The JavaScript ternary operator evaluates a statement and returns one of two values. A ternary operator can be instructed to return a value if the statement evaluates to either true or false. The syntax for the ternary operator is: statement ? if_true : if_false;.
There may be times when you only want certain code to run when certain conditions are met.
For example, let’s say you have a date of birth field on your e-commerce website. Let’s also say you only allow customers over the age of 18 to purchase goods on your website. You’ll want to check that a user is of the right age before they use their site.
The potential outcome is true or false, so we can use a ternary operator to check if a user is the right age.
In this tutorial, we’re going to discuss how to use ternary operators. We’ll refer to an example to help you get started.
What is a JavaScript Ternary Operator?
JavaScript ternary operators, known as conditional ternary operators, are similar to JavaScript if…else statements. A ternary operator checks if a condition is met, and do something depending on whether that condition is or is not met.
The ternary expression has the following syntax:
(condition) ? if true, then run : if false, then run
A condition comes first, then a question mark. The first expression will execute if the condition is met. The second condition second one will execute if the condition is not met.
Back to the Basics: if Statements
The most basic JavaScript conditional operator is an “if” statement. This statement allows executes a block of code is a condition is met. Otherwise, the program executes the contents of an optional “else” statement.
Here is an example of an if statement. We use the scenario of an e-commerce site that verifies the age of its users that we discussed earlier.
// Declare our customer object let customer = { name: "Joe", age: 15 } if (customer.age >= 18) { user_is_right_age = true; } else { user_is_right_age = false; }
Our if statement will run, and user_is_right_age will be set to false. The program first checks if the customer’s age is 18 or above. Because the user is not over 18, the else clause executes, and sets user_is_right_age to false.
Although this code works, it is a lot of code! It takes us five lines to verify the age of our customer.
What if I told you there was a way to perform this same function in only one line of code? That’s where the ternary operator comes in.
JavaScript Ternary Operator Examples
We can simplify our previous if statement by using a ternary operator:
user_is_right_age = customer.age >= 18 ? true : false;
This code, when executed, gives us the same result as our above code (false), but in fewer lines. If the conditional were true—if the user’s age was 18 or above—then our program would return true.
In the example we used above, our program checks whether the user is the right age. Our code returns false because the user is under 18—our condition was not met.
Let’s move on to another example. Say we want to check whether a customer on our site is eligible for express delivery. We could do this with a JavaScript ternary operator:
let userIsExpressCustomer = true; let shippingTimeForCustomer = userIsExpressCustomer ? 48 : 72;
Our ternary operator will check if the user is an express customer, then it will determine the shipping time for the user. As seen above, our user is an express customer, so the condition evaluates to true, they get 48-hour delivery. If our customer was not an express customer, our code would evaluate to false. Thus, the customer would receive 72-hour delivery.
Nested JavaScript Ternary Operators
A nested JavaScript ternary operator is a ternary within another ternary. These statements are useful if you want to evaluate multiple operations. But, they can quickly become difficult to read if you are not careful.
Let’s say we are an e-commerce company that offers three delivery times: standard (72), express (48 hours); and one-day (24 hours). How do we use a ternary operator in this case, given there are three options? Should we just use an if…else statement instead?
Don’t worry, you can also use a JS ternary operator with three expressions. Here’s an example of the above example in action:
let userIsExpressCustomer = false; let userIs24HourCustomer = true; let shippingTimeForCustomer = userIsExpressCustomer ? 48 : userIs24HourCustomer ? 24 : 72;
In this case, the shipping time for our customer would be 24 hours. This program first checks if the user is an express customer, which they are not. Then, it checks if they are a 24-hour customer, which is true. So, the shippingTimeForCustomer variable is assigned the value 24.
If the user was not an express or 24-hour customer, their delivery time would be 72 hours.
Conclusion
The JavaScript ternary operator is an alternative to the if statement when you want to evaluate whether a condition is true or false. You can enclose multiple ternary operators within another operator.
Conditional statements like the ternary operator run certain code only when specific conditions are met. They are one of the key components of almost every programming language and will come up in almost every JavaScript program you write.
Are you interested in learning more about JavaScript? We’ve got you covered. We have a complete guide on how to learn JavaScript. In this guide, you’ll find all the resources you will need to further advance your knowledge of JavaScript.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
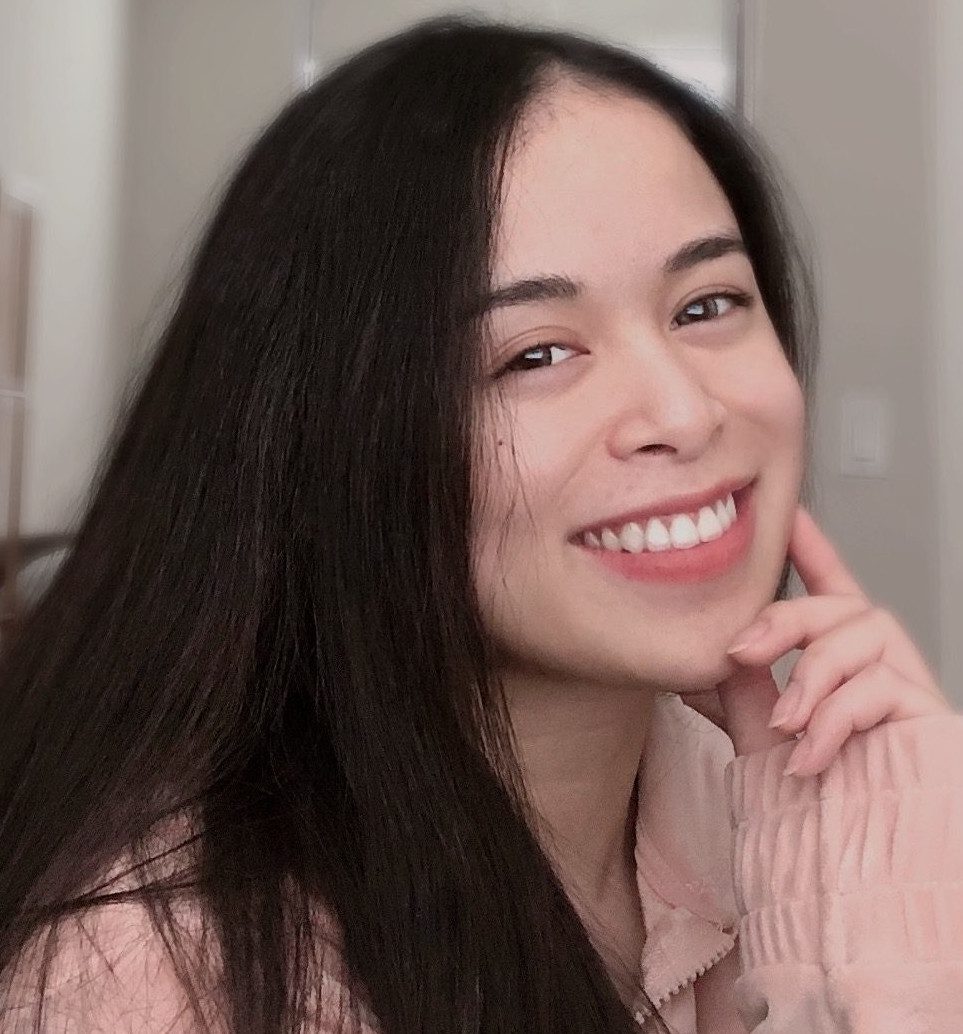
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot