HTML forms collect data from users with text fields, dropdowns, checkboxes, and more. Forms in HTML are enclosed within the <form>
tag and use the <input>
tag to create elements.
HTML forms provide a way for users to interact with a website or web application. Web forms allow users to submit data that a website or server can then process and store.
How do you create a form in HTML? That’s the question we will answer in this tutorial.
This tutorial will discuss, using an example, the basics of HTML forms, how to add input fields to a form, and how to use a form to send data to a server. By the end of this tutorial, you’ll be an expert at creating forms in HTML.
HTML Forms
In HTML, forms are made up of one or more elements. HTML forms can include the following elements:
- single-line text fields
- text boxes
- dropdown boxes
- checkboxes
- buttons
- radio buttons
These elements, aside from buttons and text boxes, use the <input>
tag in HTML code.
After a user submits a form, the form’s contents can:
- be sent to a server for further processing or
- be processed by the web browser.
Before you create a form in HTML, you should think about how you will design your form. What data do you need to collect? How will you keep your form simple? The larger your form is, the less likely it is that users will fill it out.
For this tutorial, we will make a form for a coffee house’s website. Suppose that the Three Brothers Coffee House asked us to design a form that lets them accept customer queries through their website.
We are tasked with creating a form that accepts four pieces of information. These are:
- The name of the user.
- The user’s email address.
- Whether the user has ever visited the coffee house.
- The message the user wants to send to the coffee house.
This means that our form will need to include four elements. Our form will also need one button so the user can submit their form.
HTML <form>
Tag
Forms in HTML are enclosed within the <form>
tag. Here’s an example of a form in HTML:
<form>
// Elements
</form>
The <form>
tag is used to define a form in HTML. Once defined, the <form>
tag can include form elements, such as checkboxes and single-line text fields.
The form element accepts two attributes: action
and method
. We’ll discuss these attributes later on in this tutorial.
<input>
Elements for HTML Forms
Most HTML form controls include input elements. These are used to collect different types of data from the user. Input elements are defined using the <input>
tag.
The way the <input>
element appears on a website depends on the type attribute specified. For example, if an input field is a text box, it will appear differently than if it were a radio button.
The syntax for the <input>
element is as follows:
<input type="type" id="elementId">
In this example, <input>
accepts two attributes. The first attribute, called type
, is the type of input the form field will accept. The type
attribute can have many values, but some of the most common are:
button
checkbox
email
text
number
radio
The second attribute in our example is id
. This attribute is used to uniquely identify the input element, and will be used to access the data the user has submitted by the script that we use to process the user’s form inputs.
Let’s return to the Three Brothers Coffee House. The form we are building needs to accept four pieces of information about our user. The first two pieces of information we want to collect are the user’s name and email address.
We can collect these pieces of information using two <input>
tags:
<form>
<label for="name">Name:</label><br/>
<input type="text" id="name" name="name"><br /><br />
<label for="email">Email:</label><br/>
<input type="email" id="email" name="email">
</form>
Click the button in the editor above to run the code.
As you can see, we created a form that accepts two values: the user’s name and the user’s email address. In this example, we also used the <label>
element. We will discuss this element soon.
The name
Attribute in HTML Forms
Every form element in an HTML form must have a name
attribute. This attribute is used to submit the contents of a form. If you do not specify a name
attribute in a form element, that element’s contents will not be submitted to the server.
In the above example, our “Name” field has a name
attribute with the value name
, and our “Email” field has a name
attribute with the value email
.
HTML <label>
Element
In our above example, we used the <label>
element to add an HTML label to each item in our form. The <label>
tag lets us clearly state the purpose of a form element and what data it accepts.
A <label>
tag also allows us to define the text that the computer will read to users who utilize screen-readers. This is because the screen reader will read the label when it encounters the input field on the web page.
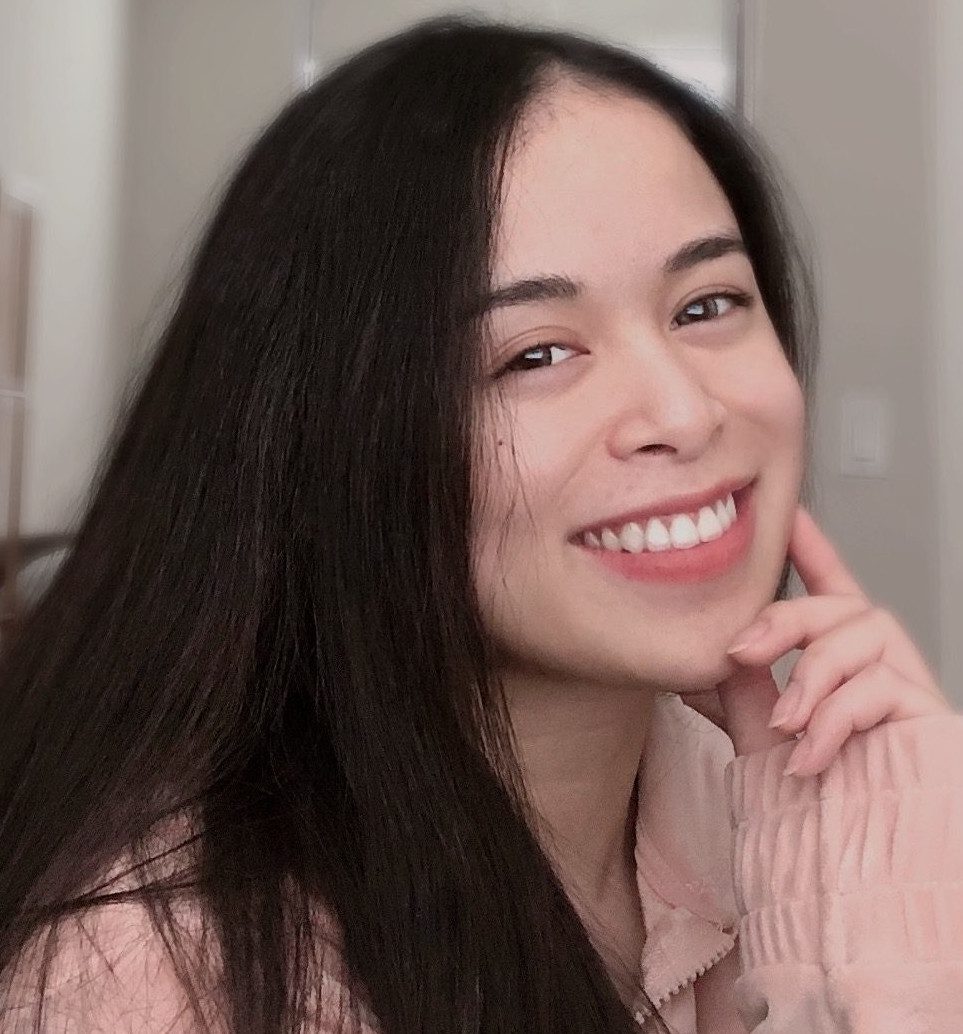
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Here’s the syntax for an HTML label:
<form>
<label for="name">Name:</label><br/>
<input type="text" id="name" name="name">
</form>
In the above example, we created a form that collects a user’s name. We used a <label>
tag to add the label Name:
to the form. Notice that we also specified an attribute called for
in our <label>
element. This attribute should be equal to the id
attribute used by the input
element to which the label is linked, as it binds a form to its corresponding label.
HTML Form Elements
Let’s return to the Three Brothers Coffee House. At the start of this tutorial, we said that we wanted to collect four pieces of information: the user’s name, their email address, whether they are a customer, and the message they want to send to the coffee house.
So far, we have created two form fields: the name field and the email field. Let’s discuss how we can create the rest of our form.
Radio Buttons
Radio buttons are a common type of input used in HTML forms. A radio button allows a user to select one value from a limited number of choices. Often, radio buttons are used for forms that collect gender information, age ranges, or yes/no responses.
The following is the syntax for a radio button:
<input type="radio" name="radio_button">
On our form, we want to ask the user whether they are a customer of the Three Brothers Coffee House. We can use a radio button to accomplish this task. Here’s the code we will use to do so:
<form>
<p>Are you a customer of the Three Brothers Coffee House?</p>
<input type="radio" id="yes" name="customer" value="yes">
<label for="yes">Yes</label><br />
<input type="radio" id="no" name="customer" value="no">
<label for="no">No</label><br />
</form>
Click the button in the editor above to run the code.
Our user can then submit one of two values: yes
or no
.
Let’s break down our code. First, we define a <form>
. We then use the <p>
tag to add some explanatory text for the user.
On the next line, we create a radio button that has the value yes
. Then we create a label for this button, which displays the text “Yes”. Next, we create a radio button with the value no
and create a label for that button, which displays the text “No”.
Notice that in our code our radio buttons have a value
attribute specified. This value is the data that will be sent to the server when the user submits the form. So, if the user clicks on the “Yes” radio button, the value yes
will be sent to the server.
HTML <textarea>
Element
We have one more input element that we want to add to our form: a text area. So far, we have collected a user’s name, their email address, and whether they are a customer. Now we want to add in a form element that allows them to send a message to the coffee house.
We can do so using the <textarea>
element, like this:
<form>
<label for="message">Message:</label><br />
<textarea id="message" name="message">
What message do you want to send to the Three Brothers Coffee House?
</textarea>
</form>
In our code, we start by defining a label
that displays the value “Message:”.
We then define a text area using the <textarea>
tag. We specify two attributes for this text area:
id
. This is used to reference the contents of our form after the user submits their response.-
name
. This is required in order for the contents of our form to be submitted.
Finally, we add the message What message do you want to send to the Three Brothers Coffee House?
between our opening and closing <textarea>
tags. This is the default message that the user will see.
The result of our code is as follows:
Click the button in the editor above to run the code.
Now we have a text area field. Users can utilize this field to submit a message to the coffee house.
HTML submit
Button
Now that we’ve defined the contents of our form, we are ready to add a submit
button. Here’s the syntax for the submit
button:
<input type="submit" value="Submit">
Submit
buttons are used to submit the contents of a form to a form handler. (A form handler is usually a page on the web server that can process the contents of the form.) The submit
button links to the form handler. You need to specify the form handler using the <form>
tag’s action
attribute.
Suppose we want to add a submit
button to our web form for the coffee house. We can do so using this code:
<form action="/post.php">
<label for="name">Name:</label>
<br/>
<input type="text" id="name" name="name">
<br />
<br />
<label for="email">Email:</label>
<br />
<input type="email" id="email" name="email">
<br />
<p>Are you a customer of the Three Brothers Coffee House?</p>
<input type="radio" id="yes" name="customer" value="yes">
<label for="yes">Yes</label>
<br />
<input type="radio" id="no" name="customer" value="no">
<label for="no">No</label>
<br /><br />
<label for="message">Message:</label><br />
<textarea id="message" name="message">
What message do you want to send to the Three Brothers Coffee House?
</textarea>
<input type="submit" value="Submit">
</form>
As you can see, we added a submit button to our form. We also specified an action
parameter in the <form>
tag. This parameter links the form to the file that will handle its contents.
Submitting a Web Form
After you’ve created a HTML form, you need to create a handler that will process the data that users submit through your form. The <form>
element provides two attributes that are used to send form data to a server: action
and method
.
Form action
Attribute
The action
attribute defines the location of the form handler code. When your form is submitted—when the user clicks the submit
button—the data it collected will be sent to the form handler.
In our above example, when the form is submitted, the data from the form is sent to the page post.php
, which includes our form handler code. Here’s an example of a form with the action
attribute defined:
<form action="/post.php">
</form>
If there is no action
attribute specified, the form is submitted to the current page.
Form method
Attribute
The method
attribute states the HTTP method that should be used when a form is submitted. By default, forms use the GET
method, but you can also use any other HTTP method, such as POST
.
Here’s an example of the form method
attribute in action:
<form action="/post.php" method="POST">
</form>
When this form is submitted, the data it collected will be sent to the server using an HTTP POST
method. (Alternatively, had we wanted to send a GET
request, we could have specified "GET"
, or just left it blank because GET
is the default method.)
In most cases, you should use the HTTP POST
method to submit data. This is because the GET
method makes the form data visible in the page’s address field. So, had we submitted a form to post.php with a field called “name” that contained the value “Pauline”, the following would have been submitted to the website:
/post.php?name=Pauline
This is an insecure method of sending data. The GET
method should never be used if you are handling sensitive information.
Conclusion
Forms are an important feature of HTML that allow you to collect and process user data.
In this tutorial, we discussed—using an example—the basics of forms in HTML and how you can create your first web form. Now you’re ready to start designing and developing your own HTML forms like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.