Golang, or Go, is Google’s prized programming language. Like other languages and frameworks, this open-source programming language has specific rules, resources, and guidelines that help programmers make the best out of their skills. If you are a new Go developer, learning and implementing Golang best practices will help you significantly.
In this article, you are going to see the top ten Golang best practices to get on a path to quality coding. First, you will get a clear picture of what Golang is, how it works, and the key concepts you need to learn to understand Golang best practices. You are going to learn the common problems you will solve by mastering Golang techniques and guidelines.
What Is Golang?
Golang is an open-source programming language that Google developers designed in 2009. The lead developers of the original Go language are Robert Griesemer, Rob Pike, and Ken Thompson. Golang is statistically typed and compiled so its implementation is primarily done by the system compiler. The semantics of open-source programming languages are similar to C and Java.
Developers of Go wanted to make a programming language that you can use instead of C++ and Java. Even if Go is a Google programming language that works more ideally with its network services, various professionals use it to program different systems. The programming language is most commonly used for writing microservices and web development software.
5 Concepts You Need to Understand for Golang Best Practices
Before you go into Golang best practices, there are some common variables you need to master. Learning the meaning of these terms will give you a clear understanding of the best practices to follow.
- Go Libraries. Like other types of programming languages, Go has libraries. These libraries are a collection of resources that help programmers save time troubleshooting unknown error messages while they work. The resources could be ready-made code, modules, or anything useful during the development process.
- Nesting. Nesting is an important skill for first-time Golang CI programmers. Nesting occurs when a programmer includes one programming construct into another construct. It is a form of object-oriented programming. It makes simpler code that saves you from future pain of troubleshooting by significantly reducing the overall programming time.
- Compiler. A compiler is computer software that allows programmers to write programs. When a programmer writes code using a high-level programming language and feeds it to the compiler, the compiler converts the code to a language the computer can understand, machine language.
- Code Splitting. Code splitting involves classifying code into several small files in a single package documentation. All the files will share the same properties and attributes but only a single common file in the main package playground will have the primary generic function. This main file will serve as the entry point during code review and execution.
- GoProxy. GoProxy is a repository for open-source Go modules. While these modules are typically free, there are some premium options. This proxy helps to keep your module downloads secure. You can install it on your system by following the instructions on the GoProxy website.
5 Common Challenges That Golang Guidelines Can Address
Coding in Golang is not always a smooth process even for the most advanced developers. There should always be room for mistakes and revisions as a project progresses. The good news is that following Golang best practices will help you minimize these errors significantly. Some common errors you will face when you ignore best practices are listed below.
Lack of Collaboration
Most large Go projects require the collaboration of two or more programmers or concurrent programming. If you leave brief notes at intervals during your part of the development process, other developers will be better suited to do their jobs. If you leave the project and another expert is asked to review it, the simple notes you have placed within would make the transition smooth.
Development of Slow Apps
One of the most popular Golang best practices involves dividing code from the same package into different files. Without this code-splitting practice, Golang apps would take too much time to load since the entire package will be processed each time a visitor clicks on a page.
Misuse of Concurrency
In Go programming, concurrency occurs when a programmer works on many computations at the same time. Without knowledge of Golang best practices, you may misuse concurrency. Make sure to reduce your cognitive load by effectively managing and integrating your computations simultaneously. This will also reduce the likelihood of a run-time error.
Lack of Resources
Since Go is still considered a modern-day or new programming language, you may get frustrated by the lack of resources in the Go community. However, learning about Go best practices will give you insight into some of the best Golang libraries, resources like a helper function, variable names, and modules you need to get started.
Complexity in Cloud-based Development
Go is one of the best programming languages for cloud-based and distributed computing. This explains why companies like SendGrid, SoundCloud, and Dropbox use Golang for their products. It is also an ideal option when it comes to scalability. Golang simplifies the development of cloud-based applications when compared to other programming languages.
Top 10 Golang Best Practices and Guidelines
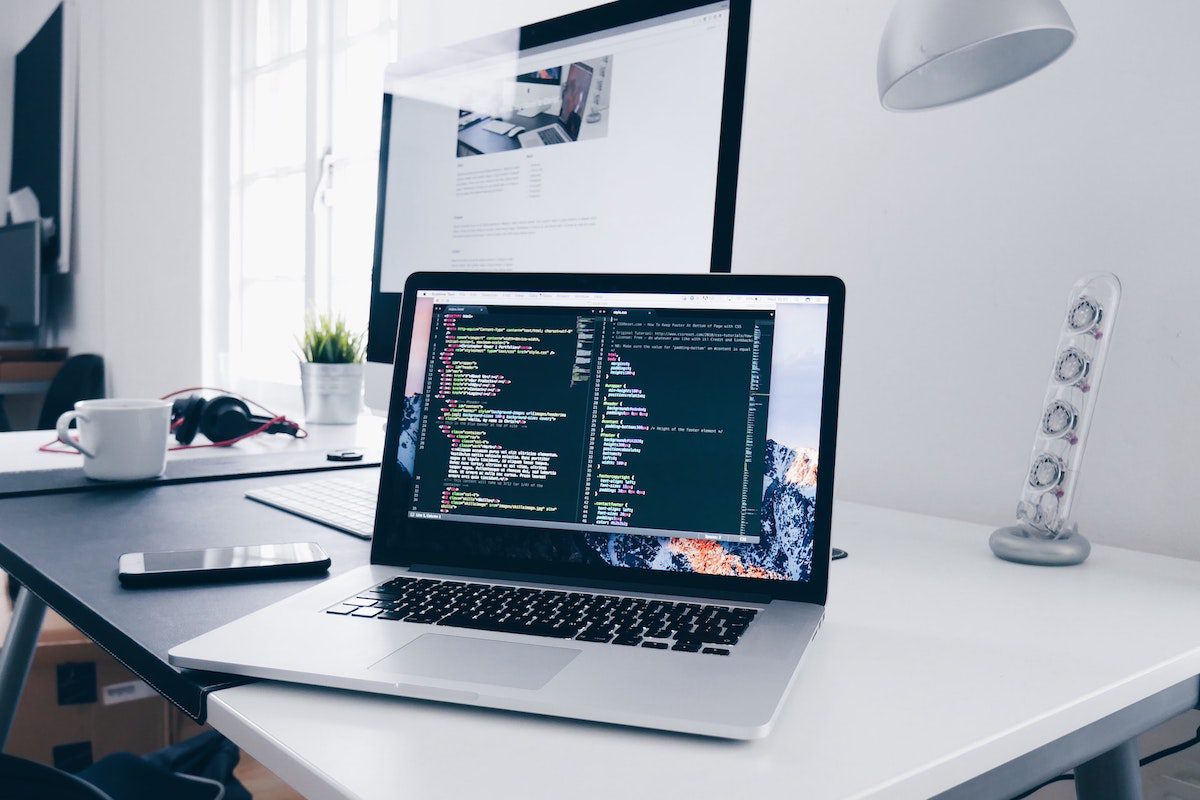
While there are several guidelines and best practices for Golang, the ten listed below are some of the most important. With time and experience, you may come up with other strategies to enhance your Go programming skills, but keep reading for some techniques to get started.
Use the Right Go Libraries
The Go library you choose should depend on what you are trying to achieve. For example, if you want to create a microservice, your target standard library should be Go kit. If your project is full-fledged web development, you may want to consider GORM as your primary resource. GORM is the perfect tool for converting data and ensuring compatibility across platforms.
Most go library code is open-source, meaning it is freely available on the internet for anyone who wants to use it. However, some resources cost money. Before you spend money on a paid library or similar resources, research thoroughly and ensure that you are not wasting your money on unhelpful or bad code for your overall goals.
Clear Errors on the Go
The best way to write a clean, fast and efficient program is to get rid of return nil errors, return errors, or any other concrete type of original error on the go. If you wait until after you’ve written an entire program before you start checking for an error message and running tests, you will run the risk of nesting.
While nesting might be great for other programming languages, advanced Go developers do not recommend it. Nesting too much during Go programming may lead to complications when it’s time to upgrade the software or revisit the code language. It makes it difficult for the future maintainers of your project to follow logical workflows.
Add Regular Notes in Your Source Code
Leaving brief notes in your codebase helps you keep track of your project and makes it easier for other programmers to get involved even in your absence. You can leave a single-line comment that describes a particular block of code. Block comments are especially important for complex lines of application code or if you are building a complex architecture.
To write the perfect comment, use code annotations or tags. You should add details like your name, module, purpose, and an explanation for any possible complexity or complication with the code you are describing. Keep all your comments straightforward, and make sure that you write the notes while you are writing an efficient code, not afterward.
Follow the Right Code Structure
How you structure and organize your Golang code base will contribute significantly to the end product. As a rule of thumb, the most essential code should come first, followed by the import statements, before adding anything else. Even when you’re writing seemingly less important code, you should still write in order of significance.
Note that Go code isn’t traditionally organized within a normal directory tree like other popular programming languages. You need to make sure that you leave only the most relevant code in a package to prevent unnecessary clutter and perform effective tests. This is a gold standard for all coding languages, not just Go.
Share Go Codes Into Smaller Files
Code splitting is a best practice that speeds up the compile time and boosts functionality in the long run. By sharing code into different files in the same package documentation, you will make your application load faster and easier to run. It also means that the browser does not need to download large files anytime someone tries to load your application.
For example, when you build a website with Golang and apply code or bundle splitting, only a fraction of the code would load when visitors are on the home page. When they visit the contact page, only the information will load. So, code would be available on-demand rather than in bulk each time a single page is visited.
Use the Right Integrated Developer Environment (IDE)
An integrated developer environment is a software that gives programmers hands-on access to the development tools they need to write, edit, compile, debug, and execute code. IDEs are important during Golang development, but not all the IDEs available will give you the best result.
At the time of writing, it is generally agreed that the best-integrated development environments for Golang developers are LiteIDE, Atom with Go-Plus, VSCode, and Vim-go. LiteIDE is one of the oldest IDEs for Go developers and has been in use since 2012. It includes useful Go features like cross-platform compatibility.
Always Use GoProxy
There is limited security whenever you use open-source resources on a private or public network. GoProxy plays a similar role that proxy browsers play when accessing the internet through your laptop smartphone. In this case, the GoProxy keeps your module downloads secure, ensuring that you only use reliable sources.
GoProxy is not the only Go module proxy in the world. However, it is one of the most popular and reliable options advanced developers use. If you do not want to use it, some great alternatives are Envoy Proxy and gobetween.
Add Specific Datatypes to Each Variable
Whether you’re creating software on your own or working with a team of developers, adding specific data types to each variable is a best practice. This makes it easier for you to identify a single line of code written among thousands, especially when you need to rewrite or edit that particular line of code.
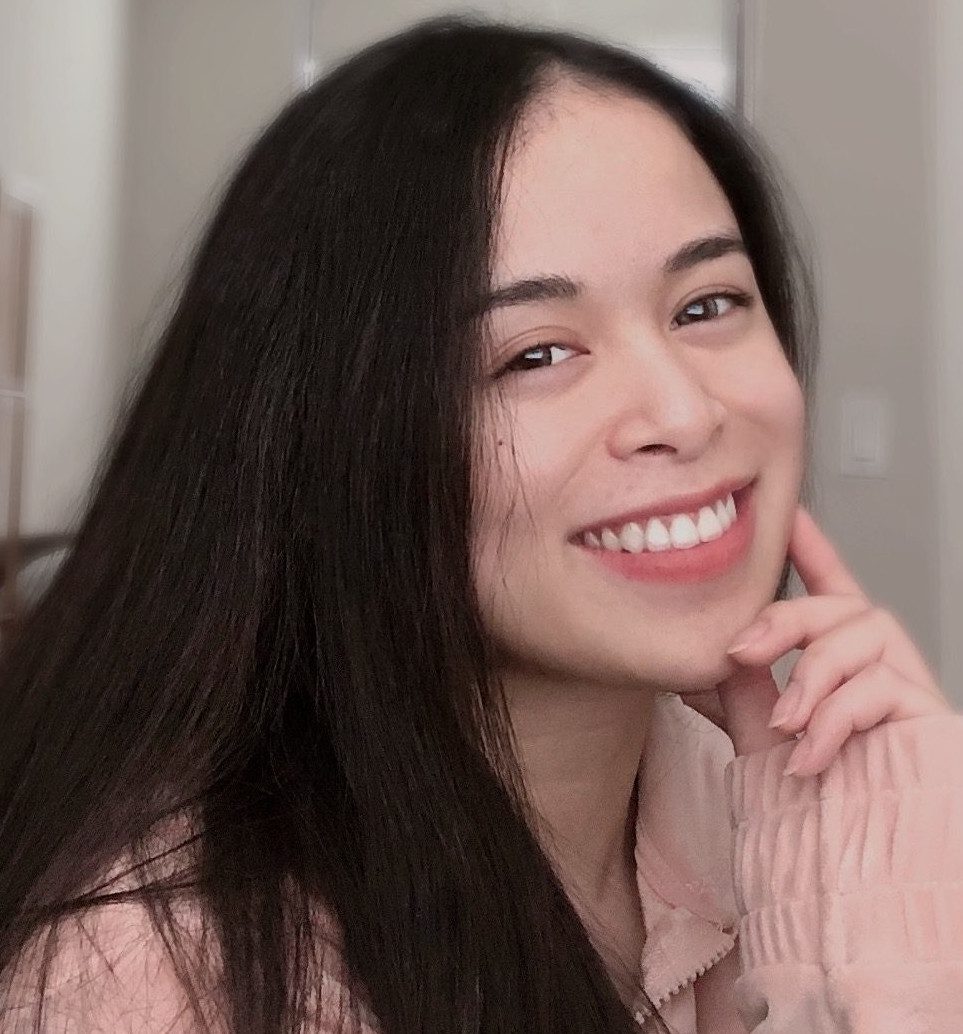
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
There are different data types used in Golang programming, including aggregate types, basic types, interface types, and reference types. You can choose one particular data type and stick to it throughout the coding process, or you can explore many different data types in one program.
Don’t Confuse Go With Java
In writing, Go shares many similarities with Java. If you already know Java, you may be tempted to use Java principles while coding Go. You must follow the design patterns and execution rules for Golang if you want to become a Golang developer. Java might be an older programming language with more resources than Go, but make sure that you don’t mix up the two.
Mind How You Use the Concurrency Feature
One of the most popular features that makes Golang unique is concurrency. It allows programmers to speed up development time by working on several aspects of the project simultaneously. While this feature is beneficial, beginners shouldn’t use it too frequently.
A wrong move in concurrency will lead to race conditions, leading to too many bugs and glitches on the finished software. To minimize this problem, make sure you run a race condition detection software to help you detect and fix the problem before proceeding.
How to Learn Golang Best Practices
The best way to learn Golang best practices is to understand and practice with the programming language itself. The more you know about Golang, the more knowledge you will acquire regarding the best guidelines and procedures.
Can a Bootcamp Help You Learn Golang Best Practices?
Yes, a coding bootcamp can significantly help you learn Golang or many other programming languages. These bootcamps offer accelerated tech training that can be compared to university degrees. These programs run for three to six months, depending on the bootcamp you choose.
Bootcamps are also considerably more affordable than traditional degrees. They also provide career services and help students develop the soft skills needed to thrive in the industry. Unfortunately, it is not easy to find a bootcamp that offers stand-alone Golang programs. You can also opt for online courses and practice Golang projects on your own.
Best Courses and Training Programs to Learn Golang Best Practices
Provider | Course | Price |
---|---|---|
Udemy | Go: Mastering Google’s Go (Golang) Programming | $84.99 |
Udemy | Learning Path: Go: Building Cloud Native Go Applications | $84.99 |
Coursera | Programming with Google Go Specialization | Free |
Udemy | Master Go (Golang) Programming: The Complete Go Bootcamp 2022 | $84.99 |
Pluralsight | Go Fundamentals | $19 monthly |
Should You Learn Golang Best Practices?
Yes, you should learn Go best practices if you intend to become a professional Go developer. According to ZipRecruiter, Go developers earn an average salary of $110,456. The best practices make programming easier. Following Golang’s best practices during web development ensures that your product meets the global requirements for Go development.
Golang Best Practices and Guidelines FAQ
Start by learning the basic concepts of Golang before working your way up to more advanced aspects of the programming language to become a good Golang developer. You also need to master all the available Go libraries, partners, and Go best practices. It also helps to create a project portfolio and complete Golang projects as you learn.
Yes, Google still uses Golang as their primary programming language for services like YouTube and other open-source Google projects. There are newer and more definitive versions of the Golang programming language released at intervals, so the best version is always available online.
Go is the right name for Google’s programming language, not Golang. However, it is popularly called Golang because of the former domain name for resources, Golang.com. While Go is the official name, Golang isn’t technically wrong either.
Yes, Golang and Java have similar structures. Both are server-side programming languages, both are in the C programming language family, and both use garbage collectors. However, Go is faster than Java, easier to read, and supports concurrency. Go is also a newer programming language than Java.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.