How to Learn TypeScript
Over the past few years, TypeScript has become an increasingly common language used by developers.
TypeScript, created by Microsoft in 2012, is a typed super set of JavaScript that allows you to write scalable and efficient web applications. Developers often talk about how TypeScript lets you “write JavaScript the way you want to.” This refers to how the language gives you more control over your programs.
According to RedMonk’s January 2020 Programming Language Rankings, TypeScript is the seventh most popular programming language used by developers. This index tracks the popularity of various different programming languages/
In this guide, we discuss the best way to learn TypeScript online. We’ll provide you with a few tips on how you can master your TypeScript skills.
What is JavaScript?
Before discussing TypeScript, let’s refresh our memories on another programming language: JavaScript.
JavaScript is a scripting language that allows you to build dynamic websites. You can add features to sites such as animated images, interactive buttons, and components of a web page using JavaScript. These elements change when a user interacts with them.
Any time you see a web page that does more than just show text, JavaScript is involved in some way.
What is TypeScript?
TypeScript is an open-source, typed super set of the JavaScript programming language. TypeScript was created by Microsoft in 2012 to make it easier for developers to create large, scalable applications. It is widely used by companies like Slack, Asana, and Microsoft.
The TypeScript language uses the same syntax and semantics as the JavaScript language. There are a few differences that makes it easier to write scalable code. As a result, you can use your existing JavaScript code to start a TypeScript application, then call your TypeScript code from a JavaScript application.
The TypeScript feature set offers support for all the latest JavaScript features. This means that when you use TypeScript, you don’t miss out on other features that have been introduced into JavaScript.
Whereas JavaScript is a dynamic scripting language, TypeScript is a static scripting language that lies on top of JavaScript code. TypeScript is not a replacement for JavaScript. It is a complementary technology used alongside JavaScript, especially when you are looking to build highly-scalable web apps.
These are main differences between JavaScript and TypeScript:
- TypeScript offers static typing, whereas JavaScript offers dynamic typing.
- TypeScript uses types and interfaces.
- TypeScript supports optional parameter functions, whereas JavaScript does not.
What is TypeScript Used For?
TypeScript is a modern web development framework. It extends JavaScript with static type definitions. These definitions make it easier to describe how an object is structured, which enhances code readability.
All JavaScript code can be TypeScript code. This is because TypeScript only extends on JavaScript. It does not replace the framework. TypeScript code is turned into JavaScript code when it is compiled.
There is no need for a developer to change their entire application to support TypeScript. At any moment, TypeScript can be added to either a client- or server-side application.
Because TypeScript is based on JavaScript, any transition will involve adding TypeScript code rather than removing JavaScript code.
How Long Does it Take to Learn TypeScript?
It takes about a month to learn the basics of TypeScript, assuming you study for at least one hour a day. Expect to spend at least six months studying TypeScript before you develop the skills you need to apply it in a professional development setting.
Because TypeScript is based on JavaScript, a lot of the terminology you hear will be familiar. This will speed up the learning process. But, TypeScript is its own technology. So, there will be a lot of concepts that you’ll need to learn about from scratch.
The time it takes you to learn TypeScript depends on how much time you commit to studying. If you study full-time, you’ll be able to learn TypeScript quicker than someone who is only studying part-time.
Is TypeScript Hard to Learn?
Learning TypeScript is a bit more difficult than learning JavaScript. This is because TypeScript extends upon JavaScript and so you need to have a good understanding of how JavaScript works first. But, with some practice and time, you should have no trouble learning TypeScript.
TypeScript has a lot of features that are not supported in JavaScript. These include decorators, access modifiers, enums, static typing, and interfaces. Expect to spend some time learning about completely new concepts that you may never have encountered in web development.
Why Should You Learn TypeScript?
While JavaScript is great for flexibility, it is not so great for building highly scalable web applications in many cases. By learning TypeScript, you’ll have a whole new set of tools that you can use to write web applications that scale effectively.
Many web developers are learning TypeScript because the language offers static typing. Static typing can improve your development experience by making it easier to write readable code. For example, tools like TSLint and TSServer allow you to improve the style and efficiency of your code.
That’s not all: TypeScript is also an in-demand skill in the labor market. According to Hired’s 2020 State of Software Engineers report, TypeScript is the fourth most in-demand coding language in the world. TypeScript is behind Go, Scala, and Ruby. Learning TypeScript should improve your chances of finding a good job in tech.
How to Learn TypeScript Fast
So, you’ve decided to learn TypeScript. That’s a great choice. But, how can you learn TypeScript fast?
To answer this question, we’re going to explore the main topics you’ll need to study to code in TypeScript effectively. Then, we’ll discuss how to learn about those topics. Let’s get started!
Build Your Skills
The first step to learning TypeScript is to master the basics. ll the other components that make up the TypeScript language. Once you’ve learned the basics, you can go on to explore more complicated facets of TypeScript. Let’s break down the top skills that you’ll need to know to learn TypeScript.
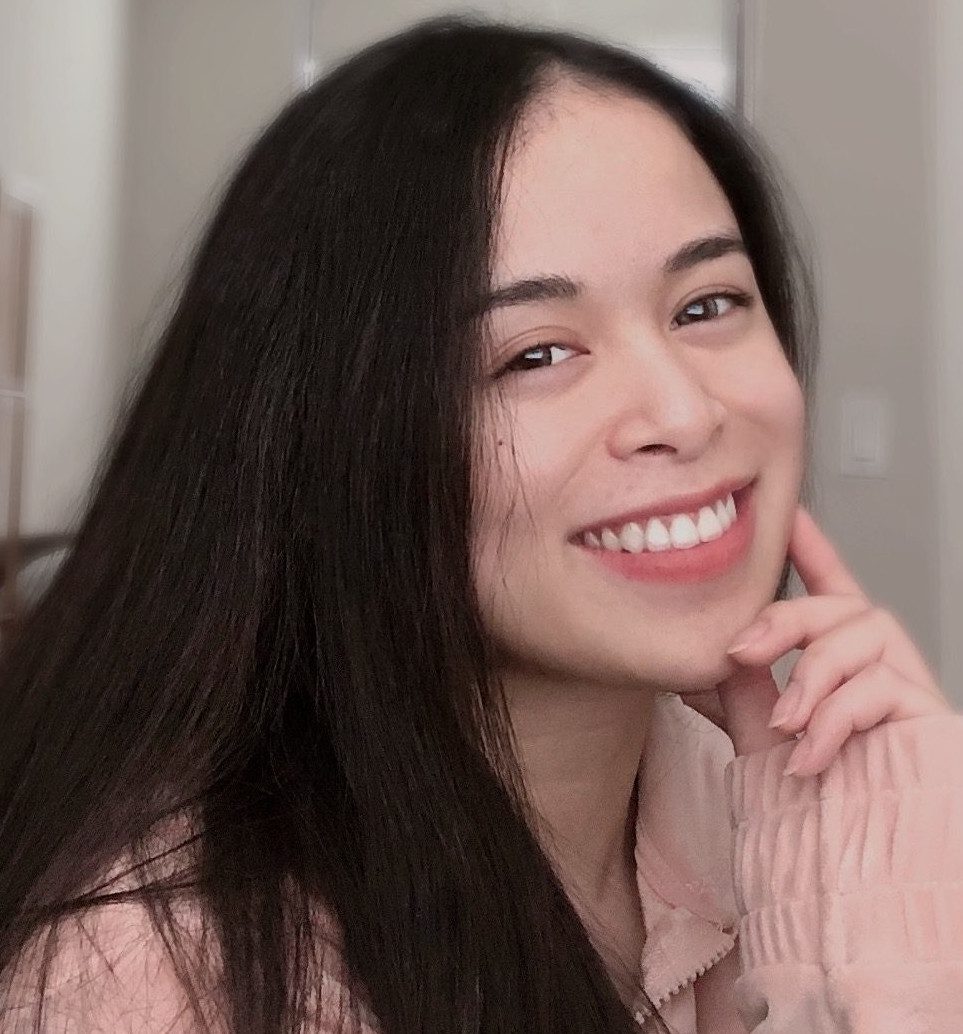
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Learn HTML, CSS, and JavaScript
Because TypeScript is built on top of JavaScript, you’ll need to learn how to code in HTML, CSS, and JavaScript before you get started.
First, you should learn the basics of HTML and CSS. These are the two programming languages used to determine the structure and style of a web page, respectively. Once you’ve learned these technologies, you’ll need to learn the basics of JavaScript.
To get you started, check out our guides on how to learn HTML and CSS:
Types
Types are an essential component of TypeScript, hence the name of the language.
In TypeScript, types can be assigned to variables when they are declared. This allows you to write more reliable and scalable code, and also enhances the readability of your programs.
To work effectively with TypeScript, you should understand the main types of data used by JavaScript. Here are the main topics you should explore:
- JavaScript variable types (i.e. String, number, null)
- any, built-in, and user-defined TypeScript types
- How to assign a type in TypeScript
- How to work with the enum data type
Variables and Commenting
Variables are used to store values in TypeScript. For instance, a variable could store the name of a user or their date of birth.
Comments allow you to add writing to your code which is not read by the browser, but can be read by developers. Comments allow you to explain how your code works.
Because TypeScript and JavaScript variables and comments work differently, you should explore both of these topics in depth. Here are a few topics related to variables and comments that you should explore:
- How to declare a variable using let, const, and var
- TypeScript’s variable syntax rules
- How to write a comment in TypeScript
Functions
TypeScript offers a number of enhancements to traditional JavaScript functions.
In TypeScript, for example, you can declare a function just like you would in JavaScript. But, you can also use TypeScript types as function arguments.
There are two types of functions you should learn about: function expressions and function declarations. You should also familiarize yourself with how the “this” keyword works in TypeScript, which is also derived from JavaScript.
Object-Oriented Programming
TypeScript is an object-oriented programming language, and adds a variety of new features to improve upon JavaScript’s object-oriented features. For instance, you can create classes using a “class” keyword in TypeScript.
You should familiarize yourself with the following topics related to object-oriented programming:
- Classes and objects.
- Inheritance.
- Polymorphism.
- Abstraction.
- How to use TypeScript’s Object, object, and {object} object types.
Interfaces
One of the core features of TypeScript is the interface. Interfaces allow you to define a specific structure for a variable. You should know what an interface can contain and how to define an interface.
Modules
Modules is a feature of TypeScript that allows you to divide your code into many small and reusable components. This can help you keep your project more organized. You should learn about the basic syntax used by TypeScript to import and export modules into files.
We’ve only covered a few of the main features of TypeScript that you can learn about—there’s still so much to explore! By familiarizing yourself with the above mentioned concepts, you’ll be on a good footing as you go on to explore other TypeScript concepts.
How to Learn TypeScript Online
There is a massive body of resources out there to help you learn TypeScript. This is a good thing—the more resources out there, the more likely there is one that meets your exact needs. However, choosing what resources to use can be intimidating.
The first thing you should do before learning TypeScript is ask yourself how you learn best.
Do you like online tutorials, or do you prefer online courses? Do you want to work on projects as part of a team, or are you comfortable learning by yourself? By considering this question upfront, you’ll be able to get a better sense of the types of materials for which you should be looking.
Online TypeScript Courses
Introduction to TypeScript by Udemy
- Cost: Free
- Audience: Beginners
This course is designed to introduce you to the basics of TypeScript. You’ll learn to compile, test, and run TypeScript on your computer. By the end of this course, you will have built a simple app using TypeScript.
TypeScript Fundamentals by Pluralsight
- Cost: Pluralsight membership ($24 / month)
- Audience: Beginners
TypeScript Fundamentals explores the concepts you need to know to write a web application in TypeScript. This course covers topics such as TypeScript syntax, code editors, typing and variables, and modules.
Understand TypeScript (2020) by Udemy
- Cost: $60.00
- Audience: Beginners
This course covers the basics of TypeScript and its main features. You’ll develop a key understanding of why TypeScript is useful and how it can be combined with other web development frameworks. This course comnes with 15 hours of video and 210 downloadable resources.
Best TypeScript Tutorials
The Internet is awash with tutorials to learn anything you could possibly want to learn. Tutorials offer a simple guide to get a specific task done using a specific tool, TypeScript in this case. Below, we examine the best TypeScript tutorials out there for beginners and advanced users as well as the top no-cost options.
Best TypeScript Tutorials for Beginners
TypeScript Tutorials
Check out Tutorials Teacher if you are new to TypeScript and want to learn more. On this website, you can learn everything from the fundamentals to the more advanced features of the language. There are lessons on basic syntax, type annotation, variables, arrays, strings, interfaces, classes, objects, and namespaces, to name a few.
One of the features offered by the site is a “Try It” option that allows you to execute and modify the lines of code online and see the results. To make the most out of this tutorial, it’s best if you have a clear understanding of object-oriented programming (OOP) and basic knowledge of JavaScript.
Complete TypeScript Tutorial for Beginners
Get to learn all the important features of TypeScript in this complete tutorial offered by TekTutorialsHub. Not only does it cover every feature of the language, but it’s also completely free. Like the website listed above, it’s best if you have a basic idea of OOP code and JavaScript.
This tutorial will walk you through key aspects of the programming language, such as syntax and basic rules. It also covers how to install TypeScript and set up the development environment. If you complete every tutorial, you will understand variable declarations, type annotations, type interfaces, string data types, numbers, BigInt, special and advanced types, and even TypeScript operators.
Learn TypeScript: The Complete Course for Beginners
You can access this TypeScript course for free, even if you don’t have an account on Educative. However, if you’ve already used your free access for one of their training courses, you’ll have to pay $34 per year to get this tutorial. This is a text-based tutorial with 114 lessons, which you can complete in about 12 hours.
It starts with the basics and progresses to more advanced concepts like type checking and array manipulation. You will learn fundamental concepts like the basics of variables, generic types, and functions. There’s also a coding environment where you can code and practice what you have learned.
TypeScript Tutorial
Javapoint is another great platform that offers tutorials and online training courses for developers and programmers. Here, you will find an extensive list of TypeScript lessons complete with images and examples.
On this website, you will find plenty of beginner-level lessons, including how to install TypeScript and how to compile and run your first program. The list also includes tutorials on TypeScript types, variables, operators, arrays, unions, strings, classes, enums, inheritance, and more.
How to Code in TypeScript
The best way to get started on coding with TypeScript is to learn the foundation and key features of this language. This tutorial series includes seven lessons that will teach you the syntax you need to get started. Before you begin, make sure to set up the proper environment to execute TypeScript programs so that you can follow along with the examples provided.
This website has tutorials on using basic types, functions, enums, classes, decorators, and interfaces. You will also learn how to create and use custom types in TypeScript. By the end of your learning journey, you will be able to leverage this JavaScript superset to build cleaner code.
Best Advanced Typescript Tutorials
TypeScript Masterclass
This tutorial is focused on more advanced concepts for students who are familiar with the basics of TypeScript. It’s created by Google Developer Expert Todd Motto and is available at Ultimate Courses for $129. It’s straightforward and comprehensive, and you’ll be able to complete the 39 lessons within three hours.
The course delves into arrow functions, type queries, mapped types, type guards, interfaces, generic functions, enums, declaration files, tsconfig, and compiler options. You’ll understand the strengths of TypeScript and learn to write better, more efficient code.
TypeScript: Beginner to Advanced Tutorial Course
KoderHQ is an excellent platform to help you and your entire team go from beginner to expert on a variety of topics through free programming tutorials. The TypeScript tutorial is divided into six sections, making it easier to navigate. If you already know the basics, then you can skip them and go directly to the intermediate-level lessons and learn about OOP programming tutorials or other advanced features such as functions, arrays, tuples, and map collections.
You can level up your TypeScript knowledge further with tutorials on standalone objects, static methods, accessors and mutators, inheritance, and composition. All in all, this is a great resource for both beginners and advanced users of this programming language.
Advanced Typescript Generics Tutorial
If you consider yourself an advanced user of this superset of JavaScript, we recommend checking out web developer Boris Hadzhiev’s tutorial on TypeScript generics. In this short tutorial, Hadzhiev goes straight to the point, addressing first the problem, then the solution, and finally the method.
In this tutorial, you’ll learn how to use generics to narrow down arguments. This guide is full of code snippets you can follow and execute on your TypeScript environment to practice what you are learning.
Exploring Advanced TypeScript Concepts – Guards, Utility Functions, and More
If you’re familiar with the basic TypeScript functions and features, then this tutorial is a great way to take your programming knowledge even further. In this 50-minute video, you will learn advanced TypeScript concepts with senior developer Kelsey Leftwich.
This tutorial includes lessons on TypeScript guards, operators, predicates, assert functions, generic classes, lookup types, utility functions, and conditional types. Leftwich explains what each line of code does and how it works.
TypeScript In-depth
TypeScript In-depth is an intermediate-level course that will help you learn all the major features of TypeScript. It’s divided into ten sections and includes several demos and tutorials that you can complete in about five hours. You’ll have to subscribe to a plan to access the lessons.
The course covers the fundamentals of TypeScript, including basic syntax for variable declarations and enum and array declarations. It then moves on to the other major aspects of the language, such as abstract classes, arrow functions, namespaces, interfaces, modules, and even generics.
Advanced TypeScript
This advanced TypeScript tutorial will build on the fundamentals that you know, allow you to harness its full power, and help you write complete code with little to no error. You will learn about async functions and code, TypeScript module augmentation, custom type guards, destructuring arrays, and other advanced features. You will also learn how to create and use decorators and how to manage async implementation.
Best Free TypeScript Tutorials
TypeScript Tutorial
If you’re looking for the best TypeScript tutorials that don’t require you to pay or subscribe to monthly plans, you’ll find plenty of this on YouTube. One of the videos you might want to check out is TypeScript Tutorial by Mosh Hamedani, a software engineer with 20 years of professional experience. This 51-minute video has nearly half a million views and 8,300 likes.
It’s perfect for novice programmers who want to build applications with Angular and React. TypeScript Tutorial starts with the key concepts of the code language, such as type annotations, arrow functions, interfaces, classes, constructors, access modifiers, modules, and properties.
Learn TypeScript
If you’re looking for interactive courses, then consider enrolling in this free TypeScript tutorial from Codecademy. This 10-hour course will help you learn the critical skills to code faster, reduce errors, and produce higher-quality code.
There is an introduction to types that goes over type inferences, type shapes, and variable type annotations. It covers topics about function declaration, complex data structures like arrays and objects, union types, type narrowing, and advanced object types. If you learn better through quizzes and projects, then you will enjoy this tutorial.
TypeScript Tutorial
The Net Ninja’s TypeScript Tutorial is another set of YouTube videos worth checking out. With more than half a million views and 20,000 likes, this playlist is one of the most popular free TypeScript tutorials on the platform.
This tutorial series is an introduction to the coding language and covers its features and workflows, among other topics. It includes lessons on TypeScript basics, objects, arrays, and classes. This tutorial also delves into more advanced features like generics and interfaces.
TypeScript Tutorial For Beginners
Joining a developer community like DEV is a great way to connect with other professionals. This is a great place to find courses for developers and tutorials on OOP languages to help you write cleaner code. TypeScript Tutorial For Beginners, created and shared by Valentino Gagliardi, is one of the best TypeScript tutorials here.
This four-part free tutorial covers everything you need to know to get started with TypeScript and write functional code to improve your developer experience. It includes 17 lessons that will introduce you to fundamental concepts like TypeScript types. You will also learn how to configure the TypeScript compiler, extend an interface, and even return types for functions.
TypeScript Tutorial
TypeScript Tutorial is another excellent go-to resource for anyone who wants to learn how to create cleaner code and avoid errors. This free tutorial is divided into ten sections where you will learn about TypeScript, its benefits, features, and applications.
This tutorial includes lectures on basic types, control flow statements, functions, classes, interfaces, advanced types, generics, and modules. You will also learn how to use the TypeScript code language in a Node.js project file.
Online TypeScript Books
Programming TypeScript by Boris Cherny
Programming TypeScript is a comprehensive resource on TypeScript. You’ll learn about the basics of TypeScript and its advantages. Then, you’ll cover more advanced topics like integrating TypeScript with other frameworks.
Essential TypeScript: From Beginner to Pro by Adam Freeman
In this book, Adam Freeman starts by talking about why TypeScript is useful. Then, you’ll quickly go on to explore all the main features of TypeScript.
By the end of reading this book, you’ll have develoepd a good understanding of how to use TypeScript for both client- and server- side development.
Pro TypeScript by Steve Fenton
This book by Steve Fenton covers topics like how the TypeScript type system, using modules, and integrating existing frameworks into your programming. If you’re looking for a solid primer on TypeScript, this book is for you.
Online TypeScript Resources
TypeScript Deep Dive
TypeScript Deep Dive is an online guide to TypeScript. In this guide, you’ll cover everything from JSX to errors in TypeScript. This guide comes with primers on many essential JavaScript concepts so that you don’t have any gaps in your knowledge before you start learning TypeScript.
Awesome TypeScript
Awesome TypeScript is a community-maintained GitHub repository with top resources for learning TypeScript. You’ll find development playgrounds, tutorials, and starter projects you can use to kickstart your journey to learning TypeScript.
Learn TypeScript Documentation
The TypeScript documentation is perhaps the best place to go for a solid introduction to TypeScript features.
The language’s documentation is frequently updated by Microsoft and the developer community, which means that everything you read should be comprehensive and up-to-date. What you read here is written by someone with intimate knowledge of how TypeScript works.
TypeScript’s documentation also includes a few code samples from which you can work. You’ll also find comprehensive hands-on playground tutorials that are great for beginners.
Attend a Coding Bootcamp
Coding boot camps are short, employment-focused career training programs. At boot camp, you’ll learn all the practical coding skills you need to pursue a career in technology.
There are dozens of coding boot camps that specialize in web development, many of which teach JavaScript and TypeScript as part of their curricula. To find out more about coding boot camps for web developers, check out the Career Karma boot camp directory.
Build a Project
Once you’ve learned the basics of TypeScript, you’ll be ready to start a project.
Projects are a good way to learn because they allow you to apply the skills you have learned. Keep this in mind—if you don’t apply your skills, you’ll forget them quickly.
To start, try building a few small projects. This will help you become more comfortable working in TypeScript. Building small projects will reduce the chance that you give up on a project because you feel overwhelmed. In fact, many online courses offer small, structured projects as part of their curriculum.
Once you’ve built a few small projects, you can scale up your efforts and work on a larger project.
Are you looking for ideas on what to build? Here are a few suggestions:
- A site that lists information on various types of chocolate
- A site to discover music from Spotify, using the Spotify API
- A portfolio website or a blog
- A weather application
- A clone of Hacker News
- A news site aggregator
- A site that helps you plan out your weekly meals
- A note-taking platform
Once you start working on a project, you’ll find that it becomes easier to continue coding. This is because you’ll have a clear goal in mind: to finish your project. When you are just getting started learning any coding technology, this mindset is incredibly valuable.
Practice Your TypeScript Skills
Practice may not make perfect, but it certainly makes you a better coder.
Coding is a skill, and like any skill, you need to practice. It’s impossible to become a good programmer by just following tutorials and reading documentation.
Practice is so important because, as you code, you’ll encounter new issues and features that you have not thought about before.
All of this work will contribute to your understanding of TypeScript and programming more broadly.
If you can, practice every day. If you’re looking for a few ideas on how to practice your skills, consider the following activities:
- Try out a coding challenge. Platforms like Codewars and Coderbyte host coding challenges that you can use to refine your skills.
- Participate in a hackathon. Hackathons are events where developers come together to build projects. Hackathons are typically time-constrained, team-focused events, and so they are a great way to practice your skills in a collaborative environment.
- Sign up to 100 Days of Code. 100 Days of Code is a challenge that encourages you to code every day for 100 days. Committing to this challenge will help stick to your practice.
If you’re still looking for ways to practice, consider starting a new project or improving upon an existing one. The more you practice, the closer you’ll be to reaching your goal of learning how to code in TypeScript.
Join a Developer Community
Because TypeScript is so popular, there is an incredibly active community of developers out there who know how to code using TypeScript.
When you start learning TypeScript, you should try to join one or two developer communities. These communities are places where developers talk about their learning and answer each other’s questions. If you’re not sure where to start, here are a few communities you may want to consider joining:
- Dev.to: Dev.to is a general developer community for people to come together and talk about code. The community has an active TypeScript thread that is great for both beginners and experts.
- StackOverflow: StackOverflow is an asks-and-answers developer community. There are already many threads about TypeScript on StackOverflow, and if you have your own developer question, you can post it on the platform.
- TypeScript Community Chat: The TypeScript community operates a community chat on Discord where members can come together and talk.
Start contributing as soon as you can when you join one of these communities. Do you see a question you can answer? Post an answer. Do you think a question needs to be clarified? Ask for clarity. The more you contribute, the better reputation you’ll develop within the community. This will go a long way in helping you find other great developers with whom to speak.
Wrapping Up
TypeScript is a programming language that allows you to write highly-scalable web applications. The language is a super set of JavaScript and provides optional static type-checking.
TypeScript’s tagline is “JavaScript that scales.” This is for a good reason. TypeScript lets you write highly efficient code that runs well as your application grows.
There are a wide range of free online TypeScript resources you can use to master the technology. This is in large part due to the growth in demand for TypeScript developers.
To summarize, here are the main steps you should follow to learn TypeScript fast:
- Find learning resources
- Master the fundamentals
- Build a project
- Practice and refine your skills
- Join a developer community
If you follow these steps, you’ll be on your way to becoming a TypeScript expert! With the skills you learn, you’ll be in a great position to start writing more efficient web applications. You may even be able to apply your newly-learned skills to help you find a job in tech.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.