What if there was a way that you could declare one variable that stores multiple values? There is? That’s great. In Java, there’s a concept called an array that allows you to do exactly this. You can store multiple values in one variable, which helps keep your code clean and tidy.
In this guide, we’re going to discuss how arrays work, why they are used, and how you can manipulate the contents of an array. We’ll walk through a few examples to show how they work.
What Is an Array?
An array, also known as a list, is a data structure that contains an ordered sequence of elements. You can store zero or more items in an array, but they all have to be the same type. For instance, an array could store one-hundred strings or fifty-two numbers.
Arrays are useful because they help you group similar values together. Let’s say you are defining an array of coffee types. Rather than declaring each type of coffee as its own variable, you could group them together in an array.
Once you’ve declared an array, you can perform the same methods and operations on every value in the array by iterating through it.
One way to think about arrays is as a collection of values. You know that to-do list that you need to get through today? That’s similar to an array. There’s a list of tasks that you need to get through, and each one is stored in a particular order.
Declaring an Array
Declaring an array in Java is simple. All you’ve got to do is choose a name for your array and specify its data type. Here’s an example of declaring an array called coffees
:
String[] coffees;
Our code creates an array called coffees
. On the left, you have the type of data which will be stored in your array. You also have a pair of empty brackets. This tells you code that you want to define an array, rather than just a string variable. On the right, you’ve got the name of the variable.
We’re not quite done yet. When you’re declaring an array, you’ve got to tell your program how many items you want your array to hold. This allows Java to allocate the memory necessary to store values in your array.
We want our coffees
array to store our three favorite blends at our local shop. To do this, we’ll tell our program that the number of elements our array should be able to hold is three:
coffees = new String[3];
This will create three empty values which can be filled in our array.
Make sure that you think about how many values you want to store in your array. It’s important to note that once you’ve defined the size of an array variable, it cannot be changed.
We can compress our code down into one line where we both declare an array and allocate memory:
String[] coffees = new String[4];
This is the best way to declare an array because it’s concise and simple. The only occasion where this method doesn’t work is when you’re not sure how many values you are going to store in your array. If this is the case, you’d probably want to declare an array first, then allocate memory once your program knows how many values will store.
In this case, our array will hold string values. However, you could also declare an array of integers, an array of objects, an array of arrays, or an array holding another data type.
Initializing an Array
We’re not quite done yet with setting up our array. So far, we’ve declared a standard array. The trouble is that it doesn’t hold any values yet. The syntax for assigning a set of initial values to an array while declaring a variable is:
String[] coffees = { "Rwanda Izuba", "French Roast", "Vietnam Da La Arabica" };
In this example, we’ve assigned three values to our array.
Accessing the Elements in an Array
The beauty of arrays is that they’re easy to access. Metaphorically, it’s just like circling an item on your to-do list that you want to focus on.
Inside an array, every item is given its own special number. These are called index numbers. Index numbers start at the number 0, and they increase by 1 for each item in your array. Consider the following list:
- 0: Rwanda Izuba
- 1: French Roast
- 2: Vietnam Da Lat Arabica
In this list, the Rwanda Izuba blend would have an index value of 0, the French Roast blend would have an index value 1, and the Vietnam Da Lat Arabica blend would have an index value of 2. Let’s take the following array:
String[] coffees = { "Rwanda Izuba", "French Roast", "Vietnam Da La Arabica" };
If you want to access the first item in this array, you could use this code:
System.out.println(coffees[0]);
Our code returns: Rwanda Izuba.
The index value is passed into your array between square brackets. The range of numbers that exist as index values is between 0 and one less than the length of your array. This is because, as we mentioned earlier, arrays are indexed from the value 0.
If you try to access an index value that doesn’t exist, an error is returned:
ArrayIndexOutOfBoundsException: Index 3 out of bounds for length 3
In this case, we tried to access index value 3 in our array. Because our index values only go up to two—remember, arrays are indexed starting from 0—an error was returned.
Let’s take it up a notch. Suppose that you want to go through every item in your array and perform an operation on them individually. We’re going to print every item in our array to the console. You can accomplish this using a for-each loop, like this:
class CoffeeArray { public static void main(String[] args) { String[] coffees = { "Rwanda Izuba", "French Roast", "Vietnam Da La Arabica" }; for (String coffee : coffees) { System.out.println(coffee); } } }
Our code returns:
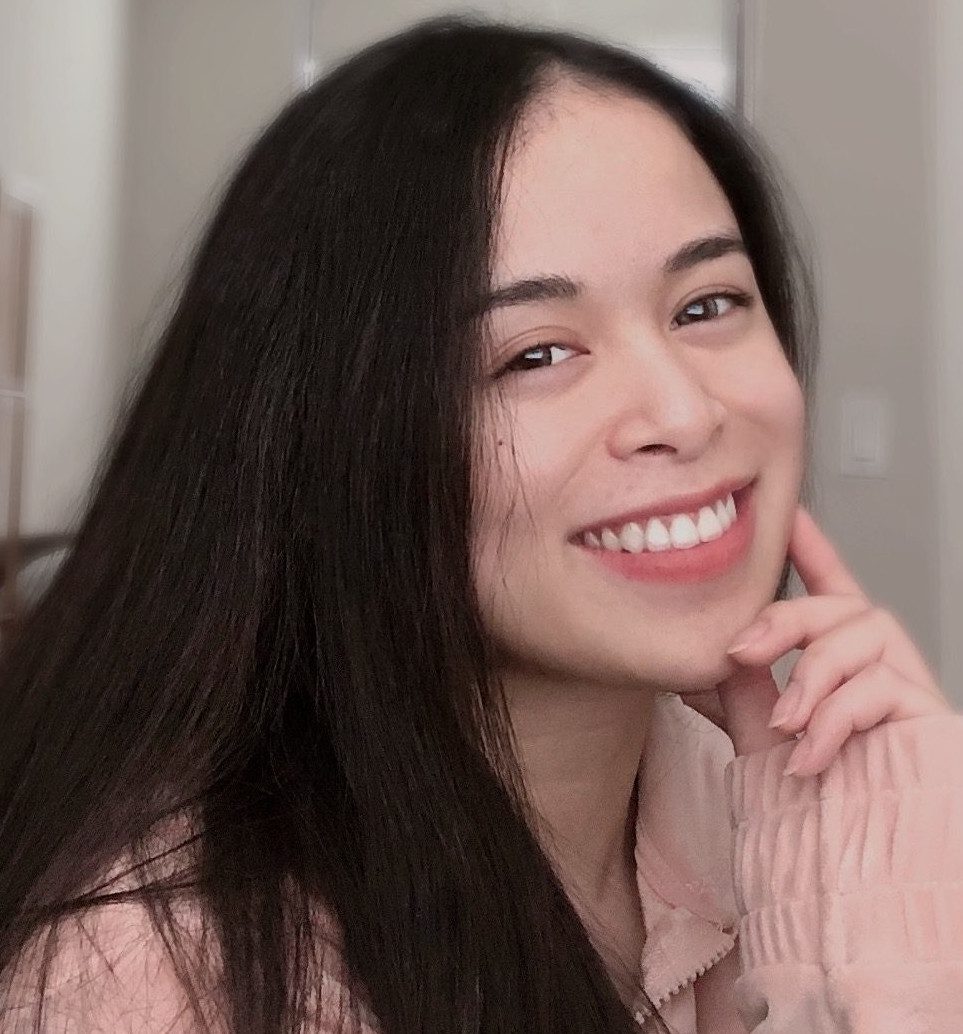
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Rwanda Izuba French Roast Vietnam Da La Arabica
In this example, our code iterates through every item in our coffees
array and prints out each item to the console. We’ve used a for-each loop instead of a “for” loop because it doesn’t require a counter, which means it is more concise and easier to read.
Changing Items in an Array
Houston, we have a problem. It turns out that our favorite French blend is not actually called French Roast. It’s called French Classic. If we want to change this, we can use indexing.
Consider the following code:
class CoffeeArray { public static void main(String[] args) { String[] coffees = { "Rwanda Izuba", "French Roast", "Vietnam Da La Arabica" }; coffees[1] = "French Classic"; System.out.println(coffees[1]); } }
We’ve used the assignment operator (=) to change the value of our coffee which exists at index position 1. We’ve changed its value from French Roast
to French Classic
. Our code returns: French Classic.
Java Array: An Example
Let’s bring together all of the concepts we’ve discussed in one example. Let’s say that we want to calculate how much we’ve spent on coffees in total over the last week.
First, we’re going to set up the class and the variables for our program:
class CoffeePrices { public static void main(String[] args) { Double[] purchases = {2.55, 2.75, 2.99, 3.05}; double sum = 0; } }
We’ve declared two variables. One variable stores our list of purchases. The next variable stores a counter of how much we’ve spent on coffee. Our next step is to create a for-each loop which loops through every coffee purchase and adds it to our “sum” variable:
for (double purchase : purchases) { sum += purchase; }
If we run our code, our program will calculate the total amount we’ve spent on coffees in the last week. There’s only one problem: our program doesn’t tell us what that value is! We can retrieve the value by printing it out to the console:
When we run all of this code together, the following is returned:
You've spent $11.34 on coffee in the last week. $11.34 on coffee. Not bad! What a useful program.
Conclusion (and Challenge)
Arrays are a type of data that can store multiple values of the same data type. You could declare an array for anything, such as a list of your favorite books, a list of players in a game, or a list of numbers of which you want to calculate the average.
Are you looking for a challenge? Read on.
Write a program that asks a user to select a travel destination to find out more about. You should store the travel destinations in an array. You should store a one-sentence description of each destination in a separate array.
When a user chooses a travel destination, find it in your array of travel destinations, and calculate its index number. Then, use that index number to print out the one-sentence description of the corresponding destination to the console.
Now you’re ready to start using arrays in Java like a professional!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.