When you’re designing a web page, you may want an element to have rounded corners. For instance, if you’re adding a headshot of a business owner to a web page, you may want the image to have rounded corners to make it more aesthetically pleasing.
That’s where the CSS border-radius property comes in. You can use the border-radius property to add rounded corners to any element in CSS.
This tutorial will discuss, with a few examples, how to use the border-radius property to add rounded corners to an element in CSS.
CSS Border Radius
Borders are the lines surrounding the outer edge of a web element.
In CSS, the border property is used to specify how a border should appear on a web page. If you’re interested in learning more about how to create borders in CSS, read our guide on CSS borders.
CSS Set Individual Rounded Corners
The border-radius property is shorthand for four subproperties used to set the border radius of each corner. These subproperties are:
- border-top-left-radius
- border-top-right-radius
- border-bottom-right-radius
- border-bottom-left-radius
These properties are used to set the border around a particular corner of a web element. Each element accepts either a length value using px, em, another length measurement, or a percentage value (%).
Let’s explore an example to illustrate how these properties work.
We have been tasked with designing a box that has a rounded top left and bottom right corner. Our box should have a 3px solid light blue border. The top left corner should be rounded by 20px and the bottom right corner should be rounded by 10px. We could use the following code to create this box:
index.html <div class="box"> <p>This is a box.</p> </div>
styles.css .box { border: 3px solid lightblue; border-top-left-radius: 20px; border-bottom-right-radius: 10px; }
Our code returns:
In our HTML code, we have created a box using a <div> tag. This box contains a <p> tag in which the text This is a box
. is stored. Our <div> tag is assigned the class box
, which means the CSS styles we apply to the box
class will be applied to the <div> tag.
In our CSS file, we have defined that any element with the box
class should have:
- A 3px-wide solid light blue border.
- A top left corner that is rounded by 20px.
- A bottom right corner that is rounded by 10px.
If you look at the image above, you can see our top left and bottom right corners are rounded. The top left corner has been rounded by 20px, and our bottom right corner has been rounded by 10px.
CSS Border Radius Shorthand
The border-radius property is shorthand for the four subproperties we discussed earlier.
Using the border-radius property, we can define rounded corners for a web element using one line of code instead of defining each corner, individually, using a separate property.
The syntax for the border-radius property depends on how many values you specify. Let’s explore an example of each of the potential ways in which you can use the border-radius property to add rounded corners to an element.
One Value
If you want to apply the same border radius to every corner of an element, you only need to specify one value when using the border-radius property.
Suppose you want to create a box with a 20px border radius around all corners. You could do so using this code:
.box { border-radius: 20px; border: 3px solid lightblue; height: 200px; width: 200px; }
Our code returns:
In this example, we have created a box that is 200px tall by 200px wide. Our box has a 3px solid light blue border. We have also used the border-radius property to add a 20px rounding effect to each corner in our box.
Two Values
If you are creating a box which should have the same rounding for the top left and bottom right corners, and a different set of roundings for the top right and bottom left corners, you can specify two values with the border-radius property.
The order in which the rounding values you specify are applied is:
- The first value is the amount by which you want the top left and bottom right corners of a box to be rounded.
- The second value is the amount by which you want the top right and bottom left corners of a box to be rounded.
Suppose you wanted the top left and bottom right corners of a box to be rounded by 20px, and the bottom left and top right corners to be rounded by 10px. You could create this box using the following code:
.box { border-radius: 20px 10px; border: 3px solid lightblue; height: 200px; width: 200px; }
Our code returns:
In our example, we have specified two values with the border-radius property. The first value is used to set the corner edge rounding for the top left and bottom right corners to 20px. The second value is used to set the corner edge rounding for the bottom left and top right corners to 10px.
Three Values
If you want to assign a box rounded corners where the top left corner has its own rounding, the top right and bottom left corners have their own rounding, and the bottom right corner has its own rounding, you can specify three values with the border-radius property.
The order in which the three values you specify apply to a box is as follows:
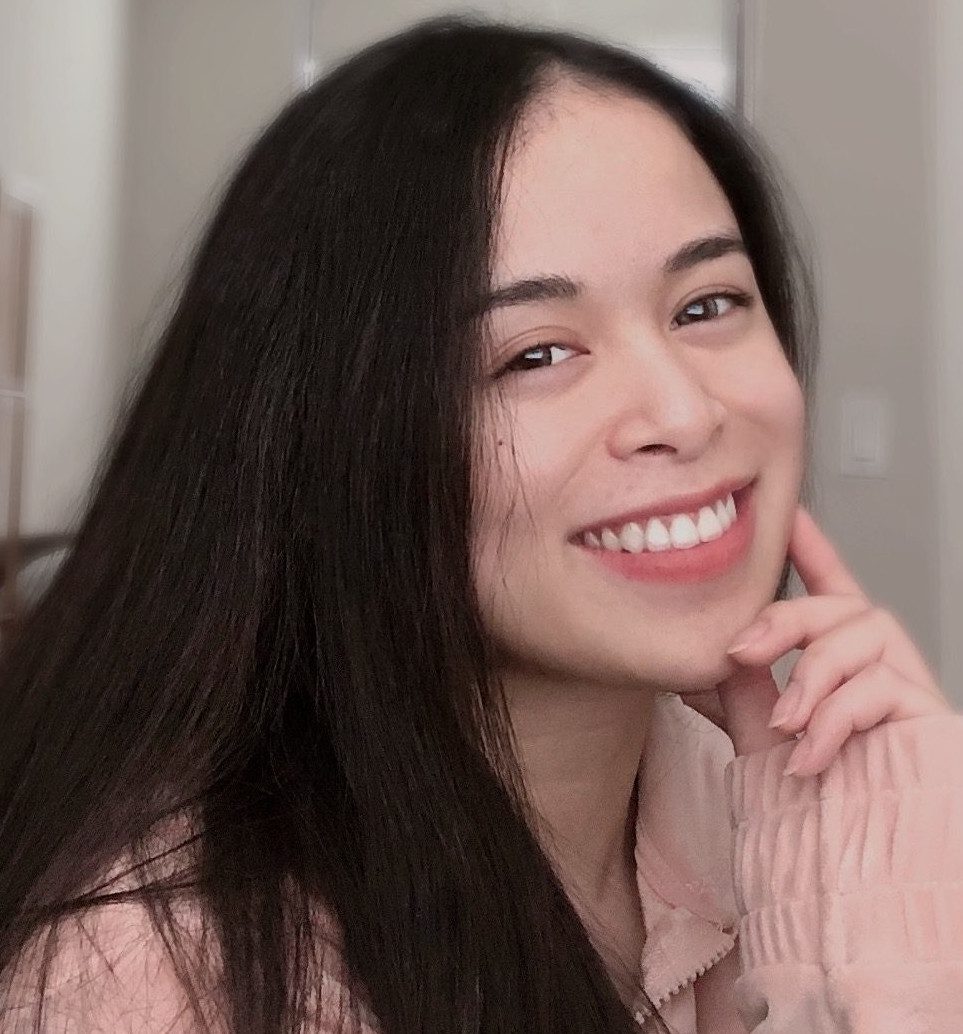
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
- The first value is the rounding for the top left corner.
- The second value is the rounding for the top right and bottom left corners.
- The third value is the rounding for the bottom right corner.
Suppose we are creating a box that should have the following roundings:
- The top left corner should be rounded by 30px.
- The top right and bottom left corners should be rounded by 20px.
- The bottom right corner should be rounded by 10px.
In the below example, we create a box that uses these corner roundings. We also apply a light blue border around our box and set the size of the box to be 200px wide by 200px long. Here is the code we could use to create our box:
.box { border-radius: 30px 20px 10px; border: 3px solid lightblue; height: 200px; width: 200px; }
Our code returns:
The top right and bottom left corners both have the same rounding. The top left corner has its own rounding, and the bottom right corner also has its own rounding.
Four Values
Let’s say you are creating a box whose corners should be rounded using four different values. You could round the corners of the box by specifying four values with the border-radius property. The values you specify will be interpreted in the following order:
- The first value applies to the top left corner.
- The second value applies to the top right corner.
- The third value applies to the bottom right corner.
- The fourth value applies to the bottom left corner.
Suppose we want to create a box that has a 5px rounding on the top left corner, a 10px rounding on the top right corner, a 15px rounding on the bottom right corner, and a 20px rounding on the bottom left corner. Our box should be 200px wide by 200px long, and should have a 3px-wide solid light blue border.
We could create this box using the following code:
.box { border-radius: 5px 10px 15px 20px; border: 3px solid lightblue; height: 200px; width: 200px; }
Our code returns:
In the above image, you can see that each corner has its own rounding value, based on the values we specified in our code.
Elliptical Corners
In our examples above, we have used border-radius to create rounded corners. We can also use the border-radius property to create elliptical borders.
We can create elliptical corners by adding a slash between two values for the element’s corners. Here’s the syntax for creating an elliptical corner:
border-radius: horizontal radius / vertical radius;
Suppose we wanted to create a 25% elliptical corner around the horizontal corners of a box and a 50% elliptical corner around the vertical corners of a box. Our box should be 200px wide by 200px long box and have a light blue border. We could do so using this code:
.box { border-radius: 25% / 30%; border: 3px solid lightblue; height: 200px; width: 200px; }
Our code returns:
As you can see, we have created a shape with elliptical rounded corners.
Conclusion
The border-radius property is used to add rounded corners to an element in CSS. The border-radius property is shorthand for the four subproperties used to add rounded corners to each corner of a web element.
Accompanied by a few examples, this tutorial discussed how to use the border-radius property and its four subproperties to create rounded corners in CSS. Now you’re ready to start using the border-radius property to create rounded corners like an expert.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.