HTML Tables are sets of data that are presented in rows and columns. To make an HTML table use the <table>
element. You can use <tr>
to create rows, <td>
to create columns, and <th>
to create table headers.
Tables are used to present data in an easy-to-understand way by using rows and columns. We encounter tables every day, from the bus timetable that tells riders when a bus will arrive, to the table of ingredients in the foods we eat.
In HTML, tables are used as a method of presenting data. This tutorial will discuss, with examples, the basics of HTML tables, cells that span multiple columns and rows, and how to style a table. By the end of this tutorial, you’ll be an expert at creating and working with tables in HTML.
Creating an HTML Table
Tables are sets of data that are presented in rows and columns. By using a table, it is easy to see the connections between a set of values in a dataset.
In HTML, tables are defined by using the <table>
element. Within the <table>
element, you can use:
- <tr> to create rows
- <td> to create columns
- <th> to create table headers.
Suppose we wanted to create a table that lists the coffees sold in a coffee shop alongside their prices, like we did above. This table should have two columns, coffee and price, and three rows. We could use the following code to define this table in HTML:
<table> <tr> <th>Coffee</th> <th>Price</th> </tr> <tr> <td>Espresso</td> <td>$2.00</td> </tr> <tr> <td>Cappuccino</td> <td>$2.50</td> </tr> <tr> <td>Latte</td> <td>$2.75</td> </tr> </table>
Here is the result of our table:
In this example, we have created a table with one row of headings, two columns, and three rows of table data. Let’s break down our code.
First, we used the <table>
HTML tag to instruct our web page to create a table. We then used a <tr>
tag to create a row, and <th>
tags to define the column headers for our table. In this case, our column headers are Coffee
and Price
.
Next, we used three more <tr>
tags to create each of the three table rows that showcase our coffee names and prices in the table’s data cells. Each of these <tr>
tags contains a <td>
tag, which is used to represent a column cell in the table. For instance, the td element is used to represent Espresso
and $2.00
in our first coffee entry.
Table Styling
There are a number of ways in which we can style a table in HTML.
Borders
In HTML, tables have no borders by default. This means that if we want a border to appear on our table, we need to use CSS. The CSS border attribute is used to add a border to an HTML table.
Suppose we wanted to add a solid black border around our table from earlier. We could do so using this code:
table, th, td { border: 1px solid black; }
When we use this style in our table code, our table changes to include a border around each of its cells and the table itself. Here is our revised table:
Now our table has a border, which makes it easier to read the data in our table. However, the style we have created above places a border around every cell and separates out each border. If we want to collapse each border into one, we can use the border-collapse property. Here’s the code we would use to accomplish this task:
table { border-collapse: collapse }
Our new table looks like this:
As you can see, our table now has one border, rather than one border around each cell and the table itself.
Align Headings
By default, headings are aligned to the center of a cell. If you want to align a heading to the left or right of a cell, you can specify a text-align property to the <th>
tag in your table (remember, the <th>
tag is used to specify headings).
Here’s the code you would use:
th { text-align: left; }
If we apply this style to our table from above, the following table is created:
Our above table has aligned the column headings to the left of our table.
There are plenty of other styles that can be applied to an HTML table, but for the purposes of this tutorial we have focused on table borders and collapsed borders. Going forward, we are going to use the styles we have defined in this part of the tutorial to make our data easier to read.
Spanning Multiple Rows and Columns
When you’re working with tables, you may want a cell to span multiple rows or columns.
For instance, suppose we wanted our price column from earlier to span over two columns. That’s where the colspan and rowspan attributes come in. Rowspan is used to span multiple rows in a table, and colspan is used to span multiple columns in a table.
Here’s an example of a table that uses colspan to span the Price
column over two columns:
<table> <tr> <th>Coffee</th> <th colspan="2">Price</th> </tr> <tr> <td>Espresso</td> <td>$2.00 (new)</td> <td>$2.00 (old)</td> </tr> </table>
Here’s how our table appears:
As you can see, the price column now spans over two rows. In addition, you can use rowspan in the same way to create a cell that spans over more than one row.
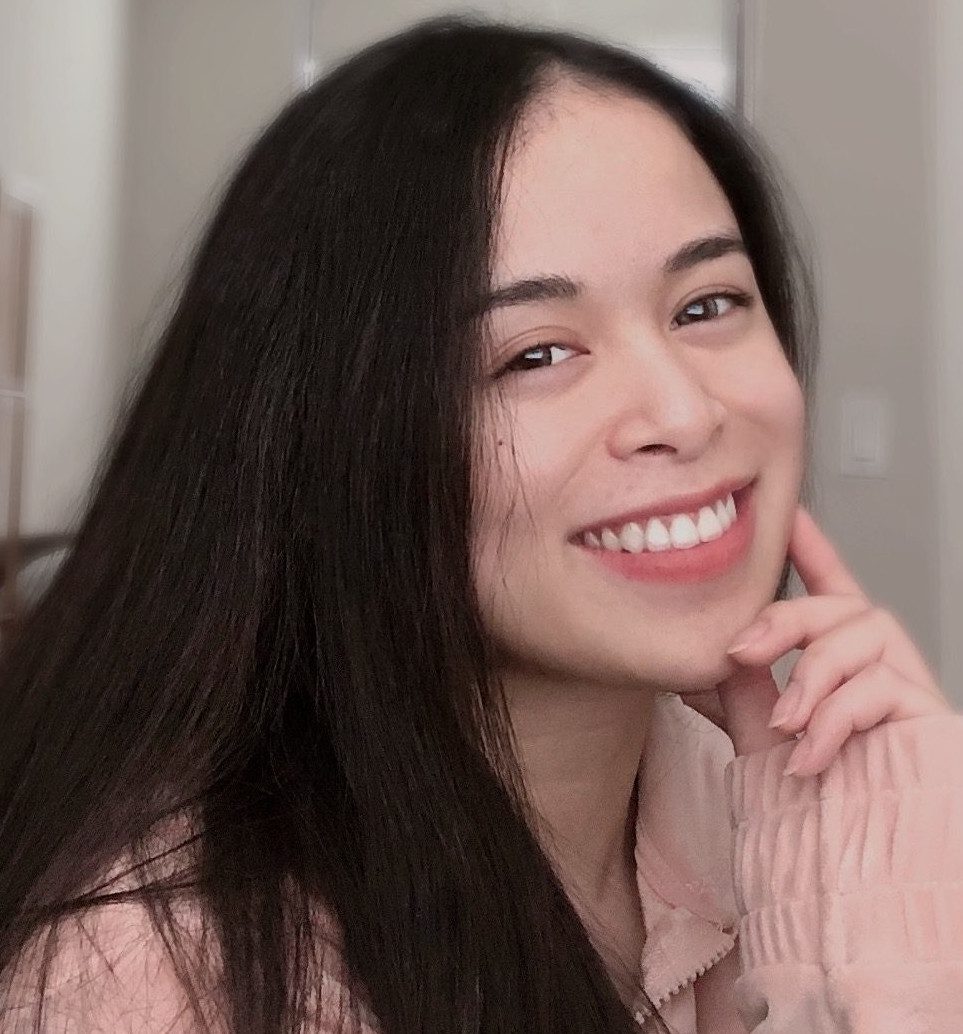
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Suppose you have a table that stores the details about a specific coffee on the menu.
You want the information about the coffee’s discounted price—which is applied during the last day of every month to entice customers to buy a coffee—and the regular price to appear under the same heading Price
, with labels to distinguish the prices. You could use the following code to accomplish this task:
<table> <tr> <th>Coffee</th> <td>Espresso</td> </tr> <tr> <th rowspan="2">Price</th> <td>$2.00 (regular)</td> </tr> <tr> <td>$1.80 (discounted)</td> </tr> </table>
Here’s the output of our table:
In this example, you can see that the Price
label spans across two rows.
Defining a Table Header, Body, and Footer
When you’re working with a table, there are three tags that are used to help you better structure your data.
The <thead>
tag is used to define the heading of your table, the <tbody>
tag is used to define the contents of your table, and the <tfoot>
tag is used to define the footer of your table.
Let’s take our table from the first example to illustrate how this works. Suppose we are building a table that lists all the coffees sold at a coffee shop. To make our code easier to read, we want to separate out the header, body, and footer of our table. We could do so using this code:
<table> <thead> <tr> <th>Coffee</th> <th>Price</th> </tr> </thead> <tbody> <tr> <td>Espresso</td> <td>$2.00</td> </tr> <tr> <td>Cappuccino</td> <td>$2.50</td> </tr> <tr> <td>Latte</td> <td>$2.75</td> </tr> </tbody> <tfoot> <tr> <th>Last Updated</th> <td>January 9th, 2020</td> </tr> </tfoot> </table>
In this table, we have used the <thead>
, <tbody>
, and <tfoot>
tags to define our table header, body, and footer, respectively.
The <thead>
tag is used to distinguish our header row, which contains the Coffee
and Price
table headings. The <tbody>
tag is used to store the rows which contain the main data for our table (the coffee prices).
The <tfoot>
tag is used to store a row which states when the table was last updated. We have placed this data in a footer tag because it is not an entry for our list of coffees and their prices.
While these tags are optional, they do help you better structure a table in HTML.
Table Captions in HTML
The <caption>
tag is used to add a title, also known as a caption
, to your tables.
The <caption>
tag must be placed immediately after the opening <table>
tag in your table. Here’s an example of the <caption>
tag being used to add the header Coffee Menu
to our list of coffees:
<table> <caption>Coffee Menu</caption> <tr> <th>Coffee</th> <th>Price</th> </tr> <tr> <td>Espresso</td> <td>$2.00</td> </tr> <tr> <td>Cappuccino</td> <td>$2.50</td> </tr> <tr> <td>Latte</td> <td>$2.75</td> </tr> </table>
Our table appears as follows:
In our code, we use the <caption>
tag to add the header Coffee Menu
to the table.
Conclusion
Tables can be as simple or as complex as you want to make them, and this article has covered just about all you need to know about the basics of tables in HTML.
This article discussed how to create a table in HTML, how to apply basic styles to a table, how to structure a table, how to span cells over multiple columns and rows, and how to use captions with a table.
Now you have the knowledge you need to start working with tables in HTML like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.