How to Use Booleans in Java
True or false. We’re not talking about a question on a standardized test. True and false are the values that can be stored inside the Boolean data type.
In programming, Booleans are used to control the flow of a program. They are also used to make comparisons between values.
In this guide, we’re going to talk about the basics of how Booleans work. We’ll discuss how to make comparisons with Booleans, and how to use logical operators. Let’s get started!
What is a Java Boolean?
A boolean is a data type that can store one of two values: true and false.
Booleans are a key part of logical operations in mathematics. They’re useful when the answer to a problem can only be one of two values. A boolean value could represent on or off, true or false, yes or no, or any other situation where only two possible outcomes are valid.
The Boolean data type is capitalized when we talk about it. This is because it was named after the mathematician George Boole, who played a key role in the fields of logic and algebra. However, when you are declaring a boolean, you should use lowercase.
In Java, booleans are declared using the boolean keyword. Let’s declare a boolean which tracks whether a coffee house has its premium beans in stock:
boolean premiumBeans = true; System.out.println(premiumBeans);
Our code returns: true. We’ve stored the value “true” inside the variable “premiumBeans”. Notice that we have declared our boolean using the boolean
keyword, which appears in lowercase.
Boolean objects represent false or true values.
Making Comparisons Using Booleans
The Boolean data type is particularly useful when making comparisons.
This is because when you are comparing two values, a comparison can only be true or false. A string can only be equal to or not equal to another number; a number can only be less than or greater than another number.
Let’s demonstrate how this works with an example. We’re going to write a program which checks whether a child at a theme park is old enough to go on a ride. We’ll start by declaring two variables:
int minimumAge = 12; int customerAge = 15;
The variable “minimumAge” tells us the minimum age you have to be to go on a ride. The variable “customerAge” is the age of the child who wants to go on the ride.
To check whether the customer is old enough to go on the ride, we can use a comparison operator:
boolean isOldEnough = customerAge >= minimumAge; System.out.println("Is the customer old enough to ride? ", String.valueOf(isOldEnough));
Our code returns:
Is the customer old enough to ride? true
We have declared a new variable called “isOldEnough”. This variable represents the specified boolean which is calculated using our comparison statement. We check whether the customer’s age is greater than or equal to the minimum age. If it is, isOldEnough will be equal to true; otherwise, it will be false.
15 (customerAge) is greater than 12 (minimumAge). This means that our expression evaluates to true, and so our boolean object represents the value true.
We can use any Java comparison operator to perform a comparison:
- ==: Equal to
- !=: Not equal to
- >: Greater than
- <: Less than
- >=: Greater than or equal to
- <=: Less than or equal to
Consider the following code:
int minimumAge = 12; int customerAge = 15; boolean isMinimumAge = customerAge == minimumAge; System.out.println("Is the customer's age equal to the minimum age? ", String.valueOf(isMinimumAge));
In this code, we have compared whether or not the customer’s age is equal to the minimum age. These numbers are not equal, so our Boolean expression evaluates to false:
Is the customer's age equal to the minimum age? False
When you are comparing to see whether two values are equal, you must use two equals signs (==). One equals sign is used to assign a value to a variable in Java; two equals signs denote that you want to make a comparison.
Using Boolean Logical Operators
Booleans can be used with Java’s logical operators to determine whether multiple expressions are met. These operators will return a boolean value: true or false.
There are three logical operators:
- && (and): Returns “true” if both values are true
- ! (not): Returns “true” if a value is false
- || (or): Returns “true” if at least one value is true
Let’s use an example to show these operators in action. For example, suppose that we’re building a program that evaluates whether a customer should receive a discount on a purchase.
A discount should be given if a user’s purchase is over $40 and the user is a loyalty card member. We could check for these conditions using this statement:
int purchasePrice = 50; boolean isLoyaltyCustomer = true; System.out.println((purchasePrice > 40) && (isLoyaltyCustomer == true));
Our code returns: true. Our code evaluates three expressions:
- Is purchasePrice greater than 40?
- Is isLoyaltyCustomer equal to true?
- Is purchasePrice greater than 40 and is isLoyaltyCustomer equal to true?
If all of these conditions are met – which they are in this case – true is returned. Otherwise, false is returned.
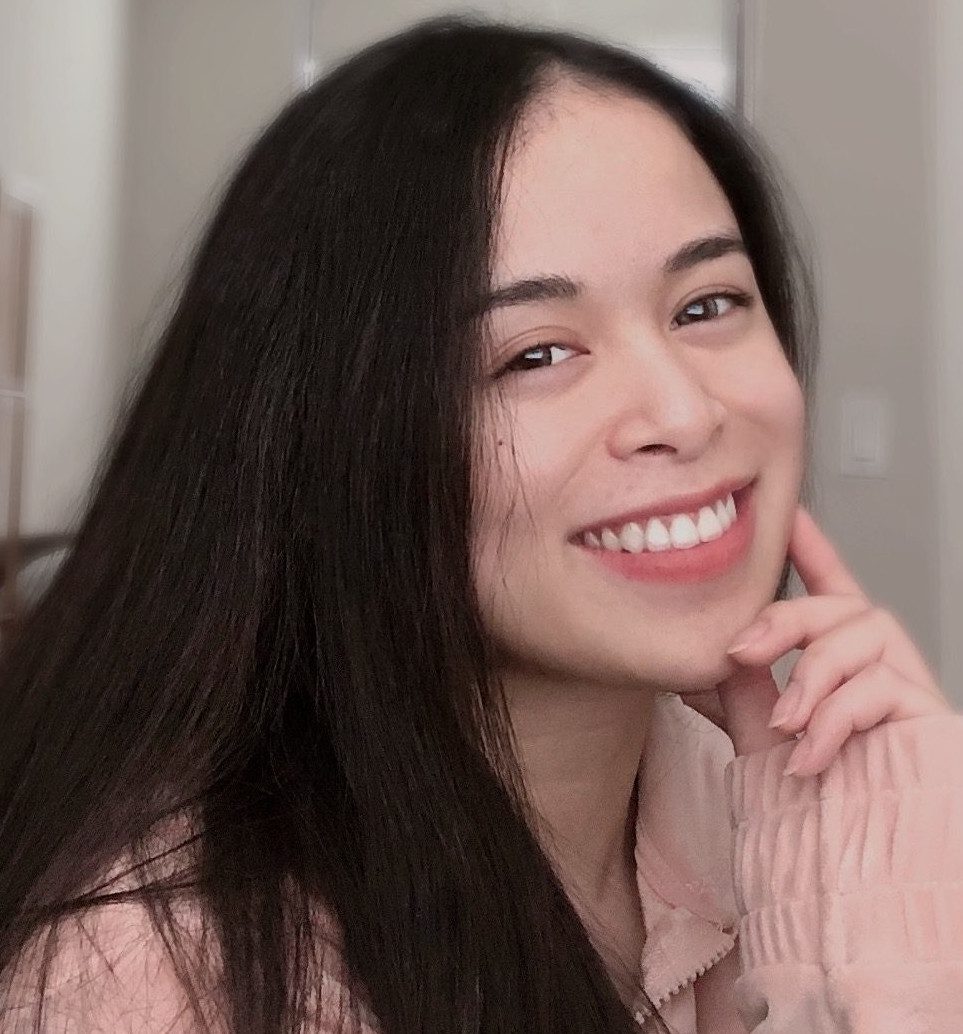
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Let’s say that we want to give our discount to people who make a purchase greater than $40 or who are loyalty card members. We could do this by changing our logical operator to “||” (the “or” operator) instead of “&&” (the “and” operator):
int purchasePrice = 30; boolean isLoyaltyCustomer = true; System.out.println((purchasePrice > 40) || (isLoyaltyCustomer == true));
Our code returns: true. Our program checks:
- Is purchasePrice greater than 40?
- Is isLoyaltyCustomer equal to true?
- Is purchasePrice greater than 40 or is isLoyaltyCustomer equal to true?
Only one of the two conditions we have specified needs to be met for true to be returned. In this case, isLoyaltyCustomer is equal to true, so our code returns true.
We can use the not operator to check whether a value is false. Suppose we only want to give the discount to people who are not loyal customers because they already receive a spare discount. We could enforce this using the following code:
boolean isLoyaltyCustomer = true; System.out.println(!isLoyaltyCustomer);
In this code, we check if “isLoyaltyCustomer” is not true. “isLoyaltyCustomer” is equal to true, so our code returns false.
Using Booleans with Conditionals
Booleans are often used with conditional statements such as an if
statement.
A conditional will evaluate a statement down to a true or false value. If a condition is true, the code within a conditional block will run; otherwise, that code will not run.
Let’s say that we want to print a message to the console if a customer is eligible for a discount. A customer is eligible for a discount only if they are a loyalty customer:
boolean isLoyaltyCustomer = true; if (isLoyaltyCustomer == true) { System.out.println("This customer is eligible for a discount."); } else { System.out.println("This customer is not eligible for a discount."); }
Our code returns a string object:
This customer is eligible for a discount.
Our code checks to see whether the value of “isLoyaltyCustomer” is equal to true. It is, and so the code within our if
statement is run.
If our customer was not a loyalty card holder, this statement would evaluate to false. In this case, the contents of our else
statement would be run.
Conclusion
Booleans allow you to store true or false values in your code. When used with comparison operators, you can use a boolean to evaluate a statement.
You can use logical operators with a boolean to determine if multiple conditions are met, if one of multiple conditions are met, or if a condition is not met. Booleans are often used with conditional statements such as an if
statement to evaluate an expression.
Now you’re ready to work with booleans in Java like a professional!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.