Handling overflowing content is an important feature of good web design.
When you’re designing an element on a web page, there may be a scenario where the contents of the element cannot fit within its borders. This may cause your web page to render in an unexpected way. That’s where the CSS overflow property comes in.
The overflow property is used to handle instances where the content of an element cannot fit within its border. With reference to examples, this tutorial will walk through how to use the CSS overflow property in your code.
CSS Overflow
The overflow property allows you to specify what happens when a piece of content is too big to fit into the space assigned to that element.
For instance, suppose you have defined a <div> tag in which a paragraph of text is set to appear. This <div> tag is only 250px tall by 500px wide, and there is too much text to fit into the space specified for the paragraph.
You could use the overflow property to handle this problem and ensure that your content appears correctly on the user’s screen. By specifying an overflow property, you can mitigate the chance that the content in a web element appears improperly.
The overflow property has four potential values. These are:
- visible. The overflow in an element is not clipped, and the content renders outside the element’s box. This is the default value for the overflow property.
- scroll. The overflow in an element is clipped, and a scrollbar is created to allow the user to view the rest of the content in an element.
- hidden. The overflow in an element is clipped, and the rest of the content will be hidden from the user.
- auto. Auto adds scrollbars to see the rest of the content in an element, but only when necessary.
The overflow property can only be applied to block-level elements that have been assigned a specific height using the “height” attribute.
CSS Overflow Examples
Let’s walk through an example of each of the potential values that can be used with the overflow property. We’ll start with the overflow: visible property.
Visible Overflow
The visible value is the default overflow set for a web element. Elements with the visible value are not clipped and their contents appear outside the element’s box.
Suppose we are designing a box that includes a few lines of text. If the text we specify is too large for the box, we want it to render outside of the box. We could use the following code to accomplish this task:
index.html <div>This is a box with some text. We are using this box to illustrate what happens when you use the CSS overflow property and assign it the value "visible".</div> styles.css div { width: 200px; height: 100px; background-color: lightgray; overflow: visible; }
Our code returns:
[Code result here]
Let’s break down our code. In our code, we have created a box that is 200px wide by 100px tall. Our box has a light gray background color. These styles are applied to the <div> tag in your code.
In addition, we used the content overflow: visible
property to instruct our code that, if the text in our <div> tag cannot fit into the element, the text should render outside the element’s box. As you can see, this has resulted in some text overflowing
outside the box.
The overflow visible value, while useful, may not be as appropriate as other values for the overflow property. This is because in most cases you do not want the contents of an element to overflow, because the overflowing contents could interfere with the other styles you have created for a web page.
Scroll Overflow
The scroll value allows you to clip the overflowing contents of a web element. Then, a scroll bar will be added to the element so the user can see the overflowing contents.
The scroll overflow value adds both a vertical and a horizontal scroll bar to a web element, even if you do not need one of these scroll bars.
Suppose we are designing a box and we want any overflowing text to stay inside our box. If any overflowing text is present, a scroll bar should appear so the user can navigate their way through the box.
Here’s the code we could use to design our box:
index.html <div>This is a box with some text. We are using this box to illustrate what happens when you use the CSS overflow property and assign it the value "scroll".</div> styles.css div { width: 200px; height: 100px; background-color: lightgray; overflow: scroll; }
Our code returns:
[Code result here]
In our code, we created a box 200px wide by 100px tall. Our box has a light gray background color.
Further, we set the overflow property to be equal to “scroll”. As you can see, because there is too much text to fit into our box, a scroll bar appears and our overflowing text remains within the borders of our box.
Auto Overflow
We mentioned in the last section that the scroll property adds both horizontal and vertical scroll bars to a web element, even if one of these scroll bars is not necessary.
This can be useful in many cases, but when you’re designing a web element you may only want the relevant scroll bar to appear. To do so, you can use the auto value.
The auto value works in a similar way to the scroll value, but it only adds scroll bars to a web element when it is necessary. Suppose we have a box of text and we only want to add scroll bars to the box when necessary. We could do so using this code:
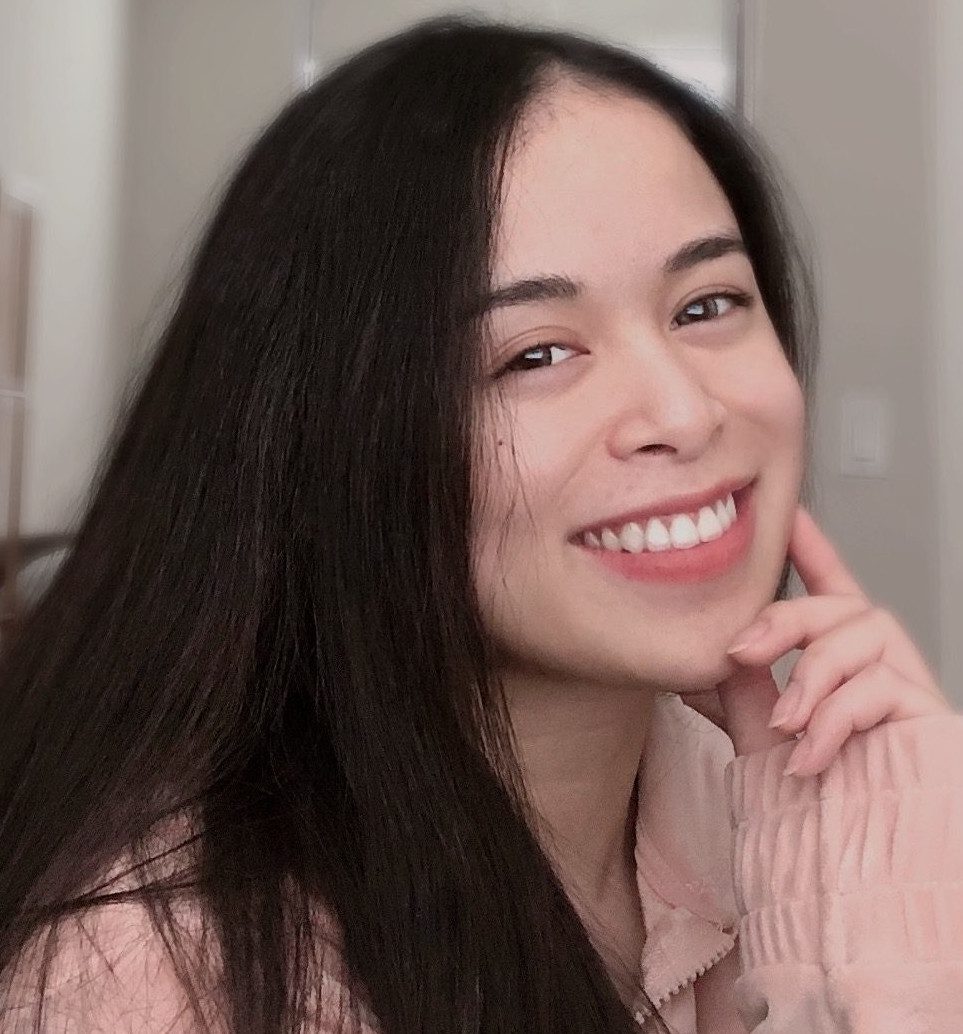
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
index.html <div>This is a box with some text. We are using this box to illustrate what happens when you use the CSS overflow property and assign it the value "auto".</div> styles.css div { width: 200px; height: 100px; background-color: lightgray; overflow: auto; }
Our code returns:
[Code result here]
In our above example, we have created a 200px wide by 100px tall box with a light gray background. We then set the value of the overflow property to auto. As you can see, in our final result only one scroll bar is showing. This is because only one scroll bar is necessary to show the overflowing contents in our box of text.
Hidden Overflow
You may decide that you want any overflowing contents in an element to be clipped, then hidden from the user. This is accomplished using the hidden overflow value.
Suppose you have a box of text whose contents you want to hide if they overflow outside the element. You could use the following code to create this box:
index.html <div>This is a box with some text. We are using this box to illustrate what happens when you use the CSS overflow property and assign it the value "hidden".</div> styles.css div { width: 200px; height: 100px; background-color: lightgray; overflow: hidden; }
Our code returns:
[Code result here]
Unlike in our previous examples, the overflowing text from our box is hidden from the user. This is because we set the value of the overflow property to hidden
in our code.
overflow-x and overflow-y
The overflow-x and overflow-y properties allow you to change how the overflow of content is handled on each axis individually.
The overflow-x property specifies how the overflow on the left and right edges of a box should be handled, and the overflow-y property specifies how the overflow on the top and bottom edges of a box should be handled.
Suppose you are designing a box and you want to set two different overflow values for the x and y-axis. The x-axis should use the hidden
overflow value, and the y-axis should use the scroll
overflow value. You could use the following code to accomplish this task:
index.html <div>This is a box with some text. We are using this box to illustrate what happens when you use the CSS x-overflow and y-overflow properties to set overflow values for the individual axes in a web element.</div> styles.css div { width: 200px; height: 100px; background-color: lightgray; overflow-x: hidden; overflow-y: vertical; }
Our code returns:
[Code result here]
As you can see, our box has no horizontal scroll bar. This is because we set the value of overflow-x to “hidden”. However, our box does have a vertical scroll bar, which appears because we set the value of overflow-y to “scroll”.
Conclusion
The CSS overflow property specifies how the contents of an element should appear if the element’s contents are too big to fit in the assigned area on the web page.
This tutorial explored, with reference to a few examples, how to use the CSS overflow property and its four values to control overflow in web elements. Now you’re ready to start using the CSS overflow property like an expert developer.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.