Adding spaces between elements on a web page is a crucial part of designing aesthetically pleasing and functional user experiences. Margins are used to create an empty area around an HTML element to separate the element from other objects on the web page.
The CSS margin property, and its four subproperties, are used to set a margin around an element in HTML.
This tutorial will discuss, with reference and examples, how to use the margin property and its subproperties to create a margin around an element in CSS. By the end of reading this tutorial, you’ll be an expert at applying a margin to a web element using CSS.
CSS Margin
The CSS margin property is used to create space around an element. This space allows you to easily separate different elements on a web page, outside of any borders.
In CSS, the margin property is shorthand for four subproperties. These subproperties are used to set the top, right, bottom, and left margins for a web element.
Every element on a web page consists of one or more boxes. Elements use the box model to outline how the box is displayed on the web page.
Margins are one of the main components of the box model and exist on the outermost layer of the box model. In the below graphic, you can see that margins are applied outside of the borders in an HTML element:
For the purposes of this tutorial, we’ll focus on the margin property. If you’re looking to learn more about the different components of the box model, you can read our articles on CSS borders and padding.
CSS Margin Individual Sides
The following properties are used to set the margin for each side of a web element:
- margin-top
- margin-right
- margin-bottom
- margin-left
These properties allow you to define the margin for an individual side of an element. Each of these properties accepts one of four values to determine the size of the margin. They are as follows:
- length specifies a margin using px, em, or another CSS length measurement.
- percentage (%) specifies a margin as a percentage of the width of the element to which the margin is applied.
- auto instructs the browser to calculate the margin.
- inherit specifies the margin should be inherited from its parent element.
Let’s walk through an example to discuss how the margin subproperties works.
Suppose we are designing a box and we want to include margins around our box to separate it from other elements on the web page. Our box should have a top margin of 50px, a left margin of 30px, a right margin of 40px, and a bottom margin of 50px. We could create this box using the following code:
index.html <div class="outer"> <p class="box"> This is a box. </p> </div>
styles.css .box { margin-top: 50px; margin-left: 30px; margin-right: 40px; margin-bottom: 40px; border: 1px solid red; } .outer { border: 1px solid blue; }
Our code returns:
In our code, we have created two boxes. The first box, which is defined using a <div> tag, is assigned the class outer
. In our CSS file, we state that any element with the outer
class should have a 1px-wide solid blue border. We have defined this border to showcase the margin that our inner box has created.
Inside our <div> tag is a <p> tag, which contains the text This is a box
. Our <p> tag has the class name box
, which means the style for box
in our CSS file is applied to our <p> element.
Our <p> tag has been assigned a 50px top margin, 30px left margin, 40px right margin, and 40px bottom margin. We have also given our <p> tag a 1px-wide solid red border. As you can see in the above image, our margin has created a gap between the border of our <p> box and our blue box. If we had no margins, these boxes would be closer together.
CSS Margin Shorthand
In the above example, we used up four lines of code to specify the margins we wanted to apply to our box. We can make our code more efficient by using the margin property.
The margin property is shorthand for the four subproperties we discussed earlier. This property allows you to specify the margins for a box on one line of code, instead of on multiple lines.
The syntax you use for the margin shorthand depends on how many values you specify. The minimum number of values you can specify is one, and the maximum is four. Let’s break down an example of how to use the margin shorthand property in all potential use cases.
Four Values
Instead of using the individual margin subproperties to create a box with four different margin values, you can use the margin shorthand property and specify four values.
If you specify four values with the margin property, the order in which your margins will appear is as follows:
- The first value is the top margin.
- The second value is the right margin.
- The third value is the bottom margin.
- The fourth value is the left margin.
Suppose we wanted to create a box with a 25px top margin, a 25px right margin, a 50px bottom margin, and a 60px left margin. We could do so using this code:
box { margin: 25px 25px 50px 60px; border: 1px solid red; }
Our code returns:
In our example, we have created an inner box with a different margin on each edge based on the values we specified above. We have also surrounded our inner box with a 1px-wide solid red margin. In addition, we reuse the code for our blue box from earlier to illustrate the size of the margins we have applied to our box.
Three Values
If you want to have a different top and bottom margin, but use the same margin for the left and right of a box, you can use the margin property and specify three values.
The order in which the three margins you specify are applied to a box is as follows:
- The first value is the top margin.
- The second value is the margin for the right and left sides of an element.
- The third value is the bottom margin.
Let’s say we wanted to create a box with a 50px margin on the top of the box, a 25px margin on the left and right of the box, and a 60px margin on the bottom of the box. We could create a box with these margins using the following code:
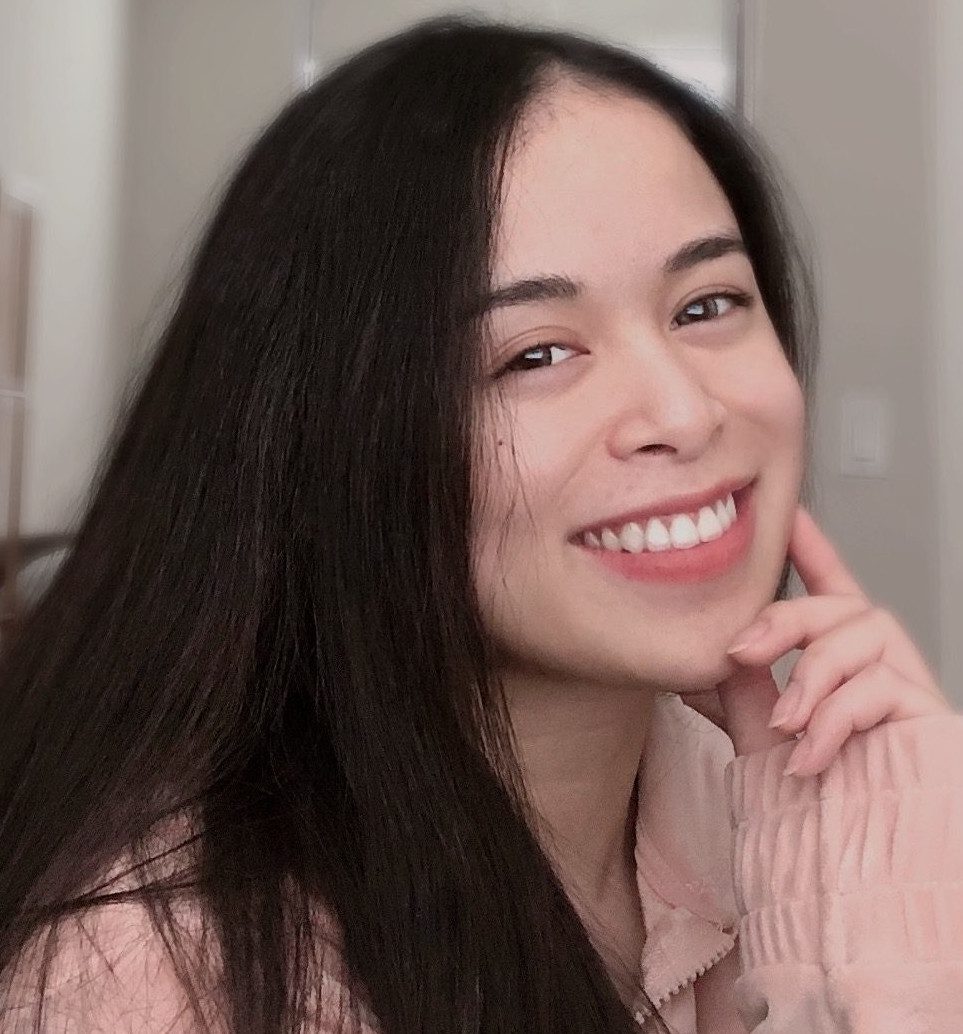
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Our code returns:
In our example, we have created an inner box with a different margin on each edge based on the values we specified above. We have also surrounded our inner box with a 1px-wide solid red margin. In addition, we reuse the code for our blue box from earlier to illustrate the size of the margins we have applied to our box.
Three Values
If you want to have a different top and bottom margin, but use the same margin for the left and right of a box, you can use the margin property and specify three values.
The order in which the three margins you specify are applied to a box is as follows:
- The first value is the top margin.
- The second value is the margin for the right and left sides of an element.
- The third value is the bottom margin.
Let’s say we wanted to create a box with a 50px margin on the top of the box, a 25px margin on the left and right of the box, and a 60px margin on the bottom of the box. We could create a box with these margins using the following code:
.box { margin: 50px 25px 60px; border: 1px solid red; }
Our code returns:
As you can see, the left and right sides of our inner box have the same margin value—25px —and the top and bottom sides have their own margins (50px and 60px, respectively).
In our code, we also defined a red border around our inner box to illustrate where our margins are applied. We also reuse the code from our first example to create a blue box which shows the gap our margins have created around the inner box.
Two Values
If you want a box to have the same margin value for the top and bottom of the box and another value for the right and left sides of the box, you can use the margin property and specify two values.
The order in which the values you specify are applied to an element is:
- The first value is the margin for the top and bottom of the box.
- The second value is the margin for the right and left sides of the box.
Let’s say you want to create a box with a 50px vertical margin and a 30px horizontal margin. You could create this box using the following CSS style:
.box { margin: 50px 30px; border: 1px solid red; }
Our code returns:
The top and bottom of our box has a 50px margin, and the left and right sides both have a 30px margin.
In our code, we also specified a red border around our inner box to showcase the size of our margins. We also reuse the code from our previous examples to create a blue box which shows the space our margins create between the inner and an outer box.
One Value
If you want every side of a box to have the same margin, you only need to specify one value when using the margin property. The value you specify will be the margin set around all borders for a box.
Suppose you want to create a box with 50px single margin around all borders. You could do so using this code:
.box { margin: 50px; border: 1px solid red; }
Our code returns:
Every border of our box has a 50px margin. We specified a red border around our box to show that our margins are applied outside the box’s border, and we also created a blue box in which our red box is placed to better illustrate our margins.
Margin inherit
The inherit value is used to inherit a margin from a parent element.
To set the margin of an inner box to be the same as the margin for an outer box, we can use the inherit value. The inherit value is useful because if you change the margin of the parent element, the margin of the child element—the box within the parent element—will automatically adjust.
Suppose we are designing a box whose parent element has a 50px left margin. We want the box contained within our parent element to have the same margin value. We could use the following code to accomplish this task:
.box { margin-left: inherit; border: 1px solid red; } .outer { margin-left: 50px; border: 1px solid blue; }
Our code returns:
Our outer box (the one with a blue border) has a left margin of 50px. In addition, our inner box (the one with a red border) has a left margin of 50px, which it inherited from its parent element.
Margin auto
The auto value is used to center an element horizontally within its container.
The auto value will make an inner element take up a certain width based on the value you have defined, then the remaining space will be divided between the left and right margins.
Let’s say that you have created a box with a 250px width inside a box with a 500px width. If you used the auto keyword to create a margin, a 125px margin would be applied to both the left and right of the box. We calculated this using the following formula:
(Outer Box Width - Inner Box Width) / 2
In this case, we used the following sum to calculate the side of our automatically-generated margins:
(500 - 250) / 2
The answer to this problem is 125, which is the margin value that will be applied to both the left and right margins of a box. Here’s an example of a box that uses these dimensions:
index.html <div class="outer"> <p class="box"> This is a box. </p> </div>
styles.css .box { width: 250px; margin: auto; border: 1px solid red; } .outer { width: 500px; border: 1px solid blue; }
Our code returns:
As you can see, an equal margin on both the left and right sides of our inner box (the one with a red border) has been created. In other words, our inner box is horizontally centered. This is because we used the margin: auto value to specify our margin for our inner box.
Our inner box does not have any margins on the top or bottom edges, because we did not specify any for our example.
Conclusion
The CSS margin property is used to create a space between the border of an element and other elements on the web page.
With reference to examples, this tutorial discussed how to use the CSS margin property, and how to use its four subproperties, to create spaces between an element’s border and other elements on a web page. Now you’re equipped with the tools you need to start using the CSS margin property like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.