Git repositories have become a popular way to manage a codebase. Using Git, you can have multiple people working on a project simultaneously.
Everyone can have a version of a project on their local machine to modify, but any changes made will only affect the main project when they are “pushed” to the main repository.
Git offers a feature called pull requests which allow developers to propose changes to a codebase without actually making those changes. In this guide, we’re going to discuss what pull requests are, why they are useful and how you can create your own pull request.
To follow along with this tutorial, you’ll need to have Git installed on your local machine. You should also have an account on GitHub, which we’ll be using to show you how to create a pull request.
What is a Pull Request?
A pull request is a way to submit a contribution to a software project using a version control system such as Git. Developers use pull requests to propose changes to a codebase.
Using a pull request, a developer can show everyone who is working on a project what changes they think are necessary. For instance, if a developer is working on a bug fix, they could create a pull request to show everyone their solution to the bug.
When a pull request is created, anyone on the project can view that pull request. In this case, it would mean that a developer could ask for feedback on their bug fix from other team members. This all happens without making a change to the final codebase.
Why Are Pull Requests Useful?
Pull requests allow developers to effectively collaborate using code. Instead of having to push a change to the main version of a project, a developer can submit a pull request. This method allows them to facilitate feedback on their code.
Because pull requests don’t involve changing the main version of a codebase, they are also a great tool for code review. If there are any bugs in your code or any ways in which your code could be improved, they could be addressed directly within the pull request.
There’s no need to worry whether bugs have been introduced into the main codebase; a pull request is stored in isolation from the main version of a project. Often, pull requests are used in public repositories for open source projects. This is because the code in these projects has many different contributors.
The code in a pull request may be a work in progress. This is because it will not affect the main version of a code until a git merge operation is run or the pull request is moved into the main version of a project by the project maintainer.
Step 1: Create a Fork
If you are contributing to someone else’s repository, you first need to have your own copy of a repository to which you have made changes. This is where forking comes in. In Git, the word “fork” refers to creating your own copy of a repository.
Note: You can skip this step if you are contributing to a repository you own.
We’re going to create a fork of the “pullrequest-tutorial” repository on Career Karma Tutorials. You can view this repository here:
https://github.com/Career-Karma-Tutorials/pullrequest-tutorialOn GitHub, you can create a fork by pressing the “Fork” button on the top right side of a repository:
When you click this button, a copy of the repository will be created under your account. In my case, if I create a copy of this repository, it will be moved to jamesgallagher432/pullrequest-tutorial. Now that we have a forked repository, we need to make a local copy of our code and create a branch.
Step 2: Create a Local Copy and Create a Branch
We have our version of the pullrequest-tutorial repository on GitHub. Although we can make changes to our code on GitHub, this is impractical for most software projects. Therefore, we are going to create a copy of our repository on our local machine.
You can create a copy of a repository using the git clone command line operation:
git clone https://github.com/username/repository_name cd repository_name
These commands create a local working copy of our code to which we can make changes and move our terminal session into the project folder. To learn more about the git clone command, read our ultimate guide to the git clone command.
We now have a copy of the repository on our local machine and we’re almost ready to make changes. The next step is to create a branch on which we can make our changes.
A branch is like another version of a repository within a repository. The default branch for a repository is called master, and is where the main version of a codebase is usually stored. Developers use branches to keep track of different versions of their code.
Suppose we want to modify the README.md file in our code. To do so, we’re going to create a branch called “update-docs” which will contain our updated code. We can do so using this code:
git checkout -b update-docs
The first command creates a new branch called “update-docs” and then changes our Git session to view the code on the update-docs branch.
Step 3: Make Changes
For this example, we are going to change our README.md to contain the following:
# pullrequest-tutorial An example repository for how to create your own pull request. Created by Career Karma tutorials.
When we save this file, our changes will be made on our local machine. But, these changes will not yet be saved to our repository. This is because Git is a distributed version control system and changes are only made to a repository when you commit them.
Before we commit our changes, we need to use “git add” to add those changes to a Git commit:
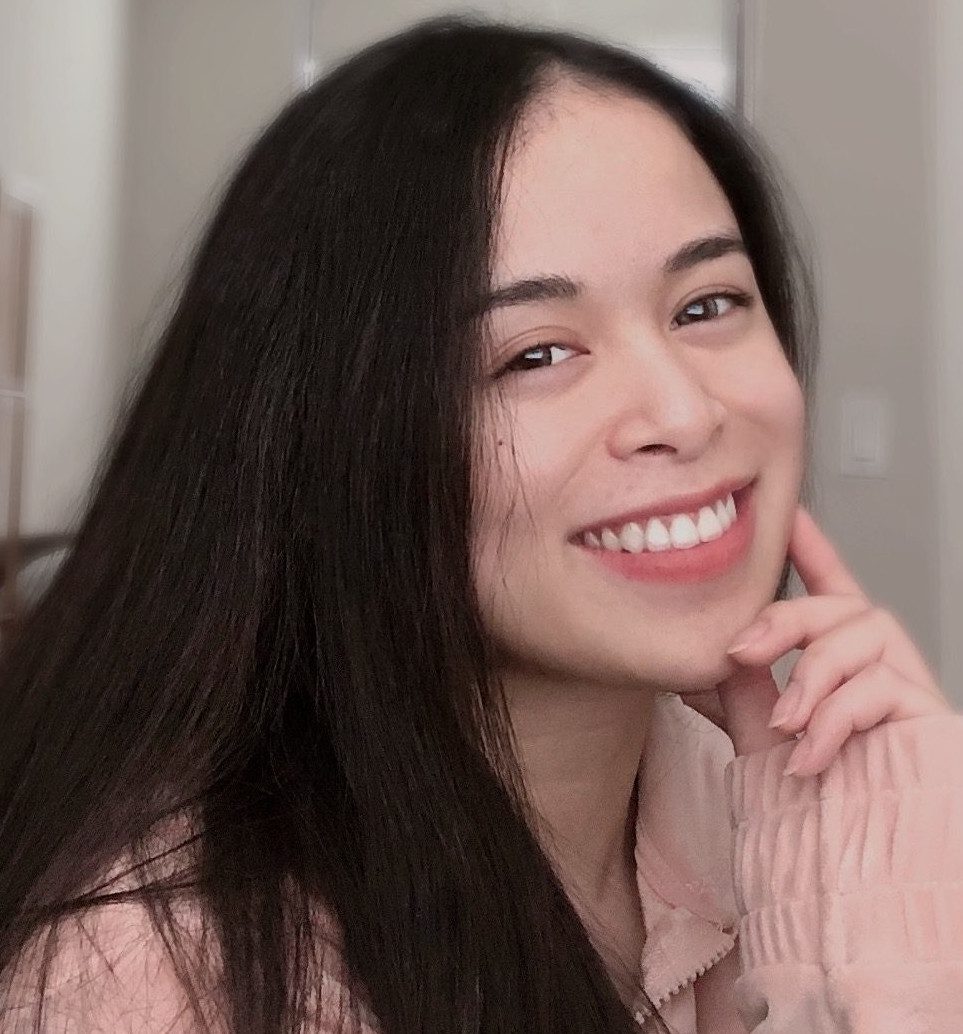
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
git add README.md
The file “README.md” is now ready to be committed to our repository.
If you want to change multiple files, you can do so. Make sure that if you change multiple files you add each of them to the staging area using “git add” otherwise they will not appear in your commit later on.
You can commit a change to a repository using the git commit command. When you use this command, you’ll need to provide a short message which describes the changes you have made.
In this example, we’re going to add the message “docs: Added created by to README.md”, which briefly tells other developers what changes we have made.
git commit -m "docs: Added created by to README.md"
If you need to write a longer commit message, you can use the git commit command without the “-m” flag. Learn more about this in our tutorial on how to use the git commit command.
At the moment, our commit is only stored on our local copy of the repository. That’s because we haven’t pushed our code to our main repository. “Pushing” is when you upload the changes you have made locally to the main version of a repository.
You can push your code using this command:
git push -u origin update-docs
This command will push your code to the version of the repository that you forked.
Step 4: Create a Pull Request
Our code is now uploaded to our remote Git repository, so we’re ready to make a pull request. To do so, we can go to the homepage of our forked version of the repository. You’ll see there is a button which says “Compare & pull request” that is associated with our new branch:
When we press this button, we can create a pull request with our changes:
In this form we can specify the name of our pull request (which is automatically set as the commit message of your most recent change) and the description for our pull request.
In this case, we are going to propose merging our pull request to our “master” branch. We do this by specifying “base: master” in the top box of the “Open a pull request” form.
We could also propose changing the Career Karma Tutorials version of the repository by specifying it as the “head repository”.
Upon clicking the “Create pull request” button, a new pull request will be added to the repository. You can view the pull request created in this tutorial on GitHub.
Wrapping Up
Congratulations, you’ve just created a pull request using Git and GitHub!
To recap, the steps you need to follow to create a pull request are:
- Create a fork (if you don’t have one already)
- Create a local copy of a repository
- Create a new branch and make changes
- Push your code
- Create a pull request in GitHub
Now you’re equipped with the knowledge you need to start contributing to projects through pull requests like a Git pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
Saw a few of James Gallagher blog posts about Git and damnnn, he writes and explains the concepts very very well… The only thing I think it’s missing is: explain if there are any differences when the repo contains multiple files; explain what –set-upstream is
Hello there! I appreciate your reading over my tutorials.
I have updated the code snippet in question to use “git push -u origin update-docs” which I think is clearer for beginners. Could you clarify your inquiry regarding “when the repo contains multiple files.” I have updated the article to include a note on how you can update multiple files but I wondered if there was anything more specific you were looking for.