With the growth of the internet, interest in APIs has skyrocketed. Application programming interfaces (APIs) let developers build their own tools upon existing platforms. You can use the Spotify API, for instance, to build programs that can use data on your listening habits and the songs you have listened to most.
APIs also help developers write different versions of their platform. With an API, the backend of a service can be standardized. Mobile apps, embedded apps, and web apps can all funnel their requests to the back-end without each having to deal with their own back-end. This reduces repetition across a codebase and helps to maintain consistency.
Two popular methods of serving data over an API are GraphQL and REST. In this guide, we discuss what GraphQL and REST are, the advantages of each technology, and why you may want to opt for GraphQL over REST.
What is REST?
REST, which is short for Representational State Transfer, is a standard method of communicating data on the web. REST divides a web service into two components: the client and the server.
Because these two services are divided, you can modify an application client without impacting the back-end. You just need to communicate the right information.
REST divides an API into endpoints, such as /api/user. These endpoints each have their own functions. One endpoint may display user information. Another endpoint may let a user update their information on a web service.
What is GraphQL?
GraphQL is technically a query language that runs on top of an API. It connects with the back-end code you have already written to provide a new way of communicating data across web services. GraphQL is not a database technology or a back-end in itself.
GraphQL lets you execute queries on a dataset. These GraphQL queries, like a SQL query, can be customized to your exact needs. This architecture means you can retrieve and send only the exact data you need for your application to work.
GraphQL gives developers more control over their data. Using GraphQL, a developer can query specific pieces of data, and bundle multiple queries into one. This makes it easier to see what data an application is consuming and cuts down on querying unnecessary data.
GraphQL is an open-source technology.
GraphQL vs. REST
Both GraphQL and REST are methods of communicating data across web services.
REST is a standard set of software principles that governs how to share data over an API. GraphQL, on the other hand, is a server-side technology that goes on top of an existing API to make data more accessible.
Retrieving Data
GraphQL and REST APIs send data in different ways. In a typical REST API, you may have an endpoint to retrieve an asset:
/posts/:id
This endpoint would return information about a particular post. To retrieve comments for that post, you may need to query another endpoint:
/posts/:id/comments
This means you need to make two web requests instead of one to retrieve the data you need. With GraphQL, this is not a problem. GraphQL lets you send a single query that contains all of the data you need to retrieve form a server:
query { Post(id: "1") { title comments { id body } } }
query {
Let’s read through our query. We are retrieving information from a Post object. This object represents a post on our API. We retrieve the title of the post.
Then, we retrieve a list of the comments that are associated with that post. There is a relationship between “comments” and the Post that we are querying. This query lets us retrieve the title of our post and all the comments in only one query.
Unnecessary Fetching
Have you ever fetched data using an API call and thought to yourself “I don’t need all of this data. I just need one piece. Why do I have to download all this unnecessary data?”
You are not alone. This is a thought that many developers have in their career. This problem arises because REST locks you into specific endpoints. If you want to retrieve additional data that is not supported by a specific endpoint, you have to write another query to retrieve the data you need.
REST APIs can only accommodate sending data over endpoints. This means a developer needs to architect an endpoint before they can make their data available. As a result, the data a server sends over is what the developer who wrote the API has decided it should send. This may not necessarily align with the data that you want to retrieve.
Say that you want to retrieve a list of usernames. Using REST, you may have an endpoint:
/api/users
This endpoint returns a list of all users. It shows the day a user signed up to the platform, the email addresses of each user, and more. But our application only wants a list of usernames. With GraphQL, we can retrieve this information specifically:
query { User { username } }
In this query, we only fetch the usernames of our users. We don’t fetch any data we do not need to retrieve.
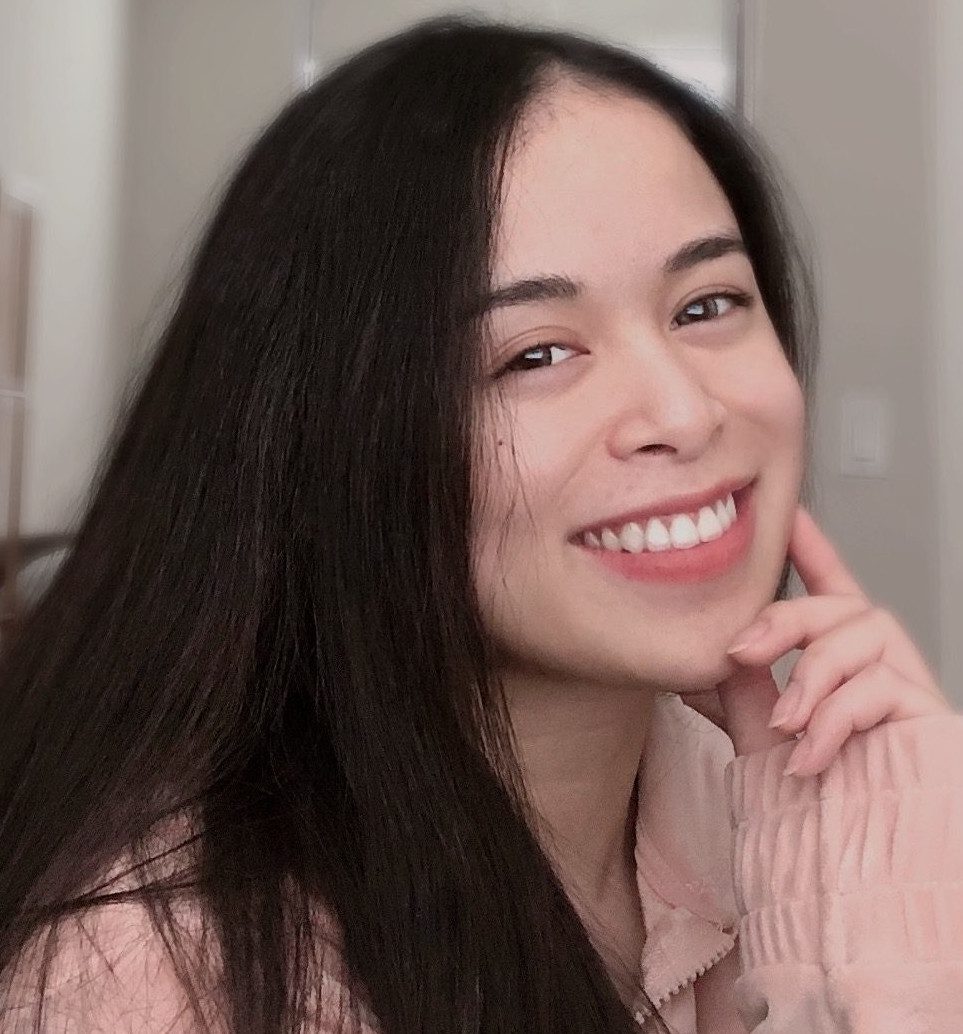
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
GraphQL vs. REST: Which is Better?
There is no clear winner when it comes to GraphQL and REST.
GraphQL is a more modern technology. It’s much more flexible than REST because you can retrieve only the data that you need. What’s more, GraphQL lets you write queries.
Queries are easier to read than API endpoints. Because you can write queries, it is easier to define a clear structure that defines what data you want to retrieve from an API. This behavior is possible because queries are written on the client-side, not the server side.
With that said, REST has its own benefits. REST principles are clearly defined and the architecture has been around much longer than GraphQL. This means there is plenty of documentation to which you can refer as you build your API.
REST has been successful in helping developers communicate information across the web. As a result of the REST architecture, developers now think in terms of APIs. This avoids the dreaded situation where each version of an application, such as a website, a mobile app, or a watch app, each interface with a back-end system on their own.
GraphQL has its drawbacks. As queries get more complicated, the human-readability advantage of GraphQL can get lost. What’s more, GraphQL comes with a lot of architectural overhead. For smaller applications, GraphQL may be more than you need.
With a GraphQL API, design can happen on the front-end. This is because you can access data using queries and mutations. With a REST API, a web developer must write single endpoints that convey information. This all happens on the back-end.
Conclusion
GraphQL is a good investment if you want more granular control over your data. Using queries, you can retrieve only the specific pieces of information you need.
REST is better if you want to develop an API with a clearly defined standard that is recognized by most developers. Because GraphQL is still new, its adoption among developers is still nascent. Now you have the knowledge you need to make a clear comparison between GraphQL and REST.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.