When designing a website, you’ll often have a button you want to look a certain way. For instance, you may want a button to have a different background color than other page elements to attract the user’s attention to the button.
Using CSS, developers can create styled buttons. CSS allows you to change a button’s color, text size, border, width, and height, among other things.
This tutorial will discuss, with examples, how to use a number of CSS properties to style an HTML button. By the end of reading this tutorial, you’ll be an expert at styling buttons using CSS.
Overview of Buttons
You can define a button in a number of different ways in HTML. The two most common approaches are the <button>
tag or an <input type=“button”>
element. These approaches return the same type of button.
HTML code is used to define a button. Here’s what an HTML button looks like without any styling:
The code we used for this button is:
<button>Click me</button>
This button uses default HTML styles, which means that right now the button looks rather plain.
If we want to style our button—in other words, to customize how it looks on the page—we need to use CSS. In this article, we will discuss how to do the following to a button:
- Change the background color.
- Change the text color.
- Change the text size.
- Add padding.
- Round the corners.
- Add a colored border.
- Use the :hover feature.
- Add a shadow.
- Change the width.
- Disable it.
- Animate it.
We will also discuss how to create horizontal and vertical button groups.
Background Color
When you’re designing a button, one of the first things you may want to do is set a background color for the button. By default, a button has a white background and black text. Using the CSS background-color property, we can change a button’s background color.
Suppose we are designing a button for a website and want the button to have a pink background. We can change the color of our button using the following CSS code:
index.html <button class="button">Click me</button> styles.css .button { width: 100px; height: 50px; background-color: pink; }
Our code returns:
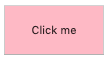
Using the background-color property, we changed the background color of our button to pink. We can change the background color of our button to any color using this property.
Text Color
To change the text color of a button, you can use the color property. Suppose we are designing a button and we want the button to have a pink background and green text. We could create this button using the following code:
index.html <button class="button">Click me</button> styles.css .button { width: 100px; height: 50px; color: green; background-color: pink; }
Our code returns:
In our code, we use the color: green; property to set the color of the text in our button to green.
Text Size
You can use the CSS font-size property to define how large the text inside a button will be. Suppose we want the text to have a font size of 20px. We can make this happen using the following code:
index.html <button class="button">Click me</button> styles.css .button { width: 100px; height: 50px; background-color: blue; color: white; font-size: 20px; }
Our code returns:
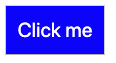
Our button is the same size as before, but now the size of the font inside of it is 20px. We used the font-size attribute to set the size of the text in our button.
Padding
When designing a button, you may decide you want a certain amount of space between the text inside a button and the button’s outer walls. That’s where the padding property comes in.
The padding property uses length indicators (px, em, and so on) or percentage values to define a certain amount of space between a button’s text and its borders.
Suppose we want to create two buttons on our page. One button should have a 40px padding, and the other should have a 20px padding. We can create these buttons using the following code:
index.html <button class="button1">Click me</button> <button class="button2">Click me</button> styles.css .button1 { padding: 20px; font-size: 16px; background-color: blue; color: white; } .button2 { padding: 40px; font-size: 16px; background-color: blue; color: white; }
Our code returns:
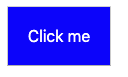
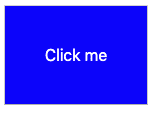
Our first button has a 20px boundary between the text inside the button and the button’s borders. Our second button has a 40px boundary between the button’s text and borders. For these buttons, to ensure there is sufficient space for this padding, we do not specify width or height attributes. (We will discuss these attributes below.)
Rounded Corners
By default, buttons in HTML have square edges. However you may decide you want your buttons to have rounded corners.
You can use the border-radius property to create rectangular buttons with rounded corners. Below are three examples of code utilizing the border-radius property. To illustrate the effect of various radius sizes, each example uses a border radius of a different size.
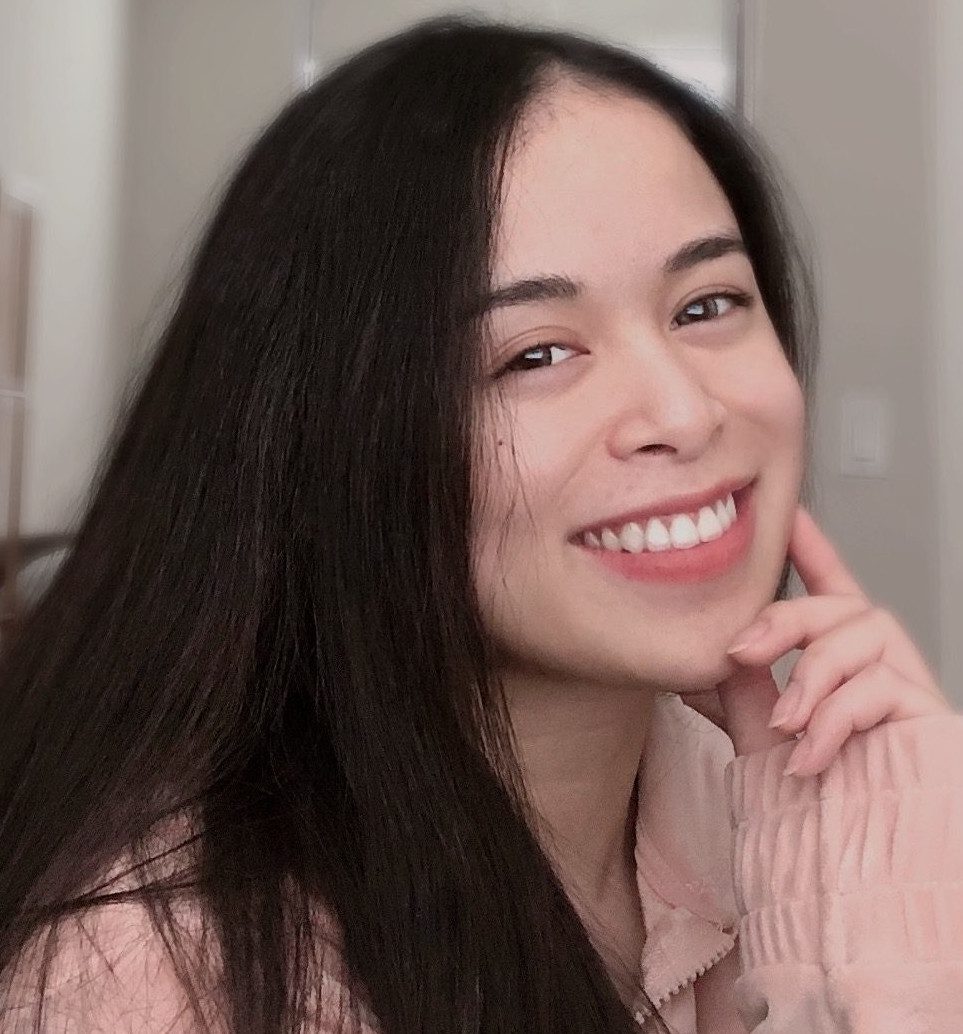
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
index.html <button class="button1">Click me</button> <button class="button2">Click me</button> <button class="button3">Click me</button> styles.css .button1 { width: 100px; height: 50px; background-color: blue; color: white; border-radius: 5px; } .button2 { width: 100px; height: 50px; background-color: blue; color: white; border-radius: 10px; } .button3 { width: 100px; height: 50px; background-color: blue; color: white; border-radius: 15px; }
Our code returns:
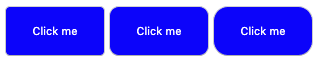
In this example, we created three buttons. The larger the border radius of a button, the more rounded the button’s corners appear.
Border Color
You can add a custom colored border to a button with the CSS border property.
Suppose you want to create a button with a colored border so the boundaries of the button are clear to the user. You can create such a button using the following code:
index.html <button class="button">Click me</button> styles.css .button { background-color: white; color: black; border: 3px solid #ffb6c1; // Light pink padding: 25px; }
Our code returns:
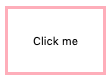
Our button has a white background, its contents are black, and it has a 25px padding on all edges. We used the border property to create a 3px-wide solid border around our button, and we set the color of our border to #ffb6c1, which is the hexadecimal value for light pink.
If you’re looking to learn more about the border property, read our article on the CSS border property.
Hover
You can use the CSS :hover selector to direct a web page to change the style of a button when a user hovers over the button with their cursor.
Suppose we are designing a button with black text and a white background, and we want its text color to change to white and its background color to change to green when the user hovers over it. We can accomplish this task using the following code:
index.html <button class="button">Click me</button> styles.css .button { background-color: white; color: black; border: 3px solid #ffb6c1; padding: 25px; } .button:hover { background-color: green; color: white; }
Here’s the result of our code:
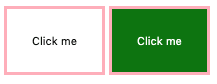
The first button in the above diagram shows how our button appears when the user is not hovering over the button. As you can see, the code returns a white button with black text, a pink border, and 25px padding. This is the default state of the button. But when the user hovers over the button, the background color of the button changes to green, and the color of the text in the button changes to white.
As soon as the user’s cursor moves away from the button, the button will return to its default state.
In addition, we can use the transition-duration attribute to specify how long it should take for the button to change after a user hovers over it with their cursor. So, if we want it to take 1 second for the above button to change (to having a green background and white text), we can use this code:
.button { … transition-duration: 1s; }
Now when the user hovers over the button, it will take one second for the button to change. You can learn more about CSS transitions in our beginner’s guide to CSS transitions.
Shadows
You can use the CSS box-shadow property to create a button shadow. The box-shadow property takes in several parameters. You can learn about these parameters in our CSS box-shadow tutorial.
Let’s say we are designing a button and we want it to have a drop shadow. We can create this shadow using the following code for the button:
index.html <button class="button">Click me</button> styles.css .button { background-color: white; color: black; border: 3px solid #ffb6c1; padding: 25px; box-shadow: 0 10px 10px 0 rgba(0,0,0,0.2); }
Our code returns:
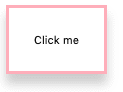
In this example, we added a shadow to our button. The parameters we specified for our shadow are as follows:
- The x-offset is 0, which means our shadow appears directly under our button.
- The y-offset is 10px, which means our shadow extends below our button by 10px.
- The blur radius is 10px, which means our shadow has a blur effect.
- The spread radius for our button’s shadow is 0, so there is no spread around the blur.
- The color of our shadow is rgba(0,0,0,0.2), which is light gray.
As you can see in the code result above, our button appears with all of these properties.
Width
The width property can define the width of a button. By default, the size of a button is based on its text content, but you can use the width property to override the default and set a specific width.
Here’s an example of three buttons with different widths:
index.html <button class="button1">Click me</button> <button class="button2">Click me</button> <button class="button3">Click me</button> styles.css .button1 { background-color: white; color: black; border: 3px solid #ffb6c1; padding: 25px; width: 250px; } .button2 { background-color: white; color: black; border: 3px solid #ffb6c1; padding: 25px; width: 50%; } .button2 { background-color: white; color: black; border: 3px solid #ffb6c1; padding: 25px; width: 100%; }
Our code returns:
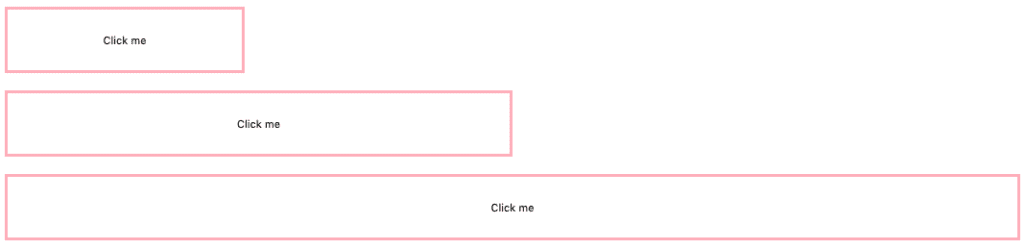
In our example, we created three buttons with different widths.
Disabling a Button
When designing a button, you may decide to disable it but keep it visible on the page. For instance, you may want a button to be disabled until a user fills out all the fields in a web form.
By using the opacity and cursor properties, you can create a disabled button. The opacity property adds transparency to a button. This makes it look like it is disabled. The default opacity is 1, which displays a color with no transparency. You can learn more about the CSS opacity property in our guide to CSS opacity.
The cursor property, when assigned the value “not-allowed”, disables the button. When the user hovers over a button with this value assigned to it, the cursor will show a stop sign.
Here’s the code for a normal button in HTML followed by the code for a disabled button:
index.html <button class="button1">Click me</button> <button class="button2">Click me</button> styles.css .button1 { background-color: #ffb6c1; color: black; padding: 25px; } .button2 { background-color: #ffb6c1; color: black; padding: 25px; border: none; opacity: 0.5; cursor: not-allowed; }
Our code returns:
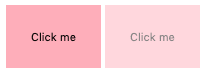
Both buttons have pink #ffb6c1
backgrounds. The background of the first button is fully opaque, which is the default; this button is functional (which is the default). The second button has the opacity property set to 0.5
and the value of the cursor property set to not-allowed
. This creates a more transparent, nonfunctional (disabled) button.
Creating Button Groups
When you’re developing a site, you may want to create a group of buttons. For instance, if you are designing a form, you may want to have a button group with three buttons that allow a user to save the form, go back, or go to the next page of the form.
Horizontal Button Group
If you want buttons to appear side by side, you need to create a CSS button group. You can create a button group by floating the buttons you want to appear in your group to the left. You can learn more about the CSS float property in our guide to CSS floating. Here’s an example of code for a horizontal button group:
index.html <button class="button">Click me</button> <button class="button">Click me</button> <button class="button">Click me</button> styles.css .button { background-color: #ffb6c1; color: black; padding: 25px; border: none; float: left; }
Our code returns:
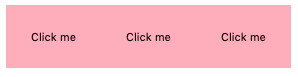
As you can see, we created three buttons. These appear side by side.
Similarly, we can specify a border CSS property to create a group of buttons that each have their own borders. Here’s the code we can use to create a button group with borders:
index.html <button class="button">Click me</button> <button class="button">Click me</button> <button class="button">Click me</button> styles.css .button { background-color: #ffb6c1; color: black; padding: 25px; border: 2px solid blue; float: left; }
Our code returns:
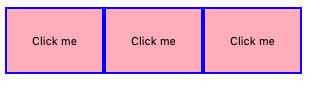
In our code, we specified a 2px solid blue border around each of our buttons. This allows us to visually distinguish each button in our group.
Vertical Button Group
Often, when you’re designing a button group, you may want buttons to appear vertically. That’s where the display: block CSS property comes in.
Here’s an example that uses display: block to display the buttons in a CSS button group vertically:
index.html <button class="button">Click me</button> <button class="button">Click me</button> <button class="button">Click me</button> styles.css .button { background-color: #ffb6c1; color: black; padding: 25px; border: 2px solid blue; display: block; }
Our code returns:
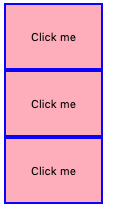
Our web browser groups these buttons together and displays them vertically.
Animating Buttons
When you’re designing a button, you may decide to make it more interactive by animating it. Below we discuss a few ways to apply animations to a button using CSS.
Pressed
In CSS, you can create an effect that makes it look like a button is pressed down when the user clicks on it.
For example, let’s say you want a light blue button with a dark gray shadow to turn pink with a light gray shadow 0.5 seconds after the user clicks on it. This will give the user a sense that they are physically pressing a button. Here’s the code you can use to accomplish this task:
index.html <button class="button">Click me</button> styles.css .button { padding: 20px; font-size: 15px; color: white; background-color: lightblue; border: none; transition-duration: 0.5s; border-radius: 8px; box-shadow: 0 5px gray; } .button:active { background-color: #ffb6c1; box-shadow: 0 5px lightgray; transform: translateY(5px); }
Here’s the result of our code:
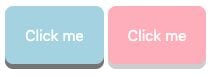
On the left you can see how our button appears before it is clicked. The button is light blue and has a dark gray box shadow. On the right, you can see the appearance of our button after it is clicked (and after the specified transition duration passes). The button has a pink background and a light gray box shadow.
Let’s break down our code. In our .button style, we:
- Create a 20px padding around our button.
- Set the font size of our button’s text to 15px.
- Set the color of our button’s text to white.
- Set the color of our button to light blue.
- Removed the default border around our button.
- Set the duration of our hover transition to 0.5 seconds.
- Set a border-radius of 8px, which creates rounded corners for our button
- Set a gray box shadow that is 5px long and appears below our button.
These set the styles for our button when the user is not hovering over the button with their cursor. In our .button:active style, we:
- Set the background color of the button to light pink.
- Set a light gray shadow that is 5px long and appears below our button.
- Set a translateY() transformation which makes our button appear pressed. You can learn more about this transition in the Career Karma CSS 2D transforms guide.
These styles allow us to create an effect that triggers when the user hovers over the button.
Fade-In
We can use the transition and opacity properties to create a fade-in effect on a button. When combined with the :hover selector, the fade-in effect activates when the user hovers over an object—in this case, a button.
Here’s the code we can use to create a fade-in button:
.button { padding: 20px; font-size: 15px; color: white; background-color: lightblue; border: none; -webkit-transition-duration: 0.5s; transition-duration: 0.5s; border-radius: 8px; opacity: 0.7; } .button:hover { opacity: 1; }
Here’s the result of our code:
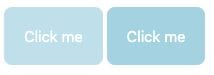
On the left, you can see that our button appears more transparent. This is the result of setting the value of the opacity property to 0.7.
After the user hovers over the button, the contents of the .button:hover CSS property are read, which sets the opacity of the button to 1. This makes our button fully opaque (not transparent).
Conclusion
HTML defines the structure of a button on a web page. Once you define a button, you can then use CSS to customize it based on your needs.
This tutorial discussed, with examples, how to style a button using a number of popular CSS properties. Now you’re ready to start styling buttons using CSS like a professional web developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.