Web forms are an essential part of many websites, and allow the operator of a website to accept input from a user. For example, a web form could be used to accept the email addresses of users who want to sign up to a website’s email newsletter.
By using CSS, you can add custom styles to form inputs on a web page. This allows you to create aesthetically pleasing form fields which visitors can use to submit information.
This tutorial will discuss, with examples, the basics of HTML inputs and how to use CSS to style input fields on a web page. By the end of reading this tutorial, you’ll be an expert at styling input fields using CSS.
HTML and CSS Input
To define a form on a web page, we must use HTML. The HTML language allows us to define the structure of our form—what form fields will appear, and where—then we can use CSS to apply custom styles to the elements in our form.
In HTML, the <input> tag is used to accept user input in a form. The basic syntax for a HTML <input> is:
<input type="typeOfInput">
Click the button in the code editor above to see the output of our HTML/CSS code.
If you’re interested in learning more about HTML inputs, read our beginner’s guide to HTML inputs. Here is a basic example of a form field that accepts a user’s name:
<label for="userName">Name</label> <input type="text" name="userName">
Click the button in the code editor above to see the output of our HTML/CSS code.
Our input field uses the default HTML styles for the <input> tag, which are rather basic. We also use a <label> tag to add a label to our form. To add custom designs, we can use CSS.
CSS Input
Let’s walk through a few examples of how to style form elements using CSS.
Setting the Width of an Input
Suppose we are designing a form field for a local stamp club that collects the names of people submitting the form. This form should take up 50% of the width of a web page. We could create this form using the following code:
<html> <label for="userName">Name</label> <input type="text" name="userName"> <style> input { width: 50%; }
Click the button in the code editor above to see the output of our HTML/CSS code.
In this example, we have created a form field that is equal to 50% of the width of the web page.
In our HTML code, we used the <label> tag to add the label Name
to our form. Then we used an <input> tag to define our form. Then, in our CSS code, we set the width of all <input> tags to be equal to 50% of their parent container. In this case, because the parent container is the web page, our <input> tag takes up 50% of the width of our site.
Input with Border
The Seattle Stamp Club has asked us to add a light blue border around each form field because light blue is their club’s color. The border should have a width of 3px. We could use the border property to add a border around each form input field.
Here is the code we would use to add borders around our input field:
<html> <label for="userName">Name</label> <input type="text" name="userName"> <style> input { border: 3px solid lightblue; }
Click the button in the code editor above to see the output of our HTML/CSS code.
In our code, we use the border style to add a border around our input field. This border is 3px thick. As you can see, our form field has a light blue border.
If you’re interested in learning more about CSS borders, read our ultimate guide to CSS borders.
Input with Bottom Border
In addition, we can also add a border to a specific edge of our form. So, if we only want a border to appear at the bottom of a field, we could use the border-bottom property. Here is the code we would use:
<html> <label for="userName">Name</label> <input type="text" name="userName"> <style> input { border-bottom: 3px solid lightblue; }
Click the button in the code editor above to see the output of our HTML/CSS code.
In our code, we use the border-bottom property to add a border to the bottom of our input field.
Input with Padding
The Seattle Stamp Club has asked us to make space appear between the contents of the form—the space where the user enters information—and the border of the form.
The Club wants a 10px padding to appear between the top and bottom borders and the content of the form. The Club also wants a 15px padding to appear between the left and right borders and the content of the form.
We could accomplish this task using the following code:
<html> <label for="userName">Name</label> <input type="text" name="userName"> <style> input { padding: 10px 15px; box-sizing: border-box; }
Click the button in the code editor above to see the output of our HTML/CSS code.
In this example, we used the padding style to apply padding between the contents of our form field and the borders of the form field. The first value (10px) sets the padding for the top and bottom edges, and the second value (15px) sets the padding for the left and right edges.
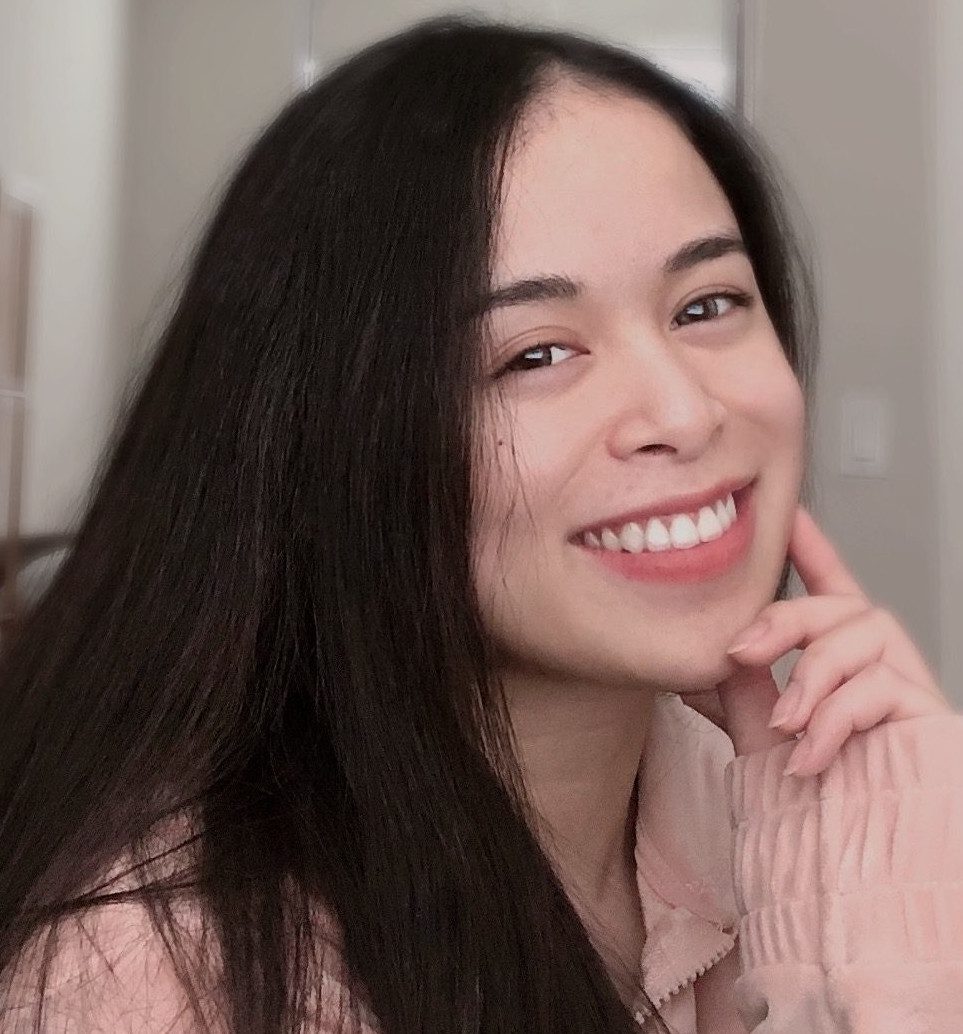
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
We have also set the value of the box-sizing property to border-box
. This ensures the padding is included in the total width of the elements.
If you’re interested in learning more about the CSS padding property, read our guide to CSS padding.
Styles with Focused Input
In most browsers, when you click on a form field, a blue outline will be added to the field. This feature is used to highlight the form you are currently focused on.
However, we can customize this by using the :focus CSS selector. The Seattle Stamp Club has asked us to add a light gray border to the bottom of our form when the user clicks on the form. We could do so using this code:
<html> <label for="userName">Name</label> <input type="text" name="userName"> <style> input:focus { outline: none; border-bottom: 3px solid lightgray; }
Click the button in the code editor above to see the output of our HTML/CSS code.
The webform appears using the default HTML styles. However, when you click on the form, a 3px-wide solid light gray border is applied to the bottom of our form.
This is because we have used a :focus selector, which allows us to apply a style when the user clicks on the input field. We also used the outline: none rule, which stops the default blue outline from appearing when the user clicks on the form input field.
To learn more about the focus selector, read our guide to the CSS :focus selector.
Input with Rounded Corners
The Seattle Stamp Club has asked us to add a light gray border around every edge of the input field, and round the corners of the inputs. We could do so using the border-radius property, which allows you to create a rounded corners
effect in CSS.
Here is the code we would use to create a form field with rounded corners:
<html> <label for="userName">Name</label> <input type="text" name="userName"> <style> input { border: 3px solid lightgray; border-radius: 10px; }
Click the button in the code editor above to see the output of our HTML/CSS code.
In this example, we define a 3px-wide solid light gray border that appears around our input field. Then, we use the border-radius property to round the corners of our form field.
If you’re interested in learning more about how to apply rounded corners to an HTML element on a web page, read our guide to CSS rounded corners.
Input with Background Color
The Seattle Stamp Club has asked us to make one final form field. This form field should have a 3px-wide solid light gray border and have a light blue background.
We could use the following code to create this form field:
<html> <label for="userName">Name</label> <input type="text" name="userName"> <style> input { border: 3px solid lightgray; background-color: lightblue; }
Click the button in the code editor above to see the output of our HTML/CSS code.
In our code, we used the border property to define a 3px-wide solid light gray border around our input fields. Then, we used the background-color property to set the background color of our form field to light blue.
Apply Styles to Specific Input Fields
In this tutorial, we have discussed how to apply styles to <input> fields. However, there is a way that you can apply styles to only a specific input type.
That’s where attribute selectors come in. Instead of using input
in your styles, if you want to apply styles to only a specific input, you could try to use one of these selectors:
- input[type=text]: Applies a style to all text fields.
- input[type=email]: Applies a style to all email fields.
- input[type=password]: Applies a style to all password fields.
- input[id=userName]: Applies a style to the element with the ID
userName
.
To learn more about how attribute selectors work, read our Career Karma guide to CSS attribute selectors.
Conclusion
By using CSS, you can create custom-designed web forms that are both aesthetically pleasing and functional. First, you use HTML to design the structure of a form, then you can use CSS styles to apply styles to the form.
This tutorial discussed, with reference to examples, the basics of HTML inputs and how you can use CSS to style input fields. Now you’re equipped with the knowledge you need to style your input fields like an expert using CSS!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.