How to Use Java’s “Abstract” Classes and Methods
Abstraction
is one of the three core principles in object-oriented programming—alongside encapsulation and inheritance.
Abstraction is one way to reduce the complexity of your programs and make your code easier to read. In Java, abstraction allows you to:
- show only certain details of a class and
- hide all other details of that class.
Abstraction can be applied to both classes and methods in Java.
This tutorial will discuss the basics of abstraction in Java, how to use abstract classes, and how to use abstract methods. We’ll also walk through a few examples to help you better understand this important concept.
Java Abstraction
Object-oriented programming is a type of programming that uses objects and classes to represent data and methods. Using objects and classes can reduce repetition in code and can make programs more efficient.
Java is an object-oriented programming language. As such, it includes a number of features used to work with objects and classes. Abstraction is one of those features. Abstraction allows you to show only certain information to a class in Java.
There are two instances where abstraction is used in Java: in classes and in methods.
Java Abstract Classes
In Java, an abstract class is a class from which you cannot create any objects. In other words, abstract classes cannot be instantiated.
To create an abstract class in Java, you can use the abstract keyword. Here’s an example of an abstract class in Java:
abstract class BankAccount { // Code here }
Since this is an abstract class, if we try to create an object with this class, our program will return an error.
For example, suppose we try to create an instance of a class called BankAccount. We want to call this instance lucyAccount, and we want it to store Lucy’s bank information. Lucy is one of our customers. We use the following code to try to create this instance:
BankAccount lucyAccount = new BankAccount();
When we run this code, however, our program returns:
BankAccount is abstract; cannot be instantiated
As you can see, you cannot assign abstract classes to an object.
However, you can still create subclasses from abstract classes. In the next section, we discuss how to create subclasses from abstract classes.
Abstract Class Inheritance
As we mentioned, you cannot assign objects an abstract class. So, in order to access the contents of an abstract class—in other words, the attributes and methods associated with that class—we have to inherit
the class.
Suppose we are creating a program that stores bank account information for a bank. Our program will include two classes: BankAccount and CheckingAccount. BankAccount will be a parent class that stores the attributes and methods for working with bank accounts. CheckingAccount will be a child class that inherits those methods.
For this example, BankAccount is an abstract class. We only want to use it to create checking accounts.
Here’s the code we can use to work with our BankAccount and CheckingAccount classes:
abstract class BankAccount { public void viewAccountNumber() { System.out.println("Account number: #1932042"); } } class CheckingAccount extends BankAccount { } class Main { public static void main(String[] args) { CheckingAccount lucyAccount = new CheckingAccount(); lucyAccount.viewAccountNumber(); } }
Our code returns:
Account number: #1932042
In this example, we created three classes:
- BankAccount. This is an abstract class. It contains a method called
viewAccountNumber()
. Because BankAccount is an abstract class, we cannot create an object of the class. - CheckingAccount. This is a child class. As a child class, it inherits the attributes and methods of the BankAccount class.
- Main. This class contains the code for our main program.
In our main program, we create an instance of the CheckingAccount class and call it lucyAccount. lucyAccount is an object. After creating the three classes in our program, we used the lucyAccount object in the method viewAccountNumber()
.
The following table summarizes key parts of this example:
NAME | TYPE | DESCRIPTION |
BankAccount | abstract/parent class | Stores the attributes and methods for working with bank accounts. |
CheckingAccount | child class | Inherits the methods of the parent class. |
Main | class | Contains the code for our main program. |
lucyAccount | object | The real-world application of our program. |
If you’re interested in learning more about inheritance in Java, read our complete guide here.
Java Abstract Methods
In addition to classes, methods can also be abstract.
When you declare an abstract method, you use the same abstract keyword as above. You do not specify any contents, and you can only declare an abstract method within an abstract class.
Here’s an example of an abstract method in Java:
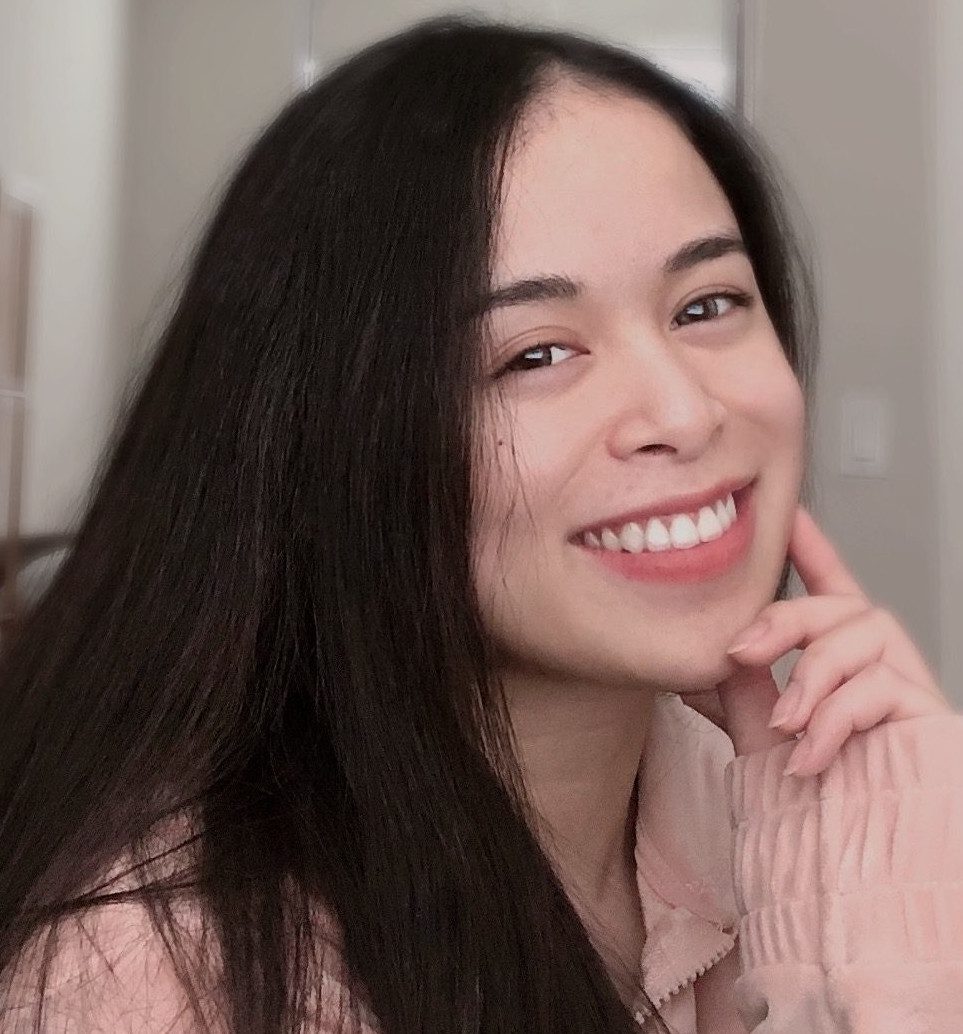
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
abstract void checkBalance();
In this example, we created an abstract method called checkBalance()
. This method has no body—in other words, there is no code associated with the method.
Let’s return to our bank example from earlier. That program contains an abstract method: lucyAccount.viewAccountNumber();
.
We used this abstract method to enable our program to display a customer’s bank account numbers. In the example above (from the previous section), the BankAccount parent class acts as a sort of template for child class(es).
In this case, the child classes are different kinds of bank accounts. This is because our customers may have more than one kind of bank account, and we may want to perform the same function on each kind of account. The child class we created above was: CheckingAccount.
Let’s go back to our customer from the example above. Lucy has a checking account, but she may also have a savings account and a brokerage account. We want to be able to display the account numbers for each of Lucy’s accounts. We can do so by creating child classes for these two additional account types. We could call them: SavingsAccount, BrokerageAccount.
Since we created the BankAccount parent class, we can efficiently and with minimal code repetition display account numbers for all three account types.
In the code below, we use the abstract keyword twice. First, we declare an abstract class (BankAccount). Then, we declare an abstract method that will later help us direct the program to display a customer’s account number(s). That abstract method declaration is: abstract void viewAccountNumber();
. Then we initialize the viewAccountNumber()
method on the CheckingAccount child class. Here’s the code:
abstract class BankAccount { abstract void viewAccountNumber(); } class CheckingAccount extends BankAccount { public void viewAccountNumber() { System.out.println("Checking account number: #1932042"); } } class Main { public static void main(String[] args) { CheckingAccount lucyAccount = new CheckingAccount(); lucyAccount.viewAccountNumber(); } }
Our code returns:
Checking account number: #1932042
This example returns the same output as our earlier example, but there are a few code differences to note. They are:
- Declaration of abstract method. In the example here, we declare an abstract class called BankAccount. This class contains an abstract method called
viewAccountNumber()
. - Extension of the abstract method. We extend the BankAccount class through the CheckingAccount class. The CheckingAccount class inherits the values stored in the BankAccount method.
In the CheckingAccount class, we define a method—called viewAccountNumber()
—that is only accessible to CheckingAccount objects. Then, in order to see the customer’s account number, we create a CheckingAccount object.
Java Abstraction Example
Now that we’ve discussed both Java abstract classes and abstract methods, we can explore a full example of abstraction in Java. To do so, we will bring together everything we discussed in the bank account examples above.
Suppose we are operating a bank that offers two types of bank accounts: checking accounts and savings accounts. We decide to write a program that allows bank account holders to check their account numbers.
At our bank, if a bank account is a checking account, the account number starts with the customer’s ID number and ends in 555. If the account is a savings account, the account number ends in 777.
We could use the following code to create this program:
abstract class BankAccount { abstract void viewAccountNumber(); } class CheckingAccount extends BankAccount { public void viewAccountNumber() { System.out.println("Checking account number: #1932042555"); } } class SavingsAccount extends BankAccount { public void viewAccountNumber() { System.out.println("Savings account number: #1932042777"); } } class Main { public static void main(String[] args) { CheckingAccount ellieCheckingAccount = new CheckingAccount(); ellieCheckingAccount.viewAccountNumber() SavingsAccount ellieSavingsAccount = new SavingsAccount(); ellieSavingsAccount.viewAccountNumber(); } }
Our code returns:
Checking account number: #1932042555 Savings account number: #1932042777
In this example, we created a class and called it BankAccount. This class had one abstract method, called viewAccountNumber()
.
Each type of account assigns account numbers differently, so we cannot implement the viewAccountNumber()
method in the BankAccount class. Instead, each subclass of the BankAccount class implements the viewAccountNumber()
method.
In this example, the CheckingAccount class assigns account numbers as a number that starts with the customer’s ID and ends with 555. The SavingsAccount class assigns account numbers as a number that starts with the customer’s ID and ends with 777.
In our code, we initialize an instance of the CheckingAccount class for a customer called Ellie. This instance is assigned the name ellieCheckingAccount, and we invoke the viewAccountNumber()
method to view the account’s number. This returns:
Checking account number: #1932042555
We also initialize an instance of the SavingsAccount class, called ellieSavingsAccount, and we invoke the viewAccountNumber()
method to view the account’s number. This returns:
Savings account number: #1932042777
Conclusion
Abstraction
is an important feature of object-oriented programming. It allows you to hide certain details in a class or method and display only essential information. To create abstract classes and methods in Java, use the abstract
keyword.
This tutorial discussed, with examples, how abstraction works, why abstraction is useful, and how you can create abstract methods and classes in Java. Now you’re ready to start working with abstract classes and methods in Java like a professional programmer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.