Have you ever wondered how Android applications work? How are the apps that help you in your day-to-day activities created? There certainly is a whole ecosystem of frameworks and languages to help create these apps, but which one is recommended by the creators of Android themselves? The answer to these questions is Kotlin — the latest addition to the Android application development frameworks available in the market, and directly backed by Google themselves.
How to Learn Kotlin
Kotlin is a powerful programming language to write mobile applications in, so it is important to understand its purpose and features before we dive into learning its dynamics.
What is Kotlin?
Kotlin is an open-source programming language, specifically designed with mobile application development in mind. It offers a competitive advantage over the traditional language for Android — Java — in aspects like code readability and cross-platform application development. Kotlin, outside of mobile application development, is a full-fledged, object-oriented programming language.
Kotlin is highly recommended by Google due to the number of benefits it offers over Java. Kotlin’s syntax is similar to Python’s, making it simple to learn and use. With the increasing community support, people are writing data analysis libraries in Kotlin, which hints at Kotlin becoming the next big thing in machine learning in the coming times.
Kotlin also finds use in web application development. The ability to compile Kotlin code to a JavaScript equivalent opens a world of cross-platform possibilities for Kotlin. This means that you only need to write your code once in Kotlin, and you can create working mobile, web, and desktop apps for your product. Before we dive into these use-cases in detail, let us take a moment to understand the advantages of using Kotlin over its counterparts.
Features of Kotlin
Kotlin offers multiple features as a programming language. Here are some of them:
Concise Code
One of the primary reasons why Java is known to have a steep learning curve is the boilerplate that it brings. And when it comes to Android applications, Java adds even more boilerplate code in every project. Kotlin aims to solve this issue with simple syntax and easy to understand notations.
A glimpse of this can be seen by comparing the traditional “Hello World” program in the two languages:
Java:
class Test { public static void main { System.out.println("Hello World!"); } }
Kotlin:
fun main() { println("Hello World!") }
Kotlin takes away the load of always declaring a class to write code, by taking the main function to the top-most level. It also simplifies the print call, and this is a fair representation of other simplifications implemented throughout the language.
Improved Null Safety
Null pointers are one of the most common issues faced by Java developers. They take up a lot of time and cause unneeded frustration. In many cases, this happens due to a reference variable not being checked for null before being accessed.
In Java, this is traditionally done by putting an if case before accessing the variable, like this:
// let foo be a variable if (foo != null) { foo.bar(); }
This code can cause errors in the application if missed out. Kotlin nicely addresses this issue, and the Kotlin compiler by default does not allow any variables to have a value of null at compile time. So if you are missing a null check somewhere in your app, or operating on a null variable, you will be notified at the compiler time itself.
This does not let null-pointer bugs reach the final application. Alternatively, you can implement safe method calls for yourself. If you were to write the above piece of code with Kotlin’s safe call, this is how it would look:
// let foo be a variable foo?.bar()
This code will call the bar method on the foo object if foo is not null, or return null if foo is null. This lets you easily make complex, chained calls like these:
baz?.foo?.bar()
Interoperability with Java
Kotlin compiles to Java bytecode by default. This makes it simple to use precompiled Java classes with Kotlin code. You can write an application using Java as well as Kotlin, and it will all compile to Java bytecode, which will be able to interoperate within the Kotlin and Java parts freely. This makes the migration of huge, legacy Java applications to Kotlins smooth, as not all code needs to be migrated at once.
Apart from that, smart IDEs like the Android Studio are capable enough of converting Java source code into Kotlin on the fly. This means you can simply convert your old Java classes into Kotlin classes using nothing more than your IDE.
Converting (Transpiling) to JavaScript
Kotlin also offers you the option of transpiling your code to JavaScript. Transpilation simply means converting a source code written in one language to that written in another. Kotlin allows you to write apps that can run on web and desktop platforms.
According to the current official documentation, the current version of Kotlin targets ES5.1 for JavaScript environments, which means that it can not benefit from some of the major improvements that were shipped with ES6. Also, it currently supports converting only Kotlin code to JavaScript equivalent, and anything present in a project that is not written in Kotlin does not get transpiled to JavaScript. This leaves a lot of room for improvement.
Native Conversion with Kotlin Native
Applications written in Java-based languages require a virtual machine to run. All Java applications require a Java Virtual Machine (JVM) to be installed on the target host. While the current version of Kotlin requires the same too, a technology called Kotlin Native is being developed to free the applications of this restriction.
Kotlin Native aims at compiling code to native executables, rather than bytecode. These executables can then be run without a virtual machine. This improves performance as the system does not need to run a virtual machine to execute a Kotlin application.
What is Kotlin Used for?
Kotlin is growing as the primary language for native Android application development. Before we set down to learn the library, let’s explore some of its main use cases:
For Creating Mobile Applications
Java was the traditional language for writing mobile applications before Kotlin because the Android framework supported a JVM (Java Virtual Machine) for natively running applications. However, writing code in Java is not the best experience for developers and the Android framework adds more boilerplate to projects.
The primary reason why Kotlin was created was to easily write native Android applications. Kotlin does this job well by simplifying the traditional Java syntax, as well as reducing the usual boilerplate in Java.
For Building Backends
Kotlin is growing as a strong alternative for server-side development. As mentioned previously, Kotlin works in all those cases where Java would normally work, as both languages compile down to similar bytecode executables, and require the same JVM to execute their binaries.
Additionally, Java libraries can directly be used in Kotlin, so you can interchange your source code’s languages to get the best out of both. The libraries that are not yet written in Kotlin can be used directly via their Java bytecode, and the features of Kotlin can be used to simplify the business logic written in the application.
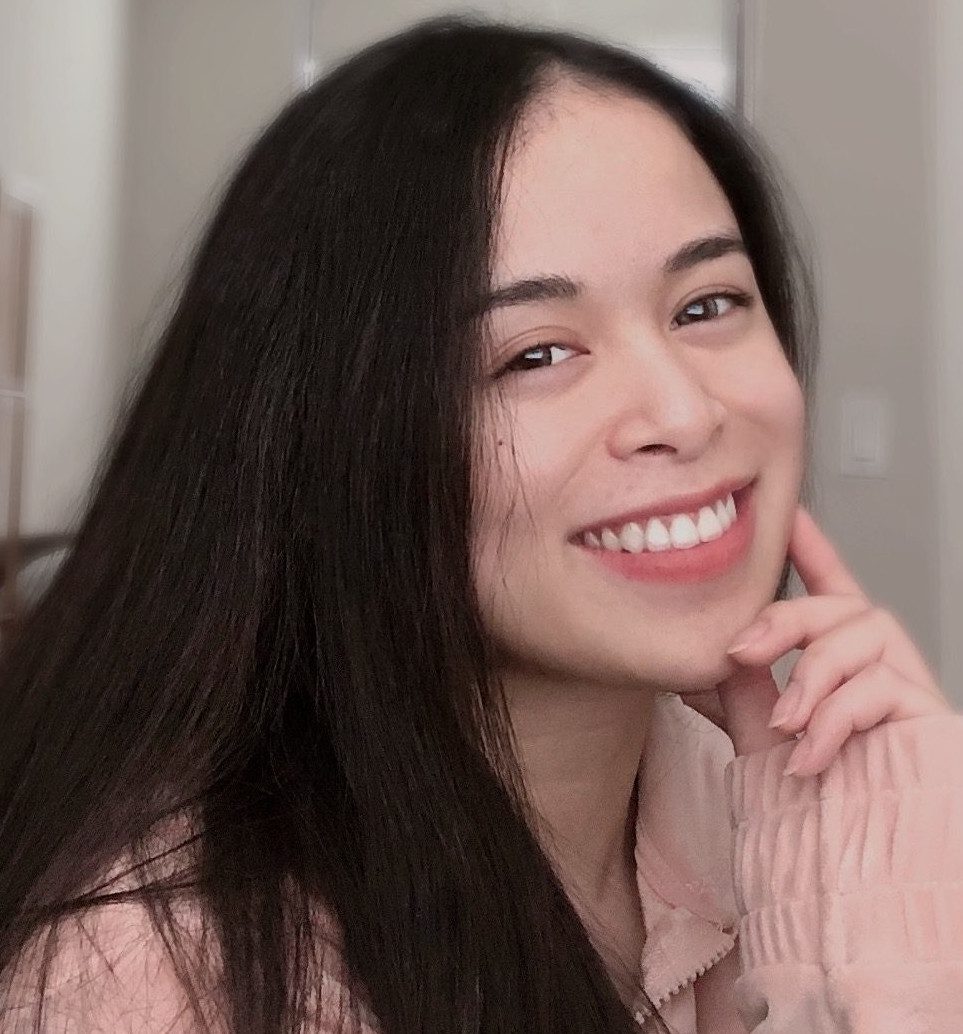
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
For Creating Frontends
Kotlin supports transpilation to JavaScript, and this ability also allows writing applications that can run on web browsers. The Kotlin/JS module facilitates this feature. It provides an interface via the kotlin.js plugin. This simplifies the process of setting up Kotlin projects that aim to build front-end applications in JavaScript.
Using Kotlin, type-safe React applications can be written quite easily. JavaScript technologies like react-redux, react-router and styled-components are supported with Kotlin/JS, and you can easily use them together with other libraries to build your components and libraries.
For writing Gradle plugins
A Gradle plugin is a piece of code that defines a part of your build logic, i.e. it allows you to define how your Gradle-based project will be built into a final executable. Gradle plugins can be written in any language that compiles down to JVM bytecode.
Groovy has been the traditional choice for writing Gradle plugins, after Java. Since Kotlin compiles down to JVM bytecode as well, it can be used to write a Gradle plugin. Kotlin provides additional benefits over Groovy like type-safe and concise code.
Learning Kotlin
Given that Kotlin is an advanced programming language with diverse uses, there is plenty to learn. Following is a list of resources to help you get started:
The Best Kotlin Resources
As Kotlin is an open-source programming language, people have tried to create content that can help you easily get started with it. First of all, let’s take a look at the free and paid video courses that are available for Kotlin:
Training Courses by Android Developers
- Platform: Android Developers Website
- Duration: Varies with every course
- Price: Free
- Prerequisites: None
- Start Date: On-Demand
Training Courses is the perfect collection of video courses and labs focused on helping you take your first steps in Kotlin. With a curated list of content from the creators of Android themselves, this collection is bound to be a great resource for a learner of any level. Courses are divided by difficulty as well as topics, so it is easy for you to find one that suits your level of experience.
Kotlin Course – Tutorial for Beginners by freeCodeCamp.org
- Platform: YouTube
- Duration: About 2.5 hours
- Price: Free
- Prerequisites: None
- Start Date: On-Demand
FreeCodeCamp offers the best courses on a variety of technical topics for free. Their Kotlin course requires no prerequisites as it builds an understanding of the language from the ground up. It focuses on the essentials of the Kotlin language and explains every feature in detail. If you couple this with another, more advanced course from the list, you will have the perfect roadmap to learn Kotlin for yourself.
Android Development Course – Build Native Apps with Kotlin Tutorial by freeCodeCamp.org
- Platform: YouTube
- Duration: About 4 hours
- Price: Free
- Prerequisites: Basic understanding of Kotlin syntax
- Start Date: On-Demand
As the name suggests, this course aims at teaching the most popular use of Kotlin: Android development. This course begins with one of the most fundamental concepts of software design — the MVVM Architecture — without wasting any time. This stands as a good measure of the difficulty as well as the aim of the course.
By the time you finish the course, you will have a solid understanding of how Kotlin works behind Android apps, and how production-level Android apps are produced. It is advisable to take a beginner-level course of Kotlin before diving into this course.
Android App Development Masterclass using Kotlin by Tim Buchalka, Jean-Paul Roberts
- Platform: Udemy
- Duration: 62 hours
- Price: About $7 when on discount, about $90 otherwise
- Prerequisites: None
- Start Date: On-Demand
If you are looking for one course that fits all of your Kotlin and Android development needs, this is the right place to end your search. The course has an adequate section on the basics of the Kotlin language, and quickly utilizes this learning with ample projects and follow-up lectures on the basics of Android.
The most remarkable feature of this course is the sufficient number of projects that are scattered throughout the course at appropriate intervals. This ensures that you are putting all your learning to use on the way, and building a strong understanding of the language.
Kotlin Books
Apart from video courses, many books are available to help you get started with Kotlin. Some top ones include:
‘Head First Kotlin: A Brain-Friendly Guide’ by Dawn Griffiths, David Griffiths
Priced at about $41 on Amazon at the time of writing this article, this is one of the best books for taking your first step in the world of Kotlin. The Head First series is known to be the best suited for beginners, as it uses a very friendly tone to explain the toughest of concepts. Head First Kotlin covers the programming language in all its depths, from fundamental syntax to lambdas and higher-order functions.
‘Kotlin Programming: The Big Nerd Ranch Guide’ by Josh Skeen, David Greenhalgh
Priced at about $34 at the moment, this book is a great asset for everyone irrespective of the level of experience one has in programming. This book walks the readers through the basics of the Kotlin programming language through hands-on examples and a clear explanation of key concepts. This book also introduces readers to the IntelliJ IDEA development environment.
‘Kotlin in Action’ by Dmitry Jemerov, Svetlana Isakova
Priced at about $36 at the moment (check the latest price here), this book is one of the best options for people who have a background in Java and are looking to learn Kotlin. This book is the best resource for experienced professionals who are looking to perfect their Kotlin skills. It covers the dynamics of the language apart from the best practices, combination with Java, as well as a primer on DSL.
Kotlin Resources
Apart from the video courses and books, there are also a great number of tutorials available around the internet. Here are some great pieces to begin with:
Tutorials by KotlinLang.org
Offered by the Kotlin organization themselves, the tutorials offer a series of beginner-friendly text and video resources to help you get started with the programming language. The landing page contains links to various ways of beginning with Kotlin, using Koans and the command-line compiler.
Kotlin Tutorial for Beginners: Learn Kotlin Programming by Guru99.com
With ample content on the history, features, setup as well as advanced use-cases, this course offers the perfect roadmap to mastering Kotlin. It is a great place, to begin with, as it offers helpful subtext throughout the tutorial. However, it does not seem practical enough to make you an expert with the given content. If you’re looking for some advanced content, you may want to couple this together with a more advanced course.
Kotlin Tutorial by TutorialsPoint.com
This resource feels more like a glossary of subtopics of Kotlin and less of a guided tutorial for learning. However, the depth of content on each subtopic is great, and this can be an important asset to you once you are done with the basics of the language and are looking for a resource that you can refer to whenever you need help.
How Long Does it Take to Learn Kotlin?
Given that Kotlin is a powerful and detailed programming language, it usually takes up to four weeks to get a good start in it. Rigorous practice, aided with example projects for a duration of another one to two weeks is adequate to make the basics pretty clear in your mind, thereby allowing you to unleash the full potential of this language.
If you are looking to learn Kotlin well enough to build professional applications, you can look forward to two months of regular learning and practice. Anything more than that will only perfect your Kotlin skills.
Should You Study Kotlin?
After compiling a great list of courses and content on Kotlin, we are now faced with the most important question of all: should you learn Kotlin?
The answer to this is simple: if you are willing to dive into the world of software development, or if you have prior experience with Java and are looking to upgrade your experience, Kotlin is the best skill to invest your time and resources into. It offers great ease of programming and varied use-cases, making it the perfect alternative for application development on all platforms.
If you are looking to make a career as an Android developer, Kotlin is one of the basic elements to be able to meet your job requirements. A solid foundation in Kotlin is essential before you set out to explore the dynamics of application development.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.