When you’re designing a website, you may decide that you want to show additional information about something on the web page when the user hovers over an element on the site.
For instance, if you are designing a Frequently Asked Questions page, you may want additional information to appear about technical terms listed on the web page. This information should only appear when the user hovers over a technical term.
To show information about something when the user hovers over an element, you can create a tooltip. In this tutorial, we’ll discuss, with reference to an example, how to create a tooltip in CSS. By the end of reading this tutorial, you’ll be an expert at creating tooltips in CSS.
CSS Tooltips
Tooltips are used to add information about an element on a web page. Tooltips appear in a number of different places on websites, especially when the author of a site wants to clarify something or add a definition to a word in a block of text.
There is no default HTML element that is used to create a tooltip. So, we have to rely on a combination of HTML and CSS to design an element that appears like a tooltip.
Let’s start by writing the HTML code for our tooltip. In this example, we are going to create a tooltip for a recipe website. When the user hovers over the term prepare the baking tray
., a tooltip should appear with some text to remind the reader how to prepare the baking tray.
Here is the HTML code for our tooltip:
<html> <div style="text-align:center;"> <p>Instructions for baking:</p> <div class="tooltip">Prepare the baking tray. <span class="contents">Line tray with greaseproof paper.</span> <div> </div>
First, in our code, we have created a <div> tag with one style
attributes set. The style we have defined aligns the text in our <div> tag to the center of the element (text-align: center). Then, we use a <p> tag to create a paragraph containing the text Instructions for baking:
.
Next, we created a divider in which our tooltip will appear. This will show the text Prepare the baking tray
. Inside our <div> tag is a <span> tag which contains the text that we want to appear when the user hovers over the term Prepare the baking tray
.
Right now, our code shows all the text on-screen. This is because we have not applied any styles to our code yet to create a tooltip. Here are the styles we are going to use to create our CSS tooltip:
<html> <div style="text-align:center;"> <p>Instructions for baking:</p> <div class="tooltip">Prepare the baking tray. <span class="contents">Line tray with greaseproof paper.</span> <div> </div> <style> .tooltip { display: inline-block; position: relative; margin: 50px; } .tooltip .tooltiptext { visibility: hidden; width: 120px; background-color: lightblue; color: white; text-align: center; padding: 10px 0; border-radius: 5px; position: absolute; z-index: 1; } .tooltip:hover .contents { visibility: visible; }
Click the button in the code editor above to see the output of our HTML/CSS code.
In our code, we created a tooltip that is triggered when the user hovers over the text Prepare the baking tray
. This tooltip contains a brief reminder of how to prepare the baking tray for the reader of our website.
Let’s break down how our CSS code works, so you know how our data tooltip was created.
.tooltip Style
First, we created a style rule called .tooltip, which is applied to the <div> tag in our HTML code. This style defines the element as an inline-level block container, which means it starts on a new line.
We also set the position of the tooltip to relative, which means the tooltip will stay in the same place even if the page is scrolled. You can learn more about CSS positioning in our guide to CSS position.
Finally, we specified a 50px margin around our tooltip text, which allows us to create a space between our tooltip text and other elements on the web page. You can learn more about margins in our beginner’s guide to CSS margin.
.tooltip .contents Style
Next, we defined a style rule called .tooltip .contents, which is applied to any element with the class name contents
whose parent class has the name tooltip
.
For this example, this means this style rule is applied to our <span> tag. This rule contains the styles for our actual tooltip attribute.
In our .contents style, we specify the value of the visibility
property to hidden
. This means that, until otherwise stated, the tooltip is hidden from the user.
We then set the width (width) of the tooltip to 120px, background color (background-color) of the tooltip to light blue, and the text color (color) of the text in the tooltip to white. In addition, we aligned the text in the tooltip to the center of the element using text-align.
Toward the end of our .contents rule, we set a 10px padding, which creates a space between the text in our tooltip and its borders. We also set a border-radius of 15px, which gives our tooltip a rounded corners effect.
Next, we set the position value of the .contents element to absolute
, which means the .contents element is positioned relative to the parent element. In this case, the parent element in our HTML code is our <div> tag, which means our tooltip will always appear next to our <div> tag in our code.
.tooltip:hover .contents Style
Finally, we defined a rule called .tooltip:hover .contents. This rule is used to show the tooltip text when the user hovers their mouse over the element with the class name tooltip
.
When this rule is executed, the visibility of the .contents element is set to visible
. This means that when the user hovers over the tooltip, the tooltip will become visible on the web page.
Position the CSS Tooltip
In our above example, our tooltip appears to the right of our text when the user hovers over the label Prepare the baking tray
. However, we can customize this so that our tooltip appears on different edges of our text.
Right Position
Our code from earlier already positions our tooltip to the right of our text. However, if we have already styled our tooltip to appear in a different way, and want to move our tooltip back to the right position, we could use this code:
.tooltip .contents { top: -5px; left: 105%; }
This CSS rule, when combined with our code from earlier, positions the tooltip to the right of our text. The top: -5px rule aligns the tooltip with the center of its container element. The left: 105% rule places the tooltip to the right of the container because it offsets the position of the tooltip to the left by 105% of the width of the tooltip.
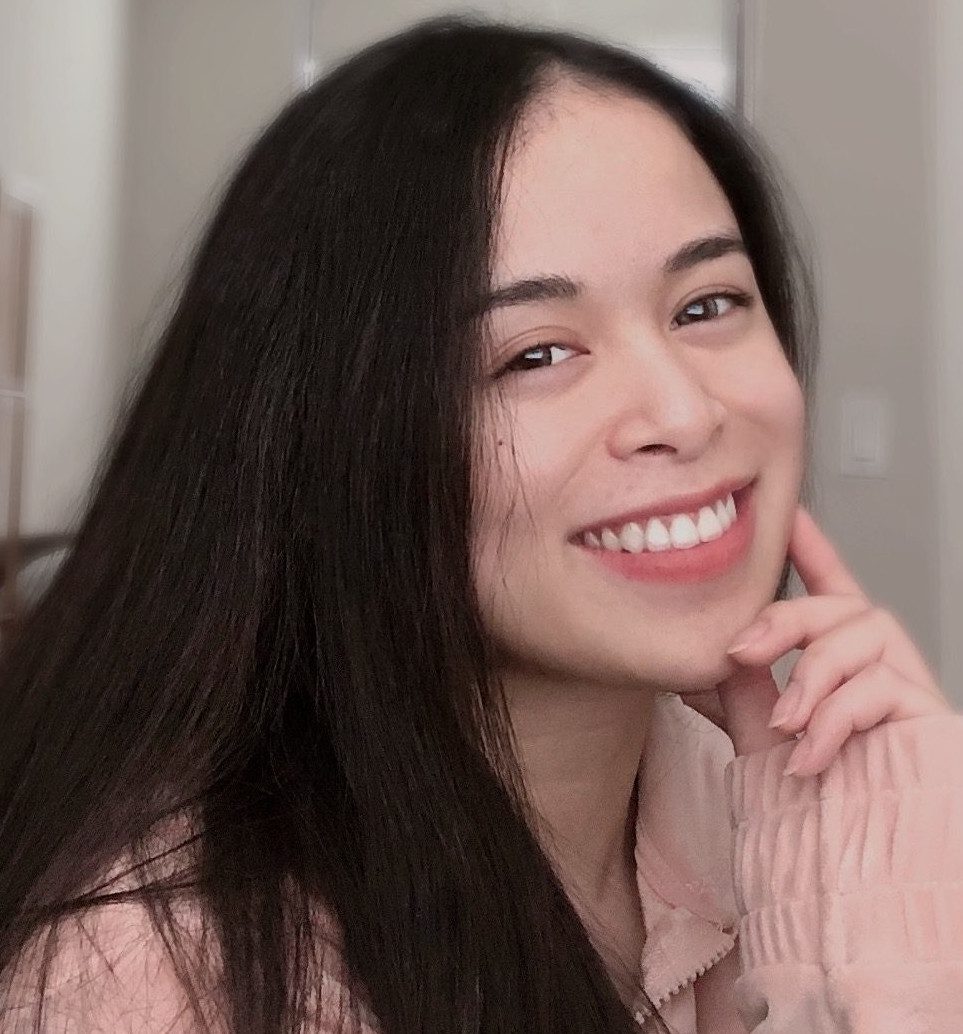
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Our code returns:
As you can see, our tooltip appears to the right of our text.
Left Position
Suppose we want our tooltip to appear to the left of our text. We could make this happen using the following code:
.tooltip .contents { top: -5px; right: 105%; }
This code, when added to our code from earlier, positions our tooltip to the left of our text. The top: -5px rule aligns the tooltip with the center of its container, as we discussed in the last example. The right: 105% rule allows us to place our tooltip to the left of the container (or, rather, offset the position of the tooltip to the right by 105% of the width of the tooltip).
Here’s what our code returns:
Our tooltip appears to the left of our text.
Top Position
Let’s say that we want our tooltip to appear above our text. We could accomplish this task using the following code:
.tooltip .contents { bottom: 100%; left: 50%; margin-left: -60px; }
Our code returns:
In this example, we used three CSS styles to position our tooltip above our text. The bottom: 100% and left: 50% styles allow us to offset our tooltip by 100% of the width of the tooltip label (Prepare the baking tray
) from the bottom and left of the tooltip label, respectively.
The margin-left style allows us to center our tooltip. Because the width of our tooltip is 120px, we have specified the margin-left value of -60px (half of 120px). This ensures our tooltip appears centered above our tooltip label.
Bottom Position
Suppose we want our tooltip to appear below the Prepare the baking tray
. label. We could do so using this code:
.tooltip .contents { top: 100%; left: 50%; margin-left: -60px; }
Our code returns:
Our code uses the same styles as our Top Position
example with one exception. In this example, we use the top: 100% style (instead of bottom: 100% like we used in the last example). This style allows us to offset our tooltip box by 100% of the width of the tooltip label (Prepare the baking tray
.), allowing us to position our tooltip box below the label.
Fade in Tooltips
When you’re designing a tooltip, you may decide that you want the tooltip text to fade in when the user hovers over the tooltip. This allows you to create a more aesthetically pleasing transition when the tooltip is set to appear on the user’s screen.
Here’s a style you can use to create a fade-in tooltip:
.tooltip .contents { opacity: 0; transition: opacity 2s; } .tooltip:hover .contents { opacity: 1; }
This style, when combined with the code from our first example, allows you to achieve a fade-in effect. Let’s break down how this works. First, we specify the opacity of our tooltip to 0. This means that the tooltip is hidden.
We then specify a transition property with two values: opacity and 2s. opacity
refers to the CSS property which we want to apply in our transition, and 2s
refers to the duration of our transition (in seconds).
Next, we create the .tooltip:hover .contents rule which sets the opacity of our tooltip to 1 when the user hovers over the tooltip.
This allows us to achieve an effect where, when the user hovers over the tooltip, the element will transition from being fully opaque (invisible) to fully visible. Because we specified our transition duration as 2s, this transition will take two seconds to complete.
If you’re interested in learning more about CSS transitions, read our guide to the CSS transition property.
Conclusion
Tooltips are used to display additional information on a web page. With help from HTML and CSS, you can design an aesthetically pleasing tooltip element that allows you to show additional information on a web page.
This tutorial explored, with reference to examples, how to create a tooltip in CSS, and how to position your tooltip to the left, right, bottom, and top of a label. We also discussed how to create a fade-in effect which is triggered when the user hovers over a tooltip label.
Now you have the knowledge you need to start designing your own CSS tooltips like a professional web designer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.