When you’re working with arrays in Java, you may encounter a situation where you want to check whether an array contains a particular value.
For instance, if you are building a program that tracks your bird-watching progress this season, you may want to build a function that allows you to check whether you’ve already seen a particular species of bird.
There are a few approaches that you can use to check whether an array contains a particular value. This tutorial will discuss, with examples, how you can check—using a for-each loop, contains()
, and stream()
—if an array in your Java code contains a certain value.
After reading this tutorial, you’ll be an expert at checking whether an array in a Java program contains a particular value.
Arrays in Java
In Java, arrays are containers that hold data of a single type. For example, an array could hold a list of names of 14 bird species you saw in your backyard bird watching this year. Such an array would contain 14 string values.
Arrays are an important data type because they allow developers to store a large number of values in one variable. This is more efficient than storing each of those values as separate variables, and it helps preserve the readability of a program.
Here’s an example of an array in Java:
String[] bagelShops; bagelShops = new String[4];
In this example, we declared an array—called bagelShops—that stores string values. Then we defined that the bagelShops variable should be capable of storing four strings. Here’s the code we would use to assign four bagel stores to this array:
String[] bagelShops = {"Absolute Bagels", "Bo's Bagels", "Brooklyn Bagel and Coffee Company", "Forest Hills Bagel"};
But what can we do to check whether an array contains a particular value? For example, what if we wanted to check if Forest Hills Bagel shop was on our list of favorite bagel stores? Let’s delve into a few approaches we can use.
Using a for-each loop
for-each loops, also known as enhanced for
loops, are a form of for loop
that you can use to loop through all items in an array or collection. For instance, you can use a for-each loop to iterate through a list of coins in an array that stores all the coins in a coin collection.
To check whether an array contains a particular value, we can use a for loop. This for loop will iterate through every item in an array and check whether each item is equal to the item for which we are searching.
Suppose we have a list of our favorite bagel shops in New York City and want to check whether Absolute Bagels is on the list. We can do so using this simple loop:
class Main { public static void main(String[] args) { String[] bagelShops = {"Absolute Bagels", "Bo's Bagels", "Brooklyn Bagel and Coffee Company", "Forest Hills Bagel"}; String shopToFind = "Absolute Bagels"; boolean found = false; for (String shop : bagelShops) { if (shop == shopToFind) { found = true; break; } } if (found) { System.out.println("Absolute Bagels is on your list of favorite bagel stores!"); } else { System.out.println("Absolute Bagels is not on your list of favorite bagel stores."); } }
Our code returns:
Absolute Bagels is on your list of favorite bagel stores.
Let’s break down our code. First we declare an array called bagelShops that stores our four favorite bagel shops in NYC.
Then we declare a variable called shopToFind and assign it the value Absolute Bagels
. This is the value for which we want to search in our bagelShops array. Next, we declare a variable called found, which will be used to track whether Absolute Bagels
is found in our list.
On the next line, we initialize a for-each loop that loops through every item in the bagelShops list. We then use an if
statement to check whether each individual shop in our bagelShops list is equal to the value stored in the shopToFind variable.
If the statement evaluates to true, found is set to true, and the for-each loop stops executing because the program runs a break
statement. If the statement evaluates to false, the code in the if statement does not run.
At the end of our program, if found is equal to true, our code will print Absolute Bagels is on your list of favorite bagel stores!
to the console; otherwise, our code will print to the console: Absolute Bagels is not on your list of favorite bagel stores.
We could also use this approach on a list of numbers. So, if we have a list of numbers and want to see whether the list contains a particular number we could use code similar to what we used in our above example. Of course, we would use numbers instead of string values in this case.
Using the contains() method
You can also check whether an array contains a particular value using the Java contains()
method. contains()
accepts one parameter: the value for which you want to search in an array.
The contains()
method can only be used to check whether an array contains a string value. If you want to search for a number in an array, you may want to use the for-each loop technique instead.
Suppose we have a list of our favorite bands and want to see if the Beatles is in that list. We could use the following code to do so:
class Main { public static void main(String[] args) { String[] favoriteBands = {"Beatles", "Revivalists", "Jonas Brothers"}; String favoriteToFind = "Beatles"; boolean found = favoriteBands.contains(favoriteToFind); if (found) { System.out.println("Beatles is on your list of favorite bands!"); } else { System.out.println("Beatles is not on your list of favorite bands."); } } }
Our code returns:
Beatles is on your list of favorite bands!
Let’s break down our code. First we declare an array called favoriteBands which stores a list of the names of our favorite bands. Then we declare a variable called favoriteToFind which stores the name of the band for which we want to search.
On the next line, we use contains()
to check whether the favoriteBands array contains the value stored in favoriteToFind (which is Beatles
in this example). We assign the outcome of the contains()
statement to a boolean called found.
If found is equal to true, our code prints Beatles is on your list of favorite bands!
to the console; otherwise, our code prints Beatles is not on your list of favorite bands.
to the console.
Using the stream() method
The Java Arrays package contains a number of functions used to work with arrays. One of these functions is stream()
, which you can use to check if an array contains a particular value. You must use the anyMatch()
method with the stream()
function to do so.
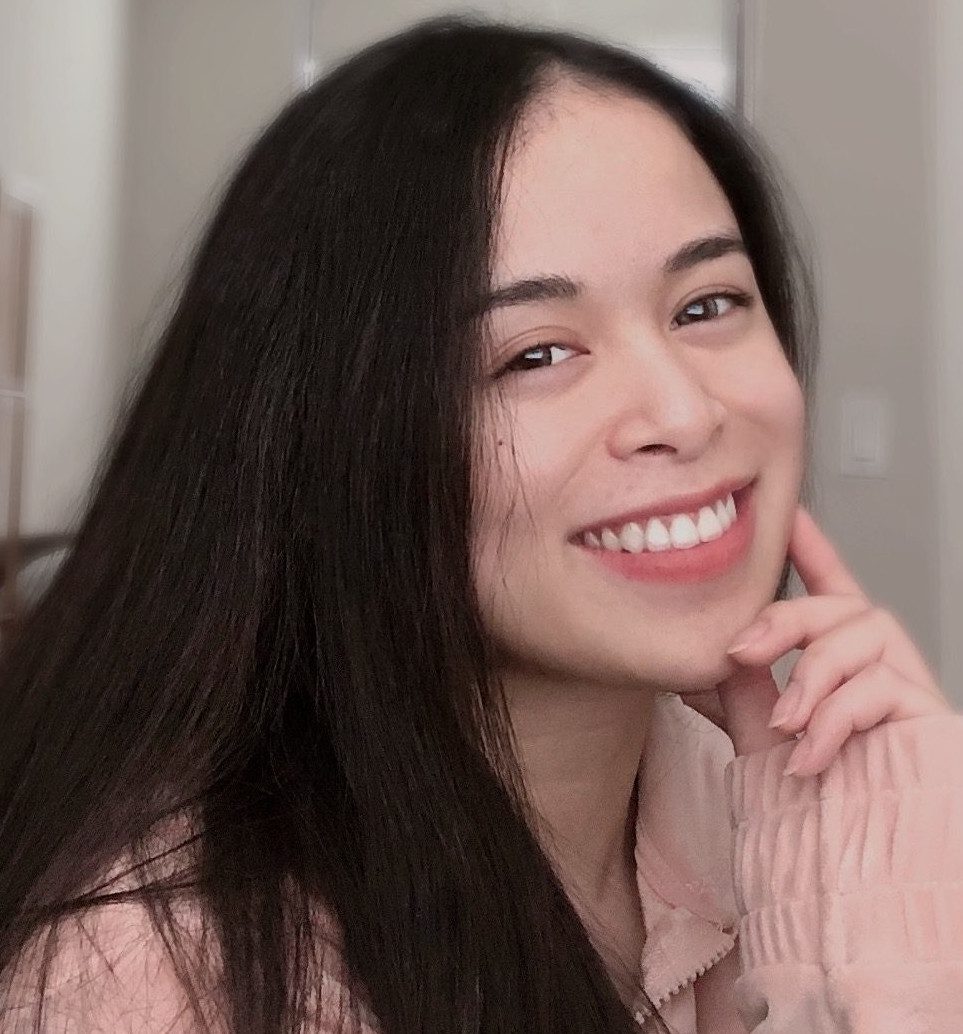
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Suppose we have a list of our friend Ada’s favorite TV shows, and we want to find out whether Parks and Recreation is on her list. We can do so using this code:
import java.util.Arrays; class Main { public static void main(String[] args) { String[] favoriteShows = {"Billions", "Silicon Valley", "Superstore"}; String showToFind = "Parks and Recreation"; boolean found = Arrays.stream(favoriteShows).anyMatch(show -> show.equals(showToFind)); if (found) { System.out.println("Parks and Recreation is on Ada's list of favorite TV shows."); } else { System.out.println("Parks and Recreation is not on Ada's list of favorite TV shows."); } } }
Our code returns:
Parks and Recreation is not on Ada's list of favorite TV shows.
Let’s break down our code. First, we import the Java Arrays package. To do so, we use the code: import java.util.Arrays;
.
Next, we declare an array called favoriteShows. This array stores a list of Ada’s favorite TV shows. Then we declare a variable called showToFind that stores the name of the show we want to find. In this case, we assign the value Parks and Recreation
to showToFind.
Then, we use the Arrays.stream()
and anyMatch()
methods to check whether the value stored in showToFind exists in the favoriteShows array. These methods return a true or false value that is assigned to the found boolean variable.
If the found variable evaluates to true, our program prints Parks and Recreation is on Ada’s list of favorite TV shows.
to the console. Otherwise, our program prints Parks and Recreation is not on Ada’s list of favorite TV shows.
to the console.
Conclusion
Checking whether an array contains a particular value is a common operation in Java. The three main approaches for checking whether an array contains a certain value are:
- for-each loop
- contains()
- stream()
This tutorial discussed, using examples, how to check whether an array in Java contains a particular value. Now you have the skills you need to check if a Java array contains a certain value like a professional developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.