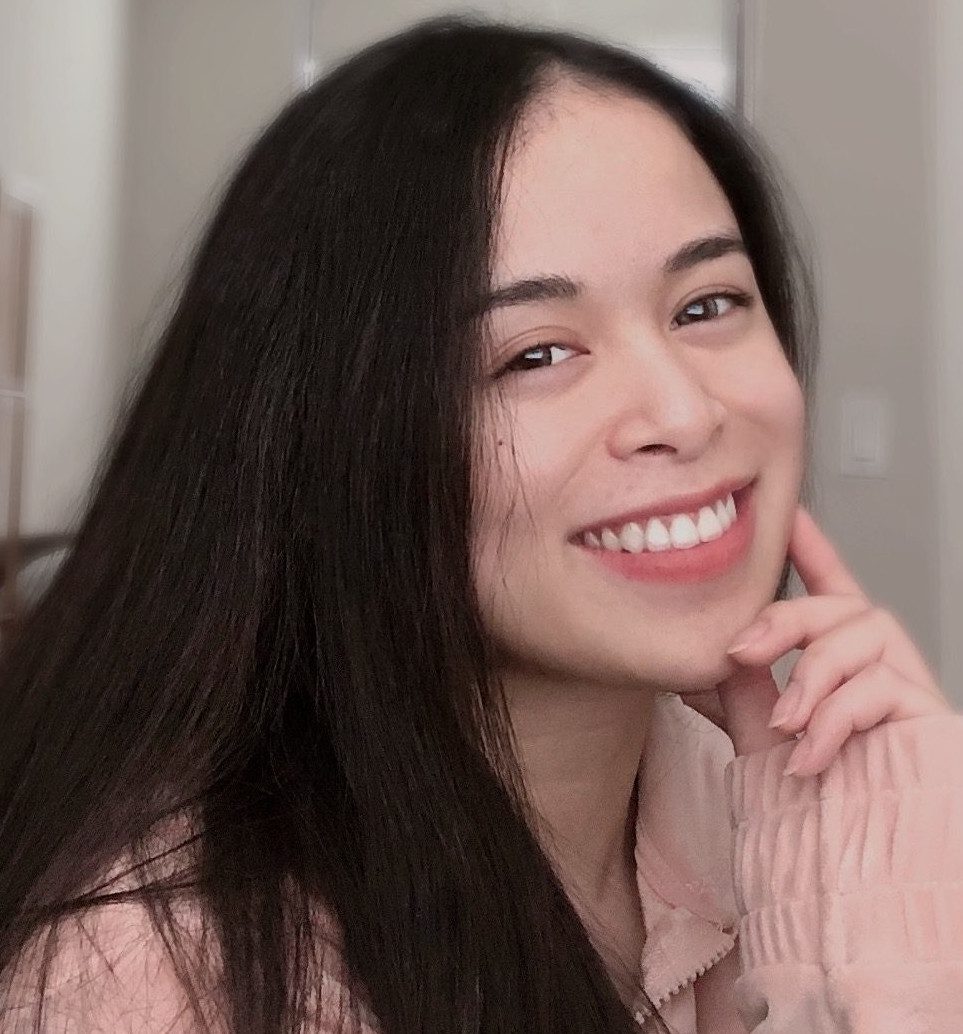
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
- DataType is the type of data the new array will store.
- newArray is the name of the new array.
- oldArray is the array whose values you want to copy to the
newArray
. - indexPos is the position at which the copy operation should begin in the
oldArray
. - length is the number of values that should be copied from
oldArray
tonewArray
.
Let’s walk through an example to demonstrate the copyOfRange() method in action. Say we want to create a copy of our coffees
array from earlier. We could do so using this code:
import java.util.Arrays; class CopyUsingRange { public static void main(String[] args) { String[] coffees = {"Espresso", "Mocha", "Latte", "Cappuccino", "Pour Over", "Flat White"}; String[] summer_coffees = Arrays.copyOfRange(coffees, 0, coffees.length); System.out.println("Summer coffees: " + Arrays.toString(summer_coffees)); } }
Our code returns:
Summer coffees: [Espresso, Mocha, Latte, Cappuccino, Pour Over, Flat White]
Let’s break down our code:
- We import the
java.util.Arrays
library which stores the copyOfRange() and toString() methods that we’ll use in our example. - We declare an array called
coffees
which stores the coffees on our standard menu. - We declare an array called
summer_coffees
and use the copyOfRange() method to create a copy of thecoffees
array. The parameters we specify are as follows:- coffees is the name of the array we want to copy.
- 0 specifies we want to copy values starting at index position 0 from the
coffees
array. - coffees.length specifies we want to copy every value in the list.
- We print out “Summer coffees: “ followed by the resulting array called
summer_coffees
to the console.
Now we’ve created a copy of our “coffees
list called summer_coffees
.
Using arraycopy() Java Methods
The arraycopy() method is used to copy data from one array to another array. The arraycopy() method is part of the System class and includes a number of options which allow you to customize the copy you create of an existing array.
Here’s the syntax of the arraycopy() method:
System.arraycopy(sourceArray, startingPos, newArray, newArrayStartingPos, length);
Let’s break down this method. copyarray() accepts five parameters:
- sourceArray is the name of the array you want to copy.
- startingPos is the index position from which you want to start copying values in the
source_array
. - newArray is the name of the new array where values will be copies.
- newArrayStartingPos is the index position at which the copied values should be added.
- length is the number of elements you want to copy to the
new_array
.
Let’s return to the coffeeshop. Suppose we wanted to copy every value in our coffees
array to a new array called summer_coffees
. We could do so using this code:
import java.util.Arrays; class ArrayCopyMethod { public static void main(String[] args) { String[] coffees = {"Espresso", "Mocha", "Latte", "Cappuccino", "Pour Over", "Flat White"}; String[] summer_coffees = new String[6]; System.arraycopy(coffees, 0, summer_coffees, 0, coffees.length); System.out.println("Summer coffees: " + Arrays.toString(summer_coffees)); } }
Our code returns:
Summer coffees: [Espresso, Mocha, Latte, Cappuccino, Pour Over, Flat White]
Let’s break down our code step-by-step:
- We import the
java.util.Arrays
package at the start of our program. This includes the toString() method we’ll use to print the array copy we create at the end of our program. - We declare an array called
coffees
which stores the coffees on our standard menu. - We initialize an array called
summer_coffees
which will hold 6 values. - We use arraycopy() to create a copy of our
coffees
array. Here are the parameters we specify:- coffees is the array we want to copy.
- 0 is the position at which we want our copy to start in the
coffees
array. - summer_coffees is the array in which we want our copied values to be added.
- 0 is the position at which we want the copied values to start being added in the
summer_coffees
array. - coffees.length is the number of array elements we want to copy. In this case, using
coffees.length
allows us to copy every element from thecoffees
list.
- We print out a message stating “Summer coffees: “, followed by the list of summer coffees we have created.
Conclusion
Copying an array is a common operation when you’re working with lists. This tutorial explored four ways in which you can copy an array in Java.
First, we discussed how to create a shallow copy using the assignment operator, then we proceeded to explain how to create a deep copy using a for
loop. Then we explored how to use the copyOfRange() method to create a copy of an array, and how the arraycopy() system method is used to copy an array.
Now you’re ready to start copying arrays in Java like a professional!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.