To initialize a list in Python assign one with square brackets, initialize with the list() function, create an empty list with multiplication, or use a list comprehension. The most common way to declare a list in Python is to use square brackets.
A list is a data structure in Python that contains an ordered sequence of zero or more elements. Lists in Python are mutable, which means they can be changed. They can contain any type of data, like a string or a dictionary.
When you’re starting to work with lists, you may be asking: how do I initialize a list in Python? Or, in other words: how do I create a Python list?
This tutorial will answer that question and break down the ways to initialize a list in Python. We’ll walk through the basics of lists and how you can initialize a list using square bracket notation, list(), list multiplication, and list comprehension.
Python List Refresher
Lists store zero or more elements. Each element in a list is referred to as an item. Lists are often called Python arrays.
In Python, items in a list are separated by commas, and the list itself is enclosed in square brackets. Here’s an example of a list in Python:
jobs = [‘Software Engineer’, ‘Web Developer’, ‘Data Analyst’]
Our list contains three values: Software Engineer, Web Developer, and Data Analyst. We can reference our list by using the variable “jobs” that we declared above.
How to Create a List in Python
You can initialize a list in Python using square brackets, the list() method, list multiplication, and a list comprehension.
Square brackets let you initialize an empty list, or a list which contains some default values. The list() method works in the same way as square brackets. Although you must add square brackets and a list of items inside the list() method if you want to add some initial values.
List multiplication lets you create a new list which repeats elements from an existing list. List comprehensions let you create a new list from the contents of an existing list.
Python Create List: Square Brackets
You can use square brackets or the list() method to initialize an empty list in Python. For the purposes of this tutorial, we’ll focus on how you can use square brackets or the list() method to initialize an empty list.
If you want to create an empty list with no values, there are two ways in which you can declare your list. First, you can declare a list with no values by specifying a set of square brackets without any component values. Here’s an example of this syntax:
jobs = [] print(jobs)
Our code returns: [].
The square brackets with nothing between them represent a blank list.
We can specify some default values by adding them between our square brackets:
jobs = ['Software Engineer','Data Analyst']
We have declared a list object with two initial values: “Software Engineer” and “Data Analyst”.
Python Create List: list() Method
Another way to make an empty list in Python with no values is to use the list() method. Here’s an example of the list() method in action:
jobs = list() print(list)
Our code returns: [].
Both of these first two approaches return the same response: an empty list. There are no standards for when it is best to use either of these approaches. Generally the blank square brackets ([]) approach is used because it is more concise.
Python Declare List: List Multiplication
One approach for initializing a list with multiple values is list multiplication. This approach allows you to create a list with a certain number of predefined values.
So, let’s say we are creating a program that asks us to name our top 10 favorite books. We want to initialize an array that will store the books we enter. We could do so using the following code:
favorite_books = [''] * 10 print(favorite_books)
Our code returns:
['', '', '', '', '', '', '', '', '', '']
Our program has created a list that contains 10 values.
Let’s break down our code. On the first line, we use the multiplication syntax to declare a list with 10 values. The value we use for each item in this list is ‘’, or a blank string. Then, we use the Python print() function to print out our list to the console.
We can use this syntax to initialize a list with any value we want. If we wanted our list to start out with 10 values equal to Choose a book., we could use the following code:
favorite_books = ['Choose a book.'] * 10 print(favorite_books)
When we execute our code, we create a list with 10 values. Each value in the list is equal to Choose a book.. We print this list to the console:
['Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.']
Python Declare List: List Comprehension
In the above section, we showed how to initialize a list using list multiplication. This approach is useful because it allows you to initialize a list with default values.
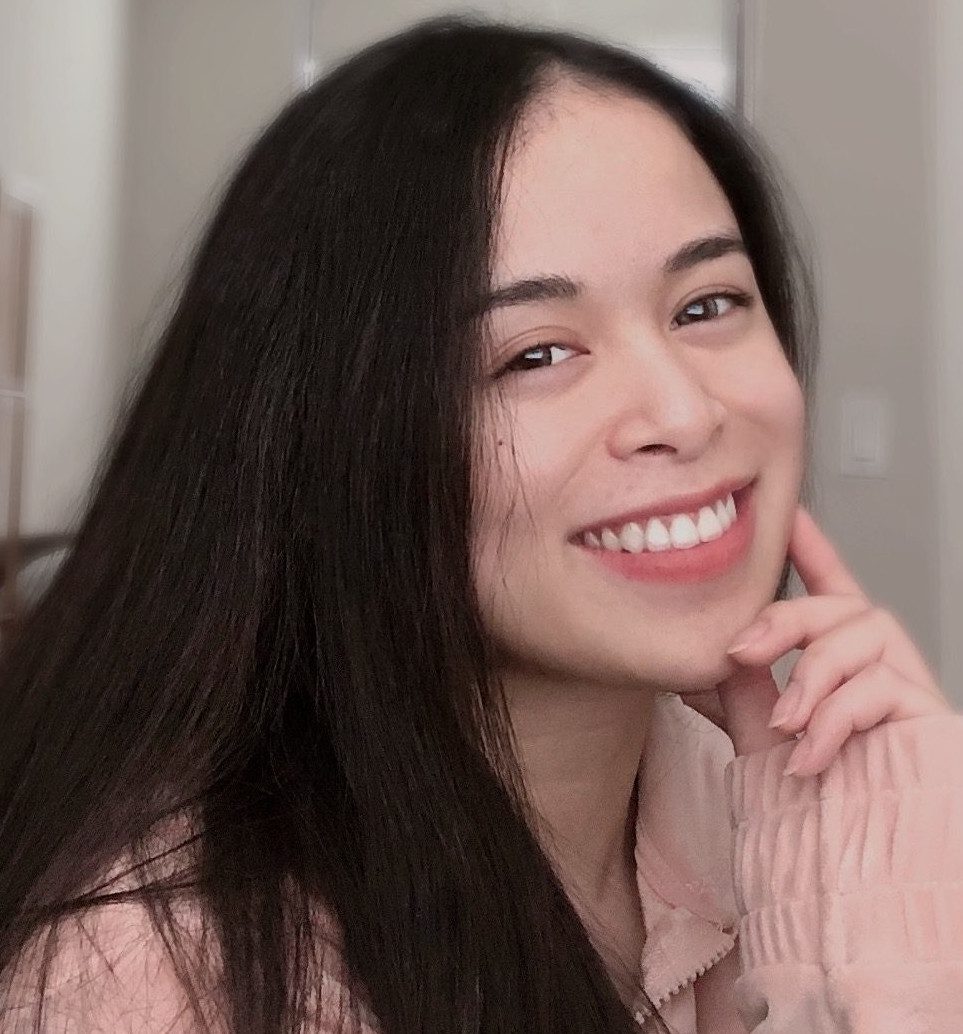
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
You can also initialize a list with default values using the list comprehension method. A Python list comprehension refers to a technique that allows you to create lists using an existing iterable object. The iterable object may be a list or a range() statement, or another type of iterable.
List comprehensions are a useful way to define a list based on an iterator because it is elegant, simple, and widely recognized.
Say that we want to create a list with 10 values equal to Choose a book., like we did in the previous example. We could do this with list comprehension using the following code:
favorite_books = ['Choose a book.' for i in range(10)] print(favorite_books)
Our code returns:
['Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.', 'Choose a book.']
In the above example, we use list comprehension to create a list. The list comprehension statement is the for i in range(10) iterator. This iterator is used to create a list that repeats the value Choose a book. ten times over.
Conclusion
Initializing a list is a core part of working with lists. This tutorial discussed how to initialize empty lists using square brackets and the list() method. It also discussed how to use the list multiplication and list comprehension techniques to create a list containing a certain number of values.
Now you’re ready to start initializing lists in Python like a pro!
Read our How to Learn Python guide for advice on how you can learn more about the Python programming language.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.