The git log command displays a record of the commits in a Git repository. By default, the git log command displays a commit hash, the commit message, and other commit metadata. You can filter the output of git log using various options.
Version control systems have one core purpose—to record how your codebase evolves over time. This allows you to see how your projects have progressed. You can find out who has contributed to your project and identify what changes have been made to your code and when.
But how do you actually view the history your Git repository creates? That’s where the git log command can be helpful.
This tutorial will discuss, with examples, the basics of the git log command and how you can use it to inspect a Git repository.
The git log Command
The git log command shows a list of all the commits made to a repository. You can see the hash of each Git commit, the message associated with each commit, and more metadata. This command is useful for displaying the history of a repository.
Whereas the git status command is focused on the current working directory, git log allows you to see the history of your repository.
git log Command Example
Let’s walk through an example to illustrate how the git log command works. We have been working on a repository called “demo-repository”. Now we want to see a list of all the commits we have pushed to our repository. To do so, we can use this command:
git log
The git log command returns a list of all commits made in a repository. Here’s an example of a single commit returned by this command:
commit 43433c674e3d6c86a889fad222dae179785893cf (HEAD -> master, origin/master, origin/HEAD) Author: James Gallagher <37276661+jamesgallagher432@users.noreply.github.com> Date: Tue Apr 7 13:09:58 2020 +0100 Update index.html
Let’s discuss this output step-by-step. Each entry returned by the git log command contains:
- The Secure Hash Algorithm (SHA) hash for the commit, which is used to uniquely identify each commit in a repository. In our above example, this was “43433c674e3d6c86a889fad222dae179785893cf”.
- The author of the commit. In our above example, this was James Gallagher. The email of the author is also specified.
- The date the commit was pushed. In our above example, this was Tuesday, April 7th.
- The message associated with the commit. The commit message returned above was “Update index.html”.
All this data gives us useful information about the commits in our repository.
Filtering the Output of git log
By default, git log returns a list of all the commits made to a repository using this structure. As you can imagine, it can quickly become difficult to read your git log statements if there are many commits to read.
git log comes with a few flags you can use to filter your logs. These are:
Filter by Most Recent
If you want to return a specific number of commits, you can do so using the -n flag. Here’s an example of this flag in action:
git log -n 3
This command returns a list of the three most recent commits made to a repository.
Filter by Author or Committer
You can also filter the commits returned by git log by the person who wrote or committed the changes. Suppose we want to see a list of commits pushed by “John Smith.” We can do so using these commands:
git log --author="John Smith" git log --committer="John smith"
The author flag limits the results to commits whose changes were made by John Smith. The committer flag limits the results to the commits that were actually committed by that individual.
You will find that, in most cases, the author and committer are the same individual. In larger projects, the author of a commit may not necessarily be the one who pushes it to a repository. This is why these two flags exist.
Filter by Date
In addition, you can filter the results of git log by date. To do so, you can use the—before and—after flags. These flags both accept a wide range of date formats, but the two most commonly used are relative references and full dates.
Suppose we want to retrieve a list of commits from after 2019-3-2. We could do so using this command:
git log --after="2019-3-2"
We have specified a date to filter our commits. Similarly, if we want to retrieve a list of commits from before yesterday, we could do so using this command:
git log --before="yesterday"
We have specified a relative value (“yesterday”).
Now, suppose we want to retrieve a list of commits that were published after 2019-3-2 and before 2019-3-19. We could do so using this command:
git log --after="2019-3-2" --before="2019-3-19"
Filter by File
When you’re using the git log command, you may only want to see a list of commits that have affected a particular file. To do so, you can specify the file whose changes you want to see.
Suppose we want to see the changes made to the “main.py” file in our code. We could do so using the following command:
git log -- main.py
The — statement instructs the git log command that the arguments we have specified are file paths and not the names of branches.
In our command, we only specified one file which we wanted to use to filter the response of the git log command. But, if you want, you can specify multiple files.
Filter by Content
You can also search for commits that have removed or added a particular line of code.
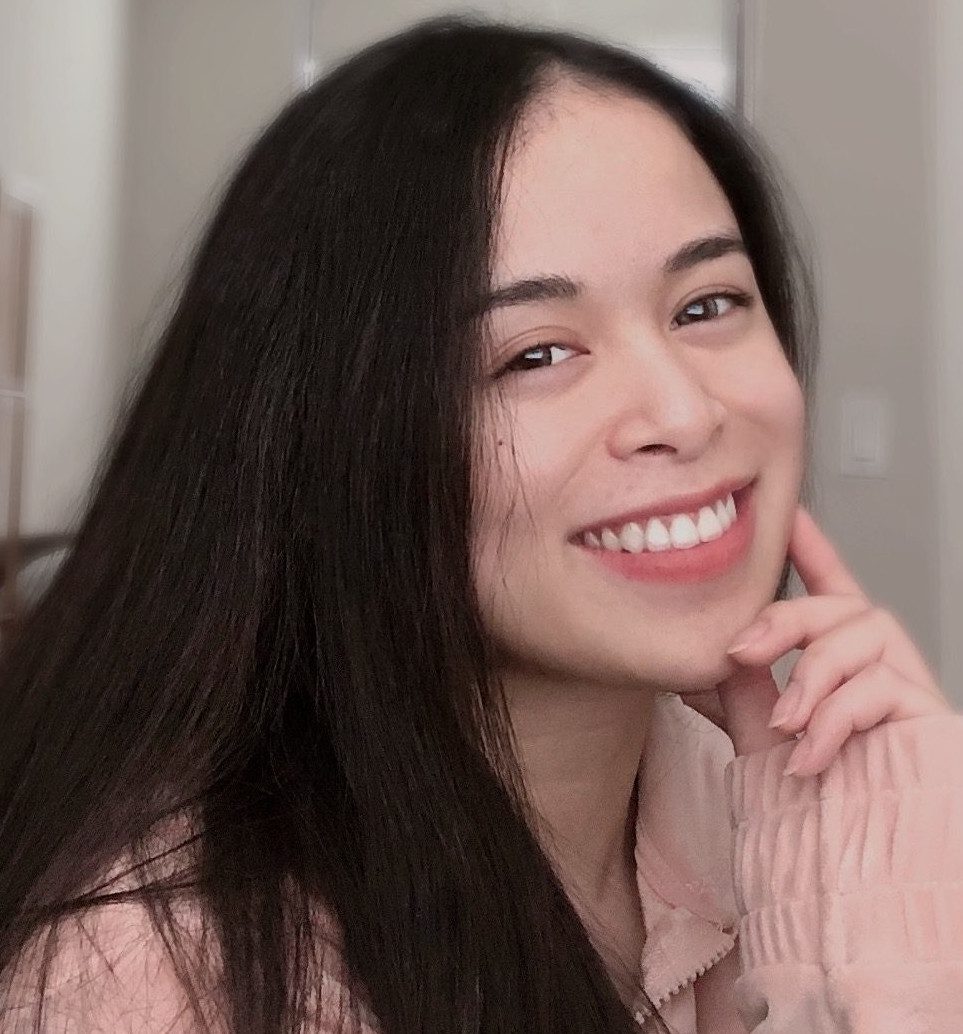
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Suppose we want to search for all commits that have added the term “# Introduction” to our code. We could do so using this command:
git log -S"# Introduction"
The -S flag is used to specify what commit change we want to look for in our list of commits. Notice that there is no space or equals sign between the -S flag. Also, the quotation marks that we used to specify the content of the commit for which we are looking.
Filter by Range
You can use the since and until parameters to pass a range of commits to git log. The syntax for these parameters is as follows:
git log <since>..<until>
Suppose we want to retrieve a list of commits made since the commit b72beb5 was pushed, and until the commit b53b22d was pushed. We could do so using this command:
git log b72beb5..b53b22d
This command returns a list of all commits between the b72beb5 and b53b22d commits.
Filter by Message
The –grep flag allows you to filter the commits returned by git log by the commit message associated with a particular commit.
For instance, suppose we want to return a list of all commits whose name starts with “feat:”. We could do so using this code:
git log --grep="feat:"
This command returns a list of all commits whose messages start with “feat:”.
Formatting the Output of git log
In the last section, we worked with a full output from the git log command. This output contained the SHA for our commit, the commit author, the date the commit was pushed, and the commit message.
While all this information is useful, there are often occasions where you only need to retrieve specific data about a commit. Luckily, the git log command comes with a few flags you can use to format the output of the command.
Return Brief Logs
By default, the git log statement returns a full log entry for each commit made to a repository. You can retrieve a list of commit IDs and their associated commit messages using the –oneline flag.
Here’s the syntax for the –oneline flag:
git log --oneline
When run in our repository from earlier, this command returns:
43433c6 (HEAD -> master, origin/master, origin/HEAD) Update index.html a7d8dc2 docs: Update README.md b53b22d feat: Update website 3b16026 feat: Launch new homepage b72beb5 first commit
We can see the commit IDs and the first line of the messages associated with a commit. This is easier to read than all of the metadata associated with a commit that the git log command would otherwise return.
Each entry appears on a single line. This git log one line technique is handy because it shows commits without displaying too much information.
Decorating the Output
The –decorate flag allows you to see all the references (i.e. branches and tags) that point to a particular commit. Here’s the syntax for this flag:
git log --decorate
This command returns:
43433c6 (HEAD -> master, origin/master, origin/HEAD) Update index.html a7d8dc2 docs: Update README.md b53b22d feat: Update website 3b16026 (tag: v1) feat: Launch new homepage b72beb5 first commit
The fourth commit in our list now has the name of its tag specified. This is because the –decorate flag reveals the references associated with each commit in our commit history.
Returning a Diff
The –stat flag allows you to display the number of lines of code added to and deleted from a repository in each commit. Here’s an example of the git log –stat command in action:
Author: James Gallagher <james@users.noreply.github.com> Date: Mon Apr 6 09:11:46 2020 +0100 feat: Update website index.html | 1 + index.js | 0 2 files changed, 1 insertion(+)
The plus signs (+) indicate insertions, and, if there were any, the minus signs (-) would indicate deletions. This data allows you to learn more about the overall changes made to a repository.
If you want to see the exact changes made to a repository, you can use the -p flag. This returns a more comprehensive diff showing the changes made in a commit.
Here is one entry returned from the git log -p command, when run on our example repository:
Author: James Gallagher <37276661+jamesgallagher432@users.noreply.github.com> Date: Tue Apr 7 13:09:58 2020 +0100 Update index.html diff --git a/index.html b/index.html index f45673f..2d2701d 100644 --- a/index.html +++ b/index.html @@ -1,2 +1,3 @@ -This is a file. -Changes have been made. +<body> + <p>This is a website.</p> +</body>
This output shows us both a description of the commit, and a detailed breakdown of each change made in the commit. We can see when people have added or removed content from files in our repository.
While this data is useful, it can quickly become difficult to read this output if there are many commits to display. You may want to use one or more of the other flags we have discussed in this article. This will help ensure the information returned by this commit is both comprehensive and easy to read.
You can read more about Git diffs in our git diff command guide.
The git shortlog Command
The git shortlog command provides a summary of a git log. The output of the git shortlog command is grouped by author which means you can easily see who made what changes to a repository.
Let’s run the git shortlog command on our repository from earlier:
git shortlog
Our command returns:
James Gallagher (5): first commit feat: Launch new homepage feat: Update website docs: Update README.md Update index.html
James is the only person who has contributed to this repository, and he has made five commits. But, if there were other contributors, their contributions would be listed here, alongside the total number of commits they have pushed to the repository.
Conclusion
The git log command shows you what changes were made to a repository, by whom, and when. You can filter the output of git log to show only the information you need to know.
The git log command comes with two types of flags. Some flags help you format the output of the log. Other flags that can help you filter the commits returned by the command.
This tutorial discussed, with reference to examples, how to use git log and the most common flags used with the command. The git log command is an important tool in your arsenal when working with Git. Once you know how to use this command effectively, you’ll be a master at inspecting Git repositories!
To learn more about Git, read our How to Learn Git guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.