CSS selectors select the elements to which you can apply styles. The most basic selector is the element selector, which selects an element by its name. Other selectors include the id, class, universal, and descendant selectors.
Selectors are one of the two parts of any CSS statement. Selectors are used to target the elements on an HTML page for a certain style.
There are a wide range of CSS selectors that can be used to precisely target the elements on a web page, and allow you a high degree of customization when you are designing one.
This guide will explore the basics of selectors in CSS how you can leverage the most commonly-used selectors in your code.
CSS Selectors
CSS selectors identify the HTML elements to which you want to apply a CSS style. Selectors can choose elements based on their name, ID, class, and other attributes. Selectors appear before a pair of brackets in a CSS rule.
In CSS, rules are used to define the styles that should apply to an element or a range of elements. Rules have two components: selectors and declarations.
Here is an example of a rule in CSS:
h1 { color: pink; }
This CSS rule sets the color of all h1 elements on a web page to pink. The two components of our rule are:
- h1, which is the element to which our style is applied. This is called a selector.
- color: pink, which is the style applied to the elements in our selector. This is called a declaration. In this example, we change the color of all h1 elements to pink.
CSS selectors are a fundamental part of designing web styles. Selectors allow you to tell the browser which elements should use a particular style. For instance, you may have a selector that instructs the browser to style all <h1> elements, or all <p> elements, on a web page.
CSS Selector Types
CSS offers a wide range of selectors. Let us explore an example of the main selectors you are likely to encounter as you design CSS rules.
CSS Element-Based Selector
The element-based selector allows you to apply a style to all instances of an element in a document to which a rule corresponds. For instance, you can use the element selector to apply a style to all <p> tags on a page.
Suppose we wanted to change the color of all the paragraph-based text on a web page to gray. We could do so using this code:
p { color: gray; }
The declarations inside our “p” selector will be applied to every paragraph (HTML <p> tag) on our web page.
CSS id Selector
The CSS id selector selects a HTML element on a page based on its ID. HTML IDs are unique so the id selector is used to apply styles to a single element on a web page. The syntax for the ID selector is a hash sign followed by the element ID.
Suppose we want to set the value of the CSS height attribute of an element called “box9” on our page to 150px. We could do so using this code:
#box9 { height: 150px; }
This rule will set the height of the element with the id “box9” to 150px.
CSS Class Selectors
CSS class selectors find the elements who have a particular class attribute. The syntax of the class selector is a period (.) and then the name of the class you want to select.
So, if you wanted to target the class “boxMiddle”, you would use the selector “.boxMiddle”.
Let us say we are designing a box on our web page. The box should have a pink background color. Our box has been assigned the class “pinkBox”, to which we can apply our styles. Here is the code we would use to set the color of elements with the pinkBox class to pink:
.pinkBox { color: pink; }
Every element on a web page with the class “pinkBox” will be set to pink.
In addition, you can also specify that only specific elements with a particular class should be affected by a style. By using only a class selector, every element with a particular class will be affected by the style you define.
If we use a class selector preceded by an element type, we can apply a style only to specific elements with a certain class. Suppose we want the background color of every HTML <div> tag with the class “pinkBox” to be set to pink. The style for this would be as follows:
div.pinkBox { color: pink; }
This style will only be applied to every <div> tag with the class “pinkBox”.
CSS Universal Selector
The CSS universal selector selects all elements on a web page. The selector is denotes using an asterisk (*), followed by no element names. It is often used to set margins or default fonts for a web page.
Suppose we wanted all elements on a web page to be aligned to the center of the page. We could use the following code to apply this style to our web page:
* { text-align: center; }
This rule applies the “text-align: center;” style to every item on a web page.
CSS Descendant Selectors
CSS descendant selectors select an element that is a descendant of another element to which a range of styles should be applied. The syntax is to specify an element, followed by a space, followed by the descendant element you want to select.
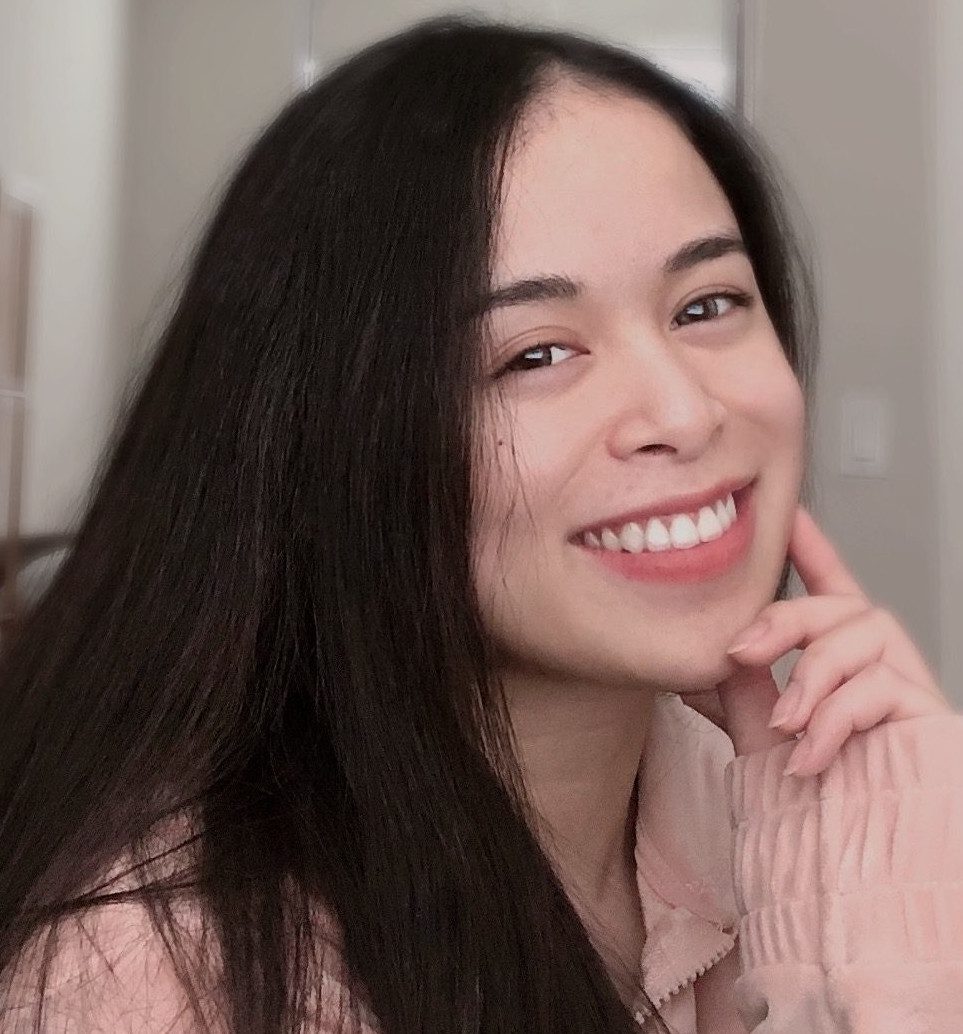
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Suppose you want to set the background color of all links in a list to yellow. However, you do not want this style to affect any other links on the web page. You could do so using this code:
li a { background-color: yellow; }
This style uses the “li a” descendant selector. This means the style we have created will only be applied to all <a> tags in an HTML <li> tag. Our style will not apply to any other <a> tags that appear on our web page.
CSS Selector Lists
If you have more than one element to which a specific style should be applied, you can use a selector list. Selector lists apply a rule to all the individual selectors in a list.
Suppose we have two CSS rules with the same style rules. These are as follows:
h2 { text-align: center; } .alignCenter { text-align: center; }
We could make our code easier to read by combining these two rules into a selector list. We can do so by adding a comma between our styles:
h2, .alignCenter { text-align: center; }
This code functions in the same way as our first example. However, our second example uses a selector list which makes our code more concise.
CSS Grouping Selectors
The CSS grouping selector applies a set of styles to multiple selectors. It is used to reduce repetition and help you write clearer code. The syntax is a lits of element selectors separated by commas, followed by the CSS rules you want to apply to the elements.
Let us suppose we are designing a web page that uses the following styles:
h1 { text-align: center; font-size: 32px; font-weight: normal; } h2 { text-align: center; font-size: 24px; font-weight: normal; } h3 { text-align: center; font-size 18px; font-weight: normal; }
In this code, the “font-weight: normal;” and “text-align: center;” styles are shared by all selectors. To reduce repetition in our code, we could group these styles together using a grouping selector. Here is the code we would use to accomplish this task:
h1, h2, h3 { text-align: center; font-weight: normal; } h1 { font-size: 32px; } h2 { font-size: 24px; } h3 { font-size: 18px; }
We have grouped together two of the styles, text-align and font-weight, which were the same throughout every tag. This has allowed us to make our code easier to read.
Conclusion
CSS selectors allow you to apply a style to a particular element or range of elements on a web page. There are a wide range of selectors offered by CSS. These include id selectors, class selectors, and grouping selectors, which you can use to create precise CSS styles.
This article only scratched the surface of selectors in CSS. There are also attribute selectors, pseudo-classes and pseudo-elements, combinators, and other selectors which are used in CSS. If you are looking to develop more advanced rules, you may want to research these selectors in more depth.
This tutorial discussed, with reference to examples, how to use the most commonly-used selectors in CSS. Now you have the knowledge you need to start using CSS selectors to create styles like an expert.
For advice on top CSS learning resources, courses, and books, check out our How to Learn CSS guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.