Stashing lets you save your code for later in a Git repository.
When you’re working with a Git repository, you may make changes to a file that you want to apply to a Git commit later on.
That’s where the git stash command comes in handy. Stashing lets you save the code on your working branch for a later date. In this tutorial, we’ll discuss, with examples, the basics of stashing in Git and how to use the git stash command.
What is a Git Stash?
Stashing lets you save code in your working directory and index for later. Sometimes, when you are writing code, you will want to save the changes you have made, but not commit them to the repository.
One scenario where this may happen is in fixing issues. Suppose a bug report comes in. You’re already working on implementing a feature. You may want to save the changes you made first toward the feature and then fix the bug.
This is because the bug report would likely take precedence over the feature you are developing. You would want to fix the bug first, before committing the feature-focused code to the repository. You would not want your bug fix and feature to appear in the commit. This is because larger and more complex commits make it more difficult for other developers to read the history of a repository.
In other words, stashing lets you work on something else in a Git repository without having to discard or commit your existing code.
Stashing allows you to save, or “stash,” the changes you have made to a file for later. Once a file has been stashed, you can work on something else. Then, you can come back later and apply the changes you have stashed to your code.
Stashing reverts the current working directory to the last commit. This means that stashing our code gives us a clean working directory with which we can work. Having a clean directory means we can make changes without having to worry about merge conflicts. We also do not have to think about sorting the changes we make between commits.
How to Stash Changes in Git
The git stash command is used to stash code for later. When you run git stash, the changes you have not pushed to a commit in your current working directory will be saved for later. This includes both staged changes (changes added to the staging area using git add) and unstaged changes.
Here’s the syntax for the git stash command:
git stash
Let’s suppose we are working on a website and our code is stored in a Git repository.
We have made changes to the index.html and index.js files in our code. We want to stash these files for later while we fix a design bug in our index.html file.
To view our changes, we can use the git status command. This returns, in our example:
On branch master Your branch is up to date with 'origin/master'. Changes to be committed: new file: index.js Changes not staged for commit: modified: index.html
This shows us that we have created a new file (index.js), and we have modified an existing one (index.html). We want to save these changes for later while we work on another part of our codebase. To do so, we are going to use the git stash command:
git stash
Our code returns:
Saved working directory and index state WIP on master: 3b16026 feat: Launch new homepage
The git stash command has saved the changes we made to our repository for later. Now, if we run the git status command again, we can see that there is nothing to commit:
On branch master Your branch is up to date with 'origin/master'. nothing to commit, working tree clean
The git stash command has saved the changes we made to our repository for later. Now, if we run the git status command again, we can see that there is nothing to commit:
When your code is stashed, your repository is reverted to its previous commit without the changes you made. The changes you made to your code have been saved for later. They can be applied to your codebase when you are ready by using the stash pop command.
Stashing Untracked Changes
The git stash command will only stash staged and unstaged changes to files already being tracked in the Git repository. By default, the stash command does not include untracked changes.
Staged changes are the changes that have been added to the staging area. The unstaged changes that are stashed are those made to files tracked by Git. If you change a new file that is not tracked by Git, it will not be added to a Git stash. Files that have been ignored will not be added to a stash.
These are default preferences applied when using the git stash command. If you want to stash your untracked files — such as a new file that has not been staged — you can use the -u flag. Here’s the syntax for the -u flag:
git stash -u
Alternatively, if you want to include ignored files in your stash, you can use the -a flag. Here’s the syntax for the -a flag:
git stash -a
Using the -a flag will tell git stash to stash changes to your ignored files. These are the files defined within the .gitignore file in your code repository (if you have one).
Apply Stashed Changes Using git stash pop
The git stash pop command is used to apply stashed changes to a repository. Suppose we have decided that we want to apply the stashed changes we made in the previous example. We could do so using this command:
git stash pop
The git stash pop command returns:
On branch master Your branch is up to date with 'origin/master'. Changes to be committed: new file: index.js Changes not staged for commit: modified: index.html Dropped refs/stash@{0} (48afd55381cf43f2332f771349c7233fb99f80a6)
Upon running the git stash pop command, the changes from our stash are applied to the local working copy of our repository. The pop command applies the code in the tash to your repository. Then, the stash is emptied.
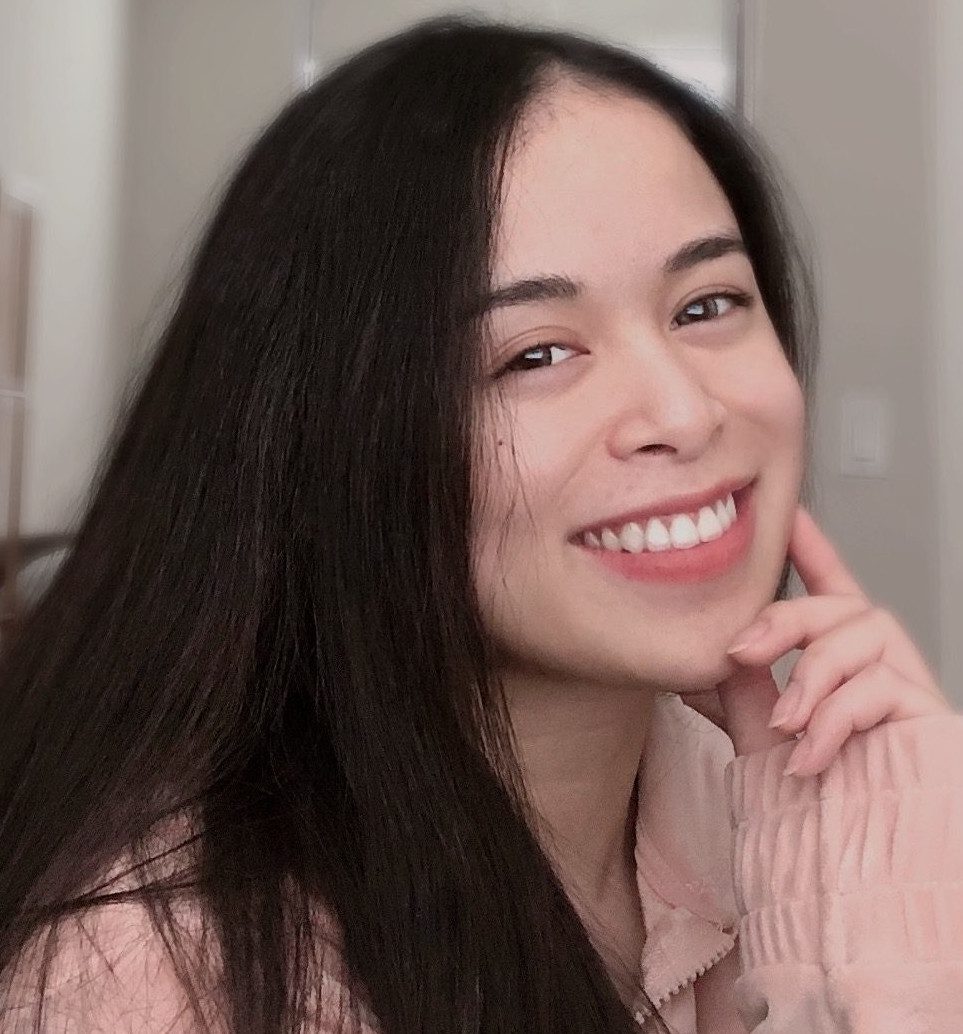
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
The git stash pop command also returns a list of changes you have made to your code, which shows what was in your stash.
You can use the git stash apply command to apply the changes you have made to your code. You can keep those changes in your stash. We can keep the changes in our stash this using the following command:
git stash apply
The command returns:
On branch master Your branch is up to date with 'origin/master'. Changes to be committed: new file: index.js Changes not staged for commit: modified: index.html
Applying Changes Across Multiple Branches
Applying the same stashed changes to many branches across your codebase is a good use case for the stash command. For instance, you may decide to apply a stash to one branch. Then, you would move to another branch and use the same stash to apply the saved changes.
Multiple Stashes
You can create multiple stashes in Git. This means can make changes to one file and stash them for later. You can make changes to another file and store them in a separate stash.
Tracking Stashes
To return a list of stashes in a repository, you can run the following command:
git stash list
Suppose we wanted to retrieve a list of all stashes we have created. We could do so by executing the above command. This command returns:
stash@{0}: WIP on master: 3b16026 feat: Launch new homepage stash@{1}: WIP on master: 3b16026 feat: Launch new homepage
The git stash list command returned a list of our stashes.
However, these stashes use only the default commit messages associated with the stash. This means that it is difficult for us to track which stash includes which changes. This is because we have not specified a description on any of our stashes.
Let’s say we want to add a description to a new stash. We could do so using this command:
git stash save "add new change to website"
The command returns:
Saved working directory and index state On master: add new change to website
Rather than being given the default message associated with our last commit, our stash has been assigned its own message.
Applying Multiple Stashes
The git stash pop command applies the most recent stash to your repository (the one with the tag stash@{0}). However, when you’re working with multiple stashes, you may want to apply a specific stash to your codebase.
You can do so by specifying the unique ID associated with the stash you want to apply to your codebase. Suppose we want to apply the stash stored at stash@{1} to our codebase. We could do so using this command:
git stash pop stash@{2}
The command applies the changes stored in stash@{2} to our repository and deletes the stash.
Creating a Branch Using a Stash
Stashing is a way to conveniently store the changes you have made to a codebase while you work on making another change.
However, there may be times when, instead of maintaining a stash, you want to move your code into its own branch.
This scenario could arise if there are conflicts that arise when you merge your code. You may also want to move a code into another branch if you are making larger changes to a codebase. That’s where the git stash branch command comes in.
You can use the git stash branch command to create a new branch to which the changes in a stash will be applied. The syntax for this command is as follows:
git stash branch <new-branch> <stash-id>
The “new-branch” parameter refers to the ID of the branch which should be created. “stash-id” refers to the ID of the stash whose code you want to apply to the new branch.
Suppose we want to apply the changes from our stash@{2} stash to a new branch called “update-site”. We could do so using this command:
git stash branch update-site stash@{2}
The command returns:
Switched to a new branch 'update-site' On branch update-site Changes to be committed: new file: index.js Changes not staged for commit: modified: index.html Dropped stash@{2} (9a15b9cd20f8988937134d1267fafbea4c6a8647)
First, the git stash branch command creates a new branch for our stashed changes. The changes we have made to our repository are applied to the new branch. Then, we are switched to viewing that branch. Finally, our stash is deleted because the stashed code has been saved in its own branch.
Delete a Git Stash
Once you have used the code in a stash, you can use the git stash drop command to delete the stash. Here’s the syntax for the git stash drop command:
git stash drop <stash>
Suppose we want to drop the stash with the ID stash@{2}. We could do so using this command:
git stash drop stash@{2}
The command returns:
Dropped stash@{2} (82079798c950b053fac0efb7b1d5693864dc96e7)
Alternatively, you can use the git stash clear command to delete all stashes associated with a repository. The syntax for this command is:
git stash clear
This command deletes all the stashes in our repository.
Stashing Specific Files
The git stash command stashes all the tracked files in your current working directory by default. However, in some cases, you may decide that you want to stash a specific file or set of files.
You can stash a specific file using the git stash push command. Here’s the syntax for using this command:
git stash push -m "<message>" <file>
The “message” parameter refers to the message which will be associated with your stash. The “file” parameter is the name of the file you want to stash.
Suppose we want to add only the changes we have made to the index.html file in our code to a stash. We could do so using this command:
git stash push -m "feat: changed index.html file" index.html
The command returns:
Saved working directory and index state On master: feat: changed index.html file
The command has only added the index.html file to a stash with the commit message “feat: changed index.html file”. All other changes we have made to our code have not been added.
Show Differences Between Stashes
When you’re working with stashes, you will likely make a number of different changes to your codebase across multiple commits before you return to the code that you stashed.
For instance, if you have stashed your code and gone on to fix a bug, you may push a few commits to fix the bug before you return to your stashed code. This means that you may want to see a summary of the differences between your stash and the most recent commit of your code (so you know what changes you have made since stashing your code).
To view the differences between a stash and your most recent commit, you can use the git stash show command. The syntax for this command is:
git stash show <stash-id>
The “stash-id” parameter is the ID of the stash whose changes you want to compare to the most recent commit of your branch. Suppose we want to compare the code in our stash@{1} stash to the current state of our code. We could do so using this command:
git stash show stash@{1}
The command returns:
index.html | 2 +- index.js | 0 2 files changed, 1 insertion(+), 1 deletion(-)
This output tells us that we have made two changes to our code: one insertion and one deletion. If we want to view the differences between our files, we can see them using the -p stash flag. The syntax for this flag is as follows:
git stash show -p stash@{1}
The above command, when executed, returns:
diff --git a/index.html b/index.html index 4dd1ef7..e859c68 100644 --- a/index.html +++ b/index.html @@ -1 +1 @@ -</div> +</html> diff --git a/index.js b/index.js new file mode 100644 index 0000000..e69de29
This output allows us to see a full comparison of the code in a stash and the most recent commit of a branch. The above output, for example, tells us that we removed the </div> line from our index.html file (denoted by the – symbol) and that we added the </html> line into our index.html file (denotes by the + symbol).
Conclusion
The git stash command stores the changes you have made to a codebase temporarily. When you’re ready, you can come back and apply the changes you have made to your codebase.
This tutorial discussed the basics of stashing in Git and how you can use the git stash command to use the Git stashing feature. Now you’re equipped with the knowledge you need to start stashing code like a Git pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.