A Guide to the Fibonacci Java Algorithm
The Fibonacci Sequence is a sequence where the next number is calculated by calculating the sum of the previous two numbers.
This sequence has its claim to fame in mathematics. It also appears in nature. For instance, most flowers have petals which are arranged like the Fibonacci Sequence.
In this guide, we’re going to talk about how to calculate the Fibonacci series of numbers using Java. We’ll walk through two Fibonacci Java algorithms to help you get started.
What is the Fibonacci Sequence?
You probably learned about the Fibonacci Sequence in high school math.
The first numbers in the Fibonacci Sequence are 0 and 1. Subsequent numbers are calculated by adding the two preceding numbers together. Let’s take a look at a longer list of the sequence:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55
This sequence can go on forever depending on how many numbers you want to calculate.
The Fibonacci Sequence can be implemented in two ways:
- Using an iterative function
- Using a recursive algorithm
We’ll walk through both of these approaches.
An Iterative Fibonacci Java Program
The iterative approach is the best place to start. Iterative programming is when you use a loop, such as a for loop, to iterate through a list and perform a task.
Iterative programming allows you to automate repetitive procedures. Because there is a clear formula for how to calculate the next number in the Fibonacci Sequence, we can use an iterative approach to implement the algorithm.
Let’s start by declaring a class and method for our program. We’ll also define three variables that we will use for our program.
public class FibonacciSequence { public static void main(String[] args) { int number = 5, firstTerm = 0, secondTerm = 1; } }
The variable “number” tracks how many terms we are going to calculate. “firstTerm” and “secondTerm” store the first and second values in the sequence, respectively. This will change to store the two items before the one that we’ve just calculated later in our program.
Now, let’s write a for loop that calculates the next Fibonacci numbers in the sequence:
for (int i = 0; i < number; ++i) { System.out.println(firstTerm); int nextNumber = firstTerm + secondTerm; firstTerm = secondTerm; secondTerm = nextNumber; }
This loop first prints out the value of firstTerm. In the first iteration, this value is 0. Next, the loop calculates the next number by adding firstTerm and secondTerm together.
Our code then assigns the value of firstTerm to the value of secondTerm. secondTerm becomes our next number.
Let’s run our code and see what happens:
0 1 1 2 3
Our code has calculated the first five values in the sequence.
A Recursive Fibonacci Java program
The Fibonacci Sequence can be calculated using a recursive algorithm. This is a function that calls itself to solve a problem. A recursive algorithm can be used because there is a consistent formula to use to calculate numbers in the Fibonacci Sequence.
Let’s start by initializing our class:
class FibonacciSequence { }
Next, we’ll write a function that uses recursion to calculate the next value in the sequence:
static void getNextValue(int number, int firstTerm, int secondTerm) { if (number > 0) { System.out.println(firstTerm); int nextNumber = firstTerm + secondTerm; firstTerm = secondTerm; secondTerm = nextNumber; getNextValue(number - 1, firstTerm, secondTerm); } }
This method adds the values of firstTerm and secondTerm to calculate the next value. This happens as long as the value of “number” is greater than 0. “number” tracks how many numbers are left to calculate in the sequence.
Once the next value has been calculated, the function getNextValue() is recursively called. This time, the value of “number” is reduced by one. This is because every time the function is run, a new number is calculated.
Let’s write a main program that uses our recursive function and declares the variables we’re going to use:
public static void main(String args[]) { int number = 5, firstTerm = 0, secondTerm = 1; getNextValue(number, firstTerm, secondTerm); }
“number” reflects the number of values we want to calculate. firstTerm is the first term in the list. secondTerm is the second term in the list.
When we call the getNextValue
method, our calculation begins. Let’s run our code to display Fibonacci numbers:
0 1 1 2 3
The first five values in the Fibonacci Sequence have been calculated!
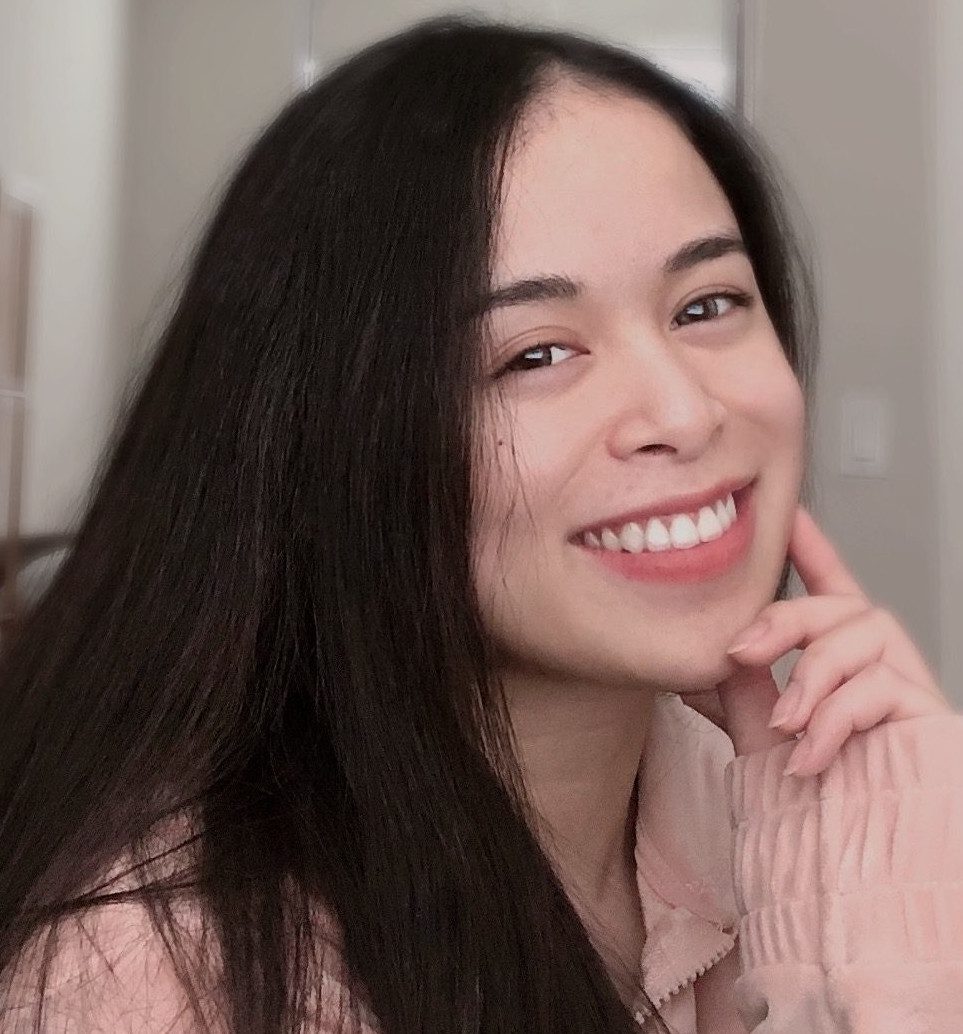
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
The Fibonacci Sequence is common in mathematics, computing, and nature. The next number in the sequence is calculated by adding the two preceding numbers together. The sequence starts with the numbers 0 and 1.
This sequence can be calculated using either an iterative or recursive approach. Now you are ready to calculate the Fibonacci series in Java.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.