When you’re designing a web element, you may decide that you want an outline to appear around the element on the page. For instance, if you are designing a box that you want to highlight, you may want to add a colored outline around the box to draw attention to the element.
That’s where the CSS outline property comes in. The outline property allows you to add a line outside the borders of an element to make the element stand out on the web page.
This tutorial will discuss, with examples, the basics of outlines and how to use the CSS outline property to draw a line outside the borders of a web element. By the end of reading this tutorial, you’ll be an expert at drawing outlines using the CSS outline property.
CSS Outline
An outline is a line drawn around an element, outside of its border. The most common use case of the outline property is to highlight an HTML element.
Here is a graphic representation of how outlines are applied in CSS:
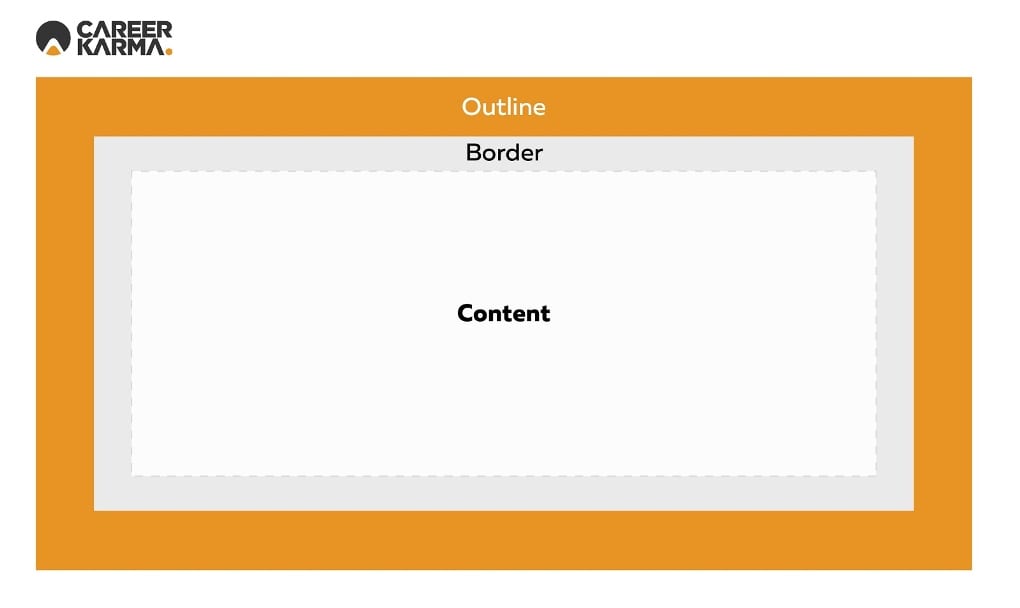
This graphic demonstrates that outlines appear both outside the content and border of a web element. You can see that the outline is the outermost layer of a web element.
There are a few differences between a border and an outline in CSS.
First, as we discussed, the outline is drawn outside of the element (unlike a border). This means that the outline we declare may overlap other content on a web page. However, outlines do not take up any space, and so they do not have any impact on surrounding elements aside from being able to overlap an element.
In addition, the outline is not part of an element’s dimensions. This means that the outline you specify will have no effect on the height and the width values of an element.
Outlines, unlike borders, also cannot have a different border width, color, or style for each edge. So, the outline you specify around an element will appear consistent across all edges.
If you’re looking to learn more about borders in CSS, read our guide to CSS borders.
CSS Outline Property
The outline property is used to add an outline to an element on a web page.
The outline property is shorthand for four sub-properties which allow you to customize how an outline appears on a web page. These sub-properties are:
- outline-color: Sets the color of the outline
- outline-style: Sets the style of the outline
- outline-width: Sets the width of the outline
The syntax for the outline property is as follows:
outline: outline-color outline-style outline-width;
The values specified with the outline property do not need to appear in any particular order.
Let’s walk through an example to illustrate how the outline property works. Suppose we want to create an outline around a box that contains the text This is a box
. Our outline should have the following characteristics:
- The outline should be red.
- The outline should be 3px thick.
- The outline should be dotted.
We also want our outline to have a 1px-thick black border. We could create this outline and border using the following code:
<html> <p class="outlineExample">This is a box.</p> </html> <style> .outlineExample { width: 100px; border: black solid 1px; outline: red dotted 3px; } </style>
Click the button in the code editor above to see the output of our HTML/CSS code.
In our code, we declared both an outline, which appears in red, and a border, which appears in black. The border is 1px-thick and appears in the solid style, and the outline is red, 3px-thick, and appears in the dotted style. We also set the width of our box to be 100px.
As you can see, the outline appears outside the border in our web element.
CSS Outline Sub-properties
The CSS outline property is shorthand for four sub-properties, as we discussed earlier. Let’s explore each of these sub-properties individually to explain how they work.
CSS Outline Width
The outline-width property specifies the width of an outline applied to a web element.
The outline-width property accepts any of the following length values:
- thin (by default, 1px)
- medium (by default, 3px)
- thick (by default, 5px)
- A size specified in px, pt, cm, em, rem, or another CSS length metric
Suppose we want to design a box with a 3px (medium) blue, solid outline. We also want our box to have a 1px-wide solid, black border. We could create this box using the following code:
<html> <p class="outlineExample">This is a box.</p> </html> <style> .outlineExample { width: 100px; border: black solid 1px; outline-style: solid; outline-color: red; outline-width: medium; } <style>
Click the button in the code editor above to see the output of our HTML/CSS code.
Let’s break down this example. In our CSS code, we set the width of our box to 100px and defined a 1px-wide solid black border around our box.
Then, we used three sub-properties to define our border.
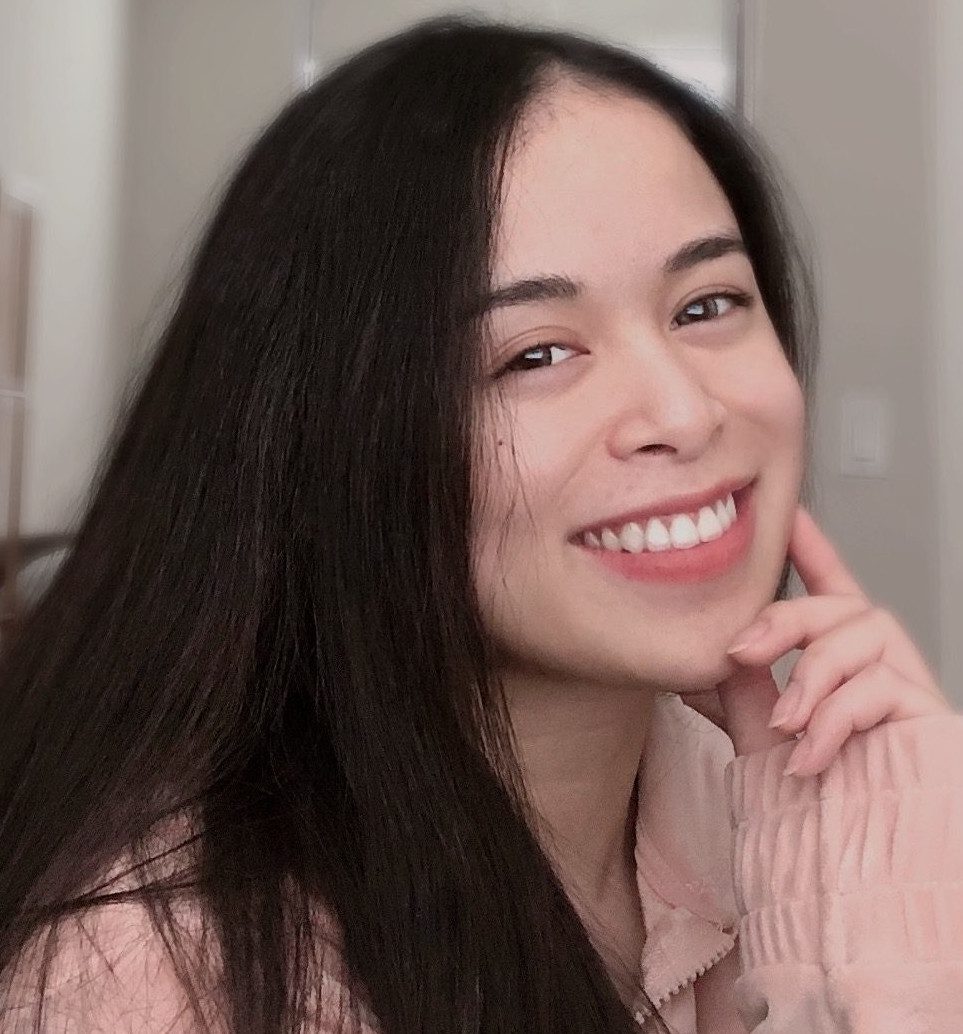
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
The outline-style property is used to set the style of our outline, which is solid in this case. The outline-color property is used to set the color of our outline, which is red in this example. (We discuss the outline-style and outline-color sub-properties in more depth later in this guide).
The outline-width property is used to set the width of our outline, which we set to medium
(which is 3px by default).
As you can see, our box has a 3px-wide red outline.
CSS Outline Color
The outline-color property allows you to set the color of the outline assigned to a particular web element. The property accepts any of the following CSS color types:
- name: A color name, such as
blue
orpink
- hex: A hexadecimal color, such as
#f7f7f7
- rgb: An RGB value, such as
rgb(102, 211, 56)
- invert: This inverts the color of the outline
- hsl: A HSL value, such as
hsl(0, 25%, 50%)
Suppose we want to design an outline around a box that appears around our box in blue. Our outline should be 3px thick and use the solid style. We could create a box with this outline using the following code:
<html> <p class="outlineExample">This is a box.</p> <html> <style> .outlineExample { width: 100px; border: black solid 1px; outline-style: solid; outline-color: blue; outline-width: medium; } <style>
Click the button in the code editor above to see the output of our HTML/CSS code.
In this example, we used the outline color property to set the color of our outline to blue. We also used the outline-style property to set the style of our outline to solid, and the outline-width property to set the style of the width of our outline to 3px (or medium
).
In our code, we also made our box 100px wide, and set a 1px solid black border around our box, which makes it easier to see our outline.
CSS Outline Style
The outline-style property allows you to set the style of your outline.
This property accepts the following values:
- dotted
- dashed
- groove
- ridge
- solid
- double
- inset
- outset
- none
- hidden
So, the ridge
value creates a ridge outline, the inset
value creates an inset outline, the groove
value creates a groove outline, and so on. To illustrate how these outlines appear, let’s create a box with an outline and apply each of these outline styles to the box individually. Here’s the basic code for our box:
<html> <p class="outlineExample">This is a box.</p> <html> <style> .outlineExample { width: 300px; border: black solid 1px; outline-color: blue; outline-width: medium; } <style>
Click the button in the code editor above to see the output of our HTML/CSS code.
This code creates a box with a blue outline that is 3px wide (using the medium
value to indicate the width of the outline). The box has a black solid border that is 1px-wide, and the box is 200px wide.
To set the style of our box, we should use the outline-style property. So, if we wanted to have a box with a double
outline style, we could use this CSS style:
<style> .outlineExample { outline-style: dotted; } <style>
Here is a graphic that shows the results of this property being used with all the potential values accepted by outline-style:
CSS Outline Offset
The outline-offset property adds space between an outline and the border of an element.
The space between an element’s outline and border defined by the outline-offset property is transparent. In addition, the outline-offset property is standalone, and cannot be used in the outline shorthand.
Suppose we want to design a box with a 1px-wide solid black border and a 2px-wide solid red outline. The outline should be offset by 10px outside the edge of our border. We could create a box with these styles using the following code:
<html> <p class="outlineExample">This is a box.</p> <html> <style> .outlineExample { width: 200px; border: black solid 1px; outline: red 2px solid; outline-offset: 10px; } <style>
Click the button in the code editor above to see the output of our HTML/CSS code.
In this example, we defined a box that is 200px wide, has a 1px-wide solid black border, and a 2px-wide red solid outline. The outline for our box is offset by 10px, which means there is a 10px gap between each border edge and the outline.
Conclusion
The CSS outline property is used to define outlines around the borders of a web element.
The outline property is shorthand for three sub-properties used to create custom outlines: outline-color, outline-width, and outline-style. In addition, the outline-offset property is used to add space between an outline and the border of an element.
This tutorial discussed, with reference to examples, the basics of outlines in CSS, how to use the outline property and its sub-properties, and how to use the outline-offset property. Now you’re equipped with the knowledge you need to start designing outlines like a CSS pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.