Java is a popular programming language used to build applications. Developers prefer it because it offers flexibility and allows them to write code that can work on any machine, regardless of the platform or architecture. It was built to be easy to debug, write, and compile. It also assists in learning other programming languages.
As a beginner, there is a lot you need to understand about Java to make your work easier. To help you, we’ve compiled this list of Java best practices. It includes guidelines and resources that will allow you to thrive in your for your web or app development career.
What Is Java?
Java is a class-based, general-purpose, object oriented language. It is built for lesser implementation dependencies. It is popular due to the fact that it is secure, fast, and reliable. This is why developers use it for building data centers and apps for laptops, game consoles, cellphones, and scientific supercomputers.
Java has several applications because of its flexibility and versatility. This is why it is used to create enterprise software, develop Android apps, and mobile Java apps. Java is also used for big data analytics, hardware device programs, and server-side technologies such as JBoss, Apache, and Glassfish.
5 Concepts You Need to Understand for Java Best Practices
To fully understand Java best practices, you need to master some basic concepts. Some of the most common ones are linked to object oriented programming and they include encapsulation, abstraction, polymorphism, inheritance, and composition.
- Encapsulation. This is a practice of grouping data and code that acts on the data into single units, for the purpose of restricting access to the data variables. Through encapsulation, developers can reuse objects such as variables or code components without requiring full access to all the data.
- Abstraction. Abstraction is used to selectively show some parts of an object and hide others. This way, only relevant characteristics are displayed to the users.
- Polymorphism. Polymorphism lets programmers use one word with different meanings and contexts. In Java, it is simply the ability of objects to take different forms. This concept allows developers to perform one action in different ways. It is a feature of object oriented programming.
- Inheritance. This concept allows developers to create child classes that inherit methods and fields of the parent class. Parent classes are known as base classes or superclasses and child classes are known as derived classes.
- Composition. This concept is related to aggregation and happens when two classes linked together are mutually dependent on one another. One cannot exist without the other and a good example of this is cars and engines. Cars cannot work without engines and engines cannot work without being added to a car. This is known as a PART-OF relationship between objects.
5 Common Challenges That Java Guidelines Can Address
Following Java guidelines and best practices can help you save time as you develop creative solutions to common problems we face daily. This includes programming remote devices, creating cloud computing solutions, or improving customer support when hiring dedicated customer support representatives is not an option for an organization.
Program Remote Devices
Java made it easier to power remote devices by using complementary technologies, such as artificial intelligence. Today it is possible to use mobile apps to power appliances in your home, remotely open and close garage doors, and even turn off slow cookers from a thousand miles away. It is called the Internet of Things and it has many implications for different industries.
Create Cloud Computing Solutions
In the past, file storage involved storing actual files in cabinets and needing space for it. Today, cloud computing has made file sharing and storage easier. Apps like Amazon Web Services, Dropbox, Salesforce, and Slack are common cloud computing services. Following Java guidelines is helpful in developing these types of cloud apps, as it ensures you are making the best use of Java’s main feature.
Improve Customer Support
Not every company has the budget for a large customer representative team to respond to questions daily. This is where chatbots come in. Using Java guidelines to streamline your process, you can build these popular bots which are powered by artificial intelligence to answer questions and resolve issues. These bots have become popular in many industries.
Generate Enterprise Applications
Java is also preferred for creating enterprise applications. This is because it has several application programming interfaces for creating enterprise apps, called Java EE. These APIs facilitate smooth scripting and efficient coding. Also, lots of libraries support Java as it also supports several other platforms.
Develop 2D and 3D Games for Android
Java is excellent for game development and it has been used to create popular games like Minecraft. Developers prefer it, especially for Android because of its high performance. The programming language offers tools like jMonkeyEngine for 3D additions to games. This open-source tool is also quite handy for 2D games.
Top 10 Java Best Practices and Guidelines
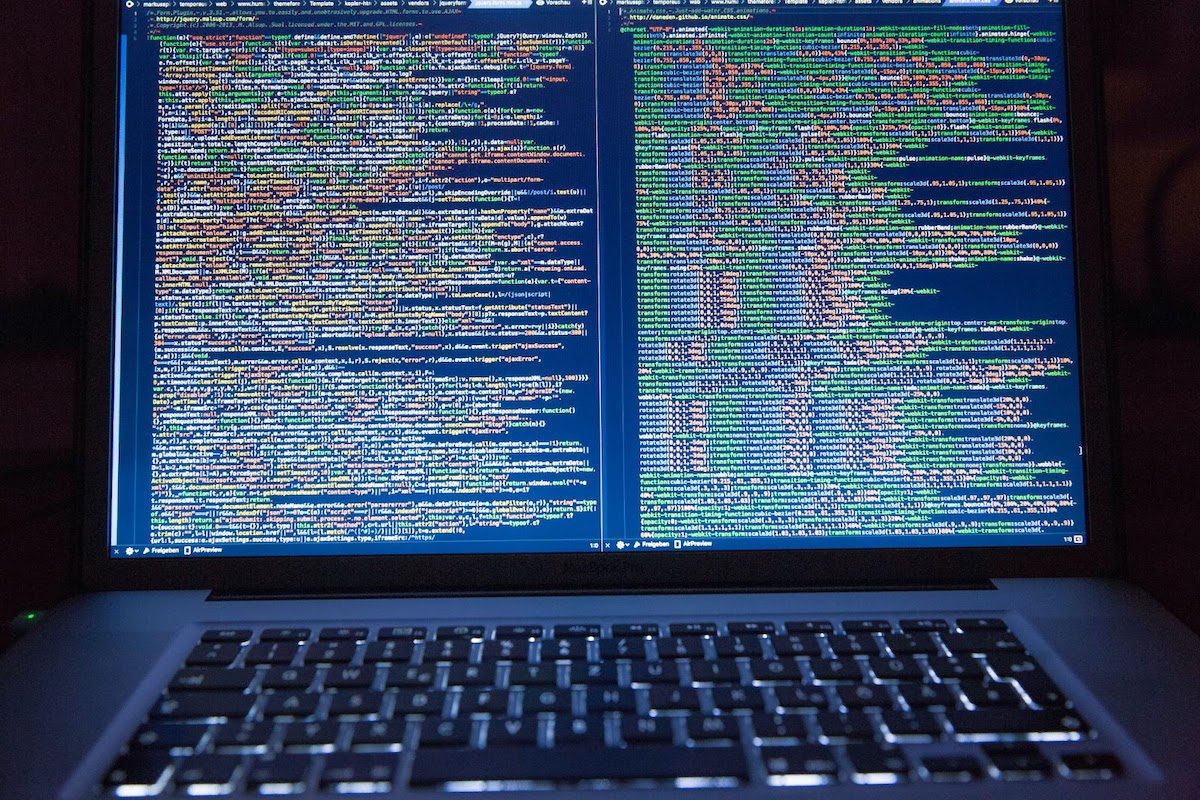
Java is a good programming language for beginners and you can quickly move up the ranks as a developer if you adhere to Java best practices and guidelines. Below are some of the top practices and guidelines to keep in mind as a Java developer.
Avoid Memory Leaks
A memory leak occurs when unused objects take up space in memory. The Java garbage collector is usually responsible for removing the unused objects, but in some cases, they may not be eligible for removal. This happens when objects are being referenced. Developers can’t control memory management in Java because the programming language manages it automatically.
However, avoiding memory leaks helps remedy this situation. Allowing memory leaks can lead to performance degradation. It helps to release database connections when you are done querying. Also, you can use the “finally” block to prevent memory leakage.
Use Strings Properly
Strings are objects backed by a char array internally. Strings are immutable just like arrays, meaning that they can’t grow. When changes are made to a string, a new string is created. String handling is a simple process in Java and needs to be properly used to prevent memory waste.
Where two strings concatenate in a loop, it leads to memory waste because it creates a new string object each time. This can also affect performance time. It’s best to avoid constructors for instantiation. Rather, instantiation should be direct to save time instead of using a constructor.
Proper Commenting
Documentation comments are useful for so many reasons, including the fact that they improve the quality and readability of your code. They are remarks that set code details and make them more intelligible. Using remarks also makes bug discovery easier, and support simpler.
When lines of code are read by others with different skill levels in Java, proper commenting gives them an overview of the code. It also offers more information that clarifies issues within the code. Also, comments describe the code workings and are read by the tester, quality assurance engineer, code reviewer, or maintenance developer who may otherwise not understand your work.
Double and Float
New developers often have problems differentiating between float and double. It seems simple but can become confusing when it is time to put them into use. The best way to differentiate between the two is to focus on the way each one is used.
Most processors take the same time to process operations on double and float. However, the former offers more precision than the latter. This is why it is important to use double where precision is a priority. Float can be used in other circumstances where it is not because it does not require as much space as double.
Don’t Leave Catch Blocks Empty
In Java, catch blocks are used for handling exceptions by declaring the kind of exception it is. Experienced developers prefer to write meaningful messages in the catch block instead of leaving it as is while exception handling.
As a beginner, you may make the mistake of leaving it empty because it is likely that an exception will be caught by a catch block. If this happens, it may not show anything, but its effects may make debugging harder. Also, it can take a lot of time to correct the error.
Use Proper Naming Conventions
Before you write your code, it is advisable to use a proper naming convention for the project. Take some time to decide the names for all interfaces, classes, variables, and methods. If it is a group effort, then ensure that everyone maintains uniformity when it comes to naming. This seems like a simple issue, but it is as important as everything else.
Some developers may decide to add random names just to give the compiler what it wants. This is not ideal. It is better to use names that are both meaningful and self-explanatory. It should not be difficult for qualified assurance engineers, other developers, and your teammates to understand what they mean.
Unreasonable Garbage Allocation
Excessive garbage allocation happens when programs generate short-term objects. This is quite common in Java development. When it happens, the garbage collector works more to remove unnecessary objects that clog the memory. It is important to check because it can negatively affect the app’s performance.
For instance, if a program creates a string after each iteration, new strings will be created because strings are immutable. To fix this problem, it is advisable to use the StringBuilder instead of the garbage collector.
Overlooking Existing Libraries
Many beginner programmers make the mistake of ignoring existing libraries that can help them create code for their apps much quicker. In many cases, this may be because they simply lack knowledge of the varied Java libraries that exist online. Make sure to always check for existing libraries before writing your source code, as that can help save a lot of time.
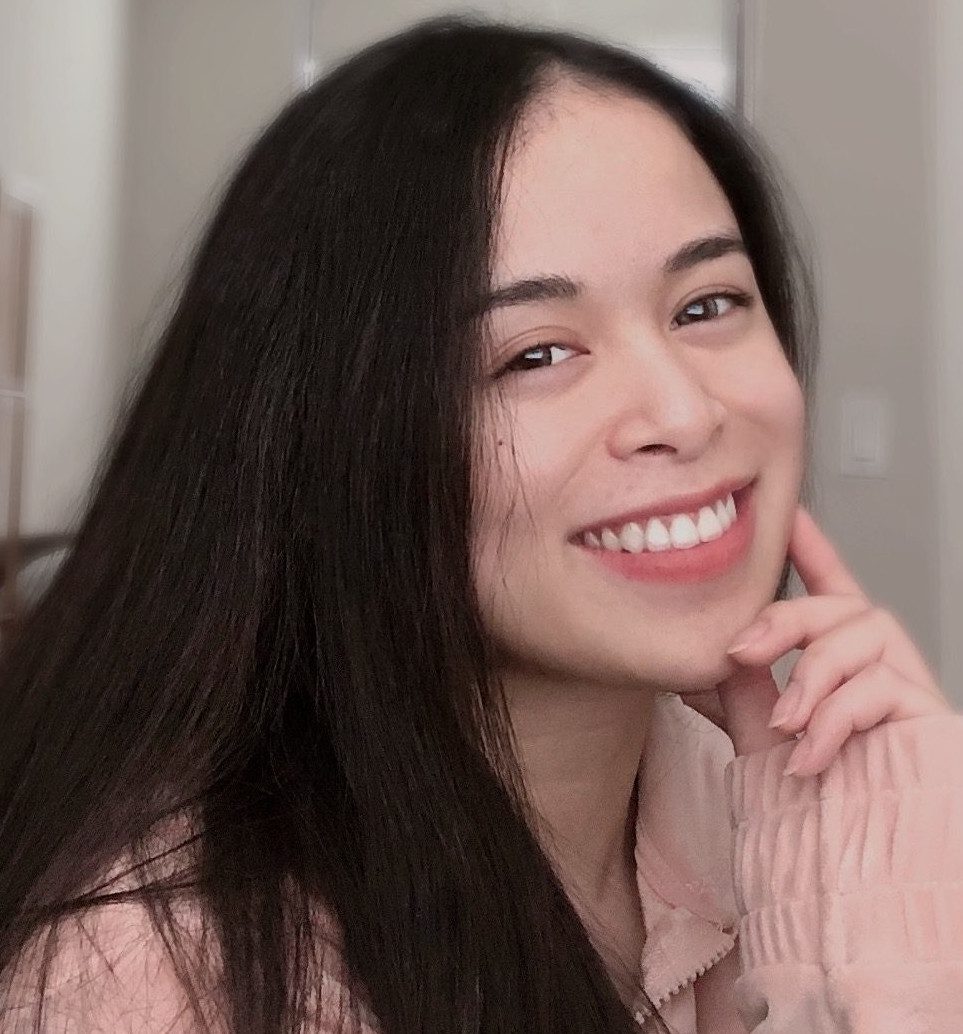
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Unnecessary Use of StringBuffer
Using StringBuffer can create unneeded objects and sometimes developers make the mistake of not weighing the pros and cons of doing so. In many cases, it is not recommended to use StringBuffer, as StringBuilder is a better option. The reason for this is that StringBuffer has a synchronized nature and this creates overhead. If synchronization is not the main priority while coding, then it is advisable to use the StringBuilder.
Avoid Object Mutability
Mutable objects can be altered during their lifecycle. The variable values and object states are alterable. Since variables point to one instance, tracking the changes in the object can be difficult. It’s best is to avoid mutable objects as much as possible.
Immutable objects remain in the same state and don’t change as time passes. They do not have side effects and are thread-safe. These characteristics make immutable objects more useful in various multithreaded environments.
How to Learn Java Best Practices
To learn Java best practices, you should begin by browsing the plethora of learning resources online. Depending on your learning preferences, you can consider enrolling in an online course, watching video tutorials, or reading articles online. If you prefer a more structured learning environment, then a coding bootcamp may be a better option for you.
Can a Bootcamp Help You Learn Java Best Practices?
Yes, a bootcamp can teach you Java best practices. Bootcamps offer intensive Java training, sometimes as part of a larger program like software engineering. Coding bootcamps are excellent because they offer flexibility, one-on-one support, and career support to help you land your first job.
Bootcamp programs are short but intensive and cover industry-relevant topics within a short timeframe. They offer hands-on learning to give students a chance to practice what they learned in the program. This is also an opportunity for students to build a portfolio to make them more competitive candidates in the job market.
Best Courses and Training Programs to Learn Java Best Practices
Provider | Course | Price |
---|---|---|
Codefellows | Advanced Software Development in Java with SpringMVC & Android | $12,000 |
The Software Guild | Java Bootcamp | $9,000 |
The Tech Academy | Java and Android Development Bootcamp | $7,950 |
UC San Diego Extension | Java Programming | $2,875 |
Zip Code Wilmington | Java Bootcamp | $12,000 |
Should You Learn Java Best Practices?
You should learn Java best practices if you want to pursue a career in web or app development. Java best practices don’t only save you time. They help you prevent errors that can slow down the performance of your app or website. They also help you build an app that is readable and understandable by other developers and testers.
Java Best Practices and Guidelines FAQ
There are a lot of good Java practices. Some of the main ones include avoiding memory leaks, using double and float properly, avoiding using catch blocks, using string properly, utilizing libraries, avoiding object mutability and unreasonable garbage allocation, and using proper commenting name conventions.
Yes, you can learn Java best practices in a bootcamp. This type of training often covers all aspects of the programming language, as well as how to use it. Since the subject is technical, students in coding bootcamps learn by practicing. This helps them to gain experience in Java programming before getting any real-world experience.
Java is used for app and web development. It is one of the most popular programming languages and works as a server-side language for backend development projects. Developers prefer to use it for Android development because of its unique features. It is mostly used for mobile computing, desktop computing, numerical computing, and games.
The concepts you should learn before understanding Java best practices include encapsulation, abstraction, polymorphism, inheritance, and composition. All of these concepts are linked to object oriented programming. This knowledge can help you write clean code.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.