JavaScript functions are custom blocks of code that can be reused. Functions allow code to be more modular and are essential to object-oriented programming. Functions can be defined through declarations or expressions.
If you’re trying to learn JavaScript and looking to know the basics, JS functions are definitely something you need to know. If you have spent any time working with a programming language, you’ll know that functions are the building blocks of complex programs, but you may not know how they work. Functions allow you to write code once for common processes, instead of repeating it multiple times.
Functions are blocks of code that perform an action and may return a value. Functions can be customized based on your needs and can be used to make your code more efficient and modular.
In this tutorial, we’ll explore the basics of functions: how to define a function, how to call a function, and when they may be useful.
How to Use the JavaScript Define Function
There are two ways to define a function in JavaScript: through declarations and expressions. Let’s start with the declaration method of defining a function.
JavaScript Function Declarations
Function Declarations define a named function. In order to define this type of function, you should start your code with the function
keyword, followed by the name of the function. Here’s an example:
function nameOfYourFunction() { // Function code }
Function names follow the same rules as variables—they can use leers, underscores, numbers, and are often written using camel case. Then, after the variable name, you’ll include a set of parentheses, where optional parameters can be held. We’ll get to those later in the article.
Then, like a for or an if statement, the code of the function will be in curly braces. Here is an example of a function that will print Google
to the console:
function printGoogle() { console.log(“Google”); }
Within the printGoogle()
JS function is a console.log()
statement that will be executed when the function is called. But, nothing will happen until we call the function. If we want to call the function, we can use this code:
printGoogle();
Now, let’s merge our code together into one function, then invoke it:
// Declare the printGoogle() function function printGoogle() { console.log(“Google”); } // Invoke the printGoogle() function printGoogle();
The output for this function will be Google
. The printGoogle()
function will return the result when it is invoked, on the last line in this case.
Now our print code is in a function, we can execute it as many times as we want by calling the printGoogle()
function.
JavaScript Function Expression
Another way of declaring a function is to create a function expression. We can do so by assigning a function to a variable.
Let’s use the same example as above. Rather than declaring the function on its own, we can instead assign it to a variable. Here’s an example:
const google = function printGoogle() { console.log(“Google”); }
In order to call this expression, we add the google()
line of code to wherever we want the expression to be run.
Function Parameters
Now we know the two ways in which we can declare a function, we can explore how to customize this feature of programming. In our above code, we have created a function that prints Google
to the console.
In order to print a different name, such as Facebook, we’d need to change the code. If we want users who visit our website to input the name of their favorite company and print it to the console, our function would not work.
So, we need to make use of parameters. If we add a name
parameter to our JS function, then we can print any name to the console through our function. Here’s an example:
function printCompany(name) { console.log(`My favorite company is ${name}!`); }
The name of the function is printCompany()
and our parameter is called name
. The parameter can then be called within the JavaScript function. In the above example, we use the name parameter to change what will be printed on the console.log()
line of code.
But we haven’t defined our name yet. To define the parameter, we need to assign it a value when we call our JavaScript function. Let’s say your favorite company is Snapchat. We’ll call the function and place the company name as an argument within the function call.
Here’s an example:
// Call printCompany() function with “Snapchat” as “name” printCompany(“Snapchat”);
When we run this code, the following will be printed:
My favorite company is Snapchat!
In our example, we call the function using printCompany()
, then we assign the name in parenthesis. So, now we can reuse our function multiple times with different names.
It’s worth noting that you can use as many parameters as you want, and you will reference them in order. We’ll use an example showing this in action below.
Return Values
In our examples so far, we have not returned any values. Instead, we have printed some text to the console. But, with a function, we can give it some parameters to process, then return a value based on what’s in the return
statement.
Here’s an example of a function adding two numbers and giving us the total:
function addNumbers(first, second) { return first + second; } addNumbers(1, 2);
In this program, our function is called and two numbers are passed through the function. When this function is executed—on the final line in our above example—we’ll get the answer 3
in return. Our list of parameters is separated by commas.
The program has added 1 and 2, with the parameter names first
and second
and returned them to the code. If we want to see these values, we can add a console.log()
function around the line where we call addNumbers(1, 2)
.
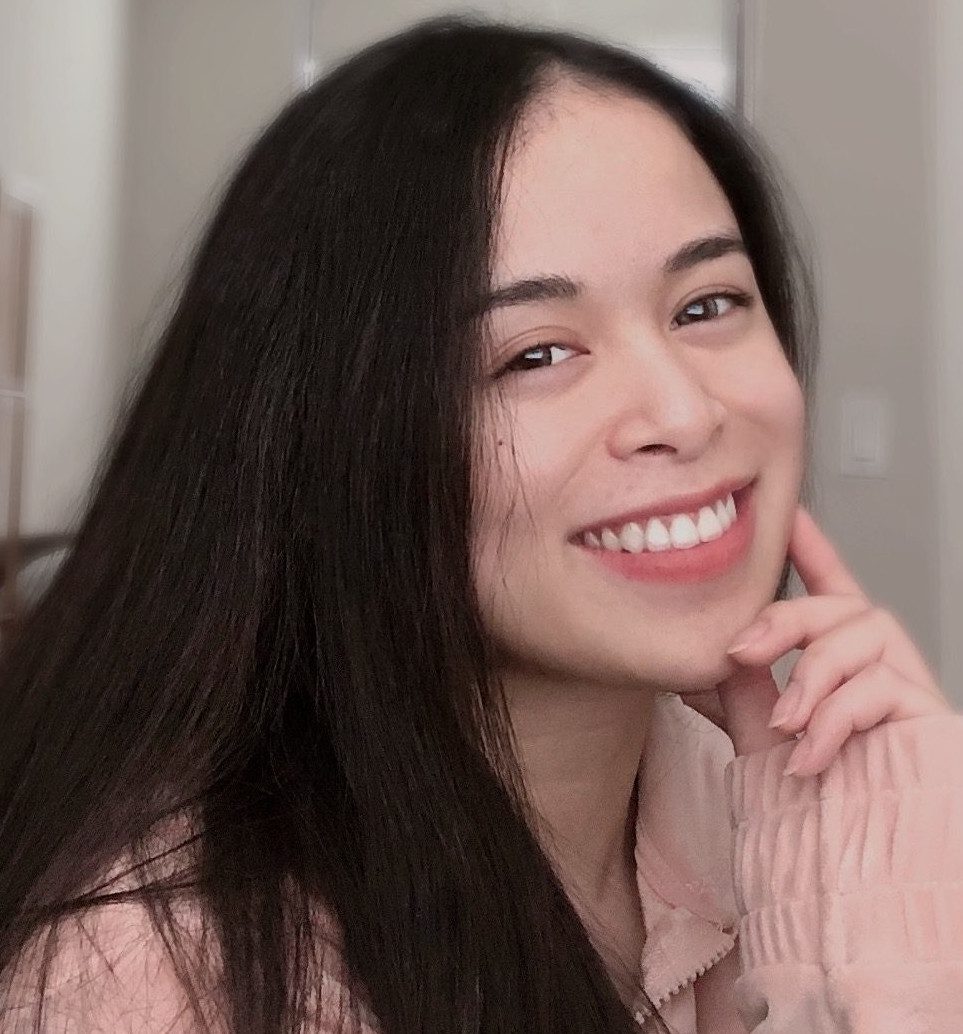
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Arrow Functions
As of ECMAScript 6, there is a more concise way to define functions known as the arrow function. These are represented by using the following expression: =>
.
These functions are a type of function expression. Let’s use an example to showcase arrow functions in action:
const addNumbers = (first, second) => { return first + second; } addNumbers(10, 15);
Instead of writing function
, we can use the arrow sign to denote we are declaring a function. There are a couple of subtle differences between arrow functions and regular functions, but you don’t need to know about those for most use cases.
When you’re only working with one variable, you don’t need parentheses around them. And, if you’re not working with any variables, you need to include a set of empty parentheses ()
where your variables would be declared.
Conclusion
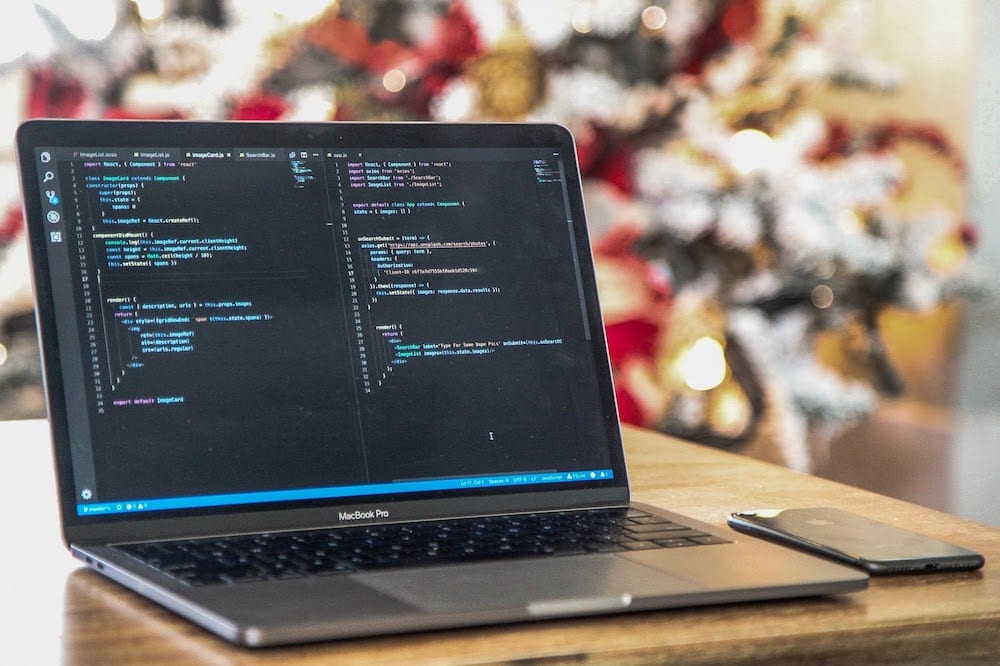
That’s all you need to know about the basics of functions. In this tutorial, we’ve covered function declarations, function expressions, returning values from functions, and arrow functions.
Overall, a function is a block of code that can perform an action and return a variable. Functions are useful if you have multiple lines of code that you may need to execute many times throughout a program.
Instead of writing those multiple lines of code every time you need to use them, you can add them to a function and call the function when you need to run the code, and pass the values you need to use into your function.
Here are some main takeaways from this article:
- Functions are subprograms designed to run certain lines of code.
- Functions are only executed when they are called. We call this invoking a function.
- Values can be passed into functions, then used within the function.
- Functions can return values.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.