What do we mean when we talk about a class in Java? It’s certainly not a class like you’d have in school, with students and teachers. A class is a blueprint for an object in your code.
Java is an object-oriented programming language. This means that it includes a number of features that help you write reusable code. Object-oriented programming is useful for programs of all sizes because it helps reduce repetition, which in turn makes your code more readable.
In this guide, we’re going to talk about two important concepts in Java: the class and the object. We’ll discuss how they work, and how you can implement them, with reference to examples.
What is a Java Class?
A class is like a blueprint for an object. Whereas a blueprint for a bedroom may say what types of doors and windows are being installed, a class tells your program what methods and values an object should be able to access and store.
Classes are useful because they allow you to declare a structure for a particular type of data once which you can reference multiple times by declaring objects.
Let’s define a class called Employee which has one variable and two methods:
public class Employee { boolean isOnVacation; public void startVacation() { isOnVacation = true; System.out.println("You are now on vacation."); } public void endVacation() { isOnVacation = false; System.out.println("You are not on vacation anymore."); } }
In our code, we’ve defined two methods: one to start an employee’s vacation, and the other to end an employee’s vacation. We have declared a boolean instance variable called isOnVacation to track this information.
We haven’t yet created any objects of our employee class. We’ve only outlined the blueprint which will later define how those objects take shape.
What is a Java Object?
An object is an instance of a particular class. Let’s create an object using our Employee blueprint from earlier. We’ll create an object called john:
Employee john = new Employee();
This code creates an instance of the class Employee. Notice that we’ve added two parenthesis after the name of our class, which tells our code to reference the Employee class. The new
keyword tells our code to create a new object of that class.
Now, let’s use our object methods:
Employee john = new Employee(); john.startVacation(); john.endVacation();
Let’s run our program and see what happens:
You are now on vacation. You are not on vacation anymore.
Our object calls the two methods we defined in our Employee class. This causes the code within those methods to run. When we execute john.startVacation()
, for example, the value of the isOnVacation
boolean is set to true, and the message “You are now on vacation.” is printed to the Java console.
Although we’ve defined the variable isOnVacation
inside our class, we can access the value it has been assigned in a particular object. Consider this code:
Employee john = new Employee(); john.startVacation(); john.endVacation(); System.out.println(john.isOnVacation);
This code will start and end John’s vacation – like in our last example – and then it will print out the value of the isOnVacation
variable to the console:
You are now on vacation. You are not on vacation anymore. false
The value of our isOnVacation
method is false
.
Java Constructors
The constructor method allows you to initialize data in a class. In our example above, we declared a variable in the main body of our class but we didn’t initialize it with a default value.
Consider the following code:
class Employee { boolean isOnVacation; public Employee() { isOnVacation = false; System.out.println("Initialized values."); } ... }
Now, let’s create an object of our Employee class:
Employee john = new Employee(); john.startVacation();
When we create our john
object, our constructor will automatically run. This method allows you to set the default values that you want your class to hold. Our method returns:
Initialized values. You are now on vacation.
Upon execution, our constructor sets the value of isOnVacation
to false. It then prints out Initialized values
to our console.
Creating Multiple Objects
What if we have two employees? Can you still use the same class? Yes! That’s the benefit of using classes: you can declare many objects using the same class.
Consider the following main program:
Employee john = new Employee(); john.startVacation(); john.endVacation(); System.out.println(john.isOnVacation); Employee ian = new Employee(); ian.startVacation(); System.out.println(ian.isOnVacation);
Let’s run our code:
Initialized values. You are now on vacation. You are not on vacation anymore. false Initialized values. You are now on vacation. true
We’ve created two objects of our Employee class: john and ian. In our code, we have started and ended John’s vacation. Then, we created an object for Ian and started his vacation.
When we print out the value of john.isOnVacation
, false is returned; when we do the same for Ian’s object, true is returned. That’s because each object of our class is separate. They use the same blueprint, but they can store their own values.
Conclusion
Classes and objects are the cornerstone of object-oriented programming. Classes allow you to define blueprints, from which you can then define objects.
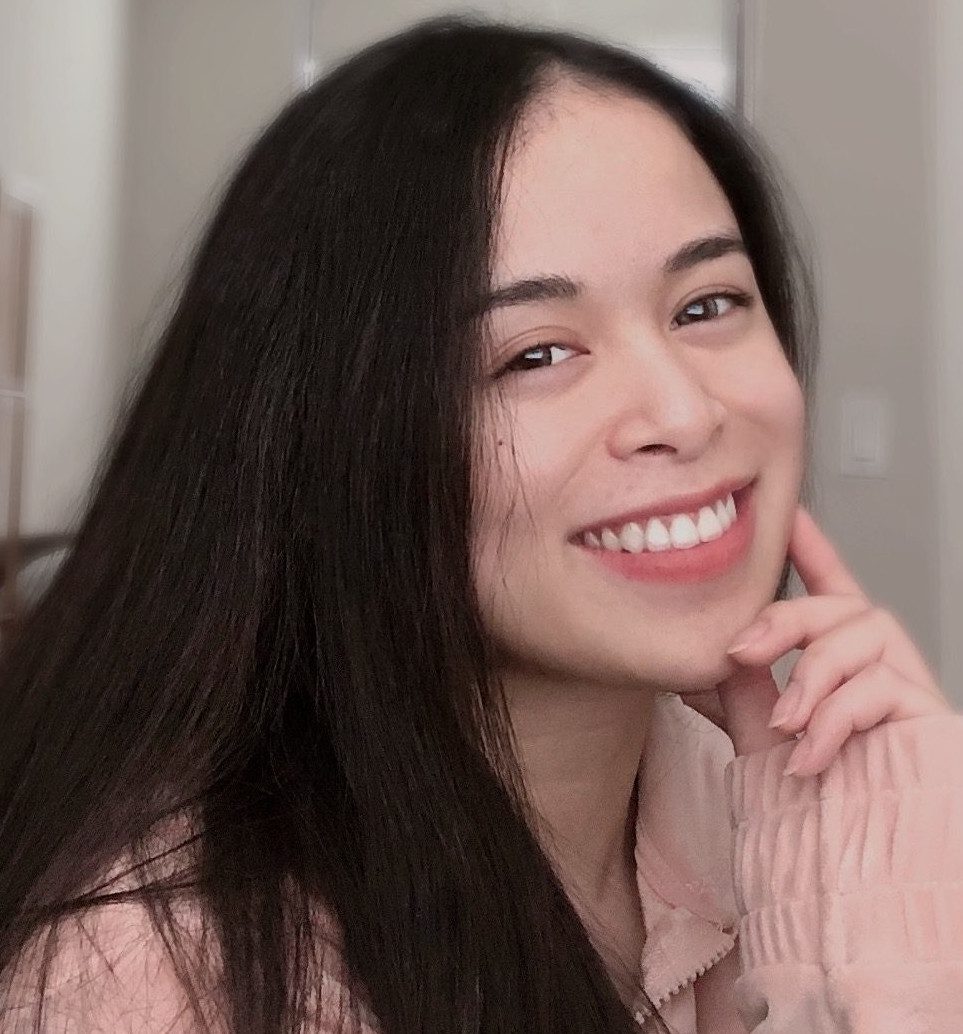
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Are you ready for a challenge? Create a program with a class that tracks information about whether a student has passed their test. It should:
- Store a variable to keep track of whether the student has passed their test.
- Have two methods that change whether the student has passed their test.
- Optionally, you could use an “if” statement to calculate whether a student’s test should be marked pass/fail based on their numerical grade.
Now you’re ready to start working with classes and objects in Java like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.