To convert a char to a string in Java, the toString()
and valueOf()
methods are used. The toString()
and valueOf()
methods are both used to convert any data type to a string. For converting a char to a string, they both function identically.
In programming, data types are used to distinguish particular types of data from each other. For instance, strings are used to store text-based data, and floating-point numbers are used to store decimal numbers.
The data type which a value holds has an impact on how you can retrieve and manipulate the value. So, you’ll often find that you want to convert data to another type in Java. One of the more common type conversion operations in Java, char to string, has more than one solution.
That’s where the toString() and valueOf() methods come in. This tutorial will discuss, with reference to examples, how to use the toString() and valueOf() methods to convert a char to a string in Java.
Java Data Types
Java contains several data types that are used to store data. For instance, char
is used to store a single character, and int
is used to store a whole number.
Each data type has its own operations, which can be used to manipulate data. Floating-points and integers, for example, can be manipulated with mathematical operations; strings can be changed using Java string methods.
For this tutorial, we are going to focus on two data types: char and string.
In Java, char
stores an individual character. char
is short for character
, which refers to the Java character class. Here’s an example of a char
in Java:
char theLetterF = ‘F’;
Strings are used to store sequences of one or more characters. Here’s an example of a string in Java:
String restaurantName = “The Two Cranes”;
Now, what if you want to convert a char into a string? Let’s discuss two approaches you can use.
Java Convert Char to String Using toString()
The Java toString() method is used to convert a value to a string. toString() accepts one parameter: the value you want to convert to a string.
To convert a char to a string, we can use the Character.toString() method. Here’s the syntax for the Character.toString() method:
Character.toString(value);
Suppose we are building a program for a tailor that stores the first letter of a customer’s first and last names. This data is stored, so the tailor knows what to monogram into a customer’s clothing.
Right now, these values are stored as chars. However, the tailor has decided that she wants to add new features into the program, and so she wants us to convert the char values to strings. We could use the following code to convert the monogram letters to a string:
class ConvertMonograms { public static void main(String[] args) { char first = 'T'; char second = 'F'; String first_string = Character.toString(first); String second_string = Character.toString(second); System.out.println("The first monogram letter is: " + first_string); System.out.println("The second monogram letter is: " + second_string); } }
Our code returns:
The first monogram letter is: T
The second monogram letter is: F
There’s a lot going on in our code, so let’s break it down. First, we declare a class called ConvertMonograms which stores the code for our program. Our class performs the following actions:
- The variables first and second are declared as chars and store the values T and F, respectively.
- The Character.toString() method is used to convert the contents of the first variable to a string, and assigns the new string to the variable
first_string
. - Character.toString() is used to convert the contents of
second
to a string, and assigns the new value to the variable second_string. - Our program prints out “The first monogram letter is: “ to the console, followed by the value stored in
first_string
” - “The second monogram letter is: “ is printed to the console, followed by the value stored in the variable
second_string
.
In simple terms, our program has converted the characters T
and F
to strings.
Java Convert Char to String Using valueOf()
The String.valueOf() method is used to convert a value to a string in Java. The string valueOf() method accepts one parameter: the value you want to convert to a string.
valueOf() works in the same way as the Character.toString() method we discussed earlier.
Let’s return to the tailors’ store. Suppose we want to use the valueOf() method instead of toString() to convert the monogram letters from a char to a string. We could use the following code to accomplish this goal:
class ConvertMonograms { public static void main(String[] args) { char first = 'T'; char second = 'F'; String first_string = String.valueOf(first); String second_string = String.valueOf(second); System.out.println("The first monogram letter is: " + first_string); System.out.println("The second monogram letter is: " + second_string); } }
Our code returns:
The first monogram letter is: T
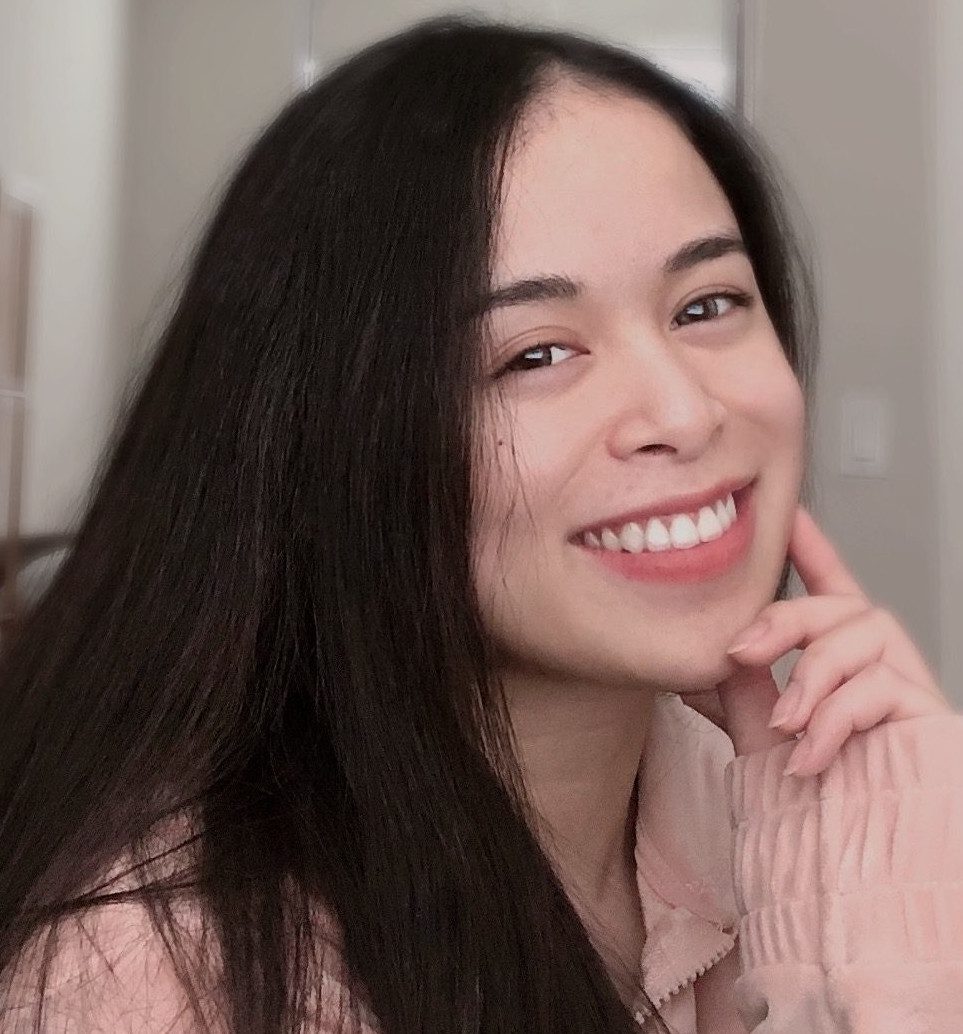
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
The second monogram letter is: F
Our code works in the same way as our first example. The only difference is that we use String.valueOf() instead of Character.toString() to convert our char values to a string.
Convert Char Array to String
Additionally, the valueOf() method can be used to convert a char array to a string.
Suppose we stored the initials of a customer, Tom Montgomery Peterson
, in a char array, and we want to convert it to a single string, so we know what to monogram on the customer’s clothes. We could use this code to convert the char array to a string:
class ConvertMonogramsToArray { public static void main(String[] args) { char[] initials = {'T', 'M', 'P'); String monogram = String.valueOf(initials); System.out.println("Monogram: " + monogram); } }
Our code returns:
Monogram: TMP
Let’s break down our code. First, we declare a class called ConvertMonogramsToArray. This class stores our code for the example. Our class executes the following functions:
- A variable called
initials
is declared which stores a list of chars. - String.valueOf() is used to convert the contents of
initials
to a string and assigns the new string to the variablemonogram
. - The string “Monogram: “ is printed to the console, followed by the monogram of the customer.
Conclusion
Converting a char to a string in Java is a common task. The toString() and valueOf() methods can both be used to convert a char to a string in Java.
This guide discussed how to use toString() to convert a char to a string, how to use valueOf() to convert a char to a string, and how to use valueOf() to convert a char array to a string. This guide also walked through an example of each of these methods in a Java program.
Now you’re ready to start converting chars to strings in Java like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.