Over the last few years, React.js has emerged as a popular framework used to develop efficient web applications. If you’ve spent any time working with JavaScript, it is likely that you have heard the word React come up at some point.
React.js, developed by Facebook, is a JavaScript library for building user interfaces. React makes it easy for developers to create aesthetically pleasing and interactive user experiences for websites.
According to Stack Overflow’s 2019 Developer Survey, React.js is the most loved web framework favored by developers. React is so popular that sites like Facebook, Codecademy, and the New York Times are all built using the React framework.
This guide will discuss the best way to learn how to code using React online. We’ll walk through what you need to know to start your journey to learning React.
What is JavaScript?
Before we discuss React, we need to talk about two concepts: front end development and JavaScript.
There are two main components to a website—front end and back end. The front end is what the user sees on a site. The back-end is what the users don’t see, such as the scripts that process forms on a site.
Back end and front end code comes together to build a website. Alongside HTML and CSS, JavaScript is a crucial technology used for front end web development and allows you to create dynamic web content.
What is React?
React.js is an open-source JavaScript library. It is used to create user interfaces and components for a web page. Facebook and the open source community maintains React.js.
You can bundle up parts of a website into components with React, which are small building blocks for your application. This component-based approach to coding allows you to reduce repetition in your code and improve the speed of a web application.
The React library is perhaps the most popular JavaScript library in the world as a result of its ease of use. React is by companies ranging from Facebook to Netflix and Slack.
React uses JSX (which is short for JavaScript Extension) that makes it easy to write an efficient web using a syntax that resembles HTML. Second, React offers a feature called Virtual DOM, which, without going into too much detail, makes websites load faster.
Should You Study React?
Learning how to code in React.js is a great investment of your time for any aspiring front-end web developer. But why is this the case?
First, a wide range of huge organizations use React, from the New York Times to Facebook. If you know how to code in React, you’ll have a great skill that you can list on your resume.
Second, React makes it easier to develop modern web applications. React offers features like declarative syntax and a wide range of external libraries that allow you to build powerful web experiences.
As React has become more popular, a growing body of starter resources has emerged. This should make it easier for you to get started.
What is React Used For?
React is used for front-end web development. This is the side of web development that concerns building the part of a website a user sees.
Back-end development, on the other hand, is the “brains” behind a website.
React lets developers create reusable components for the front-end. This brings more structure into the process of writing the front-end of a website and helps to cut down on code repetition.
How Long Does it Take to Learn ReactJS?
In short, becoming proficient with the basics of React will take you between one and six months. The exact time to master React depends on your software development experience and the time you are willing to dedicate toward learning.
If you spend 10 hours a week learning React, you’ll learn how to use the library much quicker than you would if you spent less time learning. If you spend two hours per day learning React, you should be able to master the fundamentals within a few months.
These time frames do not take into account how long it takes to learn React if you have no programming experience. Because React is a JavaScript framework, the first thing you’ll need to do is master the fundamentals of JavaScript. Then, you’ll be ready to start researching how to code using React.
Is React Hard to Learn?
You should have no trouble learning the basics of React as long as you are familiar with coding in HTML, CSS, and JavaScript.
While React has its own unique ecosystem and development practices, you do not need to be an expert coder to get started. Your knowledge of general web development practices will take you a long way.
The more familiar you are with web development, the easier it will be for you to learn React. It helps if you have experience using another framework, like Angular. This is because you’ll have a better knowledge of how frameworks relate to the broader JavaScript language.
Learn React Fast
Are you convinced that learning React is a good use of your time? Great! There’s one question left to answer: How can you learn React fast?
First, we’ll discuss the main skills you need to know about when it comes to React. Then, we’ll talk about how you can actually acquire those skills.
Build Your Skills
Learning how to code using React.js is a bit different from learning a programming language. This is because, unlike technologies like CSS or HTML, React is a library that is part of another programming language: JavaScript.
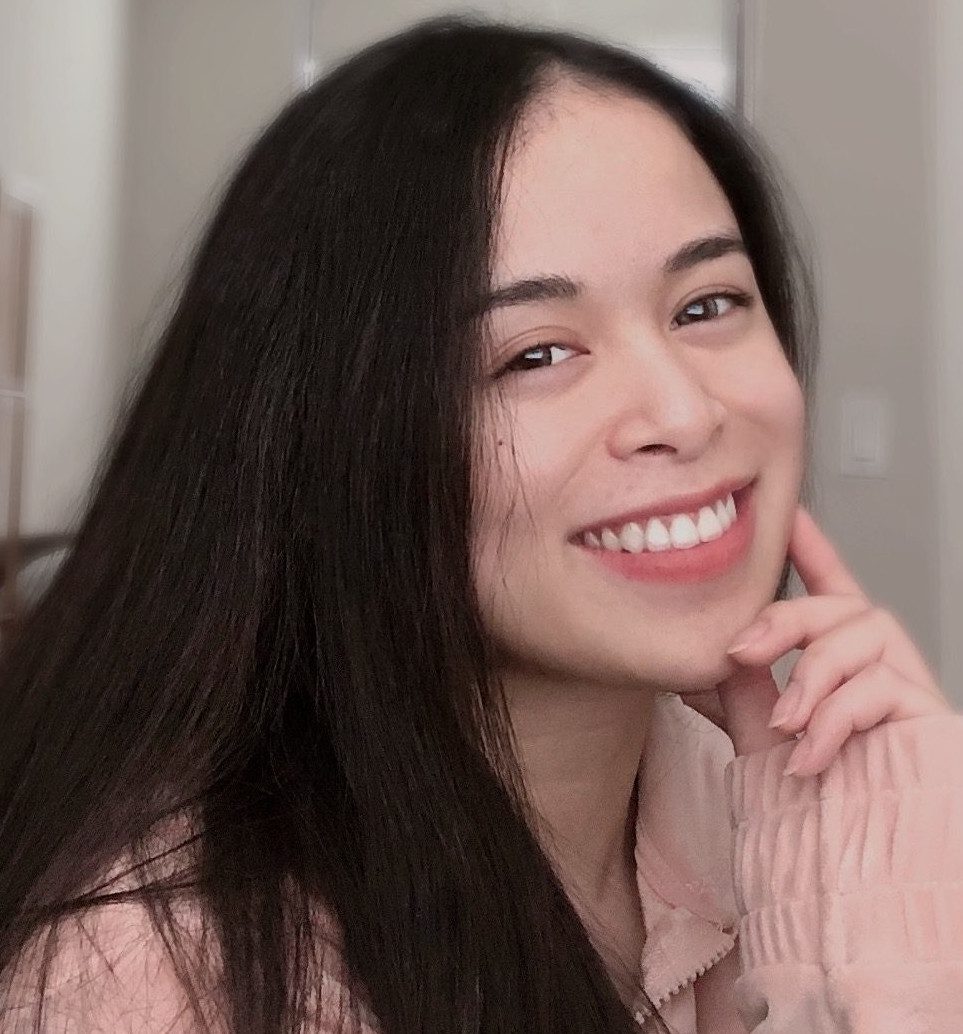
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
To help you on your journey, we have compiled a list of the top skills you’ll need to build.
Learn HTML, CSS, and JavaScript
Before you can code using React, you need to learn HTML, CSS, and JavaScript.
While HTML and CSS are not required, the JSX syntax used by React is based on HTML. You’ll need to have a good knowledge of HTML to understand how it works. As for JavaScript, React is built on JavaScript, so there is no escaping learning how to code in JavaScript.
You need to know the basics of JavaScript and have a good knowledge of HTML and CSS to get started with React. Your best bet is to focus on learning JavaScript ES6 or beyond. This will provide you with a good foundation upon which you can build your JavaScript skills. This should be in addition to learning the basics of HTML and CSS.
Here are a few resources you can use to brief yourself on the basics of HTML, CSS, and JavaScript:
- Career Karma’s Introduction to HTML, CSS, and JavaScript guides
- Codecademy’s web development tutorials
- How to Code in JavaScript by DigitalOcean
- W3Schools’ HTML, CSS, and JavaScript tutorials
- JavaScript Basics by Mozilla
JSX
JSX, or JavaScript Expression, is a syntax extension for JavaScript written for use with React. The JSX technology allows you to describe how a user interface should appear on a website. You should start learning React by exploring how JSX works. Read about how you can use JSX to create elements in a user interface.
Here are a few topics you should explore to further your understanding of JSX:
- What is JSX?
- What is a JSX element?
- How to work with attributes
- Nested and outer JSX elements
- Rendering elements using JSX
- Rendering elements using the React DOM
- The Virtual DOM
Routing
Routing, in plain English, shows how users will move throughout your website. It’s a tool that links a URL and your application. Consider this example. You’re on your homepage, and you’ve clicked and navigated to your bio page. This is routing in action.
React Router allows us to build a single page web application without the page refreshing as the user navigates. Router uses a component structure to call components, which holds and displays the appropriate information.
Components
Components are the building blocks upon which all React JS-powered applications are built. All web pages that use React use components to declare each part of the site. For instance, a navigation bar may be a component, or an article on a web page may be a component. In turn, the article component could consist of a comment component or a text component.
Components allow you to develop user interfaces that are easier to maintain and load quickly. Here are the main topics you should master when it comes to React components:
- How to create a component class
- How to render a component
- How to create an instance of a component
- How do components interact
- How to use components in a render function
- How to reference components from another file
- Controlled and uncontrolled components
Rendering Lists
Lists are an important part of any website. Building a list in React is a great way to familiarize yourself with the fundamentals of coding in React. As you start to learn about React, you should spend time learning about how you can render lists of data on a web page.
Managing States and Props
The state refers to how each object on a web page appears. Every component in a React app can have its own state, which is part of an object called this.
Props, on the other hand, are used to share code between components. To work with React, you need to have a good understanding of both states and props. Here are the main topics you should learn about:
- What is the React state?
- thiskeyword
- How to use props in React
- PropTypes
Redux
Redux is a JavaScript library that makes it easier to manage the “state” of your application. Think of state in terms of what you’ve learned about components at this point.
The state of a component is like the props which are passed to a component–like a plain JavaScript object. This object contains information that influences the way that a component is rendered.
Did that confuse you? Another way of looking at Redux is that it helps you manage the data you display and how you respond to user actions. Redux makes state management much simpler; however, there is a bit of a learning curve when getting started.
Next Steps
We have only scratched the surface of React JS concepts. Because the library is so popular, there are frequent updates made available. These updates continue to add in even more features to make using React a more pleasurable experience.
The topics we have covered above will give you a good sense of the foundational React skills. Once you’ve mastered these topics, you can go on to explore more advanced React ecosystem concepts:
- Higher-order components
- React context
- React hooks
- React render prop components
- React icons
- React forms
- React Native
- Handling API requests using React
As you can see, there’s a lot you can learn once you master the basics! When you know the essentials, these topics will come naturally to you. They are all built on the same fundamental principles used to create the React library.
Best Way to Learn React Free
React has a massive developer community supporting the library. This means there is no shortage of resources out there that you can use to learn React. While this is a good thing, it also means that it can be difficult to find the resources that are worth your time.
Before you start learning React, ask yourself: How do you learn best? Do you like tutorials? Or do you prefer online videos? Do you like working on interactive projects, or prefer to work through theory then build your own projects? By answering this question upfront, you’ll be able to refine your focus as you look for learning materials.
To help you get started, here are a few places you can go to learn React:
Online React Resources
Learn React Documentation
The official React documentation is one of the best sources of content when it comes to learning React. The React documentation is updated with every change to the library. This means that everything you read on the site should reflect the latest version of React.
The React documentation was written by the core React team and a community of passionate React developers. It is well regarded as a high-quality source of content.
Bcause the documentation was written by the React team, there are no commercial interests you have to worry about. The documentation was written solely to help people better understand React. You can find the official React documentation here.
React Resources
React Resources is a directory of resources for people who work with React. You’ll find plenty of guides to assist you along the way, divided into categories such as podcasts, books, and talks.
Getting Started with React
This guide by freeCodeCamp covers the fundamentals of React. You’ll learn to build a small project that retrieves data from an API throughout following this guide.
Other Resources
Aside from the official Intro to React tutorial, there are plenty of great guides and tutorials out there you can use to learn React. Using tutorials to learn React is a great way to refine your skills because authors usually elaborate on their thinking step-by-step.
This means that you can easily walk your way through what is being discussed. If you need to, you can always go back to an earlier point in the tutorial if you get stuck.
Here are a few of the top tutorials and guides out there for React:
Online React Courses
Another great way to learn React is to follow along with online courses. Online courses are like a class you would attend in college. But, you get to control the pace at which you go through the course. And, you can always rewind a video if you get stuck.
React Starter Kit
React Starter Kit is a free, five-part course that introduces you to React. This course is accompanied by code snippets that illustrate the concepts covered in the course.
Codecademy: React 101
Codecademy has an online course covering React development. You’ll learn about JSX, lifecycle methods, hooks, and everything else you need to know to build a web application using React.
Scrimba: Learn React for Free
Learn React for Free is an online course with 48 video tutorials. You’ll cover topics like the ReactDOM and conditional rendering in depth.
If you’re looking for more courses, check out the official React Community course list.
React Tutorials
There are a good number of React tutorials online that cater to any aspiring React developer. Besides React courses and coding challenges to help you practice, tutorials usually hone your React development skills with step-by-step instructions or processes. If you want to get better at front end development, learning the fundamental concepts of React through all available means could give you an advantage.
Advanced learners are also in luck because there are plenty of YouTube channels and websites that offer know-how on more difficult React topics. Some of these tutorials use popular websites or mobile applications to teach these concepts by breaking the elements down. In other instances, you might need to create your own application alongside the developer in the tutorial to practice your skills in real time.
Most of these tutorials are freely available on the web, so you cannot expect a certificate of completion from any of them. Nonetheless, seasoned professionals and software developers conduct these tutorials. You will also get the chance to work on real-world projects as part of the learning process.
Best React Tutorials for Beginners
React JS – React Tutorial for Beginners
Learn the core concepts of React JS through this two-and-a-half-hour tutorial by Mosh Hamedani. The first part introduces you to React and how to set up a development environment, which you will need to make sure that there are no interruptions while working on your project.
The tutorial focuses on basic concepts such as expressions, attributes, classes, and lists. You will also learn how to debug React applications when you run into problems, which will strengthen your resolution skills.
Learn React JS – Full Course for Beginners – Tutorial 2019
This in-depth five-hour React tutorial by freeCodeCamp starts with an introduction to React and the philosophy behind it. As you learn more about the core concepts of React JS, the tutorial expects you to build your own dynamic web application. There is an in-depth discussion on how to design your React application together with CSS classes and how to generate or brainstorm more projects and ideas to practice your skills.
React Crash Course for Beginners 2021 – Learn ReactJS from Scratch in this 100% Free Tutorial!
Maximilian Schwarzmüller offers a crash course for beginners that focuses on building an application with React JS. The tutorial emphasizes the importance of components because this will determine what the application looks like on-screen.
If you are an aspiring front end developer, you will gain a basic understanding of styling using CSS classes through this tutorial. For example, you will learn how to prepare your application for the web and ultimately deploy it for use.
React Tutorial for Beginners
If you are looking for a shorter tutorial, this 43-minute video for beginners covers the basics of building an application through React JS. The tutorial starts with instructions on installing React, then provides an in-depth discussion of components, and mainly focuses on writing code with React hook. It also teaches you how to pass props, which is essential when sending data from one component to another and required for any application to work optimally.
React JS Course for Beginners – 2021 Tutorial
Another tutorial by freeCodeCamp, this video is available for free and runs for more than seven hours. This tutorial is ideal if you want to have a strong understanding of React and full stack web development.
The tutorial drills deep into components, props, and states. There are also sections on how to use React with Bootstrap, which is helpful for mobile app development to achieve a dynamic interface regardless of screen size.
Best Advanced React Tutorials
Advanced React Patterns, Performance, Environment, and Testing
Gain a deep understanding of advanced React concepts through this hour-long video and learn how to create stylish and functional websites using React, CSS, APIs, and more. The tutorial mainly focuses on compound components, an advanced React pattern to achieve flexible APIs and have reliable server connections. You will also learn how to test the performance of your React app to deal with issues encountered while building and debugging.
Junior vs. Senior Code – How to Write Better Code as a Web Developer – React
This short tutorial video delves into the contrast between a junior developer and a senior developer to improve your React coding skills. You will gain insights on how senior full stack developers think and grow more comfortable with state and effect in React. More importantly, this tutorial conditions your mind to think like a programmer, so you will have a well-rounded understanding of React and be able to plan out your future projects better.
Advanced React Tutorials
Create dynamic user interfaces, optimal mobile applications, and web pages through this series of advanced React tutorials. The tutorials discuss React hooks and how to create your app without classes to easily reuse certain components for other functions without modifying others.
There is also a wide range of React programming concepts such as pure components, refs, TypeScript, and DOM. These topics are discussed thoroughly through several videos, which will help you to build better web or app interfaces.
Full React Course 2020 – Learn Fundamentals, Hooks, Context API, React Router, Custom Hooks
A 10-hour full React tutorial is available for free on YouTube by freeCodeCamp if you want to build your knowledge on essential concepts to advanced techniques in React JS. Through React hooks, CSS, and other elements, the goal is to help you master building a dynamic user interface for web and mobile platforms. This comprehensive tutorial also goes deep into React Router.
React Advanced Concepts | Every React Developer Should Know
This series of tutorials is ideal for any aspiring React developer wanting to master advanced React concepts. It deals with topics such as pure and higher-order components, as well as refs and forwarding, which enable components to connect with one another. There is also a dedicated tutorial for error boundaries, which is essential to identifying and addressing errors in components to prevent running into problems.
Best Free React Tutorials
React Website Tutorial – Beginner React JS Project Fully Responsive
Learn more about full stack development with React JS through this tutorial on creating a website from scratch. This tutorial will guide you on how to get started with website development using React, highlighting the importance of components and how to create pages. There is also a section discussing how to replace a video background with an image using React. Additionally, it covers how to do an overall polishing of your site or application.
Full React Native Project Tutorial for Beginners
This free tutorial on React Native helps beginners learn how to build Android and iOS applications. Since this tutorial does not deal with single-page applications, it runs for 10 hours as it covers how to create functionalities like user login or password options. The last few sections are about achieving a responsive layout and how to optimize your application depending on the screen size of your device.
React JS Crash Course 2021
React JS Crash Course 2021 is another free tutorial on YouTube where you will learn about components, hooks, state, and props in under two hours. The goal is to help you get started by building a task tracker app, so this tutorial will also teach you how to utilize JSX and JSON servers. Finally, it also focuses on APIs as the task tracker app will need to connect with a server for adding and deleting functionality.
Full Stack React & Firebase Tutorial – Build a Social Media App
This 12-hour course is a comprehensive tutorial on building a social media app using React and Firebase, which is a reliable platform to develop applications smoothly. This tutorial is project-focused and will teach you how to enable essential social media app functions such as registration and uploading your image.
You will also learn how to add user details and how to like and unlike a post. Once you have completed the entire process of building the app, the last part of the tutorial provides a step-by-step guide on deploying your application to Firebase.
React JS Tutorial for Beginners – Full Course in 12 Hours [2021]
Learn React and JavaScript through this 12-hour beginner tutorial where you break down and study its different elements through popular media applications. This tutorial focuses on Netflix, Spotify, Slack, and TikTok and covers what makes each one of these applications unique. This approach will help you create your own website or application and improve your web development skills by understanding popular apps.
Online React Books
There are plenty of books out there that will help you master ReactJS.
The Road to Learn React by Robin Wieruch
The Road to Learn React guides you throught he fundamentals of React. By the end of reading this book, you’ll have created a real-world project using React that demonstrates your new skills. The book comes with exercises to help you reinforce what you learn.
Learning React by Alex Banks and Eve Porcello
Learning React shows you how to create user interfaces using React and ECMAScript. You’ll cover topics like functional programming, how React works, and how to use routing to direct users around a web application.
Pure React by Dave Ceddia
This book focused on vanilla React. This is React without relying on any external libraries. Pure React was written for beginners who want to master the fundamentals of React. You’ll focus solely on React and not adjacent tools like Next.js or Apollo which are commonly used with React.
How to Get Set Up to Learn React Free
How do you actually start coding using React? That’s a great question. Here are a few tools you may want to set up in order to start working with React:
- Install NPM. NPM (Node Package Manager) is a site that allows you to install libraries into your React project. You’ll need to use NPM to access external libraries and to install React itself.
- Choose an IDE. IDEs are the development environments in which developers build applications. For React developers, Visual Studio Code (VS Code) is a popular choice, alongside Atom.
- Use Prettier. Quickly, your React code can become messy. That’s why it is handy to have a tool like Prettier at hand, which can format your code in your development environment. Prettier has a custom React feature you can use to format your React code.
- Try out create-react-app. create-react-app is a boilerplate that gives you everything you need to start a new React app. Many tutorials recommend that you start from the create-react-app project because all the basics are configured.
Practice Your React Skills
Building projects are so effective at helping you learn to code because it is a form of practice. When you are building a project, you cannot learn passively by watching a tutorial. You need to be in the weeds and focus all your attention on what you are building.
Practice is so important when you’re programming because many of the issues you will encounter will come up again and again. By doing more coding, you’ll be able to build up a clear list of errors in your mind. You can use this knowledge to resolve those mistakes more effectively in the future.
Practice every day is a great mantra to use. Try to code as much as you possibly can. If you’re looking for ways to get in more practice, here are a few suggestions:
- Work toward a project. Build a project that you are passionate about, then share it with the world to get feedback.
- Participate in coding challenges. Sign up to a platform like Codewars, which hosts a wide range of coding challenges that you can use to refine your skills.
- Sign up to 100 Days of Code. 100 Days of Code is a challenge where you publicly commit to writing code for 100 days in a row. This is a great way to stay committed to coding and to hold yourself accountable.
It’s simple—the more you practice, the better you’ll get.
Learn React in Public and Get Feedback
Learning in public introduces a new accountability mechanism in your learning. When you commit to learning something in public, you can’t easily back out. There will be people who are following your progress and who will root to see you succeed.
When you’re learning React, you should try to be vocal about your intentions, and talk widely about the goals you have set for yourself.
Once you’ve met a goal, you should try to get feedback on your work from other developers. This will give you an opportunity to hear suggestions on how you can improve from people who are more experienced at using React.
Join a React Community
If you’re new to coding or are learning to code on the site, you may not yet know any—or many—other developers. But, there is an easy way to find other people with whom to talk by joining a developer community.
Developer communities are places where you can go to talk about code, share your ideas, and collaborate on projects. Here are a few great communities for React developers that you should consider joining (if you haven’t already):
- StackOverflow: StackOverflow is a popular asks-and-answers site used by developers. There are plenty of existing threads on there about common React topics.
- Dev.to: Dev.to has an active community of React developers who come together and chat about the latest in React.
- Spectrum’s React community: Spectrum’s React community is a great place to meet other React developers and discuss coding issues.
Upon joining a community, you should start to look for ways you can add value. Do you know the answer to a question someone has? Answer it. Do you need help with a question? Post it on the site so anyone else with that problem has a place to go. The more you contribute, the easier it will be to develop connections in the community.
Wrapping Up
React.js is an established web technology that allows you to painlessly build interactive user interfaces.
The React library is based on a “learn once, write anywhere” principle. This means that when you master the basics, you should be able to easily reuse your code across a wide range of contexts.
In this guide, we laid out a few main steps you should follow to learn React fast. They were:
- Find learning resources
- Master the basics
- Learn by doing
- Practice your skills
- Join a developer community
By following all these steps, you’ll be on a good path to becoming a React master! Once you’ve learned React, there is no limit to the projects you can build. If you are interested in becoming a professional web developer, React is a great skill to have in your tool belt.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.