Designing websites that are compatible across different devices is an important step in ensuring that a website is accessible to as many different users as possible.
That’s where media queries come in. CSS media queries allow you to apply a CSS rule only when the browser matches a rule that you have defined. So, you could define a rule that applies only if a user is viewing a web page on a smartphone.
This tutorial will discuss, with examples, the basics of responsive web design, how media queries work, and how to design your own CSS media queries. By the end of reading this tutorial, you’ll be an expert at designing media queries in CSS.
Responsive Web Design and Media Queries
Responsive web design refers to a set of practices where a developer optimizes a style sheet on a site so that the site can be rendered correctly on a wide range of different devices and screen sizes.
The reason that responsive web design is so important is that the output device a viewer uses to look at a web page—and its browser window and screen size—will impact how the web page loads. If a website is not designed with different devices and screen sizes in mind, then some users may struggle to view all the content that is presented on the website.
While responsive web design encompasses a wide range of practices—from using flexbox to relative styles—media queries are a crucial part of any website that is designed with web responsiveness in mind.
Media queries allow you to adjust how styles appear on a web page depending on the type of device used, the orientation of a device, and the viewport size.
By using media queries, you can make elements appear differently on your website based on the different screens and devices through which a user is viewing your site.
Media Query Syntax
To declare a media query in CSS, you need to use the @media
rule. This rule allows you to execute a block of CSS properties only if a certain condition evaluates to true.
Here is the basic syntax for a CSS media query:
@media media-type and (media-rule) { // CSS styles }
Here are the main components of this media query:
- @media instructs the browser to create a media query.
- media-type is the type of media the code will be used for (i.e. screen or print).
- and is a logical operator that we need to use when specifying both a media type and a media rule.
- media-rule is the statement that must evaluate to true in order for the CSS in the media query to execute.
- CSS styles are the rules that will be applied to a web element if the media-rule evaluates to true.
There are four media types that can be used with a media query. These are all, print, screen, and speech. By default, the value all
is used, and multiple media types can be specified by separating each type using a comma.
CSS Media Rules
There are a few rule types that can be applied to a media query. Let’s break down each of these individually.
Width and Height
The main rule types used with CSS media rules are device width and height. These rules allow us to apply styles to a web element depending on the size of the viewport container. The term viewport container
refers to the area of a web page visible to a user.
The width and height rules are used to apply a style if the viewport is exactly equal to a certain size. So, if you wanted to change the text color of all <p> tags on a web page to blue if the viewport is 500px tall, you could do so using this rule:
@media screen and (width: 500px) { p { color: blue; } }
This rule sets the text color of all <p> tags to blue
if the width of the viewport is exactly 500px.
In most cases, though, you will want to apply a media query using ranges. So, for example, you may want to change the font size of a web page if the viewport size is equal to or greater than 500px. That’s where the min-width and min-height styles come in.
If we wanted to change the text color of all <p> elements to blue if the viewport width is equal to greater than 500px, we could use the following rule:
@media screen and (min-width: 500px) { p { color: blue; } }
This rule sets the text color of all <p> elements to blue, but only if the viewport width is equal to or greater than 500px. The way this media query works is the condition min-width: 500px is evaluated, which checks whether the viewport width is equal to or greater than 500px. If this condition evaluates to true, the rules in our p
style are applied to the web page.
Orientation
In addition, media queries can be used to apply styles to a web page depending on the orientation of a device.
Suppose we want to set the text size of all h2 elements to 20px if the user is viewing our web page in landscape mode. We could do so using this code:
@media (orientation: landscape) { h2 { font-size: 20px; } }
This rule checks if the user is viewing the web page in landscape mode, and sets the font size of all <h2> elements to 20px if that condition evaluates to true.
By default, desktops use landscape orientation.
CSS Media Query Examples
Let’s walk through two example media queries to illustrate how you can write your own media queries in CSS.
Basic Media Query Example: Hide Element
Suppose we are designing a website and we want to have a box appear that shows This site is in beta
. on all mobile devices. This box should appear only on mobile devices because we are still testing our mobile experience.
We could create this box using the following code:
<html> <p class="betaBox">This site is in beta.</p> <html> <style> .betaBox { background-color: orange; padding: 10px; } <style> @media screen and (max-width: 600px) { .betaBox { display: none; } }
Our code returns:
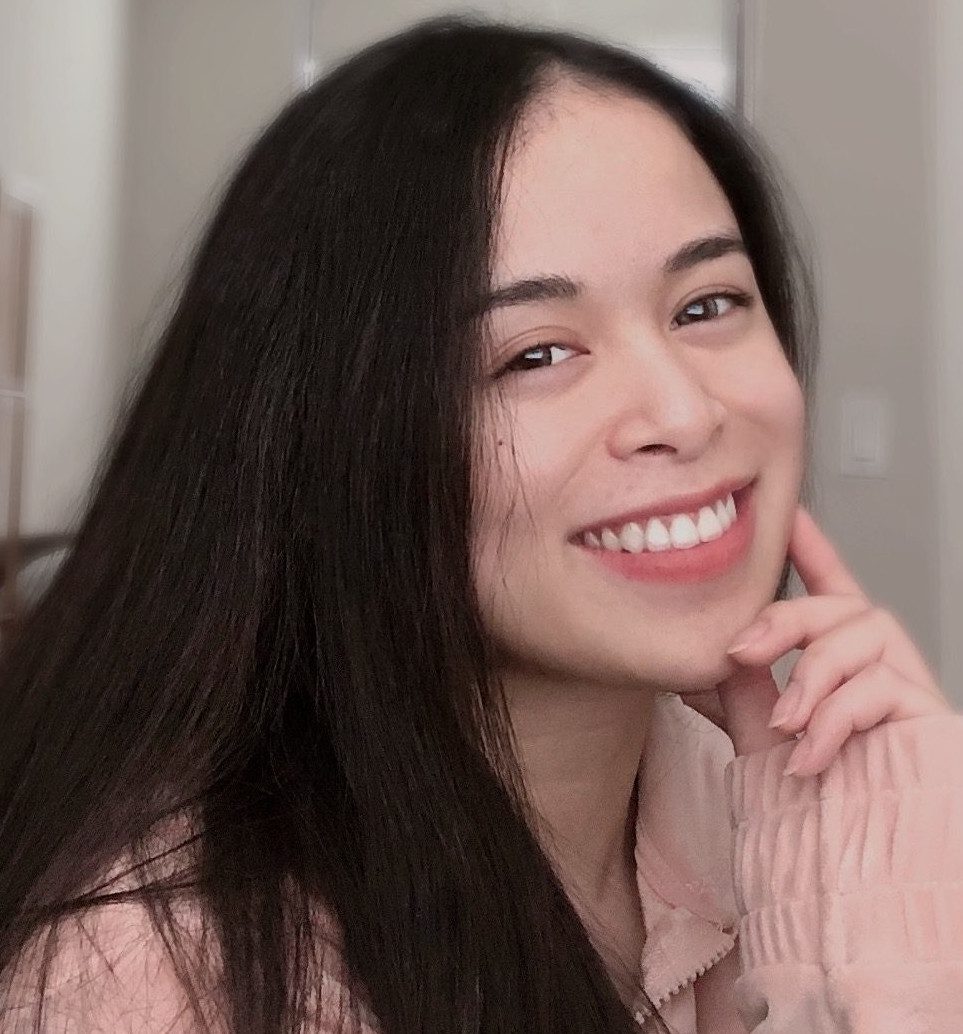
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot

Let’s break down our code. In our HTML file, we have defined a <p> tag which includes the text This site is in beta
. The class assigned to the <p> tag is betaBox
.
In our CSS file, we specified two styles. The .betaBox style sets the background color of any element with the class name betaBox
to orange and creates a 10px padding space between the contents of the element and its borders.
The next rule uses a media query. In our media query, max-width: 600px tells our browser that the style rule should only be applied if the viewport is narrower than 600px. So, if a user views our site on a mobile device, this style will be invoked.
If our media query is executed, the display: none rule is applied to every element with the class name .betaBox
.
Multiple Media Queries Example: Font Size
In our previous example, we only specified one media query. However, you are able to specify multiple media queries in a CSS file, which means that you can apply certain styles to an element on a web page depending on whether one of the multiple conditions are met.
Suppose we are designing a website and we want the font size of our <h1> headers to change depending on the screen size. Here are the rules we want to apply to our site:
- If the width of the screen is equal to or less than 480px, the font size of our headers should be 16px.
- If the width of the screen is equal to 481px and equal to or less than 600px, the header font size should be 20px.
- If the width of the screen is equal to or greater than 601px, the header font size should be 24px.
We could use the following three media queries to change the size of the fonts on our web page depending on the size of the user’s screen:
<html> <h1>This is an example header.</h1> <html> <style> @media screen and (min-width: 480px) { h1 { font-size: 16px; } } @media screen and (min-width: 481px) and (max-width: 600px) { h1 { font-size: 20px; } } @media screen and (min-width: 601px) { h1 { font-size: 24px; } } <style>
Click the button in the code editor above to see the output of our HTML/CSS code.
Let’s break down how our code works. In our HTML file, we defined an example header using a <h1> tag which shows the text This is an example header
.
Then, in our CSS file, we defined three media queries.
The first media query checks if the size of the browser viewport is equal to or less than 480px (using max-width: 480px), and sets the font size of all <h1> elements to 16px if this statement evaluates to true.
The second media query checks if the size of the viewport is equal to or greater than 481px (using min-width: 481px) and equal to or less than 600px (using max-width: 600px). If this evaluates to true, the font size for all <h1> elements is set to 20px.
The third media query checks if the size of the viewport is greater than 601px (using min-width: 601px). If this rule evaluates to true, the font size of all <h1> elements is changed to 24px.
Media Query Breakpoints
Breakpoints are used to set the points where a website will apply a media query.
When you’re designing a media query, you may ask yourself the question: what sizes should I use to set my media queries? This is a reasonable question, because when you are just starting with responsive web design you may find it difficult to figure out the sizes of the devices on which your website should render.
There are lists on the internet that target specific screen sizes, which can be useful in setting the breakpoints for your media queries. However, due to the wide range of devices that are out there—which all have their own screen sizes and specifications—these lists usually do not cover all the main points.
The best way to choose when a media query should trigger is to look through your website for any places where elements become squashed or trimmed. So, if you see an element that is not fully visible on a mobile device, you know that you may need to add a breakpoint.
Then, once you have identified these points, you can write custom media rules. For example, you may realize that a <div> tag does not render correctly on devices whose width is less than 500px. In that case, you’ll want to write a media query to design custom styles for this resolution.
However, to get you started, here is a list of breakpoints which can help you target the main devices which may be used to visit your website:
- Most phones: @media screen and (max-width: 600px) {}
- Portrait tablets and large phones: @media screen and (min-width: 600px) {}
- Landscape tablets: @media screen and (min-width: 768px) {}
- Laptops and desktop machines: @media screen (min-width: 992px) {}
- Large laptops and desktop machines: @media screen (min-width: 1200px) {}
These rules should help you get started in determining the breakpoints for your media queries, but you may end up making a few changes to suit the particular needs of your website.
Conclusion
Media queries are used in CSS to add responsive designs to a web page. Media queries allow you to apply a specific style when a condition or set of conditions is met. For example, a media query could be used to change the font size of a web page when the width of the web page is greater than 750px.
This tutorial discussed, with reference to examples, the basics of responsive web design, how media queries work, and how you can write a media query. Now you’re ready to start working with media queries in CSS like a professional web designer.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.